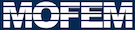 |
| v0.14.0
|
Go to the documentation of this file.
5 #ifndef __INTERFACE_HPP__
6 #define __INTERFACE_HPP__
12 struct MeshsetsManager;
36 virtual const int getValue()
const = 0;
44 virtual boost::shared_ptr<RefEntityTmp<0>>
112 virtual MPI_Comm &
get_comm()
const = 0;
146 const std::string &name)
const = 0;
219 const bool remove_parent =
false,
263 const std::string name,
const FieldSpace space,
269 std::pair<EntityType,
275 const TagType tag_type = MB_TAG_SPARSE,
301 const TagType tag_type = MB_TAG_SPARSE,
331 const std::string &name,
350 const std::string &name,
370 const std::string &name,
const bool recursive =
true,
390 const std::string &name,
391 const bool recursive =
true,
412 const double coords[],
int size,
436 const EntityType
type,
472 const EntityType
type,
473 const std::string &name,
488 const std::string &name,
573 Range &ents)
const = 0;
586 Range &ents)
const = 0;
597 Range &ents)
const = 0;
606 virtual bool check_field(
const std::string &name)
const = 0;
619 virtual const Field *
679 const EntityType
type,
695 const std::string name_field) = 0;
710 const std::string name_field) = 0;
723 const std::string name_row) = 0;
736 const std::string name_row) = 0;
749 const std::string name_row) = 0;
762 const std::string name_row) = 0;
778 const std::string name,
const bool recursive =
true) = 0;
794 const std::string name,
795 const bool recursive =
true) = 0;
810 const std::string name) = 0;
825 const std::string name) = 0;
861 Range &ents)
const = 0;
874 Range &ents)
const = 0;
886 Range &ents)
const = 0;
934 const std::string &name,
935 const bool recursive =
false) = 0;
986 const std::string &fe_name) = 0;
1001 const std::string &fe_name) = 0;
1175 const Range *
const ents_ptr =
nullptr,
1322 const std::string &fe_name,
1453 const std::string problem_name,
const std::string &fe_name,
1455 boost::shared_ptr<NumeredEntFiniteElement_multiIndex> fe_ptr =
nullptr,
1498 const Problem *problem_ptr,
const std::string &fe_name,
FEMethod &method,
1499 int lower_rank,
int upper_rank,
1500 boost::shared_ptr<NumeredEntFiniteElement_multiIndex> fe_ptr =
nullptr,
1541 const std::string problem_name,
const std::string &fe_name,
1542 FEMethod &method,
int lower_rank,
int upper_rank,
1543 boost::shared_ptr<NumeredEntFiniteElement_multiIndex> fe_ptr =
nullptr,
1597 Range const *
const ents =
nullptr,
1616 int lower_rank,
int upper_rank,
1635 int lower_rank,
int upper_rank,
1741 *dofs_elements_adjacency)
const = 0;
1785 const Problem **problem_ptr)
const = 0;
1822 virtual FieldEntityByUId::iterator
1836 virtual FieldEntityByUId::iterator
1842 #define _IT_GET_ENT_FIELD_BY_NAME_FOR_LOOP_(MFIELD, NAME, IT) \
1843 auto IT = (MFIELD).get_ent_field_by_name_begin(NAME); \
1844 IT != (MFIELD).get_ent_field_by_name_end(NAME); \
1858 virtual DofEntityByUId::iterator
1872 virtual DofEntityByUId::iterator
1878 #define _IT_GET_DOFS_FIELD_BY_NAME_FOR_LOOP_(MFIELD, NAME, IT) \
1879 DofEntityByUId::iterator IT = (MFIELD).get_dofs_by_name_begin(NAME); \
1880 IT != (MFIELD).get_dofs_by_name_end(NAME); \
1895 virtual DofEntityByUId::iterator
1910 virtual DofEntityByUId::iterator
1917 #define _IT_GET_DOFS_FIELD_BY_NAME_AND_ENT_FOR_LOOP_(MFIELD, NAME, ENT, IT) \
1918 DofEntityByUId::iterator IT = \
1919 (MFIELD).get_dofs_by_name_and_ent_begin(NAME, ENT); \
1920 IT != (MFIELD).get_dofs_by_name_and_ent_end(NAME, ENT); \
