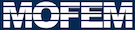 |
| v0.14.0
|
Go to the documentation of this file.
9 #ifndef __MATERIALBLOCKS_HPP__
10 #define __MATERIALBLOCKS_HPP__
27 "It makes no sense for the generic attribute type");
40 "It makes no sense for the generic attribute type");
75 const unsigned int min_number_of_atributes)
110 if (8 * attributes.size() >
sizeof(
data)) {
112 "data inconsistency, please review the number of material "
113 "properties defined");
116 memcpy(&
data, &attributes[0], 8 * attributes.size());
121 if (size >
sizeof(
data)) {
123 "data inconsistency, please review the number of material "
124 "properties defined");
126 memcpy(tag_ptr, &
data, size);
132 friend std::ostream &
operator<<(std::ostream &os,
147 double ThermalExpansion;
169 "Young modulus and/or Poisson ratio is not defined. (top tip: "
170 "check number of ELASTIC block attributes) %d !< %d",
173 if (8 * attributes.size() >
sizeof(
data)) {
175 "data inconsistency, please review the number of material "
176 "properties defined");
179 memcpy(&
data, &attributes[0], 8 * attributes.size());
184 if (size >
sizeof(
data)) {
186 "data inconsistency, please review the number of material "
187 "properties defined");
189 memcpy(tag_ptr, &
data, size);
231 "Thermal conductivity is not defined. (top tip: check number of "
232 "THERMAL block atributes)");
234 if (8 * attributes.size() >
sizeof(
data)) {
236 "data inconsistency, please review the number of material "
237 "properties defined");
240 memcpy(&
data, &attributes[0], 8 * attributes.size());
245 if (size >
sizeof(
data)) {
247 "data inconsistency, please review the number of material "
248 "properties defined");
250 memcpy(tag_ptr, &
data, size);
293 "moisture diffusivity is not defined. (top tip: check number of "
294 "MOISTURE block atributes)");
296 if (8 * attributes.size() >
sizeof(
data)) {
298 "data inconsistency, please review the number of material "
299 "properties defined");
302 memcpy(&
data, &attributes[0], 8 * attributes.size());
320 double acceleration_x;
321 double acceleration_y;
322 double acceleration_z;
340 "Material density and/or acceleration is not defined. (top tip: "
341 "check number of THERMAL block atributes)");
343 if (8 * attributes.size() >
sizeof(
data)) {
345 "data inconsistency, please review the number of material "
346 "properties defined");
349 memcpy(&
data, &attributes[0], 8 * attributes.size());
354 if (size >
sizeof(
data)) {
355 SETERRQ(PETSC_COMM_SELF, 1,
356 "data inconsistency, please review the number of material "
357 "properties defined");
359 memcpy(tag_ptr, &
data, size);
397 "All material data not defined");
399 if (8 * attributes.size() !=
sizeof(
data)) {
401 "data inconsistency, please review the number of material "
402 "properties defined");
404 memcpy(&
data, &attributes[0],
sizeof(
data));
406 memcpy(&
data, &attributes[0], 8 * attributes.size());
412 if (size >
sizeof(
data)) {
414 "data inconsistency, please review the number of material "
415 "properties defined");
417 memcpy(tag_ptr, &
data, size);
423 friend std::ostream &
operator<<(std::ostream &os,
454 if (8 * attributes.size() !=
sizeof(
data)) {
456 "data inconsistency, please review the number of material "
457 "properties defined");
459 memcpy(&
data, &attributes[0],
sizeof(
data));
464 if (size >
sizeof(
data)) {
466 "data inconsistency, please review the number of material "
467 "properties defined");
469 memcpy(tag_ptr, &
data, size);
511 "All material data not defined");
513 if (8 * attributes.size() >
sizeof(
data)) {
515 "data inconsistency, please review the number of material "
516 "properties defined");
519 memcpy(&
data, &attributes[0], 8 * attributes.size());
525 friend std::ostream &
operator<<(std::ostream &os,
531 #endif // __MATERIALBLOCKS_HPP__
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
std::size_t getSizeOfData() const
get data structure size
Body force data structure.
MoFEMErrorCode set_data(void *tag_ptr, unsigned int size) const
set data on structure
struct __attribute__((packed)) _data_
thermal block attributes
const CubitBCType tYpe
Type of data (f.e. MAT_ELATIC)
const void * getDataPtr() const
get pointer to data structure
struct __attribute__((packed)) _data_
Hotzapler soft tissue.
virtual MoFEMErrorCode fill_data(const std::vector< double > &attributes)
get data from structure
MoFEMErrorCode set_data(void *tag_ptr, unsigned int size) const
set data on structure
Mat_Elastic_EberleinHolzapfel1()
Elastic material data structure.
