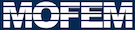 |
| v0.14.0
|
Go to the documentation of this file.
9 #ifndef __LOOPMETHODS_HPP__
10 #define __LOOPMETHODS_HPP__
278 return (*field_it)->getBitNumber();
378 *iface =
const_cast<FEMethod *
>(
this);
386 boost::shared_ptr<const NumeredEntFiniteElement>
420 inline boost::shared_ptr<FieldEntity_vector_view> &
456 const bool reset_dofs =
true);
518 #endif // __LOOPMETHODS_HPP__
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const
multi_index_container< FieldEntityEntFiniteElementAdjacencyMap, indexed_by< ordered_unique< tag< Composite_Unique_mi_tag >, composite_key< FieldEntityEntFiniteElementAdjacencyMap, const_mem_fun< FieldEntityEntFiniteElementAdjacencyMap, UId, &FieldEntityEntFiniteElementAdjacencyMap::getEntUniqueId >, const_mem_fun< FieldEntityEntFiniteElementAdjacencyMap, UId, &FieldEntityEntFiniteElementAdjacencyMap::getFeUniqueId > > >, ordered_non_unique< tag< Unique_mi_tag >, const_mem_fun< FieldEntityEntFiniteElementAdjacencyMap, UId, &FieldEntityEntFiniteElementAdjacencyMap::getEntUniqueId > >, ordered_non_unique< tag< FE_Unique_mi_tag >, const_mem_fun< FieldEntityEntFiniteElementAdjacencyMap, UId, &FieldEntityEntFiniteElementAdjacencyMap::getFeUniqueId > >, ordered_non_unique< tag< FEEnt_mi_tag >, const_mem_fun< FieldEntityEntFiniteElementAdjacencyMap, EntityHandle, &FieldEntityEntFiniteElementAdjacencyMap::getFeHandle > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< FieldEntityEntFiniteElementAdjacencyMap, EntityHandle, &FieldEntityEntFiniteElementAdjacencyMap::getEntHandle > > > > FieldEntityEntFiniteElementAdjacencyMap_multiIndex
MultiIndex container keeps Adjacencies Element and dof entities adjacencies and vice versa.
auto & getRowFieldEnts() const
std::bitset< 8 > Switches
int getNinTheLoop() const
get number of evaluated element in the loop
const RefEntity_multiIndex * refinedEntitiesPtr
container of mofem dof entities
PetscReal ts_a
shift for U_t (see PETSc Time Solver)
auto & getColFieldEntsPtr() const
int nInTheLoop
number currently of processed method
#define BITFEID_SIZE
max number of finite elements
auto getColDofsPtr() const
const EntFiniteElement_multiIndex * finiteElementsEntitiesPtr
static constexpr Switches CtxSetB
Data structure to exchange data between mofem and User Loop Methods on entities.
Vec & ts_F
residual vector
structure for User Loop Methods on finite elements
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const
auto getDataDofsPtr() const
static constexpr Switches CtxSetA
std::string feName
Name of finite element.
MoFEMErrorCode copyPetscData(const PetscData &petsc_data)
multi_index_container< boost::shared_ptr< RefElement >, indexed_by< ordered_unique< tag< Ent_mi_tag >, const_mem_fun< RefElement::interface_type_RefEntity, EntityHandle, &RefElement::getEnt > > > > RefElement_multiIndex
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
std::vector< boost::weak_ptr< FieldEntity > > FieldEntity_vector_view
Mat & snes_B
preconditioner of jacobian matrix
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const
data structure for snes (nonlinear solver) context
const FieldEntity_vector_view & getDataFieldEnts() const
auto getHiFERank() const
Get upper rank in loop for iterating elements.
MultiIndex Tag for field name.
boost::function< MoFEMErrorCode()> preProcessHook
Hook function for pre-processing.
Data structure to exchange data between mofem and User Loop Methods on entities.