1936 virtual DofEntityByUId::iterator
1938 const EntityType
type)
const = 0;
1953 virtual DofEntityByUId::iterator
1955 const EntityType
type)
const = 0;
1960 #define _IT_GET_DOFS_FIELD_BY_NAME_AND_TYPE_FOR_LOOP_(MFIELD, NAME, TYPE, IT) \
1961 DofEntityByUId::iterator IT = \
1962 (MFIELD).get_dofs_by_name_and_type_begin(NAME, TYPE); \
1963 IT != (MFIELD).get_dofs_by_name_and_type_end(NAME, TYPE); \
1978 virtual EntFiniteElement_multiIndex::index<Unique_mi_tag>::type::iterator
1993 virtual EntFiniteElement_multiIndex::index<Unique_mi_tag>::type::iterator
1999 #define _IT_GET_FES_BY_NAME_FOR_LOOP_(MFIELD, NAME, IT) \
2000 auto IT = (MFIELD).get_fe_by_name_begin(NAME); \
2001 IT != (MFIELD).get_fe_by_name_end(NAME); \
2014 #endif // __INTERFACE_HPP__
virtual MoFEMErrorCode modify_finite_element_adjacency_table(const std::string &fe_name, const EntityType type, ElementAdjacencyFunct function)=0
modify finite element table, only for advanced user
multi_index_container< FieldEntityEntFiniteElementAdjacencyMap, indexed_by< ordered_unique< tag< Composite_Unique_mi_tag >, composite_key< FieldEntityEntFiniteElementAdjacencyMap, const_mem_fun< FieldEntityEntFiniteElementAdjacencyMap, UId, &FieldEntityEntFiniteElementAdjacencyMap::getEntUniqueId >, const_mem_fun< FieldEntityEntFiniteElementAdjacencyMap, UId, &FieldEntityEntFiniteElementAdjacencyMap::getFeUniqueId > > >, ordered_non_unique< tag< Unique_mi_tag >, const_mem_fun< FieldEntityEntFiniteElementAdjacencyMap, UId, &FieldEntityEntFiniteElementAdjacencyMap::getEntUniqueId > >, ordered_non_unique< tag< FE_Unique_mi_tag >, const_mem_fun< FieldEntityEntFiniteElementAdjacencyMap, UId, &FieldEntityEntFiniteElementAdjacencyMap::getFeUniqueId > >, ordered_non_unique< tag< FEEnt_mi_tag >, const_mem_fun< FieldEntityEntFiniteElementAdjacencyMap, EntityHandle, &FieldEntityEntFiniteElementAdjacencyMap::getFeHandle > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< FieldEntityEntFiniteElementAdjacencyMap, EntityHandle, &FieldEntityEntFiniteElementAdjacencyMap::getEntHandle > > > > FieldEntityEntFiniteElementAdjacencyMap_multiIndex
MultiIndex container keeps Adjacencies Element and dof entities adjacencies and vice versa.
virtual MeshsetsManager * get_meshsets_manager_ptr()=0
get MeshsetsManager pointer
virtual std::string get_field_name(const BitFieldId id) const =0
get field name from id
virtual const EntFiniteElement_multiIndex * get_ents_finite_elements() const =0
Get the ents finite elements object.
virtual MoFEMErrorCode problem_basic_method_postProcess(const Problem *problem_ptr, BasicMethod &method, int verb=DEFAULT_VERBOSITY)=0
Set data for BasicMethod.
virtual MoFEMErrorCode loop_finite_elements(const std::string problem_name, const std::string &fe_name, FEMethod &method, boost::shared_ptr< NumeredEntFiniteElement_multiIndex > fe_ptr=nullptr, MoFEMTypes bh=MF_EXIST, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr(), int verb=DEFAULT_VERBOSITY)=0
Make a loop over finite elements.
virtual MoFEMErrorCode add_ents_to_finite_element_by_MESHSET(const EntityHandle meshset, const std::string &name, const bool recursive=false)=0
add MESHSET element to finite element database given by name
virtual MoFEMErrorCode loop_dofs(const Problem *problem_ptr, const std::string &field_name, RowColData rc, DofMethod &method, int lower_rank, int upper_rank, int verb=DEFAULT_VERBOSITY)=0
Make a loop over dofs.
