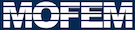 |
| v0.14.0
|
Go to the documentation of this file.
38 static constexpr
const int value =
N;
50 MPI_Comm comm = PETSC_COMM_WORLD,
90 virtual boost::shared_ptr<RefEntityTmp<0>>
97 MPI_Comm comm = PETSC_COMM_WORLD,
171 boost::shared_ptr<BasicEntityData> &ptr);
173 static boost::shared_ptr<RefEntityTmp<0>>
188 MoFEMErrorCode query_interface(boost::typeindex::type_index type_index,
212 BUILD_FIELD = 1 << 0,
215 BUILD_PROBLEM = 1 << 3,
216 PARTITION_PROBLEM = 1 << 4,
217 PARTITION_FE = 1 << 5,
218 PARTITION_GHOST_DOFS = 1 << 6,
219 PARTITION_MESH = 1 << 7
291 return basicEntityDataPtr;
321 std::reference_wrapper<moab::Interface>
moab;
338 check_number_of_ents_in_ents_field(
const std::string &name)
const;
341 check_number_of_ents_in_ents_finite_element(
const std::string &name)
const;
342 MoFEMErrorCode check_number_of_ents_in_ents_finite_element()
const;
362 return *get_meshsets_manager_ptr();
365 return *get_meshsets_manager_ptr();
391 const bool remove_parent =
false,
425 add_field(
const std::string &name,
const FieldSpace space,
428 const TagType tag_type = MB_TAG_SPARSE,
457 const TagType tag_type,
const enum MoFEMTypes bh,
461 const std::string &name,
464 const std::string &name,
467 const EntityType
type,
468 const std::string &name,
472 const std::string &name,
473 const bool recursive =
true,
476 const EntityType
type,
477 const std::string &name,
478 const bool recursive =
true,
481 MoFEMErrorCode create_vertices_and_add_to_field(
const std::string name,
482 const double coords[],
491 MoFEMErrorCode setFieldOrderImpl(boost::shared_ptr<Field> field_ptr,
507 const EntityType
type,
const std::string &name,
522 buildFieldForNoFieldImpl(boost::shared_ptr<Field> field_ptr,
523 std::map<EntityType, int> &dof_counter,
int verb);
526 std::map<EntityType, int> &dof_counter,
530 buildFieldForL2H1HcurlHdiv(
const BitFieldId id,
531 std::map<EntityType, int> &dof_counter,
532 std::map<EntityType, int> &inactive_dof_counter,
570 const EntityType
type,
580 MoFEMErrorCode list_dofs_by_field_name(
const std::string &name)
const;
582 BitFieldId get_field_id(
const std::string &name)
const;
583 FieldBitNumber get_field_bit_number(
const std::string name)
const;
584 std::string get_field_name(
const BitFieldId id)
const;
586 EntityHandle get_field_meshset(
const std::string name)
const;
587 MoFEMErrorCode get_field_entities_by_dimension(
const std::string name,
588 int dim,
Range &ents)
const;
591 MoFEMErrorCode get_field_entities_by_handle(
const std::string name,
593 bool check_field(
const std::string &name)
const;
595 const Field *get_field_structure(
const std::string &name,
605 get_finite_element_structure(
const std::string &name,
608 bool check_finite_element(
const std::string &name)
const;
614 modify_finite_element_adjacency_table(
const std::string &fe_name,
615 const EntityType
type,
618 modify_finite_element_add_field_data(
const std::string &fe_name,
619 const std::string name_filed);
621 modify_finite_element_add_field_row(
const std::string &fe_name,
622 const std::string name_row);
624 modify_finite_element_add_field_col(
const std::string &fe_name,
625 const std::string name_col);
627 modify_finite_element_off_field_data(
const std::string &fe_name,
628 const std::string name_filed);
630 modify_finite_element_off_field_row(
const std::string &fe_name,
