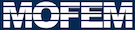 |
| v0.14.0
|
Go to the documentation of this file.
9 #ifndef __MESHSETSMANAGER_HPP__
10 #define __MESHSETSMANAGER_HPP__
34 #define _IT_CUBITMESHSETS_FOR_LOOP_(MESHSET_MANAGER, IT) \
35 CubitMeshSet_multiIndex::iterator IT = \
36 MESHSET_MANAGER.get_meshsets_manager_ptr()->getBegin(); \
37 IT != MESHSET_MANAGER.get_meshsets_manager_ptr()->getEnd(); \
49 #define _IT_CUBITMESHSETS_BY_BCDATA_TYPE_FOR_LOOP_(MESHSET_MANAGER, \
51 CubitMeshsetByType::iterator IT = \
52 MESHSET_MANAGER.get_meshsets_manager_ptr()->getBegin(CUBITBCTYPE); \
53 IT != MESHSET_MANAGER.get_meshsets_manager_ptr()->getEnd(CUBITBCTYPE); \
71 #define _IT_CUBITMESHSETS_BY_SET_TYPE_FOR_LOOP_(MESHSET_MANAGER, CUBITBCTYPE, \
73 CubitMeshsetByMask::iterator IT = \
74 MESHSET_MANAGER.get_meshsets_manager_ptr()->getBySetTypeBegin( \
76 IT != MESHSET_MANAGER.get_meshsets_manager_ptr()->getBySetTypeEnd( \
94 #define _IT_CUBITMESHSETS_BY_NAME_FOR_LOOP_(MESHSET_MANAGER, NAME, IT) \
95 CubitMeshsetByName::iterator IT = \
96 MESHSET_MANAGER.get_meshsets_manager_ptr()->getBegin(NAME); \
97 IT != MESHSET_MANAGER.get_meshsets_manager_ptr()->getEnd(NAME); \
194 template <
class CUBIT_BC_DATA_TYPE>
196 unsigned long int type)
const;
243 inline CubitMeshSet_multiIndex::iterator
getBegin()
const {
257 CubitMeshSet_multiIndex::iterator
getEnd()
const {
275 inline CubitMeshsetByType::iterator
295 inline CubitMeshsetByType::iterator
296 getEnd(
const unsigned int cubit_bc_type)
const {
313 inline CubitMeshsetByMask::iterator
331 inline CubitMeshsetByMask::iterator
351 inline CubitMeshsetByName::iterator
getBegin(
const std::string &name)
const {
369 inline CubitMeshsetByName::iterator
getEnd(
const std::string &name)
const {
391 int *
const number_of_meshsets_ptr = NULL)
const;
404 const std::string name =
"");
414 const int ms_id,
const Range &ents);
438 const std::vector<double> &attributes,
439 const std::string name =
"");
452 const std::string name =
"");
506 std::vector<const CubitMeshSets *> &vec_ptr)
const;
515 std::vector<const CubitMeshSets *>
539 std::vector<const CubitMeshSets *> &vec_ptr)
const;
582 std::vector<const CubitMeshSets *>
608 const unsigned int cubit_bc_type,
609 const int dimension,
Range &entities,
610 const bool recursive =
true)
const;
629 const unsigned int cubit_bc_type,
631 const bool recursive =
true)
const;
656 const int ms_id,
const unsigned int cubit_bc_type,
658 const int operation_type = moab::Interface::INTERSECT);
668 Range &meshsets)
const;
820 const bool clean_file_options =
true);
843 const std::string file_name =
"out_meshset.vtk",
844 const std::string file_type =
"VTK",
845 const std::string options =
"")
const;
859 const int ms_id,
const unsigned int cubit_bc_type,
const int dim,
860 const std::string file_name =
"out_meshset.vtk",
861 const bool recursive =
false,
const std::string file_type =
"VTK",
862 const std::string options =
"")
const;
868 inline boost::shared_ptr<boost::program_options::options_description> &
894 boost::shared_ptr<boost::program_options::options_description>
897 static void sortMeshsets(std::vector<const CubitMeshSets *> &vec_ptr);
900 template <
class CUBIT_BC_DATA_TYPE>
902 unsigned long int type)
const {
907 CHKERR it->getBcDataStructure(data);
908 MOFEM_LOG(
"MeshsetMngWorld", Sev::inform) << *it;
909 MOFEM_LOG(
"MeshsetMngWorld", Sev::inform) << data;
910 MOFEM_LOG(
"MeshsetMngWorld", Sev::inform) <<
"name " << it->getName();
911 for (EntityType
t = MBVERTEX;
t != MBENTITYSET; ++
t) {
913 CHKERR moab.get_number_entities_by_type(it->meshset,
t, nb,
true);
916 <<
"msId " << it->getMeshsetId() <<
" number of "
917 << moab::CN::EntityTypeName(
t) <<
" " << nb;
927 #endif //__MESHSETSMANAGER_HPP__
MoFEMErrorCode getMeshsetsByType(const unsigned int cubit_bc_type, Range &meshsets) const
get all CUBIT meshsets by CUBIT type
static void sortMeshsets(std::vector< const CubitMeshSets * > &vec_ptr)
Generic bc data structure.
