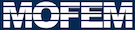 |
| v0.14.0
|
#include <users_modules/mortar_contact/src/clipper.hpp>
Definition at line 218 of file clipper.hpp.
◆ MinimaList
◆ ScanbeamList
◆ ClipperBase()
ClipperLib::ClipperBase::ClipperBase |
( |
| ) |
|
◆ ~ClipperBase()
ClipperLib::ClipperBase::~ClipperBase |
( |
| ) |
|
|
virtual |
◆ AddBoundsToLML()
TEdge* ClipperLib::ClipperBase::AddBoundsToLML |
( |
TEdge * |
e, |
|
|
bool |
IsClosed |
|
) |
| |
|
protected |
◆ AddPath()
Definition at line 1081 of file clipper.cpp.
1090 if (
E->Curr ==
E->Next->Curr && (Closed ||
E->Next != eStart))
1092 if (
E ==
E->Next)
break;
1093 if (
E == eStart) eStart =
E->Next;
1098 if (
E->Prev ==
E->Next)
1109 if (
E == eStart) eStart =
E->Next;
1116 if ((
E == eLoopStop) || (!Closed &&
E->Next == eStart))
break;
1119 if ((!Closed && (
E ==
E->Next)) || (Closed && (
E->Prev ==
E->Next)))
1128 eStart->Prev->OutIdx =
Skip;
1137 if (IsFlat &&
E->Curr.Y != eStart->Curr.Y) IsFlat =
false;
1139 while (
E != eStart);
1152 E->Prev->OutIdx =
Skip;
1153 MinimaList::value_type locMin;
1154 locMin.Y =
E->Bot.Y;
1155 locMin.LeftBound = 0;
1156 locMin.RightBound =
E;
1157 locMin.RightBound->Side =
esRight;
1158 locMin.RightBound->WindDelta = 0;
1162 if (
E->Next->OutIdx ==
Skip)
break;
1163 E->NextInLML =
E->Next;
1172 bool leftBoundIsForward;
1177 if (
E->Prev->Bot ==
E->Prev->Top)
E =
E->Next;
1182 if (
E == EMin)
break;
1183 else if (!EMin) EMin =
E;
1187 MinimaList::value_type locMin;
1188 locMin.Y =
E->Bot.Y;
1189 if (
E->Dx <
E->Prev->Dx)
1191 locMin.LeftBound =
E->Prev;
1192 locMin.RightBound =
E;
1193 leftBoundIsForward =
false;
1196 locMin.LeftBound =
E;
1197 locMin.RightBound =
E->Prev;
1198 leftBoundIsForward =
true;
1201 if (!Closed) locMin.LeftBound->WindDelta = 0;
1202 else if (locMin.LeftBound->Next == locMin.RightBound)
1203 locMin.LeftBound->WindDelta = -1;
1204 else locMin.LeftBound->WindDelta = 1;
1205 locMin.RightBound->WindDelta = -locMin.LeftBound->WindDelta;
1210 TEdge* E2 =
ProcessBound(locMin.RightBound, !leftBoundIsForward);
1213 if (locMin.LeftBound->OutIdx ==
Skip)
1214 locMin.LeftBound = 0;
1215 else if (locMin.RightBound->OutIdx ==
Skip)
1216 locMin.RightBound = 0;
1218 if (!leftBoundIsForward)
E = E2;
1226 bool result =
false;
1227 for (Paths::size_type
i = 0;
i < ppg.size(); ++
i)
1228 if (
AddPath(ppg[
i], PolyTyp, Closed)) result =
true;
1236 for (EdgeList::size_type
i = 0;
i <
m_edges.size(); ++
i)
◆ AddPaths()
◆ Clear()
void ClipperLib::ClipperBase::Clear |
( |
| ) |
|
|
virtual |
◆ CreateOutRec()
OutRec * ClipperLib::ClipperBase::CreateOutRec |
( |
| ) |
|
|
protected |
Definition at line 1416 of file clipper.cpp.
