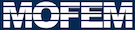 |
| v0.14.0
|
Go to the documentation of this file.
3 template <
char i>
class Index
22 cout <<
"equaling" << endl;
23 iter->data0 = result(0);
24 iter->data1 = result(1);
25 iter->data2 = result(2);
67 return s <<
a.data0 <<
" " <<
a.data1 <<
" " <<
a.data2 <<
" ";
double operator()(const int N) const
friend ostream & operator<<(ostream &s, const Tensor1 &a)
Tensor1_Expr< Tensor1, i > operator()(const Index< i > index)
const A operator=(const Tensor1_Expr< Tensor1, 'i'> &result)
Tensor1(double d0, double d1, double d2)
double & operator()(const int N)
FTensor::Index< 'i', SPACE_DIM > i
double & operator()(const int N)
ostream & operator<<(ostream &s, const Tensor1 &a)
double operator()(const int N) const