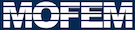 |
| v0.14.0
|
Go to the documentation of this file.
9 #ifndef __PROBLEMSMANAGER_HPP__
10 #define __PROBLEMSMANAGER_HPP__
54 const int adj_dim,
const int n_parts,
55 Tag *th_vertex_weights =
nullptr,
56 Tag *th_edge_weights =
nullptr,
57 Tag *th_part_weights =
nullptr,
59 const bool debug =
false);
109 const bool square_matrix,
124 const bool square_matrix =
true,
138 const std::string out_name,
const std::vector<std::string> &fields_row,
139 const std::vector<std::string> &fields_col,
140 const std::string main_problem,
const bool square_matrix =
true,
141 const map<std::string, boost::shared_ptr<Range>> *entityMapRow =
nullptr,
142 const map<std::string, boost::shared_ptr<Range>> *entityMapCol =
nullptr,
158 const std::vector<std::string> add_row_problems,
159 const std::vector<std::string> add_col_problems,
160 const bool square_matrix =
true,
int verb = 1);
178 const std::string problem_for_rows,
180 const std::string problem_for_cols,
181 bool copy_cols,
int verb =
VERBOSE);
221 bool part_from_moab =
false,
222 int low_proc = -1,
int hi_proc = -1,
268 const std::string &fe_name,
286 const std::string &fe_name,
287 PetscLayout *layout)
const;
313 const std::string problem_name,
const std::string
field_name,
314 const Range ents,
const int lo_coeff = 0,
316 const int hi_order = 100,
int verb =
VERBOSE,
const bool debug =
false);
324 const std::string problem_name,
const std::string
field_name,
325 const Range ents,
const int lo_coeff = 0,
327 const int hi_order = 100,
int verb =
VERBOSE,
const bool debug =
false);
347 const std::string problem_name,
const std::string
field_name,
349 Range *ents_ptr =
nullptr,
const int lo_coeff = 0,
351 const int hi_order = 100,
int verb =
VERBOSE,
const bool debug =
false);
359 const std::string problem_name,
const std::string
field_name,
361 Range *ents_ptr =
nullptr,
const int lo_coeff = 0,
363 const int hi_order = 100,
int verb =
VERBOSE,
const bool debug =
false);
382 std::vector<boost::weak_ptr<NumeredDofEntity>> &vec_dof_view,
405 std::vector<boost::weak_ptr<NumeredDofEntity>> &vec_dof_view,
407 const std::string problem_name,
RowColData rc,
410 const int lo_order = 0,
const int hi_order = 100,
int verb =
VERBOSE,
411 const bool debug =
false
432 std::vector<unsigned char> &
marker)
const;
439 std::vector<unsigned char> &
marker)
const {
457 const int hi,
const enum MarkOP op,
458 const unsigned char c,
459 std::vector<unsigned char> &
marker)
const;
475 std::vector<boost::weak_ptr<NumeredDofEntity>> &vec_dof_view,
476 const enum MarkOP op, std::vector<unsigned char> &
marker)
const;
491 const std::string row_field,
492 const std::string col_field)
const;
499 #endif //__PROBLEMSMANAGER_HPP__
DEPRECATED MoFEMErrorCode markDofs(const std::string problem_name, RowColData rc, const Range ents, std::vector< unsigned char > &marker) const
PetscBool buildProblemFromFields
MoFEMErrorCode buildProblem(const std::string name, const bool square_matrix, int verb=VERBOSE)
build problem data structures
Problem manager is used to build and partition problems.
DEPRECATED MoFEMErrorCode partitionMesh(const Range &ents, const int dim, const int adj_dim, const int n_parts, Tag *th_vertex_weights=nullptr, Tag *th_edge_weights=nullptr, Tag *th_part_weights=nullptr, int verb=VERBOSE, const bool debug=false)
Set partition tag to each finite element in the problem.
