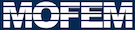 |
| v0.14.0
|
Go to the documentation of this file.
6 #ifndef __PROBLEMSMULTIINDICES_HPP__
7 #define __PROBLEMSMULTIINDICES_HPP__
21 std::vector<SmartPetscObj<IS>>
rowIs;
22 std::vector<SmartPetscObj<IS>>
colIs;
72 mutable boost::shared_ptr<NumeredDofEntity_multiIndex>
74 mutable boost::shared_ptr<NumeredDofEntity_multiIndex>
76 mutable boost::shared_ptr<NumeredEntFiniteElement_multiIndex>
119 inline boost::shared_ptr<SubProblemData> &
getSubData()
const {
149 const int field_bit_number,
const EntityHandle ent,
const int ent_dof_idx,
151 boost::shared_ptr<NumeredDofEntity> &dof_ptr)
const;
164 #define _IT_NUMEREDDOF_ROW_FOR_LOOP_(PROBLEMPTR, IT) \
165 NumeredDofEntity_multiIndex::iterator IT = \
166 PROBLEMPTR->getNumeredRowDofsBegin(); \
167 IT != PROBLEMPTR->getNumeredRowDofsEnd(); \
181 #define _IT_NUMEREDDOF_COL_FOR_LOOP_(PROBLEMPTR, IT) \
182 NumeredDofEntity_multiIndex::iterator IT = \
183 PROBLEMPTR->getNumeredColDofsBegin(); \
184 IT != PROBLEMPTR->getNumeredColDofsEnd(); \
222 #define _IT_NUMEREDDOF_ROW_BY_LOCIDX_FOR_LOOP_(PROBLEMPTR, IT) \
223 NumeredDofEntityByLocalIdx::iterator IT = \
224 PROBLEMPTR->getNumeredRowDofsByLocIdxBegin(0); \
225 IT != PROBLEMPTR->getNumeredRowDofsByLocIdxEnd( \
226 PROBLEMPTR->getNbLocalDofsRow() - 1); \
239 #define _IT_NUMEREDDOF_COL_BY_LOCIDX_FOR_LOOP_(PROBLEMPTR, IT) \
240 NumeredDofEntityByUId::iterator IT = \
241 PROBLEMPTR->getNumeredColDofsByLocIdxBegin(0); \
242 IT != PROBLEMPTR->getNumeredColDofsByLocIdxEnd( \
243 PROBLEMPTR->getNbLocalDofsRow() - 1); \
281 #define _IT_NUMEREDDOF_ROW_BY_ENT_FOR_LOOP_(PROBLEMPTR, ENT, IT) \
282 auto IT = PROBLEMPTR->getNumeredRowDofsByEntBegin(ENT); \
283 IT != PROBLEMPTR->getNumeredRowDofsByEntEnd(ENT); \
297 #define _IT_NUMEREDDOF_COL_BY_ENT_FOR_LOOP_(PROBLEMPTR, ENT, IT) \
298 auto IT = PROBLEMPTR->getNumeredColDofsByEntBegin(ENT); \
299 IT != PROBLEMPTR->getNumeredColDofsByEntEnd(ENT); \
338 #define _IT_NUMEREDDOF_ROW_BY_BITNUMBER_FOR_LOOP_(PROBLEMPTR, \
339 FIELD_BIT_NUMBER, IT) \
340 auto IT = PROBLEMPTR->numeredRowDofsPtr->lower_bound( \
341 FieldEntity::getLoBitNumberUId(FIELD_BIT_NUMBER)); \
342 IT != PROBLEMPTR->numeredRowDofsPtr->upper_bound( \
343 FieldEntity::getHiBitNumberUId(FIELD_BIT_NUMBER)); \
358 #define _IT_NUMEREDDOF_COL_BY_BITNUMBER_FOR_LOOP_(PROBLEMPTR, \
359 FIELD_BIT_NUMBER, IT) \
360 auto IT = PROBLEMPTR->numeredColDofsPtr->lower_bound( \
361 FieldEntity::getLoBitNumberUId(FIELD_BIT_NUMBER)); \
362 IT != PROBLEMPTR->numeredColDofsPtr->upper_bound( \
363 FieldEntity::getHiBitNumberUId(FIELD_BIT_NUMBER)); \
420 boost::weak_ptr<NumeredDofEntity>
429 boost::weak_ptr<NumeredDofEntity>
464 const std::string name,
465 PetscLayout *layout)
const;
490 PetscLayout *layout)
const;
492 typedef multi_index_container<boost::weak_ptr<std::vector<NumeredDofEntity>>,
493 indexed_by<sequenced<>>>
596 PetscObjectReference((PetscObject)(*is));