631 const std::string name_row);
633 modify_finite_element_off_field_col(
const std::string &fe_name,
634 const std::string name_col);
637 const std::string &name,
const bool recursive =
true);
640 const std::string &name,
641 const bool recursive =
true);
643 const EntityType
type,
644 const std::string &name);
647 const std::string &name);
652 add_ents_to_finite_element_by_MESHSET(
const EntityHandle meshset,
653 const std::string &name,
654 const bool recursive =
false);
666 MoFEMErrorCode remove_ents_from_finite_element(
const std::string name,
668 const EntityType
type,
670 MoFEMErrorCode remove_ents_from_finite_element(
const std::string name,
685 BitFEId getBitFEId(
const std::string &fe_name)
const;
692 std::string getBitFEIdName(
const BitFEId id)
const;
695 EntityHandle get_finite_element_meshset(
const std::string name)
const;
697 get_finite_element_entities_by_dimension(
const std::string name,
int dim,
699 MoFEMErrorCode get_finite_element_entities_by_type(
const std::string name,
702 MoFEMErrorCode get_finite_element_entities_by_handle(
const std::string name,
715 bool check_problem(
const std::string name);
718 modify_problem_add_finite_element(
const std::string name_problem,
719 const std::string &fe_name);
721 modify_problem_unset_finite_element(
const std::string name_problem,
722 const std::string &fe_name);
724 modify_problem_ref_level_add_bit(
const std::string &name_problem,
727 modify_problem_ref_level_set_bit(
const std::string &name_problem,
730 modify_problem_mask_ref_level_add_bit(
const std::string &name_problem,
733 modify_problem_mask_ref_level_set_bit(
const std::string &name_problem,
735 BitProblemId getBitProblemId(
const std::string &name)
const;
744 const Range *
const ents_ptr =
nullptr,
746 MoFEMErrorCode buildFiniteElements(
const boost::shared_ptr<FiniteElement> &fe,
747 const Range *ents_ptr = NULL,
759 get_problem_finite_elements_entities(
const std::string name,
760 const std::string &fe_name,
795 clear_adjacencies_finite_elements(
const Range ents,
798 clear_adjacencies_finite_elements(
const std::string name,
const Range ents,
811 problem_basic_method_preProcess(
const std::string &problem_name,
818 problem_basic_method_postProcess(
const std::string &problem_name,
829 const Problem *problem_ptr,
const std::string &fe_name,
FEMethod &method,
830 int lower_rank,
int upper_rank,
831 boost::shared_ptr<NumeredEntFiniteElement_multiIndex> fe_ptr =
nullptr,
837 const std::string problem_name,
const std::string &fe_name,
838 FEMethod &method,
int lower_rank,
int upper_rank,
839 boost::shared_ptr<NumeredEntFiniteElement_multiIndex> fe_ptr =
nullptr,
845 const std::string problem_name,
const std::string &fe_name,
847 boost::shared_ptr<NumeredEntFiniteElement_multiIndex> fe_ptr =
nullptr,
854 DofMethod &method,
int lower_rank,
int upper_rank,
858 DofMethod &method,
int lower_rank,
int upper_rank,
879 Range const *
const ents =
nullptr,
901 const Problem **problem_ptr)
const;
905 *dofs_elements_adjacency)
const;
914 const Problem *get_problem(
const std::string problem_name)
const;
917 get_ents_elements_adjacency()
const;
919 FieldEntityByUId::iterator
920 get_ent_field_by_name_begin(
const std::string &
field_name)
const;
921 FieldEntityByUId::iterator
922 get_ent_field_by_name_end(
const std::string &
field_name)
const;
923 DofEntityByUId::iterator