Mat & snes_A
jacobian matrix
MoFEMErrorCode getNodeData(const std::string field_name, VectorDouble &data, const bool reset_dofs=true)
multi_index_container< boost::shared_ptr< RefEntity >, indexed_by< ordered_unique< tag< Ent_mi_tag >, const_mem_fun< RefEntity, EntityHandle, &RefEntity::getEnt > >, ordered_non_unique< tag< Ent_Ent_mi_tag >, const_mem_fun< RefEntity, EntityHandle, &RefEntity::getParentEnt > >, ordered_non_unique< tag< Composite_EntType_and_ParentEntType_mi_tag >, composite_key< RefEntity, const_mem_fun< RefEntity, EntityType, &RefEntity::getEntType >, const_mem_fun< RefEntity, EntityType, &RefEntity::getParentEntType > > >, ordered_non_unique< tag< Composite_ParentEnt_And_EntType_mi_tag >, composite_key< RefEntity, const_mem_fun< RefEntity, EntityType, &RefEntity::getEntType >, const_mem_fun< RefEntity, EntityHandle, &RefEntity::getParentEnt > > > > > RefEntity_multiIndex
#define THROW_MESSAGE(msg)
Throw MoFEM exception.
boost::shared_ptr< DofEntity > dofPtr
DEPRECATED typedef DofMethod EntMethod
virtual ~KspMethod()=default
const Field_multiIndex * fieldsPtr
raw pointer to fields container
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const
const FieldEntityEntFiniteElementAdjacencyMap_multiIndex * adjacenciesPtr
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const
int sIze
number of processors in communicator
auto getRowDofsPtr() const
const FieldEntity_multiIndex * entitiesPtr
raw pointer to container of field entities
int getLoopSize() const
get loop size
implementation of Data Operators for Forces and Sources
auto getLoFERank() const
Get upper rank in loop for iterating elements.
data structure for ksp (linear solver) context
boost::shared_ptr< FieldEntity > entPtr
auto getNumberOfNodes() const
KSPContext
pass information about context of KSP/DM for with finite element is computed
boost::shared_ptr< Field > fieldPtr
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const
MoFEMErrorCode copySnes(const SnesMethod &snes)
Copy snes data.
Vec & ts_u_t
time derivative of state vector
static constexpr Switches CtxSetX_T
virtual MoFEMErrorCode preProcess()
function is run at the beginning of loop
Vec & snes_dx
solution update
boost::function< MoFEMErrorCode()> postProcessHook
Hook function for post-processing.
const RefElement_multiIndex * refinedFiniteElementsPtr
container of mofem finite element entities
auto getFEName() const
get finite element name
static constexpr Switches CtxSetTime
static constexpr Switches CtxSetNone
Data structure to exchange data between mofem and User Loop Methods.
int loopSize
local number oe methods to process
multi_index_container< boost::shared_ptr< FieldEntity >, indexed_by< ordered_unique< tag< Unique_mi_tag >, member< FieldEntity, UId, &FieldEntity::localUId > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< FieldEntity::interface_type_RefEntity, EntityHandle, &FieldEntity::getEnt > > > > FieldEntity_multiIndex
auto getDataVectorDofsPtr() const
constexpr auto field_name
boost::movelib::unique_ptr< bool > vecAssembleSwitch
static constexpr Switches CtxSetX_TT
MoFEMErrorCode copyBasicMethod(const BasicMethod &basic)
Copy data from other base method to this base method.
virtual ~TSMethod()=default
static constexpr Switches CtxSetX
PetscInt ts_step
time step number
base class for all interface classes
const FiniteElement_multiIndex * finiteElementsPtr
raw pointer to container finite elements
auto & getRowFieldEntsPtr() const
boost::function< MoFEMErrorCode()> operatorHook
Hook function for operator.
