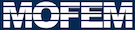 |
| v0.14.0
|
Go to the documentation of this file.
8 #ifndef __PROJECTION_MATRIX_CTX_HPP__
9 #define __PROJECTION_MATRIX_CTX_HPP__
53 string y_problem,
bool create_ksp =
true,
54 bool own_contrain_matrix =
false);
57 bool create_ksp =
true,
bool own_contrain_matrix =
false);
65 ierr = MatDestroy(&
C);
255 #endif // __PROJECTION_MATRIX_CTX_HPP__
MoFEMErrorCode ConstrainMatrixMultOpCTC_QTKQ(Mat CTC_QTKQ, Vec x, Vec f)
Multiplication operator for RT = (CCT)^-TC.
MoFEMErrorCode initializeQorP(Vec x)
initialize vectors and matrices for Q and P shell matrices, scattering is set based on x_problem and ...
MoFEMErrorCode destroyQorP()
destroy sub-matrices used for shell matrices P, Q, R, RT
virtual MPI_Comm & get_comm() const =0
PetscLogEvent MOFEM_EVENT_projCTC_QTKQ
MoFEMErrorCode recalculateCTandCCT()
re-calculate CT and CCT if C matrix has been changed since initialization
MoFEMErrorCode ConstrainMatrixDestroyOpPorQ(Mat Q)
Destroy shell matrix Q.
friend MoFEMErrorCode ConstrainMatrixMultOpCTC_QTKQ(Mat CTC_QTKQ, Vec x, Vec f)
Multiplication operator for RT = (CCT)^-TC.
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
friend MoFEMErrorCode ConstrainMatrixMultOpRT(Mat RT, Vec x, Vec f)
Multiplication operator for RT = (CCT)^-TC.
Deprecated interface functions.
ConstrainMatrixCtx(MoFEM::Interface &m_field, string x_problem, string y_problem, bool create_ksp=true, bool own_contrain_matrix=false)
friend MoFEMErrorCode ConstrainMatrixDestroyOpPorQ()
MoFEMErrorCode destroyQTKQ()
destroy sub-matrices used for shell matrix QTKQ
MoFEMErrorCode ConstrainMatrixMultOpRT(Mat RT, Vec x, Vec f)
Multiplication operator for RT = (CCT)^-TC.
friend MoFEMErrorCode ConstrainMatrixMultOpR(Mat R, Vec x, Vec f)
Multiplication operator for R = CT(CCT)^-1.
PetscLogEvent MOFEM_EVENT_projR
friend MoFEMErrorCode ConstrainMatrixMultOpP(Mat P, Vec x, Vec f)
Multiplication operator for P = CT(CCT)^-1C.
PetscLogEvent MOFEM_EVENT_projInit
friend MoFEMErrorCode ProjectionMatrixMultOpQ(Mat Q, Vec x, Vec f)
Multiplication operator for Q = I-CTC(CCT)^-1C.
PetscLogEvent MOFEM_EVENT_projRT
friend MoFEMErrorCode ConstrainMatrixDestroyOpQTKQ()
PetscLogEvent MOFEM_EVENT_projP
MoFEMErrorCode recalculateCTC()
re-calculate CTC matrix has been changed since initialization
MoFEMErrorCode ProjectionMatrixMultOpQ(Mat Q, Vec x, Vec f)
Multiplication operator for Q = I-CTC(CCT)^-1C.
MoFEM::Interface & mField
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
const FTensor::Tensor2< T, Dim, Dim > Vec
PetscLogEvent MOFEM_EVENT_projQ
virtual ~ConstrainMatrixCtx()
structure for projection matrices
MoFEMErrorCode initializeQTKQ()
initialize vectors and matrices for CTC+QTKQ shell matrices, scattering is set based on x_problem and...
MoFEMErrorCode ConstrainMatrixDestroyOpQTKQ(Mat QTKQ)
Destroy shell matrix.
MoFEMErrorCode ConstrainMatrixMultOpR(Mat R, Vec x, Vec f)
Multiplication operator for R = CT(CCT)^-1.
MoFEMErrorCode ConstrainMatrixMultOpP(Mat P, Vec x, Vec f)
Multiplication operator for P = CT(CCT)^-1C.