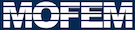 |
| v0.14.0
|
Go to the documentation of this file.
7 #ifndef __LOGMANAGER_HPP__
8 #define __LOGMANAGER_HPP__
10 namespace attrs = boost::log::attributes;
11 namespace logging = boost::log;
12 namespace keywords = boost::log::keywords;
13 namespace logging = boost::log;
14 namespace sinks = boost::log::sinks;
15 namespace src = boost::log::sources;
16 namespace attrs = boost::log::attributes;
17 namespace expr = boost::log::expressions;
37 (
char *)
"noisy", (
char *)
"verbose", (
char *)
"inform", (
char *)
"warning",
62 typedef boost::log::sources::severity_channel_logger<
SeverityLevel,
66 typedef sinks::synchronous_sink<sinks::text_ostream_backend>
SinkType;
129 static void addTag(
const std::string channel,
const std::string tag);
136 static boost::shared_ptr<std::ostream>
getStrmSelf();
150 static boost::shared_ptr<std::ostream>
getStrmSync();
157 static boost::shared_ptr<std::ostream>
getStrmNull();
166 static boost::shared_ptr<SinkType>
167 createSink(boost::shared_ptr<std::ostream> stream_ptr,
168 std::string comm_filter);
231 logging::formatting_ostream &strm);
250 namespace LogKeywords {
258 boost::log::attributes::named_scope::value_type)
260 boost::log::attributes::timer::value_type)
284 #define MOFEM_LOG_CHANNEL(channel) \
285 { MoFEM::LogManager::setLog(channel); }
296 #define MOFEM_LOG_ATTRIBUTES(channel, bit) \
297 { MoFEM::LogManager::addAttributes(channel, bit); }
308 #define MOFEM_LOG(channel, severity) \
309 BOOST_LOG_SEV(MoFEM::LogManager::getLog(channel), severity)
311 #define MOFEM_LOG_C(channel, severity, format, ...) \
312 MOFEM_LOG(channel, severity) \
313 << MoFEM::LogManager::getCLikeFormatedString(format, __VA_ARGS__)
325 #define MOFEM_LOG_FUNCTION() \
326 BOOST_LOG_NAMED_SCOPE_INTERNAL( \
327 BOOST_LOG_UNIQUE_IDENTIFIER_NAME(_boost_log_named_scope_sentry_), \
328 PETSC_FUNCTION_NAME, __FILE__, __LINE__, \
329 ::boost::log::attributes::named_scope_entry::function)
339 #define MOFEM_LOG_TAG(channel, tag) MoFEM::LogManager::addTag(channel, tag);
345 #define MOFEM_LOG_SYNCHRONISE(comm) \
346 PetscSynchronizedFlush(comm, MoFEM::LogManager::dummy_mofem_fd);
352 #define MOFEM_LOG_SEVERITY_SYNC(comm, severity) \
353 MOFEM_LOG("NULL", severity) << ([&] { \
354 MOFEM_LOG_SYNCHRONISE(comm); \
362 #define MOFEM_TAG_AND_LOG(channel, severity, tag) \
363 MOFEM_LOG_TAG(channel, tag) \
364 MOFEM_LOG(channel, severity)
370 #define MOFEM_TAG_AND_LOG_C(channel, severity, tag, format, ...) \
371 MOFEM_LOG_TAG(channel, tag) \
372 MOFEM_LOG_C(channel, severity, format, __VA_ARGS__)
374 #endif //__LOGMANAGER_HPP__
std::ostream & operator<<(std::ostream &os, const EntitiesFieldData::EntData &e)
static bool checkIfChannelExist(const std::string channel)
Check if channel exist.
static LoggerType & getLog(const std::string channel)
Get logger by channel.
static FILE * dummy_mofem_fd
Dummy file pointer (DO NOT USE)
static std::string petscStringCache
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
static void recordFormatterDefault(logging::record_view const &rec, logging::formatting_ostream &strm)
Default record formatter.
static boost::shared_ptr< SinkType > createSink(boost::shared_ptr< std::ostream > stream_ptr, std::string comm_filter)
Create a sink object.
static std::string getVLikeFormatedString(const char *fmt, va_list args)
Converts formatted output to string.
static PetscErrorCode logPetscFPrintf(FILE *fd, const char format[], va_list Argp)
Use to handle PETSc output.
boost::log::sources::severity_channel_logger< SeverityLevel, std::string > LoggerType
Definition of the channel logger.
static constexpr std::array< char *const, error+1 > severityStrings
static std::string getCLikeFormatedString(const char *fmt,...)
Converts formatted output to string.
static boost::shared_ptr< std::ostream > getStrmNull()
Get the strm null object.
implementation of Data Operators for Forces and Sources
BOOST_LOG_ATTRIBUTE_KEYWORD(scope, "Scope", boost::log::attributes::named_scope::value_type) BOOST_LOG_ATTRIBUTE_KEYWORD(timeline
static boost::shared_ptr< std::ostream > getStrmSync()
Get the strm sync object.
static boost::shared_ptr< InternalData > internalDataPtr
static boost::shared_ptr< std::ostream > getStrmWorld()
Get the strm world object.
static boost::shared_ptr< std::ostream > getStrmSelf()
Get the strm self object.
static MoFEMErrorCode getOptions()
Get logger option.
SeverityLevel
Severity levels.
sinks::synchronous_sink< sinks::text_ostream_backend > SinkType
PetscErrorCode PetscVFPrintfDefault(FILE *fd, const char *format, va_list Argp)
base class for all interface classes
static void createDefaultSinks(MPI_Comm comm)
Create default sinks.
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const
static LoggerType & setLog(const std::string channel)
Set ans resset chanel logger.
static void addAttributes(LogManager::LoggerType &lg, const int bit=0)
Add attributes to logger.
static void addTag(LogManager::LoggerType &lg, const std::string tag)
Add tag to logger.
LogAttributesBits
Tag attributes switches.
Log manager is used to build and partition problems.
virtual ~LogManager()=default
LogManager(const MoFEM::Core &core)