MoFEMErrorCode printTemperatureSet() const
print meshsets with temperature boundary conditions data structure
MoFEMErrorCode addEntitiesToMeshset(const CubitBCType cubit_bc_type, const int ms_id, const Range &ents)
add entities to cubit meshset
#define MOFEM_LOG_SEVERITY_SYNC(comm, severity)
Synchronise "SYNC" on curtain severity level.
virtual MPI_Comm & get_comm() const =0
boost::shared_ptr< boost::program_options::options_description > & getConfigFileOptionsPtr()
Get config file options, use with care.
multi_index_container< CubitMeshSets, indexed_by< hashed_unique< tag< Meshset_mi_tag >, member< CubitMeshSets, EntityHandle, &CubitMeshSets::meshset > >, ordered_non_unique< tag< CubitMeshsetType_mi_tag >, const_mem_fun< CubitMeshSets, unsigned long int, &CubitMeshSets::getBcTypeULong > >, ordered_non_unique< tag< CubitMeshsetMaskedType_mi_tag >, const_mem_fun< CubitMeshSets, unsigned long int, &CubitMeshSets::getMaskedBcTypeULong > >, ordered_non_unique< tag< CubitMeshsets_name >, const_mem_fun< CubitMeshSets, std::string, &CubitMeshSets::getName > >, hashed_unique< tag< Composite_Cubit_msId_And_MeshsetType_mi_tag >, composite_key< CubitMeshSets, const_mem_fun< CubitMeshSets, int, &CubitMeshSets::getMeshsetId >, const_mem_fun< CubitMeshSets, unsigned long int, &CubitMeshSets::getMaskedBcTypeULong > > > > > CubitMeshSet_multiIndex
Stores data about meshsets (see CubitMeshSets) storing data about boundary conditions,...
CubitMeshsetByMask::iterator getBySetTypeEnd(const unsigned int cubit_bc_type) const
get end iterator of cubit mehset of given type (instead you can use IT_CUBITMESHSETS_BY_BCDATA_TYPE_F...
MoFEMErrorCode broadcastMeshsets(int verb=DEFAULT_VERBOSITY)
Boradcats meshsets.
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
this struct keeps basic methods for moab meshset about material and boundary conditions
static bool brodcastMeshsets
CubitMeshsetByName::iterator getEnd(const std::string &name) const
get begin iterator of cubit mehset of given type (instead you can use IT_CUBITMESHSETS_BY_SET_TYPE_FO...
CubitMeshsetByType::iterator getBegin(const unsigned int cubit_bc_type) const
get begin iterator of cubit mehset of given type (instead you can use IT_CUBITMESHSETS_BY_BCDATA_TYPE...
Deprecated interface functions.
MoFEMErrorCode getMeshset(const int ms_id, const unsigned int cubit_bc_type, EntityHandle &meshset) const
get meshset from CUBIT Id and CUBIT type
DeprecatedCoreInterface Interface
MeshsetsManager * get_meshsets_manager_ptr()
return pointer to meshset manager
Tag get_ssTag_data() const
get tag handle used to store boundary data on SIDESET
MoFEMErrorCode initialiseDatabaseFromMesh(int verb=DEFAULT_VERBOSITY)
Tag get_nsTag() const
get tag handle used to store "id" of NODESET
MoFEMErrorCode clearMap()
clear multi-index container
#define CHKERR
Inline error check.
MoFEMErrorCode printForceSet() const
print meshsets with force boundary conditions data structure
MoFEMErrorCode saveMeshsetToFile(const int ms_id, const unsigned int cubit_bc_type, const std::string file_name="out_meshset.vtk", const std::string file_type="VTK", const std::string options="") const
save cubit meshset entities on the moab mesh
const MeshsetsManager * get_meshsets_manager_ptr() const
return pointer to meshset manager
virtual moab::Interface & get_moab()=0
CubitMeshSet_multiIndex::iterator getEnd() const
get begin iterator of cubit mehset of given type (instead you can use IT_CUBITMESHSETS_TYPE_FOR_LOOP(...
MoFEMErrorCode updateAllMeshsetsByEntitiesChildren(const BitRefLevel &bit)
Update all blolsets, sidesets and node sets.