1425 TEdge* Next = Edge1->NextInAEL;
1426 TEdge* Prev = Edge1->PrevInAEL;
1427 Edge1->NextInAEL = Edge2->NextInAEL;
1428 if (Edge1->NextInAEL) Edge1->NextInAEL->PrevInAEL = Edge1;
◆ DeleteFromAEL()
void ClipperLib::ClipperBase::DeleteFromAEL |
( |
TEdge * |
e | ) |
|
|
protected |
◆ DisposeAllOutRecs()
void ClipperLib::ClipperBase::DisposeAllOutRecs |
( |
| ) |
|
|
protected |
◆ DisposeLocalMinimaList()
void ClipperLib::ClipperBase::DisposeLocalMinimaList |
( |
| ) |
|
|
protected |
Definition at line 1315 of file clipper.cpp.
1317 if (e->Bot.X < result.left) result.left = e->Bot.X;
1318 if (e->Bot.X > result.right) result.right = e->Bot.X;
◆ DisposeOutRec()
void ClipperLib::ClipperBase::DisposeOutRec |
( |
PolyOutList::size_type |
index | ) |
|
|
protected |
Definition at line 1394 of file clipper.cpp.
1398 if (Edge1->NextInAEL == Edge1->PrevInAEL ||
1399 Edge2->NextInAEL == Edge2->PrevInAEL)
return;
◆ GetBounds()
IntRect ClipperLib::ClipperBase::GetBounds |
( |
| ) |
|
◆ InsertScanbeam()
void ClipperLib::ClipperBase::InsertScanbeam |
( |
const cInt |
Y | ) |
|
|
protected |
◆ LocalMinimaPending()
bool ClipperLib::ClipperBase::LocalMinimaPending |
( |
| ) |
|
|
protected |
Definition at line 1501 of file clipper.cpp.
1504 return Execute(clipType, polytree, fillType, fillType);
◆ PopLocalMinima()
◆ PopScanbeam()
bool ClipperLib::ClipperBase::PopScanbeam |
( |
cInt & |
Y | ) |
|
|
protected |
Definition at line 1377 of file clipper.cpp.
1382 OutRec* result =
new OutRec;
1383 result->IsHole =
false;
1384 result->IsOpen =
false;
◆ PreserveCollinear() [1/2]
bool ClipperLib::ClipperBase::PreserveCollinear |
( |
| ) |
|
|
inline |
◆ PreserveCollinear() [2/2]
void ClipperLib::ClipperBase::PreserveCollinear |
( |
bool |
value | ) |
|
|
inline |
◆ ProcessBound()
TEdge * ClipperLib::ClipperBase::ProcessBound |
( |
TEdge * |
E, |
|
|
bool |
IsClockwise |
|
) |
| |
|
protected |
Definition at line 964 of file clipper.cpp.
986 if (EStart->Bot.X !=
E->Bot.X && EStart->Top.X !=
E->Bot.X)
989 else if (EStart->Bot.X !=
E->Bot.X)
996 while (Result->Top.Y == Result->Next->Bot.Y && Result->Next->OutIdx !=
Skip)
997 Result = Result->Next;
1005 if (Horz->Prev->Top.X > Result->Next->Top.X) Result = Horz->Prev;
1009 E->NextInLML =
E->Next;
1016 Result = Result->Next;
1019 while (Result->Top.Y == Result->Prev->Bot.Y && Result->Prev->OutIdx !=
Skip)
1020 Result = Result->Prev;
1025 if (Horz->Next->Top.X == Result->Prev->Top.X ||
1026 Horz->Next->Top.X > Result->Prev->Top.X) Result = Horz->Next;
1031 E->NextInLML =
E->Prev;
1038 Result = Result->Prev;
1048 if (!Closed && PolyTyp ==
ptClip)
1049 throw clipperException(
"AddPath: Open paths must be subject.");
1052 throw clipperException(
"AddPath: Open paths have been disabled.");
1055 int highI = (
int)pg.size() -1;
1056 if (Closed)
while (highI > 0 && (pg[highI] == pg[0])) --highI;
1057 while (highI > 0 && (pg[highI] == pg[highI -1])) --highI;
1058 if ((Closed && highI < 2) || (!Closed && highI < 1))
return false;
1061 TEdge *edges =
new TEdge [highI +1];
1067 edges[1].Curr = pg[1];
1070 InitEdge(&edges[0], &edges[1], &edges[highI], pg[0]);
1071 InitEdge(&edges[highI], &edges[0], &edges[highI-1], pg[highI]);
1072 for (
int i = highI - 1;
i >= 1; --
i)
◆ Reset()
void ClipperLib::ClipperBase::Reset |
( |
| ) |
|
|
protectedvirtual |
Definition at line 1283 of file clipper.cpp.