924 get_dofs_by_name_begin(
const std::string &
field_name)
const;
925 DofEntityByUId::iterator
926 get_dofs_by_name_end(
const std::string &
field_name)
const;
927 DofEntityByUId::iterator
928 get_dofs_by_name_and_ent_begin(
const std::string &
field_name,
930 DofEntityByUId::iterator
931 get_dofs_by_name_and_ent_end(
const std::string &
field_name,
933 DofEntityByUId::iterator
934 get_dofs_by_name_and_type_begin(
const std::string &
field_name,
935 const EntityType
type)
const;
936 DofEntityByUId::iterator
937 get_dofs_by_name_and_type_end(
const std::string &
field_name,
938 const EntityType ent)
const;
940 EntFiniteElement_multiIndex::index<Unique_mi_tag>::type::iterator
941 get_fe_by_name_begin(
const std::string &fe_name)
const;
942 EntFiniteElement_multiIndex::index<Unique_mi_tag>::type::iterator
943 get_fe_by_name_end(
const std::string &fe_name)
const;
975 inline MPI_Comm &
get_comm()
const {
return mofemComm; }
990 boost::shared_ptr<WrapMPIComm>
998 mutable boost::ptr_map<boost::typeindex::type_index, UnknownInterface>
iFaces;
1078 virtual boost::shared_ptr<RefEntityTmp<0>>
1085 MPI_Comm comm = PETSC_COMM_WORLD,
1100 #endif // __CORE_HPP__
multi_index_container< FieldEntityEntFiniteElementAdjacencyMap, indexed_by< ordered_unique< tag< Composite_Unique_mi_tag >, composite_key< FieldEntityEntFiniteElementAdjacencyMap, const_mem_fun< FieldEntityEntFiniteElementAdjacencyMap, UId, &FieldEntityEntFiniteElementAdjacencyMap::getEntUniqueId >, const_mem_fun< FieldEntityEntFiniteElementAdjacencyMap, UId, &FieldEntityEntFiniteElementAdjacencyMap::getFeUniqueId > > >, ordered_non_unique< tag< Unique_mi_tag >, const_mem_fun< FieldEntityEntFiniteElementAdjacencyMap, UId, &FieldEntityEntFiniteElementAdjacencyMap::getEntUniqueId > >, ordered_non_unique< tag< FE_Unique_mi_tag >, const_mem_fun< FieldEntityEntFiniteElementAdjacencyMap, UId, &FieldEntityEntFiniteElementAdjacencyMap::getFeUniqueId > >, ordered_non_unique< tag< FEEnt_mi_tag >, const_mem_fun< FieldEntityEntFiniteElementAdjacencyMap, EntityHandle, &FieldEntityEntFiniteElementAdjacencyMap::getFeHandle > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< FieldEntityEntFiniteElementAdjacencyMap, EntityHandle, &FieldEntityEntFiniteElementAdjacencyMap::getEntHandle > > > > FieldEntityEntFiniteElementAdjacencyMap_multiIndex
MultiIndex container keeps Adjacencies Element and dof entities adjacencies and vice versa.
PetscLogEvent MOFEM_EVENT_operator
Event for evaluating operator of finite element.
const int getValue() const
Data structure to exchange data between mofem and User Loop Methods on entities.
structure for User Loop Methods on finite elements
int rAnk
MOFEM communicator rank.
Tag get_th_RefBitEdge() const
boost::shared_ptr< WrapMPIComm > wrapMPIMOABComm
manage creation and destruction of MOAB communicator
multi_index_container< boost::shared_ptr< RefElement >, indexed_by< ordered_unique< tag< Ent_mi_tag >, const_mem_fun< RefElement::interface_type_RefEntity, EntityHandle, &RefElement::getEnt > > > > RefElement_multiIndex
std::bitset< BITPROBLEMID_SIZE > BitProblemId
Problem Id.
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
EntFiniteElement_multiIndex entsFiniteElements
finite element entities
int * buildMoFEM
keeps flags/semaphores for different stages
Struct keeps handle to refined handle.
std::bitset< BITFIELDID_SIZE > BitFieldId
Field Id.
