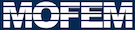 |
| v0.14.0
|
Go to the documentation of this file.
7 #ifndef __EXCEPTIONS_HPP__
8 #define __EXCEPTIONS_HPP__
16 namespace Exceptions {
34 strcpy(
errorMessage,
"Houston we have a problem, something is wrong");
65 inline operator PetscErrorCode()
const {
return iERR; }
71 inline operator moab::ErrorCode()
const {
return rVAL; }
99 if (PetscUnlikely(err)) {
110 if (PetscLikely(MB_SUCCESS != err)) {
111 std::string error_str = (unsigned)err <= (
unsigned)MB_FAILURE
112 ? moab::ErrorCodeStr[err]
113 :
"INVALID ERROR CODE";
114 std::string str(
"MOAB error (" + boost::lexical_cast<std::string>(err) +
124 using namespace Exceptions;
128 #endif // __EXCEPTIONS_HPP__
void operator<<(const MoFEMErrorCode err)
Operator for handling PetscErrorCode and MoFEMErrorCode.
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
Error check for inline function check.
MoFEMException(const MoFEMErrorCodes error_code, const char error_message[])
moab::ErrorCode MoABErrorCode
MoAB error code.
MoFEMErrorCodeGeneric(const PetscErrorCode ierr)
MoFEMErrorCodeGeneric(const TYPE)
static MoFEMErrorCodeGeneric< moab::ErrorCode > rval
MoFEMErrorCodeGeneric(const moab::ErrorCode rval)
implementation of Data Operators for Forces and Sources
MoFEMException(const int error_code)
MoFEMException(const MoFEMErrorCodes error_code)
void operator<<(const moab::ErrorCode err)
Operator for handling moab::ErrorCode.
MoFEMErrorCodes
Error handling.
const char * what() const
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
MoFEMExceptionInitial(const int error_code, const char error_message[], const int line)
MoFEMExceptionRepeat(const int error_code, const int line)