1289 locMin = &(*m_CurrentLM);
1301 result.left = result.top = result.right = result.bottom = 0;
1304 result.left = lm->LeftBound->Bot.X;
1305 result.top = lm->LeftBound->Bot.Y;
1306 result.right = lm->LeftBound->Bot.X;
1307 result.bottom = lm->LeftBound->Bot.Y;
1311 result.bottom = std::max(result.bottom, lm->LeftBound->Bot.Y);
1312 TEdge* e = lm->LeftBound;
◆ SwapPositionsInAEL()
void ClipperLib::ClipperBase::SwapPositionsInAEL |
( |
TEdge * |
edge1, |
|
|
TEdge * |
edge2 |
|
) |
| |
|
protected |
Definition at line 1431 of file clipper.cpp.
1445 throw clipperException(
"UpdateEdgeIntoAEL: invalid call");
1447 e->NextInLML->OutIdx = e->OutIdx;
1448 TEdge* AelPrev = e->PrevInAEL;
1449 TEdge* AelNext = e->NextInAEL;
1450 if (AelPrev) AelPrev->NextInAEL = e->NextInLML;
1454 e->NextInLML->WindDelta = e->WindDelta;
1455 e->NextInLML->WindCnt = e->WindCnt;
1456 e->NextInLML->WindCnt2 = e->WindCnt2;
1459 e->PrevInAEL = AelPrev;
1460 e->NextInAEL = AelNext;
◆ UpdateEdgeIntoAEL()
void ClipperLib::ClipperBase::UpdateEdgeIntoAEL |
( |
TEdge *& |
e | ) |
|
|
protected |
Definition at line 1478 of file clipper.cpp.
1491 m_ZFill = zFillFunc;
1498 return Execute(clipType, solution, fillType, fillType);
◆ m_ActiveEdges
TEdge* ClipperLib::ClipperBase::m_ActiveEdges |
|
protected |
◆ m_CurrentLM
MinimaList::iterator ClipperLib::ClipperBase::m_CurrentLM |
|
protected |
◆ m_edges
EdgeList ClipperLib::ClipperBase::m_edges |
|
protected |
◆ m_HasOpenPaths
bool ClipperLib::ClipperBase::m_HasOpenPaths |
|
protected |
◆ m_MinimaList
◆ m_PolyOuts
◆ m_PreserveCollinear
bool ClipperLib::ClipperBase::m_PreserveCollinear |
|
protected |
◆ m_Scanbeam
◆ m_UseFullRange
bool ClipperLib::ClipperBase::m_UseFullRange |
|
protected |
The documentation for this class was generated from the following files:
TEdge * FindNextLocMin(TEdge *E)
bool PopScanbeam(cInt &Y)
std::vector< IntPoint > Path
std::vector< Path > Paths
bool SlopesEqual(const TEdge &e1, const TEdge &e2, bool UseFullInt64Range)
void DisposeOutPts(OutPt *&pp)
void RangeTest(const IntPoint &Pt, bool &useFullRange)
TEdge * ProcessBound(TEdge *E, bool IsClockwise)
bool Execute(ClipType clipType, Paths &solution, PolyFillType fillType=pftEvenOdd)
void ReverseHorizontal(TEdge &e)
TEdge * RemoveEdge(TEdge *e)
void InsertScanbeam(const cInt Y)
virtual bool AddPath(const Path &pg, PolyType PolyTyp, bool Closed)
bool LocalMinimaPending()
void DeleteFromAEL(TEdge *e)
Clipper(int initOptions=0)
void DisposeOutRec(PolyOutList::size_type index)
void InitEdge2(TEdge &e, PolyType Pt)
static const int Unassigned
void DisposeLocalMinimaList()
FTensor::Index< 'i', SPACE_DIM > i
MinimaList::iterator m_CurrentLM
bool AddPaths(const Paths &ppg, PolyType PolyTyp, bool Closed)
std::priority_queue< cInt > ScanbeamList
void InitEdge(TEdge *e, TEdge *eNext, TEdge *ePrev, const IntPoint &Pt)
bool IsHorizontal(TEdge &e)
bool Pt2IsBetweenPt1AndPt3(const IntPoint pt1, const IntPoint pt2, const IntPoint pt3)