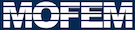 |
| v0.14.0
|
Go to the documentation of this file.
7 #ifndef __MOFEMUNKNOWNINTERFACE_HPP__
8 #define __MOFEMUNKNOWNINTERFACE_HPP__
21 Version(
const int minor,
const int major,
const int build)
25 auto str = [](
auto v) {
return boost::lexical_cast<std::string>(
v); };
53 template <
class IFACE>
58 if (error_if_registration_failed && (!p.second)) {
60 "Registration of interface typeid(IFACE).name() = %s failed",
61 typeid(IFACE).name());
92 template <
class IFACE>
97 if (!(iface =
dynamic_cast<IFACE *
>(ptr)))
99 "Cast impossible %s -> %s",
100 (boost::typeindex::type_id_runtime(*ptr).pretty_name()).c_str(),
101 (boost::typeindex::type_id<IFACE>().pretty_name()).c_str());
132 template <
class IFACE>
134 return getInterface<IFACE>(*iface);
160 template <
class IFACE,
161 typename boost::enable_if<boost::is_pointer<IFACE>,
int>
::type = 0>
164 IFaceType *iface = NULL;
165 ierr = getInterface<IFACE>(iface);
166 CHKERRABORT(PETSC_COMM_SELF,
ierr);
193 template <
class IFACE,
typename boost::enable_if<boost::is_reference<IFACE>,
197 IFaceType *iface = NULL;
198 ierr = getInterface<IFaceType>(iface);
199 CHKERRABORT(PETSC_COMM_SELF,
ierr);
228 ierr = getInterface<IFACE>(iface);
229 CHKERRABORT(PETSC_COMM_SELF,
ierr);
266 Version version =
Version(MoFEM_VERSION_MAJOR, MoFEM_VERSION_MINOR,
267 MoFEM_VERSION_BUILD));
289 typedef multi_index_container<
293 hashed_unique<member<UIdTypeMap, boost::typeindex::type_index,
305 #endif // __MOFEMUNKNOWNINTERFACE_HPP__
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
iFaceTypeMap_multiIndex iFaceTypeMap
Maps implementation to interface type name.
virtual MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const =0
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
MoFEMErrorCode registerInterface(bool error_if_registration_failed=true)
Register interface.
static MoFEMErrorCode getFileVersion(moab::Interface &moab, Version &version)
Get database major version.
UIdTypeMap(const boost::typeindex::type_index &idx)
MoFEMErrorCode getInterface(IFACE **const iface) const
Get interface pointer to pointer of interface.
DeprecatedCoreInterface Interface
Version(const int minor, const int major, const int build)
#define CHKERR
Inline error check.
static MoFEMErrorCode setFileVersion(moab::Interface &moab, Version version=Version(MoFEM_VERSION_MAJOR, MoFEM_VERSION_MINOR, MoFEM_VERSION_BUILD))
Get database major version.
implementation of Data Operators for Forces and Sources
multi_index_container< UIdTypeMap, indexed_by< hashed_unique< member< UIdTypeMap, boost::typeindex::type_index, &UIdTypeMap::classIdx > > > > iFaceTypeMap_multiIndex
Data structure for interfaces Id and class names.
virtual ~UnknownInterface()=default
@ MOFEM_OPERATION_UNSUCCESSFUL
static MoFEMErrorCode getInterfaceVersion(Version &version)
Get database major version.
boost::typeindex::type_index classIdx
base class for all interface classes
const double v
phase velocity of light in medium (cm/ns)
IFACE * getInterface() const
Function returning pointer to interface.
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
static MoFEMErrorCode getLibVersion(Version &version)
Get library version.
IFACE getInterface() const
Get interface pointer to pointer of interface.