CubitMeshsetByMask::iterator getBySetTypeBegin(const unsigned int cubit_bc_type) const
get end iterator of cubit meshset of given type (instead you can use IT_CUBITMESHSETS_BY_BCDATA_TYPE_...
implementation of Data Operators for Forces and Sources
MoFEMErrorCode printHeatFluxSet() const
print meshsets with heat flux boundary conditions data structure
CubitMeshSet_multiIndex::iterator getBegin() const
get begin iterator of cubit mehset of given type (instead you can use IT_CUBITMESHSETS_TYPE_FOR_LOOP(...
Tag get_nsTag_data() const
get tag handle used to store boundary data on NODESET
MoFEMErrorCode getEntitiesByDimension(const int ms_id, const unsigned int cubit_bc_type, const int dimension, Range &entities, const bool recursive=true) const
get entities from CUBIT/meshset of a particular entity dimension
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const
MoFEMErrorCode addMeshset(const CubitBCType cubit_bc_type, const int ms_id, const std::string name="")
add cubit meshset
MoFEMErrorCode setAtributesByDataStructure(const CubitBCType cubit_bc_type, const int ms_id, const GenericAttributeData &data, const std::string name="")
set (material) data structure to cubit meshset
CubitMeshSet_multiIndex::index< CubitMeshsetType_mi_tag >::type CubitMeshsetById
CubitMeshSet_multiIndex cubitMeshsets
cubit meshsets
CubitMeshsetByType::iterator getEnd(const unsigned int cubit_bc_type) const
get begin iterator of cubit mehset of given type (instead you can use IT_CUBITMESHSETS_BY_BCDATA_TYPE...
CubitMeshsetByName::iterator getBegin(const std::string &name) const
get begin iterator of cubit mehset of given type (instead you can use IT_CUBITMESHSETS_BY_SET_TYPE_FO...
constexpr double t
plate stiffness
Generic attribute data structure.
MoFEMErrorCode printMaterialsSet() const
print meshsets with material data structure set on it
Tag get_ssTag() const
get tag handle used to store "id" of SIDESET
MoFEMErrorCode setAtributes(const CubitBCType cubit_bc_type, const int ms_id, const std::vector< double > &attributes, const std::string name="")
set attributes to cubit meshset
MoFEMErrorCode getTags(int verb=-1)
get tags handlers used on meshsets
MoFEMErrorCode deleteMeshset(const CubitBCType cubit_bc_type, const int ms_id, const MoFEMTypes bh=MF_EXIST)
delete cubit meshset
base class for all interface classes
boost::shared_ptr< boost::program_options::options_description > configFileOptionsPtr
config file options
CubitMeshSet_multiIndex::index< CubitMeshsetMaskedType_mi_tag >::type CubitMeshsetByMask
Tag get_bhTag_header() const
get tag handle used to store of block set header (Used by Cubit)
CubitMeshSet_multiIndex & getMeshsetsMultindex()
std::bitset< 32 > CubitBCType
#define MOFEM_LOG(channel, severity)
Log.
MoFEMErrorCode printDisplacementSet() const
print meshsets with displacement boundary conditions data structure
MoFEMErrorCode printBcSet(CUBIT_BC_DATA_TYPE &data, unsigned long int type) const
MoFEMErrorCode printPressureSet() const
print meshsets with pressure boundary conditions data structure
MeshsetsManager(const MoFEM::Core &core)
Tag get_bhTag() const
get tag handle used to store "id" of BLOCKSET
Interface for managing meshsets containing materials and boundary conditions.
MoFEMTypes
Those types control how functions respond on arguments, f.e. error handling.
MoFEMErrorCode setBcData(const CubitBCType cubit_bc_type, const int ms_id, const GenericCubitBcData &data)
set boundary data structure to meshset
CubitMeshSet_multiIndex::index< CubitMeshsetType_mi_tag >::type CubitMeshsetByType
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
MoFEMErrorCode readMeshsets(int verb=DEFAULT_VERBOSITY)
Boradcats meshsets.
MultiIndex Tag for field id.
MoFEMErrorCode getCubitMeshsetPtr(const int ms_id, const CubitBCType cubit_bc_type, const CubitMeshSets **cubit_meshset_ptr) const
get cubit meshset
bool checkMeshset(const int ms_id, const CubitBCType cubit_bc_type) const
check for CUBIT Id and CUBIT type
CubitMeshSet_multiIndex::index< CubitMeshsets_name >::type CubitMeshsetByName
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
bool checkIfMeshsetContainsEntities(const int ms_id, const unsigned int cubit_bc_type, const EntityHandle *entities, int num_entities, const int operation_type=moab::Interface::INTERSECT)
Check if meshset constains entities.
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
virtual ~MeshsetsManager()=default
MoFEMErrorCode setMeshsetFromFile()
get name of config file from line command '-meshsets_config'