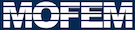 |
| v0.14.0
|
Go to the documentation of this file.
12 os <<
"D i s p l a c e m e n t \n \n";
13 os <<
"Flag for X-Translation (0/1): " << (
int)e.
data.flag1 <<
"\n";
14 os <<
"Flag for Y-Translation (0/1): " << (
int)e.
data.flag2 <<
"\n";
15 os <<
"Flag for Z-Translation (0/1): " << (
int)e.
data.flag3 <<
"\n";
16 os <<
"Flag for X-Rotation (0/1): " << (
int)e.
data.flag4 <<
"\n";
17 os <<
"Flag for Y-Rotation (0/1): " << (
int)e.
data.flag5 <<
"\n";
18 os <<
"Flag for Z-Rotation (0/1): " << (
int)e.
data.flag6 <<
"\n \n";
20 if (e.
data.flag1 == 1)
21 os <<
"Displacement magnitude (X-Translation): " << e.
data.value1 <<
"\n";
23 os <<
"Displacement magnitude (X-Translation): N/A"
25 if (e.
data.flag2 == 1)
26 os <<
"Displacement magnitude (Y-Translation): " << e.
data.value2 <<
"\n";
28 os <<
"Displacement magnitude (Y-Translation): N/A"
30 if (e.
data.flag3 == 1)
31 os <<
"Displacement magnitude (Z-Translation): " << e.
data.value3 <<
"\n";
33 os <<
"Displacement magnitude (Z-Translation): N/A"
35 if (e.
data.flag4 == 1)
36 os <<
"Displacement magnitude (X-Rotation): " << e.
data.value4 <<
"\n";
38 os <<
"Displacement magnitude (X-Rotation): N/A"
40 if (e.
data.flag5 == 1)
41 os <<
"Displacement magnitude (Y-Rotation): " << e.
data.value5 <<
"\n";
43 os <<
"Displacement magnitude (Y-Rotation): N/A"
45 if (e.
data.flag6 == 1)
46 os <<
"Displacement magnitude (Z-Rotation): " << e.
data.value6 <<
"\n \n";
48 os <<
"Displacement magnitude (Z-Rotation): N/A"
55 os <<
"F o r c e \n \n";
56 os <<
"Force magnitude: " << e.
data.value1 <<
"\n";
57 os <<
"Moment magnitude: " << e.
data.value2 <<
"\n";
58 os <<
"Force direction vector (X-component): " << e.
data.value3 <<
"\n";
59 os <<
"Force direction vector (Y-component): " << e.
data.value4 <<
"\n";
60 os <<
"Force direction vector (Z-component): " << e.
data.value5 <<
"\n";
61 os <<
"Moment direction vector (X-component): " << e.
data.value6 <<
"\n";
62 os <<
"Moment direction vector (Y-component): " << e.
data.value7 <<
"\n";
63 os <<
"Moment direction vector (Z-component): " << e.
data.value8 <<
"\n \n";
69 os <<
"V e l o c i t y \n \n";
70 if (e.
data.flag1 == 1)
71 os <<
"Velocity magnitude (X-Translation): " << e.
data.value1 <<
"\n";
73 os <<
"Velocity magnitude (X-Translation): N/A"
75 if (e.
data.flag2 == 1)
76 os <<
"Velocity magnitude (Y-Translation): " << e.
data.value2 <<
"\n";
78 os <<
"Velocity magnitude (Y-Translation): N/A"
80 if (e.
data.flag3 == 1)
81 os <<
"Velocity magnitude (Z-Translation): " << e.
data.value3 <<
"\n";
83 os <<
"Velocity magnitude (Z-Translation): N/A"
85 if (e.
data.flag4 == 1)
86 os <<
"Velocity magnitude (X-Rotation): " << e.
data.value4 <<
"\n";
88 os <<
"Velocity magnitude (X-Rotation): N/A"
90 if (e.
data.flag5 == 1)
91 os <<
"Velocity magnitude (Y-Rotation): " << e.
data.value5 <<
"\n";
93 os <<
"Velocity magnitude (Y-Rotation): N/A"
95 if (e.