RefEntity_multiIndex refinedEntities
refined entities
Tag th_ProblemLocalNbDofRow
virtual boost::shared_ptr< RefEntityTmp< 0 > > make_shared_ref_entity(const EntityHandle ent)
FieldEntityEntFiniteElementAdjacencyMap_multiIndex entFEAdjacencies
adjacencies of elements to dofs
Data structure to exchange data between mofem and User Loop Methods on entities.
multi_index_container< boost::shared_ptr< RefEntity >, indexed_by< ordered_unique< tag< Ent_mi_tag >, const_mem_fun< RefEntity, EntityHandle, &RefEntity::getEnt > >, ordered_non_unique< tag< Ent_Ent_mi_tag >, const_mem_fun< RefEntity, EntityHandle, &RefEntity::getParentEnt > >, ordered_non_unique< tag< Composite_EntType_and_ParentEntType_mi_tag >, composite_key< RefEntity, const_mem_fun< RefEntity, EntityType, &RefEntity::getEntType >, const_mem_fun< RefEntity, EntityType, &RefEntity::getParentEntType > > >, ordered_non_unique< tag< Composite_ParentEnt_And_EntType_mi_tag >, composite_key< RefEntity, const_mem_fun< RefEntity, EntityType, &RefEntity::getEntType >, const_mem_fun< RefEntity, EntityHandle, &RefEntity::getParentEnt > > > > > RefEntity_multiIndex
std::bitset< BITFEID_SIZE > BitFEId
Finite element Id.
Provide data structure for (tensor) field approximation.
Deprecated interface functions.
moab::Interface & get_moab()
RefEntityTmp< 0 > getRefEntity(const EntityHandle ent)
DeprecatedCoreInterface Interface
Problem_multiIndex pRoblems
problems multi-index
PetscLogEvent MOFEM_EVENT_preProcess
Event for preProcess finite element.
FieldSpace
approximation spaces
int get_comm_size() const
PetscLogEvent MOFEM_EVENT_createMat
implementation of Data Operators for Forces and Sources
boost::ptr_map< boost::typeindex::type_index, UnknownInterface > iFaces
Hash map of pointers to interfaces.
CoreTmp(moab::Interface &moab, MPI_Comm comm=PETSC_COMM_WORLD, const int verbose=VERBOSE)
Core interface class for user interface.
static int mpiInitialised
mpi was initialised by other agent
RefElement_multiIndex refinedFiniteElements
refined elements
int FieldCoefficientsNumber
Number of field coefficients.
int get_comm_rank() const
Tag get_th_RefParentHandle() const
Tag get_th_RefBitLevel() const
static bool isGloballyInitialised
Core base globally initialized.
int sIze
MoFEM communicator size.
MeshsetsManager & get_meshsets_manager()
get MeshsetsManager pointer
PetscBool initaliseAndBuildField
static constexpr const int value
const int getValue() const
Get the core.
static PetscBool isInitialized
petsc was initialised by other agent
Tag th_ElemType
Needed for VTK files.
PetscBool initaliseAndBuildFiniteElements
Data structure to exchange data between mofem and User Loop Methods.
WrapMPIComm(MPI_Comm comm, bool petsc)
boost::shared_ptr< CacheTuple > CacheTupleSharedPtr
multi_index_container< boost::shared_ptr< FieldEntity >, indexed_by< ordered_unique< tag< Unique_mi_tag >, member< FieldEntity, UId, &FieldEntity::localUId > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< FieldEntity::interface_type_RefEntity, EntityHandle, &FieldEntity::getEnt > > > > FieldEntity_multiIndex
FiniteElement_multiIndex finiteElements
finite elements
Wrap MPI comminitactor such that is destroyed when is out of scope.
Finite element definition.
Tag th_Part
Tag for partition number.
std::reference_wrapper< moab::Interface > moab
moab database
MPI_Comm & get_comm() const
boost::shared_ptr< BasicEntityData > & get_basic_entity_data_ptr()
Get pointer to basic entity data.
