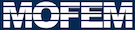 |
| v0.14.0
|
Go to the documentation of this file.
7 template <
class T,
int Tensor_Dim0,
int Tensor_Dim12>
11 data[Tensor_Dim0][(Tensor_Dim12 * (Tensor_Dim12 - 1)) / 2];
16 static_assert(
sizeof...(
d) ==
sizeof(data) /
sizeof(T),
17 "Incorrect number of Arguments. Constructor should "
18 "initialize the entire Tensor");
33 T &
unsafe(
const int N1,
const int N2,
const int N3)
36 if(N1 >= Tensor_Dim0 || N1 < 0 || N2 >= Tensor_Dim12 || N2 < 0
37 || N3 >= Tensor_Dim12 || N3 < 0 || N2 >= N3)
40 s <<
"Bad index in Tensor3_antisymmetric<T*," << Tensor_Dim0 <<
","
41 << Tensor_Dim12 <<
">.unsafe(" << N1 <<
"," << N2 <<
"," << N3
43 throw std::out_of_range(s.str());
46 return *data[N1][N3 - 1 + (N2 * (2 * (Tensor_Dim12 - 1) - N2 - 1)) / 2];
49 T
operator()(
const int N1,
const int N2,
const int N3)
const
52 if(N1 >= Tensor_Dim0 || N1 < 0 || N2 >= Tensor_Dim12 || N2 < 0
53 || N3 >= Tensor_Dim12 || N3 < 0)
56 s <<
"Bad index in Tensor3_antisymmetric<T*," << Tensor_Dim0 <<
","
57 << Tensor_Dim12 <<
">.operator(" << N1 <<
"," << N2 <<
"," << N3
58 <<
") const" << std::endl;
59 throw std::out_of_range(s.str());
64 [N3 - 1 + (N2 * (2 * (Tensor_Dim12 - 1) - N2 - 1)) / 2]
67 + (N3 * (2 * (Tensor_Dim12 - 1) - N3 - 1)) / 2]
71 T *
ptr(
const int N1,
const int N2,
const int N3)
const
74 if(N1 >= Tensor_Dim0 || N1 < 0 || N2 >= Tensor_Dim12 || N2 < 0
75 || N3 >= Tensor_Dim12 || N3 < 0)
78 s <<
"Bad index in Tensor3_antisymmetric<T*," << Tensor_Dim0 <<
","
79 << Tensor_Dim12 <<
">.ptr(" << N1 <<
"," << N2 <<
"," << N3 <<
")"
81 throw std::out_of_range(s.str());
85 ? data[N1][N3 - 1 + (N2 * (2 * (Tensor_Dim12 - 1) - N2 - 1)) / 2]
88 + (N3 * (2 * (Tensor_Dim12 - 1) - N3 - 1)) / 2]
95 template <
char i,
char j,
char k,
int Dim0,
int Dim12>
107 template <
char i,
char j,
char k,
int Dim0,
int Dim12>
116 Dim12,
i,
j,
k>(*this);
125 for(
int i = 0;
i < Tensor_Dim0; ++
i)
126 for(
int j = 0;
j < (Tensor_Dim12 * (Tensor_Dim12 - 1)) / 2; ++
j)
T operator()(const int N1, const int N2, const int N3) const
const Tensor1_Expr< const dTensor0< T, Dim, i >, typename promote< T, double >::V, Dim, i > d(const Tensor0< T * > &a, const Index< i, Dim > index, const Tensor1< int, Dim > &d_ijk, const Tensor1< double, Dim > &d_xyz)
Tensor3_antisymmetric_Expr< Tensor3_antisymmetric< T *, Tensor_Dim0, Tensor_Dim12 >, T, Dim0, Dim12, i, j, k > operator()(const Index< i, Dim0 > index1, const Index< j, Dim12 > index2, const Index< k, Dim12 > index3)
FTensor::Index< 'i', SPACE_DIM > i
Tensor3_antisymmetric(U *... d)
T & unsafe(const int N1, const int N2, const int N3)
const Tensor3_antisymmetric< T *, Tensor_Dim0, Tensor_Dim12 > & operator++() const
FTensor::Index< 'j', 3 > j
FTensor::Index< 'k', 3 > k
Tensor3_antisymmetric_Expr< const Tensor3_antisymmetric< T *, Tensor_Dim0, Tensor_Dim12 >, T, Dim0, Dim12, i, j, k > operator()(const Index< i, Dim0 > index1, const Index< j, Dim12 > index2, const Index< k, Dim12 > index3) const
T * ptr(const int N1, const int N2, const int N3) const