virtual MoFEMErrorCode clear_adjacencies_entities(const BitRefLevel bit, const BitRefLevel mask, int verb=DEFAULT_VERBOSITY)=0
clear adjacency map for entities on given bit level
virtual EntityHandle get_finite_element_meshset(const std::string name) const =0
virtual MoFEMErrorCode list_finite_elements() const =0
list finite elements in database
virtual MoFEMErrorCode modify_problem_ref_level_set_bit(const std::string &name_problem, const BitRefLevel &bit)=0
set ref level for problem
virtual MoFEMErrorCode check_number_of_ents_in_ents_finite_element() const =0
check data consistency in entsFiniteElements
Data structure to exchange data between mofem and User Loop Methods on entities.
structure for User Loop Methods on finite elements
multi_index_container< DofsSideMapData, indexed_by< ordered_non_unique< tag< TypeSide_mi_tag >, composite_key< DofsSideMapData, member< DofsSideMapData, EntityType, &DofsSideMapData::type >, member< DofsSideMapData, int, &DofsSideMapData::side > >>, ordered_unique< tag< EntDofIdx_mi_tag >, member< DofsSideMapData, int, &DofsSideMapData::dof > > > > DofsSideMap
Map entity stype and side to element/entity dof index.
virtual const FieldEntityEntFiniteElementAdjacencyMap_multiIndex * get_ents_elements_adjacency() const =0
Get the dofs elements adjacency object.
virtual MoFEMErrorCode set_moab_interface(moab::Interface &new_moab, int verb=VERBOSE)=0
Set the moab interface object.
virtual MPI_Comm & get_comm() const =0
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
virtual FieldEntityByUId::iterator get_ent_field_by_name_end(const std::string &field_name) const =0
get begin iterator of field dofs of given name (instead you can use IT_GET_ENT_FIELD_BY_NAME_FOR_LOOP...
virtual boost::shared_ptr< RefEntityTmp< 0 > > make_shared_ref_entity(const EntityHandle ent)=0
Get RefEntity.
multi_index_container< boost::shared_ptr< RefElement >, indexed_by< ordered_unique< tag< Ent_mi_tag >, const_mem_fun< RefElement::interface_type_RefEntity, EntityHandle, &RefElement::getEnt > > > > RefElement_multiIndex
virtual const Field * get_field_structure(const std::string &name, enum MoFEMTypes bh=MF_EXIST) const =0
get field structure
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
virtual MoFEMErrorCode create_vertices_and_add_to_field(const std::string name, const double coords[], int size, int verb=DEFAULT_VERBOSITY)=0
Create a vertices and add to field object.
virtual MoFEMErrorCode clear_ents_fields_by_bit_ref(const BitRefLevel bit, const BitRefLevel mask, int verb=DEFAULT_VERBOSITY)=0
virtual MoFEMErrorCode add_ents_to_finite_element_by_dim(const EntityHandle entities, const int dim, const std::string name, const bool recursive=true)=0
add entities to finite element
virtual MoFEMErrorCode clear_finite_elements_by_bit_ref(const BitRefLevel bit, const BitRefLevel mask, int verb=DEFAULT_VERBOSITY)=0
virtual MoFEMErrorCode list_dofs_by_field_name(const std::string &name) const =0
virtual MoFEMErrorCode remove_ents_from_field(const std::string name, const EntityHandle meshset, const EntityType type, int verb=DEFAULT_VERBOSITY)=0
remove entities from field
virtual FieldBitNumber get_field_bit_number(const std::string name) const =0
get field bit number
virtual int get_comm_rank() const =0
virtual DofEntityByUId::iterator get_dofs_by_name_end(const std::string &field_name) const =0
get begin iterator of field dofs of given name (instead you can use IT_GET_DOFS_FIELD_BY_NAME_FOR_LOO...
virtual MoFEMErrorCode get_problem_finite_elements_entities(const std::string name, const std::string &fe_name, const EntityHandle meshset)=0
add finite elements to the meshset
std::bitset< BITFIELDID_SIZE > BitFieldId
Field Id.