MPI_Comm mofemComm
MoFEM communicator.
constexpr auto field_name
multi_index_container< Problem, indexed_by< ordered_unique< tag< Meshset_mi_tag >, member< Problem, EntityHandle, &Problem::meshset > >, hashed_unique< tag< BitProblemId_mi_tag >, const_mem_fun< Problem, BitProblemId, &Problem::getId >, HashBit< BitProblemId >, EqBit< BitProblemId > >, hashed_unique< tag< Problem_mi_tag >, const_mem_fun< Problem, std::string, &Problem::getName > > > > Problem_multiIndex
MultiIndex for entities for Problem.
ParallelComm * pComm
MOAB communicator structure.
base class for all interface classes
int verbose
Verbosity level.
boost::function< MoFEMErrorCode(Interface &moab, const Field &field, const EntFiniteElement &fe, std::vector< EntityHandle > &adjacency)> ElementAdjacencyFunct
user adjacency function
int & getBuildMoFEM() const
Get flags/semaphores for different stages.
int ApproximationOrder
Approximation on the entity.
std::string optionsPrefix
Prefix for options on command line.
Field_multiIndex fIelds
fields
multi_index_container< boost::shared_ptr< Field >, indexed_by< hashed_unique< tag< BitFieldId_mi_tag >, const_mem_fun< Field, const BitFieldId &, &Field::getId >, HashBit< BitFieldId >, EqBit< BitFieldId > >, ordered_unique< tag< Meshset_mi_tag >, member< Field, EntityHandle, &Field::meshSet > >, ordered_unique< tag< FieldName_mi_tag >, const_mem_fun< Field, boost::string_ref, &Field::getNameRef > >, ordered_non_unique< tag< BitFieldId_space_mi_tag >, const_mem_fun< Field, FieldSpace, &Field::getSpace > > > > Field_multiIndex
Field_multiIndex for Field.
const MeshsetsManager & get_meshsets_manager() const
get MeshsetsManager pointer
char FieldBitNumber
Field bit number.
Tag th_ProblemLocalNbDofCol
Interface for managing meshsets containing materials and boundary conditions.
boost::weak_ptr< CacheTuple > CacheTupleWeakPtr
FieldApproximationBase
approximation base
MoFEMTypes
Those types control how functions respond on arguments, f.e. error handling.
MoFEMErrorCode set_moab_interface(moab::Interface &new_moab, int verb)
multi_index_container< boost::shared_ptr< FiniteElement >, indexed_by< hashed_unique< tag< FiniteElement_Meshset_mi_tag >, member< FiniteElement, EntityHandle, &FiniteElement::meshset > >, hashed_unique< tag< BitFEId_mi_tag >, const_mem_fun< FiniteElement, BitFEId, &FiniteElement::getId >, HashBit< BitFEId >, EqBit< BitFEId > >, ordered_unique< tag< FiniteElement_name_mi_tag >, const_mem_fun< FiniteElement, boost::string_ref, &FiniteElement::getNameRef > > > > FiniteElement_multiIndex
MultiIndex for entities for FiniteElement.
DofEntity_multiIndex dofsField
dofs on fields
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
keeps basic data about problem
FieldEntity_multiIndex entsFields
entities on fields
multi_index_container< boost::shared_ptr< DofEntity >, indexed_by< ordered_unique< tag< Unique_mi_tag >, const_mem_fun< DofEntity, UId, &DofEntity::getLocalUniqueId > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< DofEntity, EntityHandle, &DofEntity::getEnt > > > > DofEntity_multiIndex
MultiIndex container keeps DofEntity.
const moab::Interface & get_moab() const
multi_index_container< boost::shared_ptr< EntFiniteElement >, indexed_by< ordered_unique< tag< Unique_mi_tag >, const_mem_fun< EntFiniteElement, UId, &EntFiniteElement::getLocalUniqueId > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< EntFiniteElement::interface_type_RefEntity, EntityHandle, &EntFiniteElement::getEnt > > > > EntFiniteElement_multiIndex
MultiIndex container for EntFiniteElement.
PetscLogEvent MOFEM_EVENT_postProcess
Event for postProcess finite element.
boost::shared_ptr< BasicEntityData > basicEntityDataPtr
const int getValue() const
Get the core.