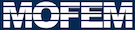 |
| v0.14.0
|
Go to the documentation of this file.
8 #ifndef __FIELD_ENTSMULTIINDICES_HPP__
9 #define __FIELD_ENTSMULTIINDICES_HPP__
13 struct EntityCacheDofs;
14 struct EntityCacheNumeredDofs;
39 FieldEntity(
const boost::shared_ptr<Field> field_ptr,
40 const boost::shared_ptr<RefEntity> ref_ents_ptr,
41 boost::shared_ptr<double *const> field_data_adaptor_ptr,
42 boost::shared_ptr<const int> t_max_order_ptr);
60 const boost::shared_ptr<Field> &field_ptr,
61 const boost::shared_ptr<RefEntity> &ref_ents_ptr);
171 (
static_cast<UId>(owner_proc) |
179 return static_cast<int>(
206 constexpr
int bit_field_mask = ~(char(0));
207 return static_cast<char>(
231 static_cast<UId>(std::numeric_limits<EntityHandle>::max())
287 template <
typename T = EntityStorage>
307 inline boost::shared_ptr<EntityStorage>
308 FieldEntity::getSharedStoragePtr<EntityStorage>()
const {
318 template <
typename T>
329 return this->
sPtr->getEntFieldData();
334 return this->
sPtr->getOrderNbDofs(order);
339 return this->
sPtr->getOrderNbDofsDiff(order);
344 return this->
sPtr->getMaxOrder();
349 return this->
sPtr->getGlobalUniqueId();
354 return this->
sPtr->getLocalUniqueId();
359 return this->
sPtr->getRefEntityPtr();
376 return this->
sPtr->getDofOrderMap();
380 template <
typename S = EntityStorage>
382 return this->
sPtr->template getSharedStoragePtr<S>();
386 return this->
sPtr->getWeakStoragePtr();
397 const bool reduce_tag_size =
false)
417 boost::shared_ptr<FieldEntity>,
419 ordered_unique<tag<Unique_mi_tag>,
420 member<FieldEntity, UId, &FieldEntity::localUId>>,
421 ordered_non_unique<tag<Ent_mi_tag>,
437 boost::shared_ptr<FieldEntity>,
442 const_mem_fun<FieldEntity, UId, &FieldEntity::getGlobalUniqueId>>
447 boost::shared_ptr<FieldEntity>,
452 ordered_non_unique<tag<Ent_mi_tag>,
459 boost::shared_ptr<FieldEntity>,
465 tag<Composite_EntType_and_Space_mi_tag>,
481 boost::shared_ptr<FieldEntity>,
483 ordered_unique<tag<Unique_mi_tag>,
484 member<FieldEntity, UId, &FieldEntity::localUId>>,
485 ordered_non_unique<tag<Ent_mi_tag>,
493 #endif // __FIELD_ENTSMULTIINDICES_HPP__
UId getGlobalUniqueIdCalculate() const
Calculate global UId.
FieldBitNumber getBitNumber() const
virtual ~FieldEntity()=default
VectorAdaptor getEntFieldData() const
Get vector of DOFs active values on entity.
multi_index_container< boost::shared_ptr< FieldEntity >, indexed_by< sequenced<>, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< FieldEntity::interface_type_RefEntity, EntityHandle, &FieldEntity::getEnt > > > > FieldEntity_multiIndex_ent_view
const std::array< ApproximationOrder, MAX_DOFS_ON_ENTITY > & getDofOrderMap(const EntityType type) const
get hash-map relating dof index on entity with its order
FieldEntity_change_order(const ApproximationOrder order, const bool reduce_tag_size=false)
int getOrderNbDofs(ApproximationOrder order) const
Get number of DOFs on entity for given order of approximation.
static UId getLoLocalEntityBitNumber(const char bit_number, const EntityHandle ent)
static UId getLoFieldEntityUId(const UId &uid)
boost::shared_ptr< S > getSharedStoragePtr() const
Get the Weak Storage pointer.
std::vector< boost::weak_ptr< FieldEntity > > FieldEntity_vector_view
boost::shared_ptr< RefEntity > & getRefEntityPtr() const
const ApproximationOrder order
boost::shared_ptr< T > sPtr
structure to change FieldEntity order
Interface to FieldEntity.
EntityHandle getEnt() const
Get the entity handle.
interface_RefEntity< RefEntity > interface_type_RefEntity
static UId getGlobalUniqueIdCalculate(const int owner_proc, const char bit_number, const EntityHandle moab_owner_handle)
Calculate UId for field entity.
