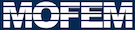 |
| v0.14.0
|
Go to the documentation of this file.
30 "It makes no sense for the generic bc type");
43 "It makes no sense for the generic bc type");
113 if (bc_data.size() !=
sizeof(
data))
115 "data inconsistency %ld != %ld", bc_data.size(),
sizeof(
data));
116 memcpy(&
data, &bc_data[0],
sizeof(
data));
122 if (size !=
sizeof(
data)) {
125 memcpy(tag_ptr, &
data, size);
131 friend std::ostream &
operator<<(std::ostream &os,
165 if (bc_data.size() !=
sizeof(
data))
166 SETERRQ(PETSC_COMM_SELF, 1,
"data inconsistency");
167 memcpy(&
data, &bc_data[0],
sizeof(
data));
173 if (size !=
sizeof(
data)) {
176 memcpy(tag_ptr, &
data, size);
222 if (bc_data.size() !=
sizeof(
data))
223 SETERRQ(PETSC_COMM_SELF, 1,
"data inconsistency");
224 memcpy(&
data, &bc_data[0],
sizeof(
data));
230 if (size !=
sizeof(
data)) {
233 memcpy(tag_ptr, &
data, size);
239 friend std::ostream &
operator<<(std::ostream &os,
281 if (bc_data.size() !=
sizeof(
data))
282 SETERRQ(PETSC_COMM_SELF, 1,
"data inconsistency");
283 memcpy(&
data, &bc_data[0],
sizeof(
data));
289 if (size !=
sizeof(
data)) {
292 memcpy(tag_ptr, &
data, size);
298 friend std::ostream &
operator<<(std::ostream &os,
339 if (bc_data.size() >
sizeof(
data))
341 "Wrong number of parameters in Cubit %d != %d", bc_data.size(),
345 if (bc_data.size() == 58) {
346 std::vector<char> new_bc_data(66, 0);
348 for (; ii != 16; ++ii)
349 new_bc_data[ii] = bc_data[ii];
350 for (; ii != bc_data.size(); ++ii)
351 new_bc_data[ii + 1] = bc_data[ii];
352 memcpy(&
data, &new_bc_data[0], new_bc_data.size());
354 memcpy(&
data, &bc_data[0], bc_data.size());
362 if (size !=
sizeof(
data)) {
365 memcpy(tag_ptr, &
data, size);
371 friend std::ostream &
operator<<(std::ostream &os,
401 if (bc_data.size() !=
sizeof(
data)) {
404 memcpy(&
data, &bc_data[0],
sizeof(
data));
410 if (size !=
sizeof(
data)) {
413 memcpy(tag_ptr, &
data, size);
419 friend std::ostream &
operator<<(std::ostream &os,
455 if (bc_data.size() !=
sizeof(
data))
456 SETERRQ(PETSC_COMM_SELF, 1,
"data inconsistency");
457 memcpy(&
data, &bc_data[0],
sizeof(
data));
463 if (size !=
sizeof(
data)) {
466 memcpy(tag_ptr, &
data, size);
472 friend std::ostream &
operator<<(std::ostream &os,
499 if (bc_data.size() !=
sizeof(
data))
500 SETERRQ(PETSC_COMM_SELF, 1,
"data inconsistency");
501 memcpy(&
data, &bc_data[0],
sizeof(
data));
507 if (size !=
sizeof(
data)) {
510 memcpy(tag_ptr, &
data, size);
521 #endif // __BCMULTIINDICES_HPP__
struct __attribute__((packed)) _data_
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
friend std::ostream & operator<<(std::ostream &os, const HeatFluxCubitBcData &e)
Print heat flux bc data.
const void * getDataPtr() const
get pointer to data structure
friend std::ostream & operator<<(std::ostream &os, const AccelerationCubitBcData &e)
Print acceleration bc data.
struct __attribute__((packed)) _data_
struct __attribute__((packed)) _data_
Generic bc data structure.
Definition of the acceleration bc data structure.
Definition of the temperature bc data structure.
std::size_t getSizeOfData() const
get data structure size
friend std::ostream & operator<<(std::ostream &os, const DisplacementCubitBcData &e)
Print displacement bc data.
