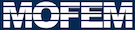 |
| v0.14.0
|
Go to the documentation of this file.
31 typedef uint128_t
UId;
43 typedef std::bitset<BITFEID_SIZE>
BitFEId;
75 using UBlasMatrix = ublas::matrix<T, ublas::row_major, VecAllocator<T>>;
84 template <
typename T,
size_t N>
102 template <
typename T,
size_t N>
104 ublas::matrix<T, ublas::row_major, ublas::bounded_array<T, N>>;
112 template <
typename T>
114 ublas::vector<T, ublas::shallow_array_adaptor<T>>;
118 template <
typename T>
121 ublas::shallow_array_adaptor<double>>;
136 using namespace Types;
140 #endif // __TYPES_HPP__
VectorBoundedArray< double, 6 > VectorDouble6
int PetscGlobalDofIdx
Index of DOF using global pets index.
UBlasMatrix< std::complex< double > > MatrixComplexDouble
VectorBoundedArray< double, 3 > VectorDouble3
ublas::matrix< double, ublas::row_major, ublas::shallow_array_adaptor< double > > MatrixShallowArrayAdaptor
std::bitset< BITPROBLEMID_SIZE > BitProblemId
Problem Id.
VectorBoundedArray< int, 5 > VectorInt5
std::bitset< BITFIELDID_SIZE > BitFieldId
Field Id.
VectorShallowArrayAdaptor< int > VectorIntAdaptor
int FEIdx
Index of the element.
std::bitset< BITFEID_SIZE > BitFEId
Finite element Id.
VectorBoundedArray< int, 3 > VectorInt3
int MoFEMDofIdx
Index of DOF using mofem native index.
UBlasMatrix< adouble > MatrixADouble
implementation of Data Operators for Forces and Sources
int ShortId
Unique Id in the field.
int EntPart
Partition owning entity.
VectorBoundedArray< int, 9 > VectorInt9
UBlasMatrix< int > MatrixInt
int FieldCoefficientsNumber
Number of field coefficients.
int EntIdx
Index of DOF on the entity.
VectorBoundedArray< int, 6 > VectorInt6
ublas::matrix< T, ublas::row_major, VecAllocator< T > > UBlasMatrix
VectorShallowArrayAdaptor< double > VectorAdaptor
VecAllocator< double > DoubleAllocator
VectorBoundedArray< adouble, 9 > VectorADouble9
std::vector< T, std::allocator< T > > VecAllocator
MatrixBoundedArray< std::complex< double >, 9 > MatrixComplexDouble3by3
std::bitset< BITINTERFACEUID_SIZE > BitIntefaceId
ublas::vector< T, ublas::bounded_array< T, N > > VectorBoundedArray
VectorBoundedArray< double, 5 > VectorDouble5
std::bitset< 32 > CubitBCType
int ApproximationOrder
Approximation on the entity.
int PetscLocalDofIdx
Index of DOF using local petsc index.
ublas::vector< T, VecAllocator< T > > UBlasVector
std::bitset< BITREFEDGES_SIZE > BitRefEdges
UBlasVector< int > VectorInt
char FieldBitNumber
Field bit number.
double FieldData
Field data type.
VecAllocator< int > IntAllocator
VecAllocator< std::complex< double > > ComplexDoubleAllocator
ublas::vector< T, ublas::shallow_array_adaptor< T > > VectorShallowArrayAdaptor
MatrixShallowArrayAdaptor< double > MatrixAdaptor
Matrix adaptor.
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
UBlasVector< adouble > VectorADouble
VectorBoundedArray< double, 9 > VectorDouble9
VectorBoundedArray< int, 4 > VectorInt4
UBlasVector< std::complex< double > > VectorComplexDouble
ublas::matrix< T, ublas::row_major, ublas::bounded_array< T, N > > MatrixBoundedArray
VectorBoundedArray< double, 4 > VectorDouble4
VectorBoundedArray< double, 12 > VectorDouble12