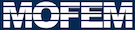 |
| v0.14.0
|
Go to the documentation of this file.
11 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
int Dim3,
12 char i,
char j,
char k,
char l>
18 template <
int Current_Dim>
22 return iterA(N1, N2, Current_Dim - 1) *
iterB(Current_Dim - 1, N3)
37 operator()(
const int N1,
const int N2,
const int N3)
const
43 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
int Dim3,
44 char i,
char j,
char k,
char l>
45 Dg_Expr<Dg_times_Tensor2_0<A, B, T, U, Dim01, Dim2, Dim3, i, j, k, l>,
51 =
Dg_times_Tensor2_0<A, B, T, U, Dim01, Dim2, Dim3, i, j, k, l>;
58 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
int Dim3,
59 char i,
char j,
char k,
char l>
60 Dg_Expr<Dg_times_Tensor2_0<A, B, T, U, Dim01, Dim2, Dim3, i, j, k, l>,
66 =
Dg_times_Tensor2_0<A, B, T, U, Dim01, Dim2, Dim3, i, j, k, l>;
73 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
int Dim3,
74 char i,
char j,
char k,
char l>
80 template <
int Current_Dim>
84 return iterA(N1, N2, Current_Dim - 1) *
iterB(N3, Current_Dim - 1)
99 operator()(
const int N1,
const int N2,
const int N3)
const
105 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
int Dim3,
106 char i,
char j,
char k,
char l>
107 Dg_Expr<Dg_times_Tensor2_1<A, B, T, U, Dim01, Dim2, Dim3, i, j, k, l>,
113 =
Dg_times_Tensor2_1<A, B, T, U, Dim01, Dim2, Dim3, i, j, k, l>;
120 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
int Dim3,
121 char i,
char j,
char k,
char l>
122 Dg_Expr<Dg_times_Tensor2_1<A, B, T, U, Dim01, Dim2, Dim3, i, j, k, l>,
128 =
Dg_times_Tensor2_1<A, B, T, U, Dim01, Dim2, Dim3, i, j, k, l>;
135 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
int Dim3,
136 char i,
char j,
char k,
char l>
142 template <
int Current_Dim>
146 return iterA(N1, Current_Dim - 1, N2) *
iterB(Current_Dim - 1, N3)
167 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
int Dim3,
168 char i,
char j,
char k,
char l>
169 Tensor3_Expr<Dg_times_Tensor2_1_0<A, B, T, U, Dim01, Dim2, Dim3, i, j, k, l>,
175 =
Dg_times_Tensor2_1_0<A, B, T, U, Dim01, Dim2, Dim3, i, j, k, l>;
177 Dim3,
i,
k,
l>(TensorExpr(
a, b));
182 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
int Dim3,
183 char i,
char j,
char k,
char l>
184 Tensor3_Expr<Dg_times_Tensor2_1_0<A, B, T, U, Dim01, Dim2, Dim3, i, j, k, l>,
190 =
Dg_times_Tensor2_1_0<A, B, T, U, Dim01, Dim2, Dim3, i, j, k, l>;
192 Dim3,
i,
k,
l>(TensorExpr(
a, b));
197 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
int Dim3,
198 char i,
char j,
char k,
char l>
204 template <
int Current_Dim>
208 return iterA(N1, Current_Dim - 1, N2) *
iterB(N3, Current_Dim - 1)
229 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
int Dim3,
230 char i,
char j,
char k,
char l>
231 Tensor3_Expr<Dg_times_Tensor2_1_1<A, B, T, U, Dim01, Dim2, Dim3, i, j, k, l>,
237 =
Dg_times_Tensor2_1_1<A, B, T, U, Dim01, Dim2, Dim3, i, j, k, l>;
239 Dim3,
i,
k,
l>(TensorExpr(
a, b));
244 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
int Dim3,
245 char i,
char j,
char k,
char l>
246 Tensor3_Expr<Dg_times_Tensor2_1_1<A, B, T, U, Dim01, Dim2, Dim3, i, j, k, l>,
252 =
Dg_times_Tensor2_1_1<A, B, T, U, Dim01, Dim2, Dim3, i, j, k, l>;
254 Dim3,
i,
k,
l>(TensorExpr(
a, b));
259 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
char i,
266 template <
int Current_Dim0,
int Current_Dim1>
270 return iterA(N1, Current_Dim0 - 1, Current_Dim1 - 1)
271 *
iterB(Current_Dim0 - 1, Current_Dim1 - 1)
274 template <
int Current_Dim1>
278 return iterA(N1, 0, Current_Dim1 - 1) *
iterB(0, Current_Dim1 - 1)
298 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
char i,
312 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
char i,
326 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
char i,
333 template <
int Current_Dim0,
int Current_Dim1>
337 return iterA(N1, Current_Dim0 - 1, Current_Dim1 - 1)