MoFEMErrorCode inheritPartition(const std::string name, const std::string problem_for_rows, bool copy_rows, const std::string problem_for_cols, bool copy_cols, int verb=VERBOSE)
build indexing and partition problem inheriting indexing and partitioning from two other problems
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
MoFEMErrorCode printPartitionedProblem(const Problem *problem_ptr, int verb=VERBOSE)
MoFEMErrorCode debugPartitionedProblem(const Problem *problem_ptr, int verb=VERBOSE)
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const
const double c
speed of light (cm/ns)
virtual ~ProblemsManager()=default
MoFEMErrorCode removeDofsOnEntitiesNotDistributed(const std::string problem_name, const std::string field_name, const Range ents, const int lo_coeff=0, const int hi_coeff=MAX_DOFS_ON_ENTITY, const int lo_order=0, const int hi_order=100, int verb=VERBOSE, const bool debug=false)
Remove DOFs from problem.
implementation of Data Operators for Forces and Sources
MoFEMErrorCode partitionGhostDofsOnDistributedMesh(const std::string name, int verb=VERBOSE)
determine ghost nodes on distributed meshes
MoFEMErrorCode removeDofsOnEntities(const std::string problem_name, const std::string field_name, const Range ents, const int lo_coeff=0, const int hi_coeff=MAX_DOFS_ON_ENTITY, const int lo_order=0, const int hi_order=100, int verb=VERBOSE, const bool debug=false)
Remove DOFs from problem.
MoFEMErrorCode addFieldToEmptyFieldBlocks(const std::string problem_name, const std::string row_field, const std::string col_field) const
add empty block to problem
MoFEMErrorCode getOptions()
MoFEMErrorCode markDofs(const std::string problem_name, RowColData rc, const enum MarkOP op, const Range ents, std::vector< unsigned char > &marker) const
Create vector with marked indices.
MoFEMErrorCode getProblemElementsLayout(const std::string name, const std::string &fe_name, PetscLayout *layout) const
Get layout of elements in the problem.
MoFEMErrorCode partitionFiniteElements(const std::string name, bool part_from_moab=false, int low_proc=-1, int hi_proc=-1, int verb=VERBOSE)
partition finite elements
MoFEMErrorCode buildComposedProblem(const std::string out_name, const std::vector< std::string > add_row_problems, const std::vector< std::string > add_col_problems, const bool square_matrix=true, int verb=1)
build composite problem
constexpr auto field_name
MoFEMErrorCode removeDofs(const std::string problem_name, RowColData rc, std::vector< boost::weak_ptr< NumeredDofEntity >> &vec_dof_view, int verb=VERBOSE, const bool debug=false)
Remove DOFs from problem on broken space.
base class for all interface classes
#define MAX_DOFS_ON_ENTITY
Maximal number of DOFs on entity.
PetscLogEvent MOFEM_EVENT_ProblemsManager
MoFEMErrorCode partitionSimpleProblem(const std::string name, int verb=VERBOSE)
partition problem dofs
PetscBool synchroniseProblemEntities
DOFs in fields, not from DOFs on elements.
MoFEMErrorCode buildProblemOnDistributedMesh(const std::string name, const bool square_matrix, int verb=VERBOSE)
build problem data structures, assuming that mesh is distributed (collective)
ProblemsManager(const MoFEM::Core &core)
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
MoFEMErrorCode getFEMeshset(const std::string prb_name, const std::string &fe_name, EntityHandle *meshset) const
create add entities of finite element in the problem
auto marker
set bit to marker
keeps basic data about problem
MoFEMErrorCode partitionGhostDofs(const std::string name, int verb=VERBOSE)
determine ghost nodes
MoFEMErrorCode modifyMarkDofs(const std::string problem_name, RowColData rc, const std::string field_name, const int lo, const int hi, const enum MarkOP op, const unsigned char c, std::vector< unsigned char > &marker) const
Mark DOFs.
MoFEMErrorCode getSideDofsOnBrokenSpaceEntities(std::vector< boost::weak_ptr< NumeredDofEntity >> &vec_dof_view, const std::string problem_name, RowColData rc, const std::string field_name, const Range ents, int bridge_dim, const int lo_coeff=0, const int hi_coeff=MAX_DOFS_ON_ENTITY, const int lo_order=0, const int hi_order=100, int verb=VERBOSE, const bool debug=false) const
Get DOFs on side entities for broke space.
MoFEMErrorCode partitionProblem(const std::string name, int verb=VERBOSE)
partition problem dofs (collective)
MoFEMErrorCode buildSubProblem(const std::string out_name, const std::vector< std::string > &fields_row, const std::vector< std::string > &fields_col, const std::string main_problem, const bool square_matrix=true, const map< std::string, boost::shared_ptr< Range >> *entityMapRow=nullptr, const map< std::string, boost::shared_ptr< Range >> *entityMapCol=nullptr, int verb=VERBOSE)
build sub problem