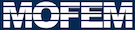 |
| v0.14.0
|
Go to the documentation of this file.
24 template <
class A,
class T,
int Dim01,
int Dim2,
char i,
char j,
char k>
31 T
operator()(
const int N1,
const int N2,
const int N3)
const
33 return iter(N1, N2, N3);
37 template <
class A,
class T,
int Tensor_Dim01,
int Tensor_Dim2,
int Dim01,
38 int Dim2,
char i,
char j,
char k>
39 class Dg_Expr<
Dg<
A, Tensor_Dim01, Tensor_Dim2>, T, Dim01, Dim2,
i,
j,
k>
47 return iter(N1, N2, N3);
49 T
operator()(
const int N1,
const int N2,
const int N3)
const
51 return iter(N1, N2, N3);
58 template <
class B,
class U>
66 template <
class B,
class U>
74 template <
class B,
class U>
84 operator=(
const U &
d);
92 template <
class A,
class T,
int Tensor_Dim0,
int Tensor_Dim12,
int Dim12,
93 int Dim0,
char i,
char j,
char k>
107 return iter(N3, N1, N2);
111 template <
class A,
class T,
int Tensor_Dim0,
int Tensor_Dim12,
int Dim12,
112 int Dim0,
char i,
char j,
char k>
127 return iter(N3, N1, N2);
130 template <
class B,
class U>
136 Dim0,
i,
j,
k> &result);
138 template <
class B,
class U>
144 Dim0,
i,
j,
k> &result);
146 template <
class B,
class U>
152 Dim0,
i,
j,
k> &result);
156 operator=(
const U &
d);
162 template <
class A,
class T,
int Dim23,
int Dim1,
char i,
char j,
char k,
172 return iter(N0, N1, N2, N3);
176 return iter(N0, N1, N2, N3);
183 template <
class B,
class U>
Dg_Expr(const Christof< A, Tensor_Dim0, Tensor_Dim12 > &a)
T operator()(const int N1, const int N2, const int N3) const
T operator()(const int N1, const int N2, const int N3) const
Dg_Expr(Christof< A, Tensor_Dim0, Tensor_Dim12 > &a)
T & operator()(const int N1, const int N2, const int N3)
const Tensor1_Expr< const dTensor0< T, Dim, i >, typename promote< T, double >::V, Dim, i > d(const Tensor0< T * > &a, const Index< i, Dim > index, const Tensor1< int, Dim > &d_ijk, const Tensor1< double, Dim > &d_xyz)
Christof< A, Tensor_Dim0, Tensor_Dim12 > & iter
Christof< A, Tensor_Dim0, Tensor_Dim12 > iter
T operator()(const int N1, const int N2, const int N3) const
Dg_Expr(Dg< A, Tensor_Dim01, Tensor_Dim2 > &a)
T operator()(const int N1, const int N2, const int N3) const
FTensor::Index< 'i', SPACE_DIM > i
FTensor::Index< 'j', 3 > j
T & operator()(const int N1, const int N2, const int N3)
T operator()(const int N1, const int N2, const int N3) const
Dg< A, Tensor_Dim01, Tensor_Dim2 > & iter
FTensor::Index< 'k', 3 > k