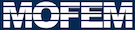 |
| v0.14.0
|
Go to the documentation of this file.
11 #define BOOST_MATH_GAUSS_NO_COMPUTE_ON_DEMAND
13 using namespace MoFEM;
15 static char help[] =
"...\n\n";
32 PETSC>::LinearForm<GAUSS>::OpBaseTimesScalar<1>;
36 PETSC>::LinearForm<GAUSS>::OpSource<1, 1>;
38 const double n = 1.44;
40 const double v =
c /
n;
58 #include <boost/math/quadrature/gauss_kronrod.hpp>
59 using namespace boost::math::quadrature;
62 OpError(boost::shared_ptr<VectorDouble> u_at_pts_ptr,
double &l2_error)
63 :
DomainEleOp(
"PHOTON_FLUENCE_RATE", OPROW), uAtPtsPtr(u_at_pts_ptr),
66 std::fill(&doEntities[MBEDGE], &doEntities[MBMAXTYPE],
false);
94 static double sourceFunction(
const double x,
const double y,
const double z) {
99 auto phi_pulse = [&](
const double r_s,
const double phi_s) {
100 const double xs = r_s * cos(phi_s);
101 const double ys = r_s * sin(phi_s);
106 const double P1 = xp * xp + yp * yp + zp1 * zp1;
107 const double P2 = xp * xp + yp * yp + zp2 * zp2;
108 return r_s * (exp(-P1 /
A) - exp(-P2 /
A));
111 auto f = [&](
const double r_s) {
112 auto g = [&](
const double phi_s) {
return phi_pulse(r_s, phi_s); };
113 return gauss_kronrod<double, 15>::integrate(
114 g, 0, 2 * M_PI, 0, std::numeric_limits<float>::epsilon());
118 gauss_kronrod<double, 15>::integrate(
119 f, 0,
beam_radius, 0, std::numeric_limits<float>::epsilon());
182 MOFEM_LOG(
"INITIAL", Sev::inform) <<
"Refractive index: " <<
n;
183 MOFEM_LOG(
"INITIAL", Sev::inform) <<
"Speed of light (cm/ns): " <<
c;
185 <<
"Phase velocity in medium (cm/ns): " <<
v;
187 <<
"Absorption coefficient (cm^-1): " <<
mu_a;
189 <<
"Scattering coefficient (cm^-1): " <<
mu_sp;
190 MOFEM_LOG(
"INITIAL", Sev::inform) <<
"Diffusion coefficient D : " <<
D;
198 MOFEM_LOG(
"INITIAL", Sev::inform) <<
"Approximation order: " <<
order;
265 std::string entity_name = it->getName();
266 if (entity_name.compare(0, 3,
"INT") == 0) {
268 boundary_ents,
true);
272 Range boundary_verts;
275 boundary_ents.merge(boundary_verts);
279 simple->getProblemName(),
"PHOTON_FLUENCE_RATE", boundary_ents);
290 auto add_domain_base_ops = [&](
auto &pipeline) {
291 auto jac_ptr = boost::make_shared<MatrixDouble>();
292 auto det_ptr = boost::make_shared<VectorDouble>();
298 auto add_domain_lhs_ops = [&](
auto &pipeline) {
300 "PHOTON_FLUENCE_RATE",
"PHOTON_FLUENCE_RATE",
301 [](
const double,
const double,
const double) {
return 1; }));
304 auto add_domain_rhs_ops = [&](
auto &pipeline) {
310 add_domain_base_ops(pipeline_mng->getOpDomainLhsPipeline());
311 add_domain_base_ops(pipeline_mng->getOpDomainRhsPipeline());
312 add_domain_lhs_ops(pipeline_mng->getOpDomainLhsPipeline());
313 add_domain_rhs_ops(pipeline_mng->getOpDomainRhsPipeline());
322 auto solver = pipeline_mng->createKSP();
324 CHKERR KSPSetFromOptions(solver);
327 auto dm =
simple->getDM();
331 MOFEM_LOG(
"INITIAL", Sev::inform) <<
"Solver start";
333 CHKERR VecGhostUpdateBegin(X, INSERT_VALUES, SCATTER_FORWARD);
334 CHKERR VecGhostUpdateEnd(X, INSERT_VALUES, SCATTER_FORWARD);
338 <<
"writing vector in binary to " <<
out_file_name <<
" ...";
340 PetscViewerBinaryOpen(PETSC_COMM_WORLD,
out_file_name, FILE_MODE_WRITE,
343 PetscViewerDestroy(&viewer);
345 MOFEM_LOG(
"INITIAL", Sev::inform) <<
"Solver done";
353 auto post_proc_fe = boost::make_shared<PostProcEle>(
mField);
355 auto u_ptr = boost::make_shared<VectorDouble>();
356 post_proc_fe->getOpPtrVector().push_back(
361 post_proc_fe->getOpPtrVector().push_back(
365 post_proc_fe->getPostProcMesh(), post_proc_fe->getMapGaussPts(),
367 {{
"PHOTON_FLUENCE_RATE", u_ptr}},
377 pipeline_mng->getDomainRhsFE() = post_proc_fe;
378 CHKERR pipeline_mng->loopFiniteElements();
379 CHKERR post_proc_fe->writeFile(
"out_initial.h5m");
399 int main(
int argc,
char *argv[]) {
402 const char param_file[] =
"param_file.petsc";
406 auto core_log = logging::core::get();
408 LogManager::createSink(LogManager::getStrmWorld(),
"INITIAL"));
409 LogManager::setLog(
"INITIAL");
415 DMType dm_name =
"DMMOFEM";
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface refernce to pointer of interface.
