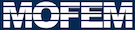 |
| v0.14.0
|
Go to the documentation of this file.
9 #ifndef __BCMULTIINDICES_HPP__
10 #define __BCMULTIINDICES_HPP__
110 const bool recursive =
false)
const;
125 const bool recursive =
false)
const;
136 const EntityType
type,
138 const bool recursive =
false)
const;
214 const std::vector<double> &attributes);
267 template <
class ATTRIBUTE_TYPE>
272 "attributes are not for ATTRIBUTE_TYPE structure");
274 std::vector<double> attributes;
276 CHKERR data.fill_data(attributes);
283 template <
class ATTRIBUTE_TYPE>
288 "attributes are not for ATTRIBUTE_TYPE structure");
295 template <
class CUBIT_BC_DATA_TYPE>
301 "bc_data are not for CUBIT_BC_DATA_TYPE structure");
303 std::vector<char> bc_data;
305 ierr = data.fill_data(bc_data);
310 template <
class CUBIT_BC_DATA_TYPE>
314 char *ptr =
const_cast<char *
>(
tagBcData);
367 typedef multi_index_container<
372 ordered_non_unique<tag<CubitMeshsetType_mi_tag>,
375 ordered_non_unique<tag<CubitMeshsetMaskedType_mi_tag>,
379 tag<CubitMeshsets_name>,
380 const_mem_fun<CubitMeshSets, std::string, &CubitMeshSets::getName>>,
382 tag<Composite_Cubit_msId_And_MeshsetType_mi_tag>,
385 const_mem_fun<CubitMeshSets, int, &CubitMeshSets::getMeshsetId>,
415 const std::vector<double> &
aTtr;
417 const std::vector<double> &attr)
448 #endif // __BCMULTIINDICES_HPP__
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
DEPRECATED unsigned long int getMaksedBcTypeULong() const
CubitMeshSets(Interface &moab, const EntityHandle meshset)
Generic bc data structure.
int * msId
cubit meshset ID
CubitMeshSets_change_attributes_data_structure(Interface &moab, const GenericAttributeData &attr)
MoFEMErrorCode printAttributes(std::ostream &os) const
print the attributes vector
multi_index_container< CubitMeshSets, indexed_by< hashed_unique< tag< Meshset_mi_tag >, member< CubitMeshSets, EntityHandle, &CubitMeshSets::meshset > >, ordered_non_unique< tag< CubitMeshsetType_mi_tag >, const_mem_fun< CubitMeshSets, unsigned long int, &CubitMeshSets::getBcTypeULong > >, ordered_non_unique< tag< CubitMeshsetMaskedType_mi_tag >, const_mem_fun< CubitMeshSets, unsigned long int, &CubitMeshSets::getMaskedBcTypeULong > >, ordered_non_unique< tag< CubitMeshsets_name >, const_mem_fun< CubitMeshSets, std::string, &CubitMeshSets::getName > >, hashed_unique< tag< Composite_Cubit_msId_And_MeshsetType_mi_tag >, composite_key< CubitMeshSets, const_mem_fun< CubitMeshSets, int, &CubitMeshSets::getMeshsetId >, const_mem_fun< CubitMeshSets, unsigned long int, &CubitMeshSets::getMaskedBcTypeULong > > > > > CubitMeshSet_multiIndex
Stores data about meshsets (see CubitMeshSets) storing data about boundary conditions,...
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
this struct keeps basic methods for moab meshset about material and boundary conditions
friend std::ostream & operator<<(std::ostream &os, const CubitMeshSets &e)
MoFEMErrorCode printBlockHeaderData(std::ostream &os) const
print material_data int stream given by os
double * tagBlockAttributes
MoFEMErrorCode getMeshsetIdEntitiesByType(Interface &moab, const EntityType type, Range &entities, const bool recursive=false) const
get entities by type
CubitMeshSets_change_add_bit_to_cubit_bc_type(const CubitBCType &bit)
void operator()(CubitMeshSets &e)
unsigned long int getMaskedBcTypeULong() const
get meshset type and mask
void operator()(CubitMeshSets &e)
const std::vector< double > & aTtr
MoFEMErrorCode setAttributes(moab::Interface &moab, const std::vector< double > &attributes)
cet Cubit block attributes
MoFEMErrorCode getBcDataStructure(CUBIT_BC_DATA_TYPE &data) const
unsigned int getMeshsetEntitiesDimension() const
Get the meshset entities dimension.
MoFEMErrorCode getTagsHandlers(Interface &moab)
Deprecated interface functions.
DeprecatedCoreInterface Interface
void operator()(CubitMeshSets &e)
std::vector< Tag > tag_handles
vector of tag handles to types of data passed from cubit
std::string getName() const
get name of block, sideset etc. (this is set in Cubit block properties)
#define CHKERR
Inline error check.
CubitBCType getBcType() const
get type of meshset
implementation of Data Operators for Forces and Sources
MoFEMErrorCode setBcDataStructure(CUBIT_BC_DATA_TYPE &data)
MoFEMErrorCode printName(std::ostream &os) const
print name of block, sideset etc. (this is set in Cubit setting properties)
MoFEMErrorCode getAttributeDataStructure(ATTRIBUTE_TYPE &data) const
fill data structure with data saved on meshset
MoFEMErrorCode setAttributeDataStructure(const ATTRIBUTE_TYPE &data)
fill meshset data with data on structure
CubitMeshSets_change_attributes(Interface &moab, const std::vector< double > &attr)
CubitMeshSets_change_name(Interface &moab, const std::string &name)
void operator()(CubitMeshSets &e)
Generic attribute data structure.
int tagBlockAttributesSize
const GenericCubitBcData & bcData
const CubitBCType meshsetsMask
CubitMeshSets_change_bc_data_structure(Interface &moab, const GenericCubitBcData &bc_data)
unsigned int * tagBlockHeaderData
std::bitset< 32 > CubitBCType
MoFEMErrorCode getAttributes(std::vector< double > &attributes) const
get Cubit block attributes
EntityHandle getMeshset() const
get bc meshset
int getMeshsetId() const
get meshset id as it set in preprocessing software
MoFEMErrorCode getBcData(std::vector< char > &bc_data) const
get bc_data vector from MoFEM database
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
@ MOFEM_DATA_INCONSISTENCY
MoFEMErrorCode getTypeFromName(const std::string &name, CubitBCType &type) const
Function that returns the CubitBCType type of the block name, sideset name etc.
const GenericAttributeData & aTtr
void operator()(CubitMeshSets &e)
MoFEMErrorCode getTypeFromBcData(const std::vector< char > &bc_data, CubitBCType &type) const
Function that returns the CubitBCType type of the contents of bc_data.
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
MoFEMErrorCode getMeshsetIdEntitiesByDimension(Interface &moab, const int dimension, Range &entities, const bool recursive=false) const
get entities form meshset
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode getBlockHeaderData(std::vector< unsigned int > &material_data) const
get block_headers vector from MoFEM database
unsigned long int getBcTypeULong() const
get bc meshset type
#define CHKERRG(n)
Check error code of MoFEM/MOAB/PETSc function.
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
MoFEMErrorCode printBcData(std::ostream &os) const
print bc_data int stream given by os