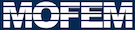 |
| v0.14.0
|
Go to the documentation of this file.
6 #ifndef __DEFINITONS_H__
7 #define __DEFINITONS_H__
12 #define DEPRECATED __attribute__((deprecated))
13 #elif defined(_MSC_VER)
14 #define DEPRECATED __declspec(deprecated)
16 #pragma message("WARNING: You need to implement DEPRECATED for this compiler")
46 "MOFEM_DATA_INCONSISTENCY",
47 "MOFEM_NOT_IMPLEMENTED",
49 "MOFEM_OPERATION_UNSUCCESSFUL",
50 "MOFEM_IMPOSSIBLE_CASE",
52 "MOFEM_MOFEMEXCEPTION_THROW",
53 "MOFEM_STD_EXCEPTION_THROW",
54 "MOFEM_ATOM_TEST_INVALID",
74 "AINSWORTH_LEGENDRE_BASE",
75 "AINSWORTH_LOBATTO_BASE",
76 "AINSWORTH_BERNSTEIN_BEZIER_BASE",
77 "DEMKOWICZ_JACOBI_BASE",
93 "NOSPACE",
"NOFIELD",
"H1",
"HCURL",
"HDIV",
"L2",
"LASTSPACE"};
109 "Cylindrical",
"Spherical"};
176 "UNKNOWNSET",
"NODESET",
"SIDESET",
"BLOCKSET",
177 "MATERIALSET",
"DISPLACEMENTSET",
"FORCESET",
"PRESSURESET",
178 "VELOCITYSET",
"ACCELERATIONSET",
"TEMPERATURESET",
"HEATFLUXSET",
179 "INTERFACESET",
"UNKNOWNNAME",
"MAT_ELASTICSET",
"MAT_INTERFSET",
180 "MAT_THERMALSET",
"BODYFORCESSET",
"MAT_MOISTURESET",
"DIRICHLET_BC",
181 "NEUMANN_BC",
"LASTSET_BC"};
215 #define MYPCOMM_INDEX 0
217 #define MAX_CORE_TMP 1
218 #define BITREFEDGES_SIZE 32
219 #define BITREFLEVEL_SIZE 64
220 #define BITFIELDID_SIZE 32
221 #define BITFEID_SIZE 32
222 #define BITPROBLEMID_SIZE 32
223 #define BITINTERFACEUID_SIZE 32
226 #define MB_TYPE_WIDTH 4
227 #define MB_ID_WIDTH (8 * sizeof(EntityHandle) - MB_TYPE_WIDTH)
228 #define MB_TYPE_MASK ((EntityHandle)0xF << MB_ID_WIDTH)
231 #define MB_START_ID ((EntityID)1)
233 ((EntityID)MB_ID_MASK)
234 #define MB_ID_MASK (~MB_TYPE_MASK)
236 #define MAX_DOFS_ON_ENTITY 512
237 #define MAX_PROCESSORS_NUMBER 1024
238 #define DOF_UID_MASK \
239 (MAX_DOFS_ON_ENTITY - 1)
240 #define ENTITY_UID_MASK (~DOF_UID_MASK)
242 #define NOT_USED(x) ((void)(x))
251 #define BARRIER_PCOMM_RANK_START(PCMB) \
253 for (unsigned int i = 0; i < PCMB->proc_config().proc_rank(); i++) \
254 MPI_Barrier(PCMB->proc_config().proc_comm()); \
259 #define BARRIER_RANK_START(PCMB) \
261 macro_is_deprecated_using_deprecated_function(); \
262 for (unsigned int i = 0; i < PCMB->proc_config().proc_rank(); i++) \
263 MPI_Barrier(PCMB->proc_config().proc_comm()); \
273 #define BARRIER_PCOMM_RANK_END(PCMB) \
275 for (unsigned int i = PCMB->proc_config().proc_rank(); \
276 i < PCMB->proc_config().proc_size(); i++) \
277 MPI_Barrier(PCMB->proc_config().proc_comm()); \
282 #define BARRIER_RANK_END(PCMB) \
284 macro_is_deprecated_using_deprecated_function(); \
285 for (unsigned int i = PCMB->proc_config().proc_rank(); \
286 i < PCMB->proc_config().proc_size(); i++) \
287 MPI_Barrier(PCMB->proc_config().proc_comm()); \
297 #define BARRIER_MOFEM_RANK_START(MOFEM) \
299 for (int i = 0; i < (MOFEM)->get_comm_rank(); i++) \
300 MPI_Barrier((MOFEM)->get_comm()); \
310 #define BARRIER_MOFEM_RANK_END(MOFEM) \
312 for (int i = (MOFEM)->get_comm_rank(); i < (MOFEM)->get_comm_size(); i++) \
313 MPI_Barrier((MOFEM)->get_comm()); \
346 #define MoFEMFunctionBegin \
347 PetscFunctionBegin; \
372 #define CATCH_ERRORS \
