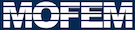 |
| v0.14.0
|
Go to the documentation of this file.
14 #ifndef __FIELDMULTIINDICES_HPP__
15 #define __FIELDMULTIINDICES_HPP__
60 virtual ~Field() =
default;
64 boost::weak_ptr<std::vector<DofEntity>>,
66 indexed_by<sequenced<>>>;
68 typedef std::array<std::array<int, MAX_DOFS_ON_ENTITY>, MBMAXTYPE>
206 "Too many fields allowed, can be more but ...");
253 inline const std::array<ApproximationOrder, MAX_DOFS_ON_ENTITY> &
289 template <
typename FIELD,
typename REFENT>
295 const boost::shared_ptr<REFENT> &ref_ents_ptr)
300 template <
typename FIELD,
typename REFENT>
304 const boost::shared_ptr<REFENT> &ref_ents_ptr)
359 inline std::array<ApproximationOrder, MAX_DOFS_ON_ENTITY> &
373 mutable boost::shared_ptr<FIELD>
sFieldPtr;
376 template <
typename T>
438 inline std::array<ApproximationOrder, MAX_DOFS_ON_ENTITY> &
454 typedef multi_index_container<
455 boost::shared_ptr<Field>,
457 hashed_unique<tag<BitFieldId_mi_tag>,
458 const_mem_fun<Field, const BitFieldId &, &Field::getId>,
459 HashBit<BitFieldId>, EqBit<BitFieldId>>,
460 ordered_unique<tag<Meshset_mi_tag>,
461 member<Field, EntityHandle, &Field::meshSet>>,
463 tag<FieldName_mi_tag>,
464 const_mem_fun<Field, boost::string_ref, &Field::getNameRef>>,
465 ordered_non_unique<tag<BitFieldId_space_mi_tag>,
466 const_mem_fun<Field, FieldSpace, &Field::getSpace>>>>
469 typedef multi_index_container<
470 boost::shared_ptr<Field>,
472 ordered_unique<tag<BitFieldId_mi_tag>,
473 const_mem_fun<Field, const BitFieldId &, &Field::getId>,
479 #endif // __FIELDMULTIINDICES_HPP__
auto getSpaceName() const
Get field approximation space.
TagType tagFieldDataVertsType
std::array< std::array< int, MAX_DOFS_ON_ENTITY >, MBMAXTYPE > DofsOrderMap
FieldBitNumber getBitNumber() const
FieldCoefficientsNumber getNbOfCoeffs() const
Get number of field coefficients.
virtual ~interface_FieldImpl()=default
const std::array< ApproximationOrder, MAX_DOFS_ON_ENTITY > & getDofOrderMap(const EntityType type) const
get hash-map relating dof index on entity with its order
keeps information about DOF on the entity
EntityHandle getMeshset() const
Get field meshset.
const static char *const ApproximationBaseNames[]
FieldSpace * tagSpaceData
tag keeps field space
MoFEMErrorCode rebuildDofsOrderMap()
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
FieldSpace getSpace() const
Get field approximation space.
boost::shared_ptr< REFENT > & getRefEntityPtr() const
std::array< ApproximationOrder, MAX_DOFS_ON_ENTITY > & getDofOrderMap(const EntityType type) const
get hash-map relating dof index on entity with its order
std::bitset< BITFIELDID_SIZE > BitFieldId
Field Id.
FieldOrderTable & getFieldOrderTable()
Get the Field Order Table.
Tag th_FieldData
Tag storing field values on entity in the field.
FieldOrderFunct FieldOrderTable[MBMAXTYPE]
user adjacency function table
std::string getName() const
Get field name.
SequenceDofContainer sequenceDofContainer
FieldOrderTable & getFieldOrderTable()
interface_Field(const boost::shared_ptr< T > &ptr)
boost::string_ref getNameRef() const
auto getApproxBaseName() const
Get approximation base.
Provide data structure for (tensor) field approximation.
