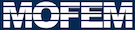 |
| v0.14.0
|
Go to the documentation of this file.
128 for (
i = 1;
i <
k;
i++ )
146 *more = (
a[
k-1] !=
n );
230 beta = (
int * ) malloc ( ( dim_num + 1 ) *
sizeof (
int ) );
232 for (
i = 0;
i <= s;
i++ )
234 weight = (
double ) one_pm;
238 for (
j = 1;
j <= j_hi;
j++ )
242 weight = weight * (
double ) (
j );
246 weight = weight * (
double ) (
d +
n - 2 *
i );
250 weight = weight / 2.0;
254 weight = weight / (
double ) (
j );
256 if (
j <=
d +
n -
i )
258 weight = weight / (
double ) (
j );
271 comp_next ( beta_sum, dim_num + 1, beta, &more, &
h, &
t );
274 for ( dim = 0; dim < dim_num; dim++ )
276 x[dim+
k*dim_num] = (
double ) ( 2 * beta[dim+1] + 1 )
345 arg1 = dim_num + rule + 1;
418 for (
i = 2;
i <= mn;
i++ )
420 value = ( value * ( mx +
i ) ) /
i;
453 static int value = 2147483647;
579 fprintf ( stderr,
"\n" );
580 fprintf ( stderr,
"I4_POWER - Fatal error!\n" );
581 fprintf ( stderr,
" I^J requested, with I = 0 and J negative.\n" );
593 fprintf ( stderr,
"\n" );
594 fprintf ( stderr,
"I4_POWER - Fatal error!\n" );
595 fprintf ( stderr,
" I^J requested, with I = 0 and J = 0.\n" );
610 for (
k = 1;
k <=
j;
k++ )
667 value = (
double * ) malloc ( point_num *
sizeof (
double ) );
669 for ( point = 0; point < point_num; point++ )
674 for ( dim = 0; dim < dim_num; dim++ )
676 if ( 0 != expon[dim] )
678 for ( point = 0; point < point_num; point++ )
680 value[point] = value[point] * pow ( x[dim+point*dim_num], expon[dim] );
767 for (
i = 1;
i <=
n;
i++ )
769 value = value * (
double ) (
i );
809 for (
i = 0;
i <
n;
i++ )
811 value = value +
a1[
i] *
a2[
i];
892 fprintf ( stderr,
"\n" );
893 fprintf ( stderr,
"R8VEC_UNIFORM_01_NEW - Fatal error!\n" );
894 fprintf ( stderr,
" Input value of SEED = 0.\n" );
898 r = (
double * ) malloc (
n *
sizeof (
double ) );
900 for (
i = 0;
i <
n;
i++ )
904 *seed = 16807 * ( *seed -
k * 127773 ) -
k * 2836;
911 r[
i] = (
double ) ( *seed ) * 4.656612875E-10;
967 for ( dim = 0; dim < dim_num; dim++ )
969 for (
i = 1;
i <= expon[dim];
i++ )
976 for ( dim = 0; dim < dim_num; dim++ )
979 value = value / (
double ) (
k );
987 int point_num,
double x[],
double w[] )
1046 quad_error =
r8_abs ( quad - exact );
1109 x = (
double * ) malloc ( dim_num *
n *
sizeof (
double ) );
1111 for (
j = 0;
j <
n;
j++ )
1115 for (
i = 0;
i <= dim_num;
i++ )
1117 e[
i] = -log ( e[
i] );
1121 for (
i = 0;
i <= dim_num;
i++ )
1123 total = total + e[
i];
1126 for (
i = 0;
i < dim_num;
i++ )
1128 x[
i+dim_num*
j] = e[
i] / total;
1198 phy = (
double * ) malloc ( dim_num * point_num *
sizeof (
double ) );
1206 for ( point = 0; point < point_num; point++ )
1208 for ( dim = 0; dim < dim_num; dim++ )
1210 phy[dim+point*dim_num] =
t[dim+0*dim_num];
1212 for ( vertex = 1; vertex < dim_num + 1; vertex++ )
1214 phy[dim+point*dim_num] = phy[dim+point*dim_num]
1215 + (
t[dim+vertex*dim_num] -
t[dim+0*dim_num] ) * ref[vertex-1+point*dim_num];
1259 for (
i = 1;
i <= dim_num;
i++ )
1261 volume = volume / ( (
double )
i );
1297 # define TIME_SIZE 40
1300 const struct tm *tm;
1306 now = time ( NULL );
1307 tm = localtime ( &now );
1309 len = strftime ( time_buffer,
TIME_SIZE,
"%d %B %Y %I:%M:%S %p", tm );
1311 fprintf ( stdout,
"%s\n", time_buffer );
double r8_factorial(int n)
int i4_power(int i, int j)
double * r8vec_uniform_01_new(int n, int *seed)
int i4_max(int i1, int i2)
double * simplex_unit_sample(int dim_num, int n, int *seed)
double * monomial_value(int dim_num, int point_num, double x[], int expon[])
void comp_next(int n, int k, int a[], int *more, int *h, int *t)
int gm_rule_size(int rule, int dim_num)
int i4_choose(int n, int k)
double r8vec_dot_product(int n, double a1[], double a2[])
constexpr double t
plate stiffness
FTensor::Index< 'i', SPACE_DIM > i
double * simplex_unit_to_general(int dim_num, int point_num, double t[], double ref[])
int i4_min(int i1, int i2)
FTensor::Index< 'j', 3 > j
void gm_rule_set(int rule, int dim_num, int point_num, double w[], double x[])
double simplex_unit_volume(int dim_num)
double simplex_unit_monomial_quadrature(int dim_num, int expon[], int point_num, double x[], double w[])
double simplex_unit_monomial_int(int dim_num, int expon[])
FTensor::Index< 'k', 3 > k