MoFEMErrorCode fill_data(const std::vector< char > &bc_data)
get data from structure
const void * getDataPtr() const
get pointer to data structure
DisplacementCubitBcData()
friend std::ostream & operator<<(std::ostream &os, const ForceCubitBcData &e)
Print force bc data.
const void * getDataPtr() const
get pointer to data structure
MoFEMErrorCode set_data(void *tag_ptr, unsigned int size) const
set data on structure
const void * getDataPtr() const
get pointer to data structure
std::size_t getSizeOfData() const
get data structure size
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
struct __attribute__((packed)) _data_
struct __attribute__((packed)) _data_
friend std::ostream & operator<<(std::ostream &os, const PressureCubitBcData &e)
Print pressure bc data.
Definition of the force bc data structure.
struct __attribute__((packed)) _data_
Definition of the displacement bc data structure.
virtual const CubitBCType & getType() const
get data type
virtual const void * getDataPtr() const =0
get pointer to data structure
struct __attribute__((packed)) _data_
attributes of DisplacementCubitBcData
friend std::ostream & operator<<(std::ostream &os, const CfgCubitBcData &e)
Print cfd_bc data.
const CubitBCType tYpe
Type of boundary condition.
MoFEMErrorCode fill_data(const std::vector< char > &bc_data)
get data from structure
std::size_t getSizeOfData() const
get data structure size
Definition of the pressure bc data structure.
virtual MoFEMErrorCode fill_data(const std::vector< char > &bc_data)
get data from structure
MoFEMErrorCode fill_data(const std::vector< char > &bc_data)
get data from structure
virtual std::size_t getSizeOfData() const =0
get data structure size
MoFEMErrorCode fill_data(const std::vector< char > &bc_data)
get data from structure
AccelerationCubitBcData()
MoFEMErrorCode set_data(void *tag_ptr, unsigned int size) const
set data on structure
virtual ~GenericCubitBcData()
virtual MoFEMErrorCode set_data(void *tag_ptr, unsigned int size) const
set data on structure
implementation of Data Operators for Forces and Sources
MoFEMErrorCode set_data(void *tag_ptr, unsigned int size) const
set data on structure
MoFEMErrorCode fill_data(const std::vector< char > &bc_data)
get data from structure
std::size_t getSizeOfData() const
get data structure size
MoFEMErrorCode fill_data(const std::vector< char > &bc_data)
get data from structure
std::size_t getSizeOfData() const
get data structure size
MoFEMErrorCode set_data(void *tag_ptr, unsigned int size) const
set data on structure
Definition of the velocity bc data structure.
const void * getDataPtr() const
get pointer to data structure
MoFEMErrorCode fill_data(const std::vector< char > &bc_data)
get data from structure
std::bitset< 32 > CubitBCType
const void * getDataPtr() const
get pointer to data structure
std::size_t getSizeOfData() const
get data structure size
@ MOFEM_DATA_INCONSISTENCY
MoFEMErrorCode fill_data(const std::vector< char > &bc_data)
get data from structure
MoFEMErrorCode set_data(void *tag_ptr, unsigned int size) const
set data on structure
const void * getDataPtr() const
get pointer to data structure
MoFEMErrorCode set_data(void *tag_ptr, unsigned int size) const
set data on structure
std::size_t getSizeOfData() const
get data structure size
Definition of the heat flux bc data structure.
friend std::ostream & operator<<(std::ostream &os, const TemperatureCubitBcData &e)
Print temperature bc data.
GenericCubitBcData(const CubitBCType type)
@ MOFEM_ATOM_TEST_INVALID
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
friend std::ostream & operator<<(std::ostream &os, const VelocityCubitBcData &e)
Print velocity bc data.
const void * getDataPtr() const
get pointer to data structure
Definition of the cfd_bc data structure.
std::size_t getSizeOfData() const
get data structure size
MoFEMErrorCode set_data(void *tag_ptr, unsigned int size) const
set data on structure
struct __attribute__((packed)) _data_
MoFEMErrorCode set_data(void *tag_ptr, unsigned int size) const
set data on structure