373 catch (MoFEMExceptionInitial const &ex) { \
374 return PetscError(PETSC_COMM_SELF, ex.lINE, PETSC_FUNCTION_NAME, __FILE__, \
375 ex.errorCode, PETSC_ERROR_INITIAL, ex.what()); \
377 catch (MoFEMExceptionRepeat const &ex) { \
378 return PetscError(PETSC_COMM_SELF, ex.lINE, PETSC_FUNCTION_NAME, __FILE__, \
379 ex.errorCode, PETSC_ERROR_REPEAT, " "); \
381 catch (MoFEMException const &ex) { \
382 SETERRQ(PETSC_COMM_SELF, ex.errorCode, ex.errorMessage); \
384 catch (boost::bad_weak_ptr & ex) { \
385 std::string message("Boost bad weak ptr: " + std::string(ex.what()) + \
386 " at " + boost::lexical_cast<std::string>(__LINE__) + \
387 " : " + std::string(__FILE__) + " in " + \
388 std::string(PETSC_FUNCTION_NAME)); \
389 SETERRQ(PETSC_COMM_SELF, MOFEM_STD_EXCEPTION_THROW, message.c_str()); \
391 catch (std::out_of_range & ex) { \
392 std::string message("Std out of range error: " + std::string(ex.what()) + \
393 " at " + boost::lexical_cast<std::string>(__LINE__) + \
394 " : " + std::string(__FILE__) + " in " + \
395 std::string(PETSC_FUNCTION_NAME)); \
396 SETERRQ(PETSC_COMM_SELF, MOFEM_STD_EXCEPTION_THROW, message.c_str()); \
398 catch (std::exception const &ex) { \
399 std::string message("Std error: " + std::string(ex.what()) + " at " + \
400 boost::lexical_cast<std::string>(__LINE__) + " : " + \
401 std::string(__FILE__) + " in " + \
402 std::string(PETSC_FUNCTION_NAME)); \
403 SETERRQ(PETSC_COMM_SELF, MOFEM_STD_EXCEPTION_THROW, message.c_str()); \
416 #define MoFEMFunctionReturn(a) \
419 PetscFunctionReturn(a)
440 #define MoFEMFunctionBeginHot PetscFunctionBeginHot
447 #define MoFEMFunctionReturnHot(a) PetscFunctionReturn(a)
449 #define CHKERRQ_PETSC(n) CHKERRQ(n)
454 #define CHKERRQ_MOAB(a) \
455 if (PetscUnlikely(MB_SUCCESS != (a))) { \
456 std::string error_str = (unsigned)(a) <= (unsigned)MB_FAILURE \
457 ? moab::ErrorCodeStr[a] \
458 : "INVALID ERROR CODE"; \
459 std::string str("MOAB error (" + boost::lexical_cast<std::string>((a)) + \
460 ") " + error_str + " at line " + \
461 boost::lexical_cast<std::string>(__LINE__) + " : " + \
462 std::string(__FILE__)); \
463 SETERRQ(PETSC_COMM_SELF, MOFEM_MOAB_ERROR, str.c_str()); \
484 if ((boost::is_same<BOOST_TYPEOF((n)), \
485 MoFEMErrorCodeGeneric<PetscErrorCode>>::value)) { \
486 CHKERRQ_PETSC((n)); \
487 } else if (boost::is_same<BOOST_TYPEOF((n)), \
488 MoFEMErrorCodeGeneric<moab::ErrorCode>>::value) { \
535 #define CHKERR MoFEM::ErrorChecker<__LINE__>() <<
541 #define MOAB_THROW(err) \
543 if (PetscUnlikely(MB_SUCCESS != (err))) { \
544 std::string error_str = (unsigned)(err) <= (unsigned)MB_FAILURE \
545 ? moab::ErrorCodeStr[err] \
546 : "INVALID ERROR CODE"; \
547 throw MoFEMException(MOFEM_MOAB_ERROR, \
549 boost::lexical_cast<std::string>((err)) + ") " + \
550 error_str + " at line " + \
551 boost::lexical_cast<std::string>(__LINE__) + \
552 " : " + std::string(__FILE__)) \
561 #define THROW_MESSAGE(msg) \
563 throw MoFEM::MoFEMException( \
564 MOFEM_MOFEMEXCEPTION_THROW, \
565 ("MoFEM error " + boost::lexical_cast<std::string>((msg)) + \
566 " at line " + boost::lexical_cast<std::string>(__LINE__) + " : " + \
567 std::string(__FILE__)) \