338 *
iterB(Current_Dim1 - 1, Current_Dim0 - 1)
341 template <
int Current_Dim1>
345 return iterA(N1, 0, Current_Dim1 - 1) *
iterB(Current_Dim1 - 1, 0)
365 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
char i,
379 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
char i,
393 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
char i,
400 template <
int Current_Dim0,
int Current_Dim1>
404 return iterA(Current_Dim0 - 1, N1, Current_Dim1 - 1)
405 *
iterB(Current_Dim0 - 1, Current_Dim1 - 1)
408 template <
int Current_Dim1>
412 return iterA(0, N1, Current_Dim1 - 1) *
iterB(0, Current_Dim1 - 1)
432 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
char i,
446 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
char i,
460 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
char i,
467 template <
int Current_Dim0,
int Current_Dim1>
471 return iterA(Current_Dim0 - 1, N1, Current_Dim1 - 1)
472 *
iterB(Current_Dim1 - 1, Current_Dim0 - 1)
475 template <
int Current_Dim1>
479 return iterA(0, N1, Current_Dim1 - 1) *
iterB(Current_Dim1 - 1, 0)
499 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
char i,
513 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
char i,
527 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
char i,
534 template <
int Current_Dim0,
int Current_Dim1>
538 return iterA(Current_Dim0 - 1, Current_Dim1 - 1, N1)
539 *
iterB(Current_Dim0 - 1, Current_Dim1 - 1)
542 template <
int Current_Dim1>
546 return iterA(0, Current_Dim1 - 1, N1) *
iterB(0, Current_Dim1 - 1)
566 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
char i,
580 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
char i,
594 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
char i,
601 template <
int Current_Dim0,
int Current_Dim1>
605 return iterA(Current_Dim0 - 1, Current_Dim1 - 1, N1)
606 *
iterB(Current_Dim1 - 1, Current_Dim0 - 1)
609 template <
int Current_Dim1>
613 return iterA(0, Current_Dim1 - 1, N1) *
iterB(Current_Dim1 - 1, 0)
633 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
char i,
647 template <
class A,
class B,
class T,
class U,
int Dim01,
int Dim2,
char i,
promote< T, U >::V eval(const int N1, const Number< Current_Dim0 > &, const Number< Current_Dim1 > &) const
Dg_Expr< A, T, Dim01, Dim2, i, j, k > iterA
Tensor2_Expr< B, U, Dim3, Dim2, l, k > iterB
promote< T, U >::V operator*(const Ddg_Expr< A, T, Dim, Dim, i, j, k, l > &a, const Ddg_Expr< B, U, Dim, Dim, i, k, j, l > &b)
Tensor2_Expr< B, U, Dim2, Dim3, k, l > iterB
promote< T, U >::V eval(const int N1, const Number< 1 > &, const Number< Current_Dim1 > &) const
Tensor2_Expr< B, U, Dim01, Dim3, j, l > iterB
promote< T, U >::V operator()(const int N1, const int N2, const int N3) const
promote< T, U >::V eval(const int N1, const Number< 1 > &, const Number< 1 > &) const
Dg_times_Tensor2_1_0(const Dg_Expr< A, T, Dim01, Dim2, i, j, k > &a, const Tensor2_Expr< B, U, Dim01, Dim3, j, l > &b)
promote< T, U >::V operator()(const int N1, const int N2, const int N3) const
Dg_Expr< A, T, Dim01, Dim2, i, j, k > iterA
promote< T, U >::V eval(const int N1, const int N2, const int N3, const Number< Current_Dim > &) const
promote< T, U >::V eval(const int N1, const int N2, const int N3, const Number< 1 > &) const
promote< T, U >::V eval(const int N1, const Number< 1 > &, const Number< Current_Dim1 > &) const
Dg_Expr< A, T, Dim01, Dim2, j, k, i > iterA
promote< T, U >::V eval(const int N1, const Number< 1 > &, const Number< Current_Dim1 > &) const
Dg_Expr< A, T, Dim01, Dim2, i, j, k > iterA
Dg_times_Tensor2_12(const Dg_Expr< A, T, Dim01, Dim2, i, j, k > &a, const Tensor2_Expr< B, U, Dim01, Dim2, j, k > &b)
promote< T, U >::V eval(const int N1, const Number< 1 > &, const Number< 1 > &) const
promote< T, U >::V operator()(const int N1, const int N2, const int N3) const