UId getGlobalUniqueId() const
static UId getLocalUniqueIdCalculate(const char bit_number, const EntityHandle handle)
Get the Local Unique Id Calculate.
boost::shared_ptr< FieldData *const > & getEntFieldDataPtr() const
Get shared ptr to vector adaptor pointing to the field tag data on entity.
static UId getHiFieldEntityUId(const UId &uid)
multi_index_container< boost::shared_ptr< FieldEntity >, indexed_by< sequenced<>, ordered_non_unique< tag< Composite_EntType_and_Space_mi_tag >, composite_key< FieldEntity, const_mem_fun< FieldEntity::interface_type_RefEntity, EntityType, &FieldEntity::getEntType >, const_mem_fun< FieldEntity::interface_type_Field, FieldSpace, &FieldEntity::getSpace > > > > > FieldEntity_multiIndex_spaceType_view
virtual ~EntityStorage()=default
FieldSpace
approximation spaces
boost::shared_ptr< FieldEntity > & getFieldEntityPtr() const
implementation of Data Operators for Forces and Sources
#define MAX_PROCESSORS_NUMBER
Maximal number of processors.
auto getVectorAdaptor(T1 ptr, const size_t n)
Get Vector adaptor.
static auto getHandleFromUniqueId(const UId uid)
Get the Handle From Unique Id.
static boost::shared_ptr< FieldData *const > makeSharedFieldDataAdaptorPtr(const boost::shared_ptr< Field > &field_ptr, const boost::shared_ptr< RefEntity > &ref_ents_ptr)
Return shared pointer to entity field data vector adaptor.
UId getLocalUniqueIdCalculate()
Get the Local Unique Id Calculate object.
static UId getLoBitNumberUId(const FieldBitNumber bit_number)
boost::shared_ptr< const ApproximationOrder > tagMaxOrderPtr
boost::shared_ptr< RefEntity > & getRefEntityPtr() const
FieldEntity_multiIndex::index< Unique_mi_tag >::type FieldEntityByUId
Entity index by field name.
Struct keeps handle to entity in the field.
int getNbDofsOnEnt() const
UId getGlobalUniqueId() const
Get global unique id.
multi_index_container< boost::shared_ptr< FieldEntity >, indexed_by< ordered_unique< tag< Unique_mi_tag >, const_mem_fun< FieldEntity, UId, &FieldEntity::getGlobalUniqueId > > > > FieldEntity_multiIndex_global_uid_view
multi-index view on DofEntity by uid
FieldEntity(const boost::shared_ptr< Field > field_ptr, const boost::shared_ptr< RefEntity > ref_ents_ptr, boost::shared_ptr< double *const > field_data_adaptor_ptr, boost::shared_ptr< const int > t_max_order_ptr)
FieldCoefficientsNumber getNbOfCoeffs() const
VectorShallowArrayAdaptor< double > VectorAdaptor
boost::weak_ptr< EntityCacheNumeredDofs > entityCacheColDofs
multi_index_container< boost::shared_ptr< FieldEntity >, indexed_by< ordered_unique< tag< Unique_mi_tag >, member< FieldEntity, UId, &FieldEntity::localUId > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< FieldEntity::interface_type_RefEntity, EntityHandle, &FieldEntity::getEnt > > > > FieldEntity_multiIndex
boost::weak_ptr< EntityCacheNumeredDofs > entityCacheRowDofs
static constexpr int proc_shift
boost::shared_ptr< FieldData *const > fieldDataAdaptorPtr
int getOrderNbDofs(ApproximationOrder order) const
const std::array< int, MAX_DOFS_ON_ENTITY > & getDofOrderMap() const
get hash-map relating dof index on entity with its order
interface_Field< Field, RefEntity > interface_type_Field
#define MAX_DOFS_ON_ENTITY
Maximal number of DOFs on entity.
void operator()(boost::shared_ptr< FieldEntity > &e)
boost::weak_ptr< EntityCacheDofs > entityCacheDataDofs
VectorAdaptor getEntFieldData() const
ApproximationOrder getMaxOrder() const
Get order set to the entity (Allocated tag size for such number)
boost::shared_ptr< T > getSharedStoragePtr() const
Get the Weak Storage pointer.
int ApproximationOrder
Approximation on the entity.
int getOrderNbDofsDiff(ApproximationOrder order) const
boost::weak_ptr< EntityStorage > & getWeakStoragePtr() const
static constexpr int ent_shift
static constexpr int dof_shift
char FieldBitNumber
Field bit number.
interface_FieldEntity(const boost::shared_ptr< T > &sptr)
const ApproximationOrder * getMaxOrderPtr() const
Get pinter to Tag keeping approximation order.
static UId getHiBitNumberUId(const FieldBitNumber bit_number)
friend std::ostream & operator<<(std::ostream &os, const FieldEntity &e)
int getNbDofsOnEnt() const
Get number of active DOFs on entity.
static UId getHiLocalEntityBitNumber(const char bit_number, const EntityHandle ent)
EntityType getEntType() const
Get entity type.
std::vector< FieldData > data
FieldSpace getSpace() const
ApproximationOrder getMaxOrder() const
const Field * getFieldRawPtr() const
const std::array< int, MAX_DOFS_ON_ENTITY > & getDofOrderMap() const
get hash-map relating dof index on entity with its order
static auto getFieldBitNumberFromUniqueId(const UId uid)
Get the Field Bit Number From Unique Id.
UId & getLocalUniqueId() const
boost::weak_ptr< EntityStorage > weakStoragePtr
EntityHandle getOwnerEnt() const
UId localUId
Local unique id for this entity. Unique on CPU partition.
boost::weak_ptr< EntityStorage > & getWeakStoragePtr() const
const UId & getLocalUniqueId() const
Get global unique id.
static auto getOwnerFromUniqueId(const UId uid)
int getOrderNbDofsDiff(ApproximationOrder order) const
Get difference of number of DOFs between order and order-1.