576 #define CHK_MOAB_THROW(err, msg) \
578 if (PetscUnlikely(static_cast<int>(MB_SUCCESS) != (err))) \
581 throw MoFEMException( \
583 ("MOAB error (" + boost::lexical_cast<std::string>((err)) + ") " + \
584 boost::lexical_cast<std::string>((msg)) + " at line " + \
585 boost::lexical_cast<std::string>(__LINE__) + " : " + \
586 std::string(__FILE__)) \
596 #define CHK_THROW_MESSAGE(err, msg) \
598 if (PetscUnlikely((err) != MOFEM_SUCCESS)) \
599 THROW_MESSAGE(msg); \
606 #define SSTR(x) toString(x)
608 #define TENSOR1_VEC_PTR(VEC) &VEC[0], &VEC[1], &VEC[2]
610 #define SYMMETRIC_TENSOR4_MAT_PTR(MAT) \
611 &MAT(0, 0), &MAT(0, 1), &MAT(0, 2), &MAT(0, 3), &MAT(0, 4), &MAT(0, 5), \
612 &MAT(1, 0), &MAT(1, 1), &MAT(1, 2), &MAT(1, 3), &MAT(1, 4), &MAT(1, 5), \
613 &MAT(2, 0), &MAT(2, 1), &MAT(2, 2), &MAT(2, 3), &MAT(2, 4), &MAT(2, 5), \
614 &MAT(3, 0), &MAT(3, 1), &MAT(3, 2), &MAT(3, 3), &MAT(3, 4), &MAT(3, 5), \
615 &MAT(4, 0), &MAT(4, 1), &MAT(4, 2), &MAT(4, 3), &MAT(4, 4), &MAT(4, 5), \
616 &MAT(5, 0), &MAT(5, 1), &MAT(5, 2), &MAT(5, 3), &MAT(5, 4), &MAT(5, 5)
618 #define TENSOR4_MAT_PTR(MAT) &MAT(0, 0), MAT.size2()
620 #define TENSOR2_MAT_PTR(MAT) \
621 &MAT(0, 0), &MAT(1, 0), &MAT(2, 0), &MAT(3, 0), &MAT(4, 0), &MAT(5, 0), \
622 &MAT(6, 0), &MAT(7, 0), &MAT(8, 0)
624 #define SYMMETRIC_TENSOR2_MAT_PTR(MAT) \
625 &MAT(0, 0), &MAT(0, 1), &MAT(0, 2), &MAT(0, 3), &MAT(0, 4), &MAT(0, 5)
627 #define SYMMETRIC_TENSOR2_VEC_PTR(VEC) \
628 &VEC[0], &VEC[1], &VEC[2], &VEC[3], &VEC[4], &VEC[5]
630 #endif //__DEFINITONS_H__
const static char *const CubitBCNames[]
Names of types of sets and boundary conditions.
@ MOFEM_STD_EXCEPTION_THROW
const static char *const ApproximationBaseNames[]
@ L2
field with C-1 continuity
HVecFormatting
Format in rows of vectorial base functions.
CoordinateTypes
Coodinate system.
@ USER_BASE
user implemented approximation base
FieldSpace
approximation spaces
const static char *const CoordinateTypesNames[]
Coordinate system names.
@ MAT_THERMALSET
block name is "MAT_THERMAL"
VERBOSITY_LEVELS
Verbosity levels.
@ BODYFORCESSET
block name is "BODY_FORCES"
@ MAT_ELASTICSET
block name is "MAT_ELASTIC"
@ LASTSPACE
FieldSpace in [ 0, LASTSPACE )
HVecDiffFormatting
Format in rows of vectorial base gradients of base functions.
MoFEMErrorCodes
Error handling.
@ MOFEM_OPERATION_UNSUCCESSFUL
@ MOFEM_MOFEMEXCEPTION_THROW
@ AINSWORTH_BERNSTEIN_BEZIER_BASE
const static char *const FieldSpaceNames[]
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
@ HCURL
field with continuous tangents
@ MOFEM_DATA_INCONSISTENCY
FieldApproximationBase
approximation base
MoFEMTypes
Those types control how functions respond on arguments, f.e. error handling.
@ MOFEM_ATOM_TEST_INVALID
const static char *const MoFEMErrorCodesNames[]
@ HDIV
field with continuous normal traction
@ MAT_MOISTURESET
block name is "MAT_MOISTURE"
DEPRECATED void macro_is_deprecated_using_deprecated_function()
Is used to indicate that macro is deprecated Do nothing just triggers error at the compilation.
CubitBC
Types of sets and boundary conditions.
@ NOFIELD
scalar or vector of scalars describe (no true field)