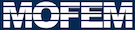 |
| v0.14.0
|
Go to the documentation of this file.
13 mutable T *restrict data[(Tensor_Dim * (Tensor_Dim + 1)) / 2];
18 static_assert(
sizeof...(
d) ==
sizeof(data) /
sizeof(T),
19 "Incorrect number of Arguments. Constructor should "
20 "initialize the entire Tensor");
25 static_assert(
sizeof...(
d) ==
sizeof(data) /
sizeof(T),
26 "Incorrect number of Arguments. Constructor should "
27 "initialize the entire Tensor");
44 data, d00, d01, d02, d11, d12, d22);
53 if(N1 >= Tensor_Dim || N1 < 0 || N2 >= Tensor_Dim || N2 < 0)
56 s <<
"Bad index in Tensor2_symmetric<T*," << Tensor_Dim
57 <<
">.operator(" << N1 <<
"," << N2 <<
")" << std::endl;
58 throw std::out_of_range(s.str());
61 return N1 > N2 ? *data[N1 + (N2 * (2 * Tensor_Dim - N2 - 1)) / 2]
62 : *data[N2 + (N1 * (2 * Tensor_Dim - N1 - 1)) / 2];
68 if(N1 >= Tensor_Dim || N1 < 0 || N2 >= Tensor_Dim || N2 < 0)
71 s <<
"Bad index in Tensor2_symmetric<T*," << Tensor_Dim
72 <<
">.operator(" << N1 <<
"," << N2 <<
") const" << std::endl;
73 throw std::out_of_range(s.str());
76 return N1 > N2 ? *data[N1 + (N2 * (2 * Tensor_Dim - N2 - 1)) / 2]
77 : *data[N2 + (N1 * (2 * Tensor_Dim - N1 - 1)) / 2];
80 T *
ptr(
const int N1,
const int N2)
const
83 if(N1 >= Tensor_Dim || N1 < 0 || N2 >= Tensor_Dim || N2 < 0)
86 s <<
"Bad index in Tensor2_symmetric<T*," << Tensor_Dim <<
">.ptr("
87 << N1 <<
"," << N2 <<
")" << std::endl;
88 throw std::out_of_range(s.str());
91 return N1 > N2 ? data[N1 + (N2 * (2 * Tensor_Dim - N2 - 1)) / 2]
92 : data[N2 + (N1 * (2 * Tensor_Dim - N1 - 1)) / 2];
103 template <
char i,
char j,
int Dim0,
int Dim1>
111 template <
char i,
char j,
int Dim0,
int Dim1>
122 template <
char i,
char j,
int Dim>
130 template <
char i,
char j,
int Dim>
136 T, Dim,
i,
j>(*this);
146 template <
char i,
int N,
int Dim>
156 template <
char i,
int N,
int Dim>
167 template <
char i,
int N,
int Dim>
177 template <
char i,
int N,
int Dim>
190 template <
char i,
int Dim>
201 template <
char i,
int Dim>
217 for(
int i = 0;
i < (Tensor_Dim * (Tensor_Dim + 1)) / 2; ++
i)
227 template <
char i,
int Dim>
233 template <
char i,
int Dim>
242 return *data[
N - 1 + ((
N - 1) * (2 * Tensor_Dim -
N)) / 2]
249 template <
class T,
int Tensor_Dim,
int I>
254 template <
class...
U>
265 for(
int i = 0;
i < (Tensor_Dim * (Tensor_Dim + 1)) / 2; ++
i)
Tensor1_Expr< const Tensor2_number_0< const Tensor2_symmetric< T *, Tensor_Dim >, T, N >, T, Dim, i > operator()(const Number< N > &n1, const Index< i, Dim > index1) const
T internal_contract(Number< N >) const
Tensor2_symmetric(U *... d)
Tensor1_Expr< Tensor2_number_rhs_1< Tensor2_symmetric< T *, Tensor_Dim >, T, N >, T, Dim, i > operator()(const Index< i, Dim > index1, const Number< N > &n1)
Tensor2_symmetric(const int i, U *... d)
T * ptr(const int N1, const int N2) const
const Tensor1_Expr< const dTensor0< T, Dim, i >, typename promote< T, double >::V, Dim, i > d(const Tensor0< T * > &a, const Index< i, Dim > index, const Tensor1< int, Dim > &d_ijk, const Tensor1< double, Dim > &d_xyz)
Tensor2_symmetric_Expr< const Tensor2_symmetric< T *, Tensor_Dim >, T, Dim, i, j > operator()(const Index< i, Dim > index1, const Index< j, Dim > index2) const
Tensor1_Expr< Tensor2_number_rhs_0< Tensor2_symmetric< T *, Tensor_Dim >, T, N >, T, Dim, i > operator()(const Number< N > &n1, const Index< i, Dim > index1)
T operator()(const Index< i, Dim > index1, const Index< i, Dim > index2) const
T & operator()(const int N1, const int N2)
T operator()(const Index< i, Dim > index1, const Index< i, Dim > index2)
Tensor2_Expr< const Tensor2_symmetric< T *, Tensor_Dim >, T, Dim0, Dim1, i, j > operator()(const Index< i, Dim0 > index1, const Index< j, Dim1 > index2) const
Tensor2_Expr< Tensor2_symmetric< T *, Tensor_Dim >, T, Dim0, Dim1, i, j > operator()(const Index< i, Dim0 > index1, const Index< j, Dim1 > index2)
constexpr IntegrationType I
Tensor2_symmetric(U *... d)
T operator()(const int N1, const int N2) const
const Tensor2_symmetric< T *, Tensor_Dim > & operator++() const
Tensor2_symmetric(T *d00, T *d01, T *d02, T *d11, T *d12, T *d22, const int i=1)
FTensor::Index< 'i', SPACE_DIM > i
Tensor2_symmetric(T *d00, T *d01, T *d11, const int i=1)
Tensor2_symmetric_Expr< Tensor2_symmetric< T *, Tensor_Dim >, T, Dim, i, j > operator()(const Index< i, Dim > index1, const Index< j, Dim > index2)
Tensor1_Expr< const Tensor2_number_1< const Tensor2_symmetric< T *, Tensor_Dim >, T, N >, T, Dim, i > operator()(const Index< i, Dim > index1, const Number< N > &n1) const
FTensor::Index< 'j', 3 > j
T internal_contract(Number< 1 >) const
const Tensor2_symmetric< PackPtr< T *, I >, Tensor_Dim > & operator++() const
Tensor1_Expr< const Tensor2_numeral_0< const Tensor2_symmetric< T *, Tensor_Dim >, T >, T, Dim, i > operator()(const int N, const Index< i, Dim > index1) const
Tensor1_Expr< const Tensor2_numeral_1< const Tensor2_symmetric< T *, Tensor_Dim >, T >, T, Dim, i > operator()(const Index< i, Dim > index1, const int N) const