FieldApproximationBase getApproxBase() const
multi_index_container< boost::shared_ptr< Field >, indexed_by< ordered_unique< tag< BitFieldId_mi_tag >, const_mem_fun< Field, const BitFieldId &, &Field::getId >, LtBit< BitFieldId > > > > Field_multiIndex_view
DeprecatedCoreInterface Interface
FieldSpace
approximation spaces
int tagNameSize
number of bits necessary to keep field name
const BitFieldId & getId() const
Get unique field id.
std::string getName() const
const DofsOrderMap & getDofOrderMap() const
get hash-map relating dof index on entity with its order
implementation of Data Operators for Forces and Sources
auto getApproxBaseName() const
#define BITFIELDID_SIZE
max number of fields
boost::string_ref getNameRef() const
Get string reference to field name.
std::array< ApproximationOrder, MAX_DOFS_ON_ENTITY > & getDofOrderMap(const EntityType type) const
get hash-map relating dof index on entity with its order
const Field * getFieldRawPtr() const
Tag th_FieldDataVerts
Tag storing field values on vertices in the field.
int FieldCoefficientsNumber
Number of field coefficients.
Pointer interface for MoFEM::Field.
const Field * getFieldRawPtr() const
boost::string_ref getNameRef() const
friend std::ostream & operator<<(std::ostream &os, const Field &e)
FieldApproximationBase * tagBaseData
tag keeps field base
FieldApproximationBase getApproxBase() const
Get approximation base.
const BitFieldId & getId() const
boost::shared_ptr< FIELD > sFieldPtr
const void * tagName
tag keeps name of the field
FieldCoefficientsNumber getNbOfCoeffs() const
multi_index_container< boost::weak_ptr< std::vector< DofEntity > >, indexed_by< sequenced<> >> SequenceDofContainer
FieldCoefficientsNumber * tagNbCoeffData
FieldCoefficientsNumber getNbOfCoeffs() const
FieldSpace getSpace() const
static FieldBitNumber getBitNumberCalculate(const BitFieldId &id)
Calculate number of set bit in Field ID. Each field has uid, get getBitNumber get number of bit set f...
boost::function< int(const int order)> FieldOrderFunct
user adjacency function
auto getSpaceName() const
const static char *const FieldSpaceNames[]
Tag th_AppOrder
Tag storing approximation order on entity.
Field(moab::Interface &moab, const EntityHandle meshset)
constructor for moab field
FieldBitNumber getBitNumber() const
Get number of set bit in Field ID. Each field has uid, get getBitNumber get number of bit set for giv...
EntityHandle getMeshset() const
multi_index_container< boost::shared_ptr< Field >, indexed_by< hashed_unique< tag< BitFieldId_mi_tag >, const_mem_fun< Field, const BitFieldId &, &Field::getId >, HashBit< BitFieldId >, EqBit< BitFieldId > >, ordered_unique< tag< Meshset_mi_tag >, member< Field, EntityHandle, &Field::meshSet > >, ordered_unique< tag< FieldName_mi_tag >, const_mem_fun< Field, boost::string_ref, &Field::getNameRef > >, ordered_non_unique< tag< BitFieldId_space_mi_tag >, const_mem_fun< Field, FieldSpace, &Field::getSpace > > > > Field_multiIndex
Field_multiIndex for Field.
interface_FieldImpl(const boost::shared_ptr< FIELD > &field_ptr, const boost::shared_ptr< REFENT > &ref_ents_ptr)
char FieldBitNumber
Field bit number.
EntityHandle getMeshset() const
FieldApproximationBase getApproxBase() const
FieldApproximationBase
approximation base
const void * tagNamePrefixData
tag keeps name prefix of the field
FieldSpace getSpace() const
SequenceDofContainer & getDofSequenceContainer() const
Get reference to sequence data container.
const Field * getFieldRawPtr() const
FieldBitNumber getBitNumberCalculate() const
Calculate number of set bit in Field ID. Each field has uid, get getBitNumber get number of bit set f...
std::string getName() const
FieldBitNumber getBitNumber() const
FieldOrderTable forderTable
nb. DOFs table for entities
EntityHandle meshSet
keeps entities for this meshset
interface_Field(const boost::shared_ptr< FIELD > &field_ptr, const boost::shared_ptr< REFENT > &ref_ents_ptr)
int getCoordSysDim(const int d=0) const
const BitFieldId & getId() const
BitFieldId * tagId
Tag field rank.