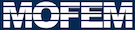 |
| v0.14.0
|
#include <users_modules/mortar_contact/src/clipper.hpp>
|
| Clipper (int initOptions=0) |
|
bool | Execute (ClipType clipType, Paths &solution, PolyFillType fillType=pftEvenOdd) |
|
bool | Execute (ClipType clipType, Paths &solution, PolyFillType subjFillType, PolyFillType clipFillType) |
|
bool | Execute (ClipType clipType, PolyTree &polytree, PolyFillType fillType=pftEvenOdd) |
|
bool | Execute (ClipType clipType, PolyTree &polytree, PolyFillType subjFillType, PolyFillType clipFillType) |
|
bool | ReverseSolution () |
|
void | ReverseSolution (bool value) |
|
bool | StrictlySimple () |
|
void | StrictlySimple (bool value) |
|
| ClipperBase () |
|
virtual | ~ClipperBase () |
|
virtual bool | AddPath (const Path &pg, PolyType PolyTyp, bool Closed) |
|
bool | AddPaths (const Paths &ppg, PolyType PolyTyp, bool Closed) |
|
virtual void | Clear () |
|
IntRect | GetBounds () |
|
bool | PreserveCollinear () |
|
void | PreserveCollinear (bool value) |
|
|
void | SetWindingCount (TEdge &edge) |
|
bool | IsEvenOddFillType (const TEdge &edge) const |
|
bool | IsEvenOddAltFillType (const TEdge &edge) const |
|
void | InsertLocalMinimaIntoAEL (const cInt botY) |
|
void | InsertEdgeIntoAEL (TEdge *edge, TEdge *startEdge) |
|
void | AddEdgeToSEL (TEdge *edge) |
|
bool | PopEdgeFromSEL (TEdge *&edge) |
|
void | CopyAELToSEL () |
|
void | DeleteFromSEL (TEdge *e) |
|
void | SwapPositionsInSEL (TEdge *edge1, TEdge *edge2) |
|
bool | IsContributing (const TEdge &edge) const |
|
bool | IsTopHorz (const cInt XPos) |
|
void | DoMaxima (TEdge *e) |
|
void | ProcessHorizontals () |
|
void | ProcessHorizontal (TEdge *horzEdge) |
|
void | AddLocalMaxPoly (TEdge *e1, TEdge *e2, const IntPoint &pt) |
|
OutPt * | AddLocalMinPoly (TEdge *e1, TEdge *e2, const IntPoint &pt) |
|
OutRec * | GetOutRec (int idx) |
|
void | AppendPolygon (TEdge *e1, TEdge *e2) |
|
void | IntersectEdges (TEdge *e1, TEdge *e2, IntPoint &pt) |
|
OutPt * | AddOutPt (TEdge *e, const IntPoint &pt) |
|
OutPt * | GetLastOutPt (TEdge *e) |
|
bool | ProcessIntersections (const cInt topY) |
|
void | BuildIntersectList (const cInt topY) |
|
void | ProcessIntersectList () |
|
void | ProcessEdgesAtTopOfScanbeam (const cInt topY) |
|
void | BuildResult (Paths &polys) |
|
void | BuildResult2 (PolyTree &polytree) |
|
void | SetHoleState (TEdge *e, OutRec *outrec) |
|
void | DisposeIntersectNodes () |
|
bool | FixupIntersectionOrder () |
|
void | FixupOutPolygon (OutRec &outrec) |
|
void | FixupOutPolyline (OutRec &outrec) |
|
bool | IsHole (TEdge *e) |
|
bool | FindOwnerFromSplitRecs (OutRec &outRec, OutRec *&currOrfl) |
|
void | FixHoleLinkage (OutRec &outrec) |
|
void | AddJoin (OutPt *op1, OutPt *op2, const IntPoint offPt) |
|
void | ClearJoins () |
|
void | ClearGhostJoins () |
|
void | AddGhostJoin (OutPt *op, const IntPoint offPt) |
|
bool | JoinPoints (Join *j, OutRec *outRec1, OutRec *outRec2) |
|
void | JoinCommonEdges () |
|
void | DoSimplePolygons () |
|
void | FixupFirstLefts1 (OutRec *OldOutRec, OutRec *NewOutRec) |
|
void | FixupFirstLefts2 (OutRec *InnerOutRec, OutRec *OuterOutRec) |
|
void | FixupFirstLefts3 (OutRec *OldOutRec, OutRec *NewOutRec) |
|
Definition at line 261 of file clipper.hpp.
◆ MaximaList
◆ Clipper()
ClipperLib::Clipper::Clipper |
( |
int |
initOptions = 0 | ) |
|
Definition at line 1510 of file clipper.cpp.
1513 throw clipperException(
"Error: PolyTree struct is needed for open path clipping.");
◆ AddEdgeToSEL()
void ClipperLib::Clipper::AddEdgeToSEL |
( |
TEdge * |
edge | ) |
|
|
private |
◆ AddGhostJoin()
void ClipperLib::Clipper::AddGhostJoin |
( |
OutPt * |
op, |
|
|
const IntPoint |
offPt |
|
) |
| |
|
private |
Definition at line 2004 of file clipper.cpp.
2008 rb->WindCnt = lb->WindCnt;
2009 rb->WindCnt2 = lb->WindCnt2;
◆ AddJoin()
Definition at line 1978 of file clipper.cpp.
1980 const LocalMinimum *lm;
1983 TEdge* lb = lm->LeftBound;
1984 TEdge* rb = lm->RightBound;
◆ AddLocalMaxPoly()
◆ AddLocalMinPoly()
Definition at line 1877 of file clipper.cpp.
1887 if (e2->WindDelta == 0)
AddOutPt(e2, Pt);
1888 if( e1->OutIdx == e2->OutIdx )
1893 else if (e1->OutIdx < e2->OutIdx)
◆ AddOutPt()
Definition at line 2499 of file clipper.cpp.