virtual MoFEMErrorCode remove_ents(const Range ents, int verb=DEFAULT_VERBOSITY)=0
remove entities form mofem database
virtual const Problem * get_problem(const std::string problem_name) const =0
Get the problem object.
virtual MoFEMErrorCode remove_ents_from_field_by_bit_ref(const BitRefLevel bit, const BitRefLevel mask, int verb=DEFAULT_VERBOSITY)=0
remove entities from field
virtual MoFEMErrorCode add_broken_field(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const std::vector< std::pair< EntityType, std::function< MoFEMErrorCode(BaseFunction::DofsSideMap &)>> > list_dof_side_map, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
virtual const FiniteElement_multiIndex * get_finite_elements() const =0
Get the finite elements object.
virtual MoFEMErrorCode get_finite_element_entities_by_type(const std::string name, EntityType type, Range &ents) const =0
get entities in the finite element by type
virtual DofEntityByUId::iterator get_dofs_by_name_and_ent_end(const std::string &field_name, const EntityHandle ent) const =0
get begin iterator of field dofs of given name and ent (instead you can use IT_GET_DOFS_FIELD_BY_NAME...
virtual EntityHandle get_field_meshset(const std::string name) const =0
get field meshset
virtual MoFEMErrorCode modify_finite_element_off_field_data(const std::string &fe_name, const std::string name_field)=0
unset finite element field data
Data structure to exchange data between mofem and User Loop Methods on entities.
virtual MoFEMErrorCode remove_parents_by_parents(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
Remove paremts from entities having parents in passed range.
multi_index_container< boost::shared_ptr< RefEntity >, indexed_by< ordered_unique< tag< Ent_mi_tag >, const_mem_fun< RefEntity, EntityHandle, &RefEntity::getEnt > >, ordered_non_unique< tag< Ent_Ent_mi_tag >, const_mem_fun< RefEntity, EntityHandle, &RefEntity::getParentEnt > >, ordered_non_unique< tag< Composite_EntType_and_ParentEntType_mi_tag >, composite_key< RefEntity, const_mem_fun< RefEntity, EntityType, &RefEntity::getEntType >, const_mem_fun< RefEntity, EntityType, &RefEntity::getParentEntType > > >, ordered_non_unique< tag< Composite_ParentEnt_And_EntType_mi_tag >, composite_key< RefEntity, const_mem_fun< RefEntity, EntityType, &RefEntity::getEntType >, const_mem_fun< RefEntity, EntityHandle, &RefEntity::getParentEnt > > > > > RefEntity_multiIndex
virtual MoFEMErrorCode modify_problem_mask_ref_level_set_bit(const std::string &name_problem, const BitRefLevel &bit)=0
set dof mask ref level for problem
virtual MoFEMErrorCode add_ents_to_field_by_dim(const Range &ents, const int dim, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
Provide data structure for (tensor) field approximation.
virtual bool check_problem(const std::string name)=0
check if problem exist
Deprecated interface functions.
virtual const DofEntity_multiIndex * get_dofs() const =0
Get the dofs object.
DeprecatedCoreInterface Interface
virtual MoFEMErrorCode remove_parents_by_ents(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
Remove parents from entities.
virtual MoFEMErrorCode rebuild_database(int verb=DEFAULT_VERBOSITY)=0
Clear database and initialize it once again.
FieldSpace
approximation spaces
virtual MoFEMErrorCode problem_basic_method_preProcess(const Problem *problem_ptr, BasicMethod &method, int verb=DEFAULT_VERBOSITY)=0
Set data for BasicMethod.
virtual DofEntityByUId::iterator get_dofs_by_name_and_type_begin(const std::string &field_name, const EntityType type) const =0
get begin iterator of field dofs of given name and ent type (instead you can use IT_GET_DOFS_FIELD_BY...
virtual const FiniteElement * get_finite_element_structure(const std::string &name, enum MoFEMTypes bh=MF_EXIST) const =0
get finite element struture
virtual MoFEMErrorCode remove_ents_from_finite_element(const std::string name, const EntityHandle meshset, const EntityType type, int verb=DEFAULT_VERBOSITY)=0
remove entities from given refinement level to finite element database
Deprecated interface functions.