virtual ~BasicMethod()=default
unsigned int getFieldBitNumber(std::string field_name) const
PetscReal ts_aa
shift for U_tt shift for U_tt
const DofEntity_multiIndex * dofsPtr
raw pointer container of dofs
static constexpr Switches CtxSetDX
multi_index_container< boost::shared_ptr< Field >, indexed_by< hashed_unique< tag< BitFieldId_mi_tag >, const_mem_fun< Field, const BitFieldId &, &Field::getId >, HashBit< BitFieldId >, EqBit< BitFieldId > >, ordered_unique< tag< Meshset_mi_tag >, member< Field, EntityHandle, &Field::meshSet > >, ordered_unique< tag< FieldName_mi_tag >, const_mem_fun< Field, boost::string_ref, &Field::getNameRef > >, ordered_non_unique< tag< BitFieldId_space_mi_tag >, const_mem_fun< Field, FieldSpace, &Field::getSpace > > > > Field_multiIndex
Field_multiIndex for Field.
MoFEMErrorCode copyTs(const TSMethod &ts)
Copy TS solver data.
data structure for TS (time stepping) context
boost::weak_ptr< CacheTuple > cacheWeakPtr
MoFEMErrorCode copyKsp(const KspMethod &ksp)
copy data form another method
boost::shared_ptr< NumeredDofEntity > dofNumeredPtr
const FTensor::Tensor2< T, Dim, Dim > Vec
const Problem * problemPtr
raw pointer to problem
boost::function< bool(FEMethod *fe_method_ptr)> exeTestHook
Tet if element to skip element.
auto & getColFieldEnts() const
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const
std::pair< int, int > loHiFERank
Llo and hi processor rank of iterated entities.
UBlasVector< double > VectorDouble
multi_index_container< boost::shared_ptr< FiniteElement >, indexed_by< hashed_unique< tag< FiniteElement_Meshset_mi_tag >, member< FiniteElement, EntityHandle, &FiniteElement::meshset > >, hashed_unique< tag< BitFEId_mi_tag >, const_mem_fun< FiniteElement, BitFEId, &FiniteElement::getId >, HashBit< BitFEId >, EqBit< BitFEId > >, ordered_unique< tag< FiniteElement_name_mi_tag >, const_mem_fun< FiniteElement, boost::string_ref, &FiniteElement::getNameRef > > > > FiniteElement_multiIndex
MultiIndex for entities for FiniteElement.
Partitioned (Indexed) Finite Element in Problem.
EntityHandle getFEEntityHandle() const
boost::shared_ptr< const NumeredEntFiniteElement > numeredEntFiniteElementPtr
virtual MoFEMErrorCode postProcess()
function is run at the end of loop
static constexpr Switches CtxSetF
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
keeps basic data about problem
Field_multiIndex::index< FieldName_mi_tag >::type::iterator field_it
PetscReal ts_dt
time step size
boost::weak_ptr< CacheTuple > getCacheWeakPtr() const
Get the cache weak ptr object.
multi_index_container< boost::shared_ptr< DofEntity >, indexed_by< ordered_unique< tag< Unique_mi_tag >, const_mem_fun< DofEntity, UId, &DofEntity::getLocalUniqueId > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< DofEntity, EntityHandle, &DofEntity::getEnt > > > > DofEntity_multiIndex
MultiIndex container keeps DofEntity.
boost::shared_ptr< FieldEntity_vector_view > & getDataFieldEntsPtr() const
virtual ~SnesMethod()=default
Mat & ts_B
Preconditioner for ts_A.
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const
boost::shared_ptr< Field > fieldPtr
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
multi_index_container< boost::shared_ptr< EntFiniteElement >, indexed_by< ordered_unique< tag< Unique_mi_tag >, const_mem_fun< EntFiniteElement, UId, &EntFiniteElement::getLocalUniqueId > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< EntFiniteElement::interface_type_RefEntity, EntityHandle, &EntFiniteElement::getEnt > > > > EntFiniteElement_multiIndex
MultiIndex container for EntFiniteElement.
virtual ~PetscData()=default
Vec & ts_u_tt
second time derivative of state vector
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
virtual MoFEMErrorCode operator()()
function is run for every finite element
auto getLoHiFERank() const
Get lo and hi processor rank of iterated entities.
KSPContext ksp_ctx
Context.
boost::movelib::unique_ptr< bool > matAssembleSwitch