MoFEMErrorCode fill_data(const std::vector< double > &attributes)
get data from structure
std::size_t getSizeOfData() const
get data structure size
MoFEMErrorCode fill_data(const std::vector< double > &attributes)
get data from structure
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
std::size_t getSizeOfData() const
get data structure size
const void * getDataPtr() const
get pointer to data structure
MoFEMErrorCode set_data(void *tag_ptr, unsigned int size) const
set data on structure
std::size_t getSizeOfData() const
get data structure size
Linear interface data structure.
const void * getDataPtr() const
get pointer to data structure
MoFEMErrorCode fill_data(const std::vector< double > &attributes)
get data from structure
const void * getDataPtr() const
get pointer to data structure
Arbitrary block attributes data structure.
implementation of Data Operators for Forces and Sources
const void * getDataPtr() const
get pointer to data structure
std::size_t getSizeOfData() const
get data structure size
@ MAT_THERMALSET
block name is "MAT_THERMAL"
unsigned int minNumberOfAtributes
minimal number of attributes
virtual MoFEMErrorCode set_data(void *tag_ptr, unsigned int size) const
set data on structure
@ BODYFORCESSET
block name is "BODY_FORCES"
Transverse Isotropic material data structure.
moisture transport material data structure
virtual const CubitBCType & getType() const
get data type
friend std::ostream & operator<<(std::ostream &os, const Block_BodyForces &e)
Print Mat_Elastic data.
MoFEMErrorCode fill_data(const std::vector< double > &attributes)
get data from structure
@ MAT_ELASTICSET
block name is "MAT_ELASTIC"
virtual MoFEMErrorCode set_data(void *tag_ptr, unsigned int size) const
set data on structure
struct __attribute__((packed)) _data_
body forces
friend std::ostream & operator<<(std::ostream &os, const Mat_Thermal &e)
Print Mat_Elastic data.
friend std::ostream & operator<<(std::ostream &os, const Mat_Elastic &e)
Print Mat_Elastic data.
struct __attribute__((packed)) _data_
block tag data structute
const void * getDataPtr() const
get pointer to data structure
const void * getDataPtr() const
get pointer to data structure
Generic attribute data structure.
virtual std::size_t getSizeOfData() const =0
get data structure size
GenericAttributeData(const CubitBCType type, const unsigned int min_number_of_atributes)
friend std::ostream & operator<<(std::ostream &os, const Mat_Moisture &e)
Print Mat_Elastic data.
virtual unsigned int getMinMumberOfAtributes() const
get minimal number of attributes which blockset has to have
std::bitset< 32 > CubitBCType
virtual MoFEMErrorCode fill_data(const std::vector< double > &attributes)
get data from structure
friend std::ostream & operator<<(std::ostream &os, const BlockSetAttributes &e)
Print data.
struct __attribute__((packed)) _data_
transverse isotropic
struct __attribute__((packed)) _data_
moisture block attributes
Thermal material data structure.
@ MOFEM_DATA_INCONSISTENCY
struct __attribute__((packed)) _data_
generic block attributes
const void * getDataPtr() const
get pointer to data structure
MoFEMErrorCode set_data(void *tag_ptr, unsigned int size) const
set data on structure
std::size_t getSizeOfData() const
get data structure size
virtual const void * getDataPtr() const =0
get pointer to data structure
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
MoFEMErrorCode fill_data(const std::vector< double > &attributes)
get data from structure
MoFEMErrorCode set_data(void *tag_ptr, unsigned int size) const
set data on structure
friend std::ostream & operator<<(std::ostream &os, const Mat_Elastic_TransIso &e)
Print Mat_Elastic_TransIso data.
@ MAT_MOISTURESET
block name is "MAT_MOISTURE"
MoFEMErrorCode fill_data(const std::vector< double > &attributes)
get data from structure
std::size_t getSizeOfData() const
get data structure size
MoFEMErrorCode fill_data(const std::vector< double > &attributes)
get data from structure
std::size_t getSizeOfData() const
get data structure size
friend std::ostream & operator<<(std::ostream &os, const Mat_Elastic_EberleinHolzapfel1 &e)
Print Mat_Elastic data.
struct __attribute__((packed)) _data_
inteface
friend std::ostream & operator<<(std::ostream &os, const Mat_Interf &e)
Print Mat_Interf data.