Dg_Expr< A, T, Dim01, Dim2, k, i, j > iterA
promote< T, U >::V eval(const int N1, const int N2, const int N3, const Number< 1 > &) const
promote< T, U >::V eval(const int N1, const Number< 1 > &, const Number< 1 > &) const
promote< T, U >::V eval(const int N1, const int N2, const int N3, const Number< Current_Dim > &) const
Dg_Expr< A, T, Dim01, Dim2, j, k, i > iterA
promote< T, U >::V eval(const int N1, const int N2, const int N3, const Number< Current_Dim > &) const
promote< T, U >::V eval(const int N1, const Number< Current_Dim0 > &, const Number< Current_Dim1 > &) const
promote< T, U >::V eval(const int N1, const Number< Current_Dim0 > &, const Number< Current_Dim1 > &) const
Dg_Expr< A, T, Dim01, Dim2, i, j, k > iterA
Tensor2_Expr< B, U, Dim01, Dim2, j, k > iterB
Dg_times_Tensor2_01(const Dg_Expr< A, T, Dim01, Dim2, j, k, i > &a, const Tensor2_Expr< B, U, Dim01, Dim01, j, k > &b)
Dg_times_Tensor2_10(const Dg_Expr< A, T, Dim01, Dim2, j, k, i > &a, const Tensor2_Expr< B, U, Dim01, Dim01, k, j > &b)
promote< T, U >::V eval(const int N1, const Number< 1 > &, const Number< 1 > &) const
Dg_times_Tensor2_20(const Dg_Expr< A, T, Dim01, Dim2, k, i, j > &a, const Tensor2_Expr< B, U, Dim2, Dim01, j, k > &b)
promote< T, U >::V eval(const int N1, const int N2, const int N3, const Number< 1 > &) const
promote< T, U >::V eval(const int N1, const Number< Current_Dim0 > &, const Number< Current_Dim1 > &) const
promote< T, U >::V eval(const int N1, const Number< Current_Dim0 > &, const Number< Current_Dim1 > &) const
Tensor2_Expr< B, U, Dim01, Dim2, j, k > iterB
Dg_times_Tensor2_1(const Dg_Expr< A, T, Dim01, Dim2, i, j, k > &a, const Tensor2_Expr< B, U, Dim3, Dim2, l, k > &b)
FTensor::Index< 'i', SPACE_DIM > i
Dg_Expr< A, T, Dim01, Dim2, j, i, k > iterA
promote< T, U >::V operator()(const int N1) const
Tensor2_Expr< B, U, Dim3, Dim01, l, j > iterB
Dg_times_Tensor2_0(const Dg_Expr< A, T, Dim01, Dim2, i, j, k > &a, const Tensor2_Expr< B, U, Dim2, Dim3, k, l > &b)
Dg_times_Tensor2_02(const Dg_Expr< A, T, Dim01, Dim2, j, i, k > &a, const Tensor2_Expr< B, U, Dim01, Dim2, j, k > &b)
Dg_Expr< A, T, Dim01, Dim2, i, j, k > iterA
Dg_times_Tensor2_21(const Dg_Expr< A, T, Dim01, Dim2, i, j, k > &a, const Tensor2_Expr< B, U, Dim2, Dim01, k, j > &b)
promote< T, U >::V eval(const int N1, const Number< 1 > &, const Number< Current_Dim1 > &) const
promote< T, U >::V operator()(const int N1) const
Dg_Expr< A, T, Dim01, Dim2, i, j, k > iterA
Tensor2_Expr< B, U, Dim2, Dim01, k, j > iterB
promote< T, U >::V operator()(const int N1, const int N2, const int N3) const
FTensor::Index< 'j', 3 > j
Tensor2_Expr< B, U, Dim01, Dim01, j, k > iterB
promote< T, U >::V operator()(const int N1) const
Tensor2_Expr< B, U, Dim2, Dim01, j, k > iterB
promote< T, U >::V eval(const int N1, const Number< 1 > &, const Number< 1 > &) const
Dg_times_Tensor2_1_1(const Dg_Expr< A, T, Dim01, Dim2, i, j, k > &a, const Tensor2_Expr< B, U, Dim3, Dim01, l, j > &b)
promote< T, U >::V eval(const int N1, const Number< 1 > &, const Number< Current_Dim1 > &) const
promote< T, U >::V eval(const int N1, const Number< Current_Dim0 > &, const Number< Current_Dim1 > &) const
promote< T, U >::V eval(const int N1, const Number< 1 > &, const Number< 1 > &) const
Tensor2_Expr< B, U, Dim01, Dim01, k, j > iterB
promote< T, U >::V operator()(const int N1) const
FTensor::Index< 'k', 3 > k
promote< T, U >::V eval(const int N1, const int N2, const int N3, const Number< Current_Dim > &) const
promote< T, U >::V eval(const int N1, const int N2, const int N3, const Number< 1 > &) const
promote< T, U >::V eval(const int N1, const Number< 1 > &, const Number< Current_Dim1 > &) const
promote< T, U >::V operator()(const int N1) const
FTensor::Index< 'l', 3 > l
promote< T, U >::V operator()(const int N1) const