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
virtual BitFieldId get_field_id(const std::string &name) const =0
Get field Id.
virtual moab::Interface & get_moab()=0
virtual MoFEMErrorCode add_ents_to_finite_element_by_type(const EntityHandle entities, const EntityType type, const std::string name, const bool recursive=true)=0
add entities to finite element
virtual MoFEMErrorCode remove_parents_by_bit_ref(const BitRefLevel bit, const BitRefLevel mask, int verb=DEFAULT_VERBOSITY)=0
Remove parent from entities on bit level.
implementation of Data Operators for Forces and Sources
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
virtual MoFEMErrorCode remove_ents_by_bit_ref(const BitRefLevel bit, const BitRefLevel mask, int verb=DEFAULT_VERBOSITY)=0
remove entities form mofem database
virtual ~CoreInterface()=default
int FieldCoefficientsNumber
Number of field coefficients.
virtual MoFEMErrorCode remove_ents_from_finite_element_by_bit_ref(const BitRefLevel bit, const BitRefLevel mask, int verb=DEFAULT_VERBOSITY)=0
remove elements from given refinement level to finite element database
virtual boost::shared_ptr< BasicEntityData > & get_basic_entity_data_ptr()=0
Get pointer to basic entity data.
virtual FieldEntityByUId::iterator get_ent_field_by_name_begin(const std::string &field_name) const =0
get begin iterator of field ents of given name (instead you can use IT_GET_ENT_FIELD_BY_NAME_FOR_LOOP...
virtual MoFEMErrorCode delete_field(const std::string name, int verb=DEFAULT_VERBOSITY)=0
Delete field.
virtual MoFEMErrorCode modify_problem_unset_finite_element(const std::string name_problem, const std::string &fe_name)=0
unset finite element from problem, this remove entities assigned to finite element to a particular pr...
virtual MoFEMErrorCode delete_finite_element(const std::string name, int verb=DEFAULT_VERBOSITY)=0
delete finite element from mofem database
virtual int get_comm_size() const =0
virtual MoFEMErrorCode cache_problem_entities(const std::string prb_name, CacheTupleWeakPtr cache_ptr)=0
Cache variables.
virtual const FieldEntity_multiIndex * get_field_ents() const =0
Get the field ents object.
virtual MoFEMErrorCode get_field_entities_by_handle(const std::string name, Range &ents) const =0
get entities in the field by handle
virtual MoFEMErrorCode clear_ents_fields(const Range ents, int verb=DEFAULT_VERBOSITY)=0
virtual bool check_field(const std::string &name) const =0
check if field is in database
virtual MoFEMErrorCode delete_ents_by_bit_ref(const BitRefLevel bit, const BitRefLevel mask, const bool remove_parent=false, int verb=DEFAULT_VERBOSITY, MoFEMTypes mf=MF_ZERO)=0
delete entities form mofem and moab database
Data structure to exchange data between mofem and User Loop Methods.
virtual MoFEMErrorCode clear_dofs_fields(const Range ents, int verb=DEFAULT_VERBOSITY)=0
virtual const Problem_multiIndex * get_problems() const =0
Get the problems object.
virtual EntFiniteElement_multiIndex::index< Unique_mi_tag >::type::iterator get_fe_by_name_end(const std::string &fe_name) const =0
get end iterator of finite elements of given name (instead you can use IT_GET_FES_BY_NAME_FOR_LOOP(MF...
boost::shared_ptr< CacheTuple > CacheTupleSharedPtr
multi_index_container< boost::shared_ptr< FieldEntity >, indexed_by< ordered_unique< tag< Unique_mi_tag >, member< FieldEntity, UId, &FieldEntity::localUId > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< FieldEntity::interface_type_RefEntity, EntityHandle, &FieldEntity::getEnt > > > > FieldEntity_multiIndex
virtual MoFEMErrorCode modify_finite_element_add_field_data(const std::string &fe_name, const std::string name_field)=0
set finite element field data
Finite element definition.