2508 return outRec->Pts->Prev;
2522 return e && (e->Prev->NextInLML != e) && (e->Next->NextInLML != e);
2528 return e && e->Top.Y == Y && !e->NextInLML;
2534 return e->Top.Y == Y && e->NextInLML;
◆ AppendPolygon()
void ClipperLib::Clipper::AppendPolygon |
( |
TEdge * |
e1, |
|
|
TEdge * |
e2 |
|
) |
| |
|
private |
Definition at line 2403 of file clipper.cpp.
2416 p1_rt->Next = p2_rt;
2417 p2_rt->Prev = p1_rt;
2418 p2_lft->Next = p1_lft;
2419 p1_lft->Prev = p2_lft;
2423 p1_rt->Next = p2_lft;
2424 p2_lft->Prev = p1_rt;
2425 p1_lft->Prev = p2_rt;
2426 p2_rt->Next = p1_lft;
2430 outRec1->BottomPt = 0;
2431 if (holeStateRec == outRec2)
2433 if (outRec2->FirstLeft != outRec1)
2434 outRec1->FirstLeft = outRec2->FirstLeft;
2435 outRec1->IsHole = outRec2->IsHole;
2438 outRec2->BottomPt = 0;
2439 outRec2->FirstLeft = outRec1;
2441 int OKIdx = e1->OutIdx;
2442 int ObsoleteIdx = e2->OutIdx;
2450 if( e->OutIdx == ObsoleteIdx )
2459 outRec2->Idx = outRec1->Idx;
2468 outRec->IsOpen = (e->WindDelta == 0);
2469 OutPt* newOp =
new OutPt;
2470 outRec->Pts = newOp;
2471 newOp->Idx = outRec->Idx;
2473 newOp->Next = newOp;
2474 newOp->Prev = newOp;
2475 if (!outRec->IsOpen)
2477 e->OutIdx = outRec->Idx;
2483 OutPt* op = outRec->Pts;
2485 bool ToFront = (e->Side ==
esLeft);
2486 if (ToFront && (pt == op->Pt))
return op;
2487 else if (!ToFront && (pt == op->Prev->Pt))
return op->Prev;
2489 OutPt* newOp =
new OutPt;
2490 newOp->Idx = outRec->Idx;
2493 newOp->Prev = op->Prev;
2494 newOp->Prev->Next = newOp;
2496 if (ToFront) outRec->Pts = newOp;
◆ BuildIntersectList()
void ClipperLib::Clipper::BuildIntersectList |
( |
const cInt |
topY | ) |
|
|
private |
Definition at line 2888 of file clipper.cpp.
2919 return node2->Pt.Y < node1->Pt.Y;
2925 return (inode.Edge1->NextInSEL == inode.Edge2) ||
2926 (inode.Edge1->PrevInSEL == inode.Edge2);
◆ BuildResult()
void ClipperLib::Clipper::BuildResult |
( |
Paths & |
polys | ) |
|
|
private |
Definition at line 3228 of file clipper.cpp.
3234 pn->Contour.push_back(op->Pt);
3241 for (PolyOutList::size_type
i = 0;
i <
m_PolyOuts.size();
i++)
3244 if (!outRec->PolyNd)
continue;
◆ BuildResult2()
void ClipperLib::Clipper::BuildResult2 |
( |
PolyTree & |
polytree | ) |
|
|
private |
Definition at line 3249 of file clipper.cpp.
3261 IntersectNode inode = int1;
3262 int1.Edge1 = int2.Edge1;
3263 int1.Edge2 = int2.Edge2;
3265 int2.Edge1 = inode.Edge1;
3266 int2.Edge2 = inode.Edge2;
3273 if (e2.Curr.X == e1.Curr.X)
3275 if (e2.Top.Y > e1.Top.Y)
3276 return e2.Top.X <
TopX(e1, e2.Top.Y);
3277 else return e1.Top.X >
TopX(e2, e1.Top.Y);
3279 else return e2.Curr.X < e1.Curr.X;
3288 if (b1 < b2) {Left = std::max(
a1,b1); Right = std::min(
a2,b2);}
3289 else {Left = std::max(
a1,b2); Right = std::min(
a2,b1);}
◆ ClearGhostJoins()
void ClipperLib::Clipper::ClearGhostJoins |
( |
| ) |
|
|
private |
◆ ClearJoins()
void ClipperLib::Clipper::ClearJoins |
( |
| ) |
|
|
private |
◆ CopyAELToSEL()
void ClipperLib::Clipper::CopyAELToSEL |
( |
| ) |
|
|
private |
◆ DeleteFromSEL()
void ClipperLib::Clipper::DeleteFromSEL |
( |
TEdge * |
e | ) |
|
|
private |
Definition at line 2116 of file clipper.cpp.
2121 if (e1->WindDelta == 0 && e2->WindDelta == 0)
return;
2124 else if (e1->PolyTyp == e2->PolyTyp &&
◆ DisposeIntersectNodes()
void ClipperLib::Clipper::DisposeIntersectNodes |
( |
| ) |
|
|
private |
◆ DoMaxima()
void ClipperLib::Clipper::DoMaxima |
( |
TEdge * |
e | ) |
|
|
private |
Definition at line 2989 of file clipper.cpp.
3001 else throw clipperException(
"DoMaxima error");
3012 bool IsMaximaEdge =
IsMaxima(e, topY);
3017 IsMaximaEdge = (!eMaxPair || !
IsHorizontal(*eMaxPair));
3023 TEdge* ePrev = e->PrevInAEL;
◆ DoSimplePolygons()
void ClipperLib::Clipper::DoSimplePolygons |
( |
| ) |
|
|
private |
Definition at line 4250 of file clipper.cpp.