data.flag6 == 1)
96 os <<
"Velocity magnitude (Z-Rotation): " << e.
data.value6 <<
"\n \n";
98 os <<
"Velocity magnitude (Z-Rotation): N/A"
105 os <<
"A c c e l e r a t i o n \n \n";
106 if (e.
data.flag1 == 1)
107 os <<
"Acceleration magnitude (X-Translation): " << e.
data.value1 <<
"\n";
109 os <<
"Acceleration magnitude (X-Translation): N/A"
111 if (e.
data.flag2 == 1)
112 os <<
"Acceleration magnitude (Y-Translation): " << e.
data.value2 <<
"\n";
114 os <<
"Acceleration magnitude (Y-Translation): N/A"
116 if (e.
data.flag3 == 1)
117 os <<
"Acceleration magnitude (Z-Translation): " << e.
data.value3 <<
"\n";
119 os <<
"Acceleration magnitude (Z-Translation): N/A"
121 if (e.
data.flag4 == 1)
122 os <<
"Acceleration magnitude (X-Rotation): " << e.
data.value4 <<
"\n";
124 os <<
"Acceleration magnitude (X-Rotation): N/A"
126 if (e.
data.flag5 == 1)
127 os <<
"Acceleration magnitude (Y-Rotation): " << e.
data.value5 <<
"\n";
129 os <<
"Acceleration magnitude (Y-Rotation): N/A"
131 if (e.
data.flag6 == 1)
132 os <<
"Acceleration magnitude (Z-Rotation): " << e.
data.value6 <<
"\n \n";
134 os <<
"Acceleration magnitude (Z-Rotation): N/A"
141 os <<
"T e m p e r a t u r e \n \n";
142 if (e.
data.flag1 == 1)
143 os <<
"Temperature: " << e.
data.value1 <<
"\n";
145 os <<
"Temperature (default case): N/A"
147 if (e.
data.flag2 == 1)
148 os <<
"Temperature (thin shell middle): " << e.
data.value2 <<
"\n";
150 os <<
"Temperature (thin shell middle): N/A"
152 if (e.
data.flag3 == 1)
153 os <<
"Temperature (thin shell gradient): " << e.
data.value3 <<
"\n";
155 os <<
"Temperature (thin shell gradient): N/A"
157 if (e.
data.flag4 == 1)
158 os <<
"Temperature (thin shell top): " << e.
data.value4 <<
"\n";
160 os <<
"Temperature (thin shell top): N/A"
162 if (e.
data.flag5 == 1)
163 os <<
"Temperature (thin shell bottom): " << e.
data.value5 <<
"\n \n";
165 os <<
"Temperature (thin shell bottom): N/A"
172 os <<
"P r e s s u r e \n \n";
173 os <<
"Pressure flag2: " << (
int)e.
data.flag2 <<
"\n";
174 os <<
"Pressure value: " << e.
data.value1 <<
"\n \n";
180 os <<
"H e a t F l u x \n \n";
181 if (e.
data.flag1 == 1)
182 os <<
"Heat flux value: " << e.
data.value1 <<
"\n";
184 os <<
"Heat flux is applied on thin shells"
186 if (e.
data.flag2 == 1)
187 os <<
"Heat flux value (thin shell top): " << e.
data.value2 <<
"\n";
189 os <<
"Heat flux value (thin shell top): N/A"
191 if (e.
data.flag3 == 1)
192 os <<
"Heat flux value (thin shell bottom): " << e.
data.value3 <<
"\n \n";
194 os <<
"Heat flux value (thin shell bottom): N/A"
201 os <<
"CFD BC \n \n";
std::ostream & operator<<(std::ostream &os, const EntitiesFieldData::EntData &e)
Definition of the acceleration bc data structure.
Definition of the temperature bc data structure.
Definition of the force bc data structure.
Definition of the displacement bc data structure.
Definition of the pressure bc data structure.
implementation of Data Operators for Forces and Sources
Definition of the velocity bc data structure.
Definition of the heat flux bc data structure.
Definition of the cfd_bc data structure.