virtual MoFEMErrorCode modify_finite_element_off_field_col(const std::string &fe_name, const std::string name_row)=0
unset field col which finite element use
virtual MoFEMErrorCode modify_problem_ref_level_add_bit(const std::string &name_problem, const BitRefLevel &bit)=0
add ref level to problem
virtual MeshsetsManager & get_meshsets_manager()=0
get MeshsetsManager pointer
constexpr auto field_name
virtual MoFEMErrorCode clear_problems(int verb=DEFAULT_VERBOSITY)=0
clear problems
multi_index_container< Problem, indexed_by< ordered_unique< tag< Meshset_mi_tag >, member< Problem, EntityHandle, &Problem::meshset > >, hashed_unique< tag< BitProblemId_mi_tag >, const_mem_fun< Problem, BitProblemId, &Problem::getId >, HashBit< BitProblemId >, EqBit< BitProblemId > >, hashed_unique< tag< Problem_mi_tag >, const_mem_fun< Problem, std::string, &Problem::getName > > > > Problem_multiIndex
MultiIndex for entities for Problem.
virtual MoFEMErrorCode get_finite_element_entities_by_dimension(const std::string name, int dim, Range &ents) const =0
get entities in the finite element by dimension
virtual MoFEMErrorCode get_finite_element_entities_by_handle(const std::string name, Range &ents) const =0
get entities in the finite element by handle
virtual MoFEMErrorCode clear_database(int verb=DEFAULT_VERBOSITY)=0
Clear database.
virtual const Field_multiIndex * get_fields() const =0
Get the fields object.
base class for all interface classes
boost::function< MoFEMErrorCode(Interface &moab, const Field &field, const EntFiniteElement &fe, std::vector< EntityHandle > &adjacency)> ElementAdjacencyFunct
user adjacency function
virtual DofEntityByUId::iterator get_dofs_by_name_begin(const std::string &field_name) const =0
get begin iterator of field dofs of given name (instead you can use IT_GET_DOFS_FIELD_BY_NAME_FOR_LOO...
virtual MoFEMErrorCode add_ents_to_finite_element_by_bit_ref(const BitRefLevel bit, const BitRefLevel mask, const std::string name, EntityType type, int verb=DEFAULT_VERBOSITY)=0
add TET entities from given refinement level to finite element database given by name
virtual MoFEMErrorCode clear_finite_elements(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
int ApproximationOrder
Approximation on the entity.
multi_index_container< boost::shared_ptr< Field >, indexed_by< hashed_unique< tag< BitFieldId_mi_tag >, const_mem_fun< Field, const BitFieldId &, &Field::getId >, HashBit< BitFieldId >, EqBit< BitFieldId > >, ordered_unique< tag< Meshset_mi_tag >, member< Field, EntityHandle, &Field::meshSet > >, ordered_unique< tag< FieldName_mi_tag >, const_mem_fun< Field, boost::string_ref, &Field::getNameRef > >, ordered_non_unique< tag< BitFieldId_space_mi_tag >, const_mem_fun< Field, FieldSpace, &Field::getSpace > > > > Field_multiIndex
Field_multiIndex for Field.
virtual MoFEMErrorCode check_number_of_ents_in_ents_field() const =0
check data consistency in entitiesPtr
virtual MoFEMErrorCode clear_inactive_dofs(int verb=DEFAULT_VERBOSITY)=0
virtual EntFiniteElement_multiIndex::index< Unique_mi_tag >::type::iterator get_fe_by_name_begin(const std::string &fe_name) const =0
get begin iterator of finite elements of given name (instead you can use IT_GET_FES_BY_NAME_FOR_LOOP(...
virtual DofEntityByUId::iterator get_dofs_by_name_and_ent_begin(const std::string &field_name, const EntityHandle ent) const =0
get begin iterator of field dofs of given name and ent(instead you can use IT_GET_DOFS_FIELD_BY_NAME_...
virtual MoFEMErrorCode list_problem() const =0
list problems
char FieldBitNumber
Field bit number.
virtual const RefEntity_multiIndex * get_ref_ents() const =0
Get the ref ents object.
virtual const RefElement_multiIndex * get_ref_finite_elements() const =0
Get the ref finite elements object.