4252 outrec2->IsHole = outrec->IsHole;
4253 outrec->IsHole = !outrec2->IsHole;
4254 outrec2->FirstLeft = outrec->FirstLeft;
4255 outrec->FirstLeft = outrec2;
4261 outrec2->IsHole = outrec->IsHole;
4262 outrec2->FirstLeft = outrec->FirstLeft;
4271 while (op != outrec->Pts);
4278 std::reverse(p.begin(), p.end());
4284 for (Paths::size_type
i = 0;
i < p.size(); ++
i)
4292 c.StrictlySimple(
true);
4294 c.Execute(
ctUnion, out_polys, fillType, fillType);
4301 c.StrictlySimple(
true);
4303 c.Execute(
ctUnion, out_polys, fillType, fillType);
◆ Execute() [1/4]
◆ Execute() [2/4]
Definition at line 1544 of file clipper.cpp.
1549 if (!outrec.FirstLeft ||
1550 (outrec.IsHole != outrec.FirstLeft->IsHole &&
1551 outrec.FirstLeft->Pts))
return;
1553 OutRec* orfl = outrec.FirstLeft;
1554 while (orfl && ((orfl->IsHole == outrec.IsHole) || !orfl->Pts))
1555 orfl = orfl->FirstLeft;
1556 outrec.FirstLeft = orfl;
◆ Execute() [3/4]
◆ Execute() [4/4]
◆ ExecuteInternal()
bool ClipperLib::Clipper::ExecuteInternal |
( |
| ) |
|
|
protectedvirtual |
Definition at line 1596 of file clipper.cpp.
1608 if (!outRec->Pts)
continue;
1626 TEdge *e = edge.PrevInAEL;
1628 while (e && ((e->PolyTyp != edge.PolyTyp) || (e->WindDelta == 0))) e = e->PrevInAEL;
1631 if (edge.WindDelta == 0)
1637 edge.WindCnt = edge.WindDelta;
1644 edge.WindCnt2 = e->WindCnt2;
1650 if (edge.WindDelta == 0)
1654 TEdge *e2 = e->PrevInAEL;
1657 if (e2->PolyTyp == e->PolyTyp && e2->WindDelta != 0)
◆ FindOwnerFromSplitRecs()
bool ClipperLib::Clipper::FindOwnerFromSplitRecs |
( |
OutRec & |
outRec, |
|
|
OutRec *& |
currOrfl |
|
) |
| |
|
private |
◆ FixHoleLinkage()
void ClipperLib::Clipper::FixHoleLinkage |
( |
OutRec & |
outrec | ) |
|
|
private |
◆ FixupFirstLefts1()
void ClipperLib::Clipper::FixupFirstLefts1 |
( |
OutRec * |
OldOutRec, |
|
|
OutRec * |
NewOutRec |
|
) |
| |
|
private |
Definition at line 3654 of file clipper.cpp.
3662 for (PolyOutList::size_type
i = 0;
i <
m_PolyOuts.size(); ++
i)
3666 if (outRec->Pts && outRec->FirstLeft == OldOutRec)
3667 outRec->FirstLeft = NewOutRec;
◆ FixupFirstLefts2()
void ClipperLib::Clipper::FixupFirstLefts2 |
( |
OutRec * |
InnerOutRec, |
|
|
OutRec * |
OuterOutRec |
|
) |
| |
|
private |
Definition at line 3670 of file clipper.cpp.
3674 for (JoinList::size_type
i = 0;
i <
m_Joins.size();
i++)
3678 OutRec *outRec1 =
GetOutRec(join->OutPt1->Idx);
3679 OutRec *outRec2 =
GetOutRec(join->OutPt2->Idx);
3681 if (!outRec1->Pts || !outRec2->Pts)
continue;
3682 if (outRec1->IsOpen || outRec2->IsOpen)
continue;
3686 OutRec *holeStateRec;
3687 if (outRec1 == outRec2) holeStateRec = outRec1;
3692 if (!
JoinPoints(join, outRec1, outRec2))
continue;
◆ FixupFirstLefts3()
void ClipperLib::Clipper::FixupFirstLefts3 |
( |
OutRec * |
OldOutRec, |
|
|
OutRec * |
NewOutRec |
|
) |
| |
|
private |
Definition at line 3695 of file clipper.cpp.
3698 outRec1->Pts = join->OutPt1;
3699 outRec1->BottomPt = 0;
3701 outRec2->Pts = join->OutPt2;
◆ FixupIntersectionOrder()
bool ClipperLib::Clipper::FixupIntersectionOrder |
( |
| ) |
|
|
private |
Definition at line 2966 of file clipper.cpp.
2969 eNext = e->NextInAEL;
2977 else if( e->OutIdx >= 0 && eMaxPair->OutIdx >= 0 )
2984 else if (e->WindDelta == 0)
◆ FixupOutPolygon()
void ClipperLib::Clipper::FixupOutPolygon |
( |
OutRec & |
outrec | ) |
|
|
private |
Definition at line 3172 of file clipper.cpp.
3195 for (PolyOutList::size_type
i = 0;
i <
m_PolyOuts.size(); ++
i)
3201 if (cnt < 2)
continue;
3203 for (
int i = 0;
i < cnt; ++
i)
3205 pg.push_back(p->Pt);
3208 polys.push_back(pg);
◆ FixupOutPolyline()
void ClipperLib::Clipper::FixupOutPolyline |
( |
OutRec & |
outrec | ) |
|
|
private |
Definition at line 3145 of file clipper.cpp.
3147 if (pp->Prev == pp || pp->Prev == pp->Next)
3155 if ((pp->Pt == pp->Next->Pt) || (pp->Pt == pp->Prev->Pt) ||
3161 pp->Prev->Next = pp->Next;
3162 pp->Next->Prev = pp->Prev;
3166 else if (pp == lastOK)
break;
3169 if (!lastOK) lastOK = pp;
◆ GetLastOutPt()
OutPt * ClipperLib::Clipper::GetLastOutPt |
( |
TEdge * |
e | ) |
|
|
private |
Definition at line 2538 of file clipper.cpp.
2540 if ((e->Next->Top == e->Top) && !e->Next->NextInLML)
2542 else if ((e->Prev->Top == e->Top) && !e->Prev->NextInLML)
◆ GetOutRec()
OutRec * ClipperLib::Clipper::GetOutRec |
( |
int |
idx | ) |
|
|
private |
◆ InsertEdgeIntoAEL()
void ClipperLib::Clipper::InsertEdgeIntoAEL |
( |
TEdge * |
edge, |
|
|
TEdge * |
startEdge |
|
) |
| |
|
private |
Definition at line 3348 of file clipper.cpp.
3355 result->Prev = outPt->Prev;
3356 result->Next = outPt;
3357 outPt->Prev->Next = result;
3358 outPt->Prev = result;
3364 bool JoinHorz(OutPt* op1, OutPt* op1b, OutPt* op2, OutPt* op2b,
3365 const IntPoint Pt,
bool DiscardLeft)
3369 if (Dir1 == Dir2)
return false;
◆ InsertLocalMinimaIntoAEL()
void ClipperLib::Clipper::InsertLocalMinimaIntoAEL |
( |
const cInt |
botY | ) |
|
|
private |
Definition at line 2014 of file clipper.cpp.
2026 if (!lb || !rb)
continue;
2038 AddJoin(jr->OutPt1, Op1, jr->OffPt);
2042 if (lb->OutIdx >= 0 && lb->PrevInAEL &&
2043 lb->PrevInAEL->Curr.X == lb->Bot.X &&
2044 lb->PrevInAEL->OutIdx >= 0 &&
2046 (lb->WindDelta != 0) && (lb->PrevInAEL->WindDelta != 0))
2048 OutPt *Op2 =
AddOutPt(lb->PrevInAEL, lb->Bot);
2052 if(lb->NextInAEL != rb)
2055 if (rb->OutIdx >= 0 && rb->PrevInAEL->OutIdx >= 0 &&
2057 (rb->WindDelta != 0) && (rb->PrevInAEL->WindDelta != 0))
2059 OutPt *Op2 =
AddOutPt(rb->PrevInAEL, rb->Bot);
2063 TEdge* e = lb->NextInAEL;
2082 TEdge* SelPrev = e->PrevInSEL;
2083 TEdge* SelNext = e->NextInSEL;
2085 if( SelPrev ) SelPrev->NextInSEL = SelNext;
2087 if( SelNext ) SelNext->
PrevInSEL = SelPrev;
2094 void Clipper::SetZ(IntPoint& pt, TEdge& e1, TEdge& e2)
2096 if (pt.Z != 0 || !m_ZFill)
return;
2097 else if (pt == e1.Bot) pt.Z = e1.
Bot.Z;
2098 else if (pt == e1.Top) pt.Z = e1.Top.Z;
2099 else if (pt == e2.Bot) pt.Z = e2.Bot.Z;
2100 else if (pt == e2.Top) pt.Z = e2.Top.Z;
2101 else (*m_ZFill)(e1.Bot, e1.Top, e2.Bot, e2.Top, pt);
2108 bool e1Contributing = ( e1->OutIdx >= 0 );
2109 bool e2Contributing = ( e2->OutIdx >= 0 );
◆ IntersectEdges()
Definition at line 2142 of file clipper.cpp.
2147 if ((e1->WindDelta == 0) && abs(e2->WindCnt) == 1 &&
2153 else if ((e2->WindDelta == 0) && (abs(e1->WindCnt) == 1) &&
2166 if ( e1->PolyTyp == e2->PolyTyp )
2170 int oldE1WindCnt = e1->WindCnt;
2171 e1->WindCnt = e2->WindCnt;
2172 e2->WindCnt = oldE1WindCnt;
2175 if (e1->WindCnt + e2->WindDelta == 0 ) e1->WindCnt = -e1->WindCnt;
2176 else e1->WindCnt += e2->WindDelta;
2177 if ( e2->WindCnt - e1->WindDelta == 0 ) e2->WindCnt = -e2->WindCnt;
2178 else e2->WindCnt -= e1->WindDelta;
2183 else e1->WindCnt2 = ( e1->WindCnt2 == 0 ) ? 1 : 0;
2185 else e2->WindCnt2 = ( e2->WindCnt2 == 0 ) ? 1 : 0;
2188 PolyFillType e1FillType, e2FillType, e1FillType2, e2FillType2;
2213 default: e1Wc =
Abs(e1->WindCnt);
2219 default: e2Wc =
Abs(e2->WindCnt);
2222 if ( e1Contributing && e2Contributing )
2224 if ((e1Wc != 0 && e1Wc != 1) || (e2Wc != 0 && e2Wc != 1) ||
2237 else if ( e1Contributing )
2239 if (e2Wc == 0 || e2Wc == 1)
2246 else if ( e2Contributing )
2248 if (e1Wc == 0 || e1Wc == 1)
2255 else if ( (e1Wc == 0 || e1Wc == 1) && (e2Wc == 0 || e2Wc == 1))
2260 switch (e1FillType2)
2264 default: e1Wc2 =
Abs(e1->WindCnt2);
2266 switch (e2FillType2)
2270 default: e2Wc2 =
Abs(e2->WindCnt2);
2273 if (e1->PolyTyp != e2->PolyTyp)
2277 else if (e1Wc == 1 && e2Wc == 1)
2280 if (e1Wc2 > 0 && e2Wc2 > 0)
2284 if ( e1Wc2 <= 0 && e2Wc2 <= 0 )
2288 if (((e1->PolyTyp ==
ptClip) && (e1Wc2 > 0) && (e2Wc2 > 0)) ||
2289 ((e1->PolyTyp ==
ptSubject) && (e1Wc2 <= 0) && (e2Wc2 <= 0)))
2303 TEdge *e2 = e->PrevInAEL;
2307 if (e2->OutIdx >= 0 && e2->WindDelta != 0)
2309 if (!eTmp) eTmp = e2;
2310 else if (eTmp->OutIdx == e2->OutIdx) eTmp = 0;
2316 outrec->FirstLeft = 0;
2317 outrec->IsHole =
false;
2321 outrec->FirstLeft =
m_PolyOuts[eTmp->OutIdx];
2322 outrec->IsHole = !outrec->FirstLeft->IsHole;
2330 if (!outRec1->BottomPt)
2332 if (!outRec2->BottomPt)
2334 OutPt *OutPt1 = outRec1->BottomPt;
◆ IsContributing()
bool ClipperLib::Clipper::IsContributing |
( |
const TEdge & |
edge | ) |
const |
|
private |
Definition at line 1777 of file clipper.cpp.
1779 return (edge.WindCnt2 > 0);
1781 return (edge.WindCnt2 < 0);
1789 return (edge.WindCnt2 == 0);
1791 return (edge.WindCnt2 <= 0);
1793 return (edge.WindCnt2 >= 0);
1802 return (edge.WindCnt2 == 0);
1804 return (edge.WindCnt2 <= 0);
1806 return (edge.WindCnt2 >= 0);
1813 return (edge.WindCnt2 != 0);
1815 return (edge.WindCnt2 > 0);
1817 return (edge.WindCnt2 < 0);
1821 if (edge.WindDelta == 0)
1826 return (edge.WindCnt2 == 0);
1828 return (edge.WindCnt2 <= 0);
1830 return (edge.WindCnt2 >= 0);
1848 e2->OutIdx = e1->OutIdx;
1852 if (e->PrevInAEL == e2)
1853 prevE = e2->PrevInAEL;
1855 prevE = e->PrevInAEL;
1859 e1->OutIdx = e2->OutIdx;
1863 if (e->PrevInAEL == e1)
1864 prevE = e1->PrevInAEL;
1866 prevE = e->PrevInAEL;
1869 if (prevE && prevE->OutIdx >= 0)
1873 if (xPrev == xE && (e->WindDelta != 0) && (prevE->WindDelta != 0) &&
◆ IsEvenOddAltFillType()
bool ClipperLib::Clipper::IsEvenOddAltFillType |
( |
const TEdge & |
edge | ) |
const |
|
private |
◆ IsEvenOddFillType()
bool ClipperLib::Clipper::IsEvenOddFillType |
( |
const TEdge & |
edge | ) |
const |
|
private |
Definition at line 1761 of file clipper.cpp.
1764 if (edge.WindCnt != 1)
return false;
◆ IsHole()
bool ClipperLib::Clipper::IsHole |
( |
TEdge * |
e | ) |
|
|
private |
◆ IsTopHorz()
bool ClipperLib::Clipper::IsTopHorz |
( |
const cInt |
XPos | ) |
|
|
private |
◆ JoinCommonEdges()
void ClipperLib::Clipper::JoinCommonEdges |
( |
| ) |
|
|
private |
Definition at line 3708 of file clipper.cpp.
3720 outRec2->IsHole = outRec1->IsHole;
3721 outRec1->IsHole = !outRec2->IsHole;
3722 outRec2->FirstLeft = outRec1->FirstLeft;
3723 outRec1->FirstLeft = outRec2;
3733 outRec2->IsHole = outRec1->IsHole;
3734 outRec2->FirstLeft = outRec1->FirstLeft;
3745 outRec2->BottomPt = 0;
3746 outRec2->Idx = outRec1->Idx;
3748 outRec1->IsHole = holeStateRec->IsHole;
3749 if (holeStateRec == outRec2)
3750 outRec1->FirstLeft = outRec2->FirstLeft;
3751 outRec2->FirstLeft = outRec1;
3762 DoublePoint
GetUnitNormal(
const IntPoint &pt1,
const IntPoint &pt2)
3764 if(pt2.X == pt1.X && pt2.Y == pt1.Y)
3765 return DoublePoint(0, 0);
3767 double Dx = (
double)(pt2.X - pt1.X);
3768 double dy = (
double)(pt2.Y - pt1.Y);
3769 double f = 1 *1.0/ std::sqrt( Dx*Dx + dy*dy );
3772 return DoublePoint(dy, -Dx);
3781 this->MiterLimit = miterLimit;
3782 this->ArcTolerance = arcTolerance;
◆ JoinPoints()
Definition at line 3487 of file clipper.cpp.
3503 else if (isHorizontal)
3509 while (op1->Prev->Pt.Y == op1->Pt.Y && op1->Prev != op1b && op1->Prev != op2)
3511 while (op1b->Next->Pt.Y == op1b->Pt.Y && op1b->Next != op1 && op1b->Next != op2)
3513 if (op1b->Next == op1 || op1b->Next == op2)
return false;
3516 while (op2->Prev->Pt.Y == op2->Pt.Y && op2->Prev != op2b && op2->Prev != op1b)
3518 while (op2b->Next->Pt.Y == op2b->Pt.Y && op2b->Next != op2 && op2b->Next != op1)
3520 if (op2b->Next == op2 || op2b->Next == op1)
return false;
3524 if (!
GetOverlap(op1->Pt.X, op1b->Pt.X, op2->Pt.X, op2b->Pt.X, Left, Right))
3531 bool DiscardLeftSide;
3532 if (op1->Pt.X >= Left && op1->Pt.X <= Right)
3534 Pt = op1->Pt; DiscardLeftSide = (op1->Pt.X > op1b->Pt.X);
3536 else if (op2->Pt.X >= Left&& op2->Pt.X <= Right)
3538 Pt = op2->Pt; DiscardLeftSide = (op2->Pt.X > op2b->Pt.X);
3540 else if (op1b->Pt.X >= Left && op1b->Pt.X <= Right)
3542 Pt = op1b->Pt; DiscardLeftSide = op1b->Pt.X > op1->Pt.X;
3546 Pt = op2b->Pt; DiscardLeftSide = (op2b->Pt.X > op2->Pt.X);
3548 j->OutPt1 = op1;
j->OutPt2 = op2;
3549 return JoinHorz(op1, op1b, op2, op2b, Pt, DiscardLeftSide);
3558 while ((op1b->Pt == op1->Pt) && (op1b != op1)) op1b = op1b->Next;
3559 bool Reverse1 = ((op1b->Pt.Y > op1->Pt.Y) ||
3564 while ((op1b->Pt == op1->Pt) && (op1b != op1)) op1b = op1b->Prev;
3565 if ((op1b->Pt.Y > op1->Pt.Y) ||
3569 while ((op2b->Pt == op2->Pt) && (op2b != op2))op2b = op2b->Next;
3570 bool Reverse2 = ((op2b->Pt.Y > op2->Pt.Y) ||
3575 while ((op2b->Pt == op2->Pt) && (op2b != op2)) op2b = op2b->Prev;
3576 if ((op2b->Pt.Y > op2->Pt.Y) ||
3580 if ((op1b == op1) || (op2b == op2) || (op1b == op2b) ||
3581 ((outRec1 == outRec2) && (Reverse1 == Reverse2)))
return false;
3612 while (FirstLeft && !FirstLeft->Pts)
3621 for (PolyOutList::size_type
i = 0;
i <
m_PolyOuts.size(); ++
i)
3625 if (outRec->Pts && firstLeft == OldOutRec)
3628 outRec->FirstLeft = NewOutRec;
3640 OutRec* orfl = OuterOutRec->FirstLeft;
3641 for (PolyOutList::size_type
i = 0;
i <
m_PolyOuts.size(); ++
i)
◆ PopEdgeFromSEL()
bool ClipperLib::Clipper::PopEdgeFromSEL |
( |
TEdge *& |
edge | ) |
|
|
private |
◆ ProcessEdgesAtTopOfScanbeam()
void ClipperLib::Clipper::ProcessEdgesAtTopOfScanbeam |
( |
const cInt |
topY | ) |
|
|
private |
Definition at line 3041 of file clipper.cpp.
3048 TEdge* ePrev = e->PrevInAEL;
3049 if ((e->OutIdx >= 0) && (e->WindDelta != 0) && ePrev && (ePrev->OutIdx >= 0) &&
3050 (ePrev->Curr.X == e->Curr.X) && (ePrev->WindDelta != 0))
3052 IntPoint pt = e->Curr;
3054 SetZ(pt, *ePrev, *e);
3078 if( e->OutIdx >= 0 )
3083 TEdge* ePrev = e->PrevInAEL;
3084 TEdge* eNext = e->NextInAEL;
3085 if (ePrev && ePrev->Curr.X == e->Bot.X &&
3086 ePrev->Curr.Y == e->Bot.Y && op &&
3087 ePrev->OutIdx >= 0 && ePrev->Curr.Y > ePrev->Top.Y &&
3089 (e->WindDelta != 0) && (ePrev->WindDelta != 0))
3091 OutPt* op2 =
AddOutPt(ePrev, e->Bot);
3094 else if (eNext && eNext->Curr.X == e->Bot.X &&
3095 eNext->Curr.Y == e->Bot.Y && op &&
3096 eNext->OutIdx >= 0 && eNext->Curr.Y > eNext->Top.Y &&
3098 (e->WindDelta != 0) && (eNext->WindDelta != 0))
3100 OutPt* op2 =
AddOutPt(eNext, e->Bot);
3111 OutPt *pp = outrec.Pts;
3112 OutPt *lastPP = pp->Prev;
3113 while (pp != lastPP)
3116 if (pp->Pt == pp->Prev->Pt)
3118 if (pp == lastPP) lastPP = pp->Prev;
3119 OutPt *tmpPP = pp->Prev;
3120 tmpPP->Next = pp->Next;
3121 pp->Next->Prev = tmpPP;
3141 outrec.BottomPt = 0;
3142 OutPt *pp = outrec.Pts;
◆ ProcessHorizontal()
void ClipperLib::Clipper::ProcessHorizontal |
( |
TEdge * |
horzEdge | ) |
|
|
private |
Definition at line 2672 of file clipper.cpp.
2676 bool IsLastHorz = (horzEdge == eLastHorz);
2688 while (maxIt !=
m_Maxima.end() && *maxIt < e->Curr.X)
2690 if (horzEdge->OutIdx >= 0 && !IsOpen)
2691 AddOutPt(horzEdge, IntPoint(*maxIt, horzEdge->Bot.Y));
2697 while (maxRit !=
m_Maxima.rend() && *maxRit > e->Curr.X)
2699 if (horzEdge->OutIdx >= 0 && !IsOpen)
2700 AddOutPt(horzEdge, IntPoint(*maxRit, horzEdge->Bot.Y));
2711 if (e->Curr.X == horzEdge->Top.X && horzEdge->NextInLML &&
2712 e->Dx < horzEdge->NextInLML->Dx)
break;
2714 if (horzEdge->OutIdx >= 0 && !IsOpen)
2720 if (eNextHorz->OutIdx >= 0 &&
2722 horzEdge->Top.X, eNextHorz->Bot.X, eNextHorz->Top.X))
2725 AddJoin(op2, op1, eNextHorz->Top);
2727 eNextHorz = eNextHorz->NextInSEL;
2734 if(e == eMaxPair && IsLastHorz)
2736 if (horzEdge->OutIdx >= 0)
2745 IntPoint Pt = IntPoint(e->Curr.X, horzEdge->Curr.Y);
2750 IntPoint Pt = IntPoint(e->Curr.X, horzEdge->Curr.Y);
2759 if (!horzEdge->NextInLML || !
IsHorizontal(*horzEdge->NextInLML))
break;
2762 if (horzEdge->OutIdx >= 0)
AddOutPt(horzEdge, horzEdge->Bot);
2767 if (horzEdge->OutIdx >= 0 && !op1)
2773 if (eNextHorz->OutIdx >= 0 &&
2775 horzEdge->Top.X, eNextHorz->Bot.X, eNextHorz->Top.X))
2778 AddJoin(op2, op1, eNextHorz->Top);
2780 eNextHorz = eNextHorz->NextInSEL;
2785 if (horzEdge->NextInLML)
2787 if(horzEdge->OutIdx >= 0)
2789 op1 =
AddOutPt( horzEdge, horzEdge->Top);
2791 if (horzEdge->WindDelta == 0)
return;
2793 TEdge* ePrev = horzEdge->PrevInAEL;
2794 TEdge* eNext = horzEdge->NextInAEL;
2795 if (ePrev && ePrev->Curr.X == horzEdge->Bot.X &&
2796 ePrev->Curr.Y == horzEdge->Bot.Y && ePrev->WindDelta != 0 &&
2797 (ePrev->OutIdx >= 0 && ePrev->Curr.Y > ePrev->Top.Y &&
2800 OutPt* op2 =
AddOutPt(ePrev, horzEdge->Bot);
2801 AddJoin(op1, op2, horzEdge->Top);
2803 else if (eNext && eNext->Curr.X == horzEdge->Bot.X &&
2804 eNext->Curr.Y == horzEdge->Bot.Y && eNext->WindDelta != 0 &&
2805 eNext->OutIdx >= 0 && eNext->Curr.Y > eNext->Top.Y &&
2808 OutPt* op2 =
AddOutPt(eNext, horzEdge->Bot);
2809 AddJoin(op1, op2, horzEdge->Top);
2817 if (horzEdge->OutIdx >= 0)
AddOutPt(horzEdge, horzEdge->Top);
2829 if (IlSize == 0)
return true;
2837 throw clipperException(
"ProcessIntersections error");
◆ ProcessHorizontals()
void ClipperLib::Clipper::ProcessHorizontals |
( |
| ) |
|
|
private |
Definition at line 2548 of file clipper.cpp.
2552 if (result && (result->OutIdx ==
Skip ||
2553 (result->NextInAEL == result->PrevInAEL && !
IsHorizontal(*result))))
return 0;
◆ ProcessIntersections()
bool ClipperLib::Clipper::ProcessIntersections |
( |
const cInt |
topY | ) |
|
|
private |
Definition at line 2859 of file clipper.cpp.
2861 e->PrevInSEL = e->PrevInAEL;
2862 e->NextInSEL = e->NextInAEL;
2863 e->Curr.X =
TopX( *e, topY );
2873 while( e->NextInSEL )
2877 if(e->Curr.X > eNext->Curr.X)
◆ ProcessIntersectList()
void ClipperLib::Clipper::ProcessIntersectList |
( |
| ) |
|
|
private |
◆ ReverseSolution() [1/2]
bool ClipperLib::Clipper::ReverseSolution |
( |
| ) |
|
|
inline |
◆ ReverseSolution() [2/2]
void ClipperLib::Clipper::ReverseSolution |
( |
bool |
value | ) |
|
|
inline |
◆ SetHoleState()
void ClipperLib::Clipper::SetHoleState |
( |
TEdge * |
e, |
|
|
OutRec * |
outrec |
|
) |
| |
|
private |
Definition at line 2337 of file clipper.cpp.
2351 outRec1 = outRec1->FirstLeft;
2352 if (outRec1 == outRec2)
return true;
◆ SetWindingCount()
void ClipperLib::Clipper::SetWindingCount |
( |
TEdge & |
edge | ) |
|
|
private |
Definition at line 1660 of file clipper.cpp.
1665 edge.WindCnt = edge.WindDelta;
1667 edge.WindCnt2 = e->WindCnt2;
1673 if (e->WindCnt * e->WindDelta < 0)
1677 if (
Abs(e->WindCnt) > 1)
1681 if (e->WindDelta * edge.WindDelta < 0) edge.WindCnt = e->WindCnt;
1683 else edge.WindCnt = e->WindCnt + edge.WindDelta;
1687 edge.WindCnt = (edge.WindDelta == 0 ? 1 : edge.WindDelta);
1692 if (edge.WindDelta == 0)
1693 edge.WindCnt = (e->WindCnt < 0 ? e->WindCnt - 1 : e->WindCnt + 1);
1695 else if (e->WindDelta * edge.WindDelta < 0) edge.WindCnt = e->WindCnt;
1697 else edge.WindCnt = e->WindCnt + edge.WindDelta;
1699 edge.WindCnt2 = e->WindCnt2;
1709 if (e->WindDelta != 0)
1710 edge.WindCnt2 = (edge.WindCnt2 == 0 ? 1 : 0);
1716 while ( e != &edge )
1718 edge.WindCnt2 += e->WindDelta;
1758 if (edge.WindDelta == 0 && edge.WindCnt != 1)
return false;
◆ StrictlySimple() [1/2]
bool ClipperLib::Clipper::StrictlySimple |
( |
| ) |
|
|
inline |
◆ StrictlySimple() [2/2]
void ClipperLib::Clipper::StrictlySimple |
( |
bool |
value | ) |
|
|
inline |
◆ SwapPositionsInSEL()
void ClipperLib::Clipper::SwapPositionsInSEL |
( |
TEdge * |
edge1, |
|
|
TEdge * |
edge2 |
|
) |
| |
|
private |
Definition at line 2594 of file clipper.cpp.