double mu_sp
scattering coefficient (cm^-1)
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpGradTimesTensor< 1, 1, SPACE_DIM > OpDomainGradTimesVec
boost::shared_ptr< VectorDouble > uAtPtsPtr
Problem manager is used to build and partition problems.
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::BiLinearForm< GAUSS >::OpMass< 1, 1 > OpDomainMass
PipelineManager interface.
MoFEMErrorCode setIntegrationRules()
PetscErrorCode DMoFEMMeshToLocalVector(DM dm, Vec l, InsertMode mode, ScatterMode scatter_mode)
set local (or ghosted) vector values on mesh for partition only
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
Simple interface for fast problem set-up.
double flux_magnitude
impulse magnitude
PhotonDiffusion(MoFEM::Interface &m_field)
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::BiLinearForm< GAUSS >::OpMass< 1, FIELD_DIM > OpDomainMass
MoFEMErrorCode readMesh()
Deprecated interface functions.
DeprecatedCoreInterface Interface
static constexpr int approx_order
MoFEMErrorCode setupProblem()
const double c
speed of light (cm/ns)
#define CHKERR
Inline error check.
auto createDMVector(DM dm)
Get smart vector from DM.
virtual moab::Interface & get_moab()=0
implementation of Data Operators for Forces and Sources
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpBaseTimesScalar< 1 > OpDomainTimesScalarField
Simple interface for fast problem set-up.
MoFEM::Interface & mField
MoFEMErrorCode initialCondition()
void simple(double P1[], double P2[], double P3[], double c[], const int N)
double mu_a
absorption coefficient (cm^-1)
BcMapByBlockName & getBcMapByBlockName()
Get the bc map.
OpPostProcMapInMoab< SPACE_DIM, SPACE_DIM > OpPPMap
Get value at integration points for scalar field.
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
MoFEMErrorCode runProgram()
const double n
refractive index of diffusive medium
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpSource< 1, 1 > OpDomainSource
#define MOFEM_LOG_TAG(channel, tag)
Tag channel.
Volume finite element base.
MoFEMErrorCode solveSystem()
OpError(boost::shared_ptr< VectorDouble > u_at_pts_ptr, double &l2_error)
FormsIntegrators< DomainEleOp >::Assembly< A >::LinearForm< I >::OpGradTimesTensor< 1, FIELD_DIM, SPACE_DIM > OpGradTimesTensor
MoFEMErrorCode boundaryCondition()
const double v
phase velocity of light in medium (cm/ns)
boost::shared_ptr< FEMethod > & getDomainLhsFE()
MoFEMErrorCode assembleSystem()
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define MOFEM_LOG(channel, severity)
Log.
SmartPetscObj< Vec > vectorDuplicate(Vec vec)
Create duplicate vector of smart vector.
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::BiLinearForm< GAUSS >::OpGradGrad< 1, 1, SPACE_DIM > OpDomainGradGrad
#define CATCH_ERRORS
Catch errors.
#define _IT_CUBITMESHSETS_BY_SET_TYPE_FOR_LOOP_(MESHSET_MANAGER, CUBITBCTYPE, IT)
Iterator that loops over a specific Cubit MeshSet having a particular BC meshset in a moFEM field.
ForcesAndSourcesCore::UserDataOperator UserDataOperator
Set inverse jacobian to base functions.
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
PetscErrorCode PetscOptionsGetString(PetscOptions *, const char pre[], const char name[], char str[], size_t size, PetscBool *set)
PetscErrorCode PetscOptionsGetScalar(PetscOptions *, const char pre[], const char name[], PetscScalar *dval, PetscBool *set)
ElementsAndOps< SPACE_DIM >::DomainEle DomainEle
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpSource< 1, FIELD_DIM > OpDomainSource
static double sourceFunction(const double x, const double y, const double z)
Pulse is infinitely short.
PetscErrorCode PetscOptionsGetInt(PetscOptions *, const char pre[], const char name[], PetscInt *ivalue, PetscBool *set)
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
constexpr int SPACE_DIM
[Define dimension]
int main(int argc, char *argv[])
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
OpCalculateHOJacForVolume OpCalculateHOJacVolume
Post post-proc data at points from hash maps.
PetscErrorCode PetscOptionsGetBool(PetscOptions *, const char pre[], const char name[], PetscBool *bval, PetscBool *set)
MoFEMErrorCode outputResults()