Interface for managing meshsets containing materials and boundary conditions.
boost::weak_ptr< CacheTuple > CacheTupleWeakPtr
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
virtual MoFEMErrorCode clear_problem(const std::string name, int verb=DEFAULT_VERBOSITY)=0
clear problem
virtual MoFEMErrorCode modify_finite_element_off_field_row(const std::string &fe_name, const std::string name_row)=0
unset field row which finite element use
FieldApproximationBase
approximation base
MoFEMTypes
Those types control how functions respond on arguments, f.e. error handling.
virtual MoFEMErrorCode clear_dofs_fields_by_bit_ref(const BitRefLevel bit, const BitRefLevel mask, int verb=DEFAULT_VERBOSITY)=0
multi_index_container< boost::shared_ptr< FiniteElement >, indexed_by< hashed_unique< tag< FiniteElement_Meshset_mi_tag >, member< FiniteElement, EntityHandle, &FiniteElement::meshset > >, hashed_unique< tag< BitFEId_mi_tag >, const_mem_fun< FiniteElement, BitFEId, &FiniteElement::getId >, HashBit< BitFEId >, EqBit< BitFEId > >, ordered_unique< tag< FiniteElement_name_mi_tag >, const_mem_fun< FiniteElement, boost::string_ref, &FiniteElement::getNameRef > > > > FiniteElement_multiIndex
MultiIndex for entities for FiniteElement.
virtual MoFEMErrorCode modify_problem_add_finite_element(const std::string name_problem, const std::string &fe_name)=0
add finite element to problem, this add entities assigned to finite element to a particular problem
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
virtual MoFEMErrorCode modify_problem_mask_ref_level_add_bit(const std::string &name_problem, const BitRefLevel &bit)=0
set dof mask ref level for problem
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
virtual MoFEMErrorCode build_field(const std::string field_name, int verb=DEFAULT_VERBOSITY)=0
build field by name
keeps basic data about problem
virtual bool check_finite_element(const std::string &name) const =0
Check if finite element is in database.
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
virtual MoFEMErrorCode get_field_entities_by_dimension(const std::string name, int dim, Range &ents) const =0
get entities in the field by dimension
multi_index_container< boost::shared_ptr< DofEntity >, indexed_by< ordered_unique< tag< Unique_mi_tag >, const_mem_fun< DofEntity, UId, &DofEntity::getLocalUniqueId > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< DofEntity, EntityHandle, &DofEntity::getEnt > > > > DofEntity_multiIndex
MultiIndex container keeps DofEntity.
virtual MoFEMErrorCode list_fields() const =0
list entities in the field
virtual MoFEMErrorCode get_field_entities_by_type(const std::string name, EntityType type, Range &ents) const =0
get entities in the field by type
virtual DofEntityByUId::iterator get_dofs_by_name_and_type_end(const std::string &field_name, const EntityType type) const =0
get begin iterator of field dofs of given name end ent type(instead you can use IT_GET_DOFS_FIELD_BY_...
virtual MoFEMErrorCode add_problem(const std::string &name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add problem.
virtual MoFEMErrorCode delete_problem(const std::string name)=0
Delete problem.
multi_index_container< boost::shared_ptr< EntFiniteElement >, indexed_by< ordered_unique< tag< Unique_mi_tag >, const_mem_fun< EntFiniteElement, UId, &EntFiniteElement::getLocalUniqueId > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< EntFiniteElement::interface_type_RefEntity, EntityHandle, &EntFiniteElement::getEnt > > > > EntFiniteElement_multiIndex
MultiIndex container for EntFiniteElement.
virtual MoFEMErrorCode set_field_order_by_entity_type_and_bit_ref(const BitRefLevel &bit, const BitRefLevel &mask, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
virtual MoFEMErrorCode add_field(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
virtual const int getValue() const =0
Get the core.
virtual MoFEMErrorCode clear_adjacencies_finite_elements(const BitRefLevel bit, const BitRefLevel mask, int verb=DEFAULT_VERBOSITY)=0
clear adjacency map for finite elements on given bit level
virtual MoFEMErrorCode loop_entities(const std::string field_name, EntityMethod &method, Range const *const ents=nullptr, int verb=DEFAULT_VERBOSITY)=0
Loop over field entities.