2606 return dir ==
dLeftToRight ? e->NextInAEL : e->PrevInAEL;
2612 if (HorzEdge.Bot.X < HorzEdge.Top.X)
2614 Left = HorzEdge.Bot.X;
2615 Right = HorzEdge.Top.X;
2619 Left = HorzEdge.Top.X;
2620 Right = HorzEdge.Bot.X;
◆ m_ClipFillType
◆ m_ClipType
ClipType ClipperLib::Clipper::m_ClipType |
|
private |
◆ m_ExecuteLocked
bool ClipperLib::Clipper::m_ExecuteLocked |
|
private |
◆ m_GhostJoins
JoinList ClipperLib::Clipper::m_GhostJoins |
|
private |
◆ m_IntersectList
◆ m_Joins
◆ m_Maxima
◆ m_ReverseOutput
bool ClipperLib::Clipper::m_ReverseOutput |
|
private |
◆ m_SortedEdges
TEdge* ClipperLib::Clipper::m_SortedEdges |
|
private |
◆ m_StrictSimple
bool ClipperLib::Clipper::m_StrictSimple |
|
private |
◆ m_SubjFillType
◆ m_UsingPolyTree
bool ClipperLib::Clipper::m_UsingPolyTree |
|
private |
The documentation for this class was generated from the following files:
std::vector< IntPoint > Path
void AddLocalMaxPoly(TEdge *e1, TEdge *e2, const IntPoint &pt)
bool EdgesAdjacent(const IntersectNode &inode)
std::vector< Path > Paths
bool SlopesEqual(const TEdge &e1, const TEdge &e2, bool UseFullInt64Range)
void IntersectEdges(TEdge *e1, TEdge *e2, IntPoint &pt)
void InsertEdgeIntoAEL(TEdge *edge, TEdge *startEdge)
void DisposeOutPts(OutPt *&pp)
void DisposeIntersectNodes()
PolyFillType m_ClipFillType
OutRec * GetLowermostRec(OutRec *outRec1, OutRec *outRec2)
cInt TopX(TEdge &edge, const cInt currentY)
void FixupFirstLefts2(OutRec *InnerOutRec, OutRec *OuterOutRec)
bool E2InsertsBeforeE1(TEdge &e1, TEdge &e2)
void SetWindingCount(TEdge &edge)
void AddEdgeToSEL(TEdge *edge)
TEdge * GetMaximaPair(TEdge *e)
int PointCount(OutPt *Pts)
static OutRec * ParseFirstLeft(OutRec *FirstLeft)
void SwapPositionsInSEL(TEdge *edge1, TEdge *edge2)
TEdge * GetMaximaPairEx(TEdge *e)
void ProcessHorizontal(TEdge *horzEdge)
void BuildIntersectList(const cInt topY)
double Area(const Path &poly)
bool GetOverlap(const cInt a1, const cInt a2, const cInt b1, const cInt b2, cInt &Left, cInt &Right)
void InsertScanbeam(const cInt Y)
bool ProcessIntersections(const cInt topY)
OutPt * GetBottomPt(OutPt *pp)
OutPt * AddOutPt(TEdge *e, const IntPoint &pt)
bool JoinHorz(OutPt *op1, OutPt *op1b, OutPt *op2, OutPt *op2b, const IntPoint Pt, bool DiscardLeft)
bool IntersectListSort(IntersectNode *node1, IntersectNode *node2)
void DeleteFromAEL(TEdge *e)
const double c
speed of light (cm/ns)
void UpdateEdgeIntoAEL(TEdge *&e)
void UpdateOutPtIdxs(OutRec &outrec)
void ReversePolyPtLinks(OutPt *pp)
Clipper(int initOptions=0)
void FixupFirstLefts1(OutRec *OldOutRec, OutRec *NewOutRec)
void AddGhostJoin(OutPt *op, const IntPoint offPt)
bool HorzSegmentsOverlap(cInt seg1a, cInt seg1b, cInt seg2a, cInt seg2b)
void FixupOutPolyline(OutRec &outrec)
TEdge * GetNextInAEL(TEdge *e, Direction dir)
bool IsContributing(const TEdge &edge) const
OutPt * DupOutPt(OutPt *outPt, bool InsertAfter)
void SimplifyPolygons(const Paths &in_polys, Paths &out_polys, PolyFillType fillType=pftEvenOdd)
static const int Unassigned
void ReversePath(Path &p)
OutPt * GetLastOutPt(TEdge *e)
void DeleteFromSEL(TEdge *e)
void FixupFirstLefts3(OutRec *OldOutRec, OutRec *NewOutRec)
FTensor::Index< 'i', SPACE_DIM > i
PolyFillType m_SubjFillType
bool FixupIntersectionOrder()
OutPt * AddLocalMinPoly(TEdge *e1, TEdge *e2, const IntPoint &pt)
bool PopLocalMinima(cInt Y, const LocalMinimum *&locMin)
bool IsHorizontal(TEdge &e)
IntersectList m_IntersectList
void GetHorzDirection(TEdge &HorzEdge, Direction &Dir, cInt &Left, cInt &Right)
void FixupOutPolygon(OutRec &outrec)
FTensor::Index< 'j', 3 > j
ClipperOffset(double miterLimit=2.0, double roundPrecision=0.25)
bool IsMaxima(TEdge *e, const cInt Y)
bool IsEvenOddAltFillType(const TEdge &edge) const
virtual bool ExecuteInternal()
bool PopEdgeFromSEL(TEdge *&edge)
bool IsIntermediate(TEdge *e, const cInt Y)
OutRec * GetOutRec(int idx)
bool Poly2ContainsPoly1(OutPt *OutPt1, OutPt *OutPt2)
bool OutRec1RightOfOutRec2(OutRec *outRec1, OutRec *outRec2)
void SwapPositionsInAEL(TEdge *edge1, TEdge *edge2)
DoublePoint GetUnitNormal(const IntPoint &pt1, const IntPoint &pt2)
bool IsEvenOddFillType(const TEdge &edge) const
bool JoinPoints(Join *j, OutRec *outRec1, OutRec *outRec2)
void ProcessHorizontals()
void SwapPolyIndexes(TEdge &Edge1, TEdge &Edge2)
void ReversePaths(Paths &p)
void SetHoleState(TEdge *e, OutRec *outrec)
void AppendPolygon(TEdge *e1, TEdge *e2)
void SwapSides(TEdge &Edge1, TEdge &Edge2)
bool Pt2IsBetweenPt1AndPt3(const IntPoint pt1, const IntPoint pt2, const IntPoint pt3)
void ProcessEdgesAtTopOfScanbeam(const cInt topY)
void ProcessIntersectList()
void BuildResult(Paths &polys)
void AddJoin(OutPt *op1, OutPt *op2, const IntPoint offPt)
void SimplifyPolygon(const Path &in_poly, Paths &out_polys, PolyFillType fillType=pftEvenOdd)