608 PetscObjectReference((PetscObject)(*is));
620 PetscObjectReference((PetscObject)(*ao));
632 PetscObjectReference((PetscObject)(*ao));
647 typedef multi_index_container<
650 ordered_unique<tag<Meshset_mi_tag>,
651 member<Problem, EntityHandle, &Problem::meshset>>,
652 hashed_unique<tag<BitProblemId_mi_tag>,
653 const_mem_fun<Problem, BitProblemId, &Problem::getId>,
655 hashed_unique<tag<Problem_mi_tag>,
656 const_mem_fun<Problem, std::string, &Problem::getName>>>>
751 if (pp <=
rowIs.size()) {
755 *is =
rowIs[pp].get();
756 PetscObjectReference((PetscObject)(*is));
763 if (pp <=
colIs.size()) {
767 *is =
colIs[pp].get();
768 PetscObjectReference((PetscObject)(*is));
774 #endif //__PROBLEMSMULTIINDICES_HPP__
void operator()(Problem &e)
void operator()(Problem &p)
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
std::vector< SmartPetscObj< IS > > rowIs
const auto & getNumeredFiniteElementsPtr() const
get access to reference for multi-index storing finite elements
BitRefLevel * tagBitRefLevel
BitRef level of finite elements in problem.
DofIdx nbGhostDofsRow
Number of ghost DOFs in row.
friend std::ostream & operator<<(std::ostream &os, const Problem &e)
auto getNumeredRowDofsByEntBegin(const EntityHandle ent) const
MoFEMErrorCode getNumberOfElementsByNameAndPart(MPI_Comm comm, const std::string name, PetscLayout *layout) const
Get number of finite elements by name on processors.
SmartPetscObj< AO > colMap
mapping form main problem indices to sub-problem indices
DofIdx getNbDofsCol() const
NumeredDofEntity_multiIndex::iterator getNumeredRowDofsBegin() const
set prof dof bit ref mask
ProblemFiniteElementChangeBitUnSet(const BitFEId _f_id)
MoFEMErrorCode eraseElements(Range entities) const
Erase elements by entities.
void operator()(Problem &e)
std::bitset< BITPROBLEMID_SIZE > BitProblemId
Problem Id.
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
SmartPetscObj< AO > rowMap
mapping form main problem indices to sub-problem indices
ProblemChangeRefLevelBitAdd(const BitRefLevel _bit)
void operator()(Problem &e)
BitFEId getBitFEId() const
Get the BitFEIDs in problem
add finite element to problem
auto getNumeredColDofsByEntBegin(const EntityHandle ent) const
BitProblemId getId() const
BitFEId * tagBitFEId
IDs of finite elements in problem.
auto & getRowDofsSequence() const
Get reference to sequence data numbered dof container.
DofIdx getNbGhostDofsCol() const
DofIdx getNbGhostDofsRow() const
SmartPetscObj< IS > colIs
indices of main problem of which sub problem is this
int tagNameSize
Size of problem name.
auto getNumeredRowDofsByLocIdxBegin(const DofIdx locidx) const
std::bitset< BITFEID_SIZE > BitFEId
Finite element Id.
NumeredDofEntity_multiIndex::iterator getNumeredRowDofsEnd() const
ProblemChangeRefLevelBitDofMaskAdd(const BitRefLevel _bit)
std::pair< BitFieldId, BitFieldId > BlockFieldPair
std::vector< const Problem * > colProblemsAdd
MoFEMErrorCode getColMap(AO *ao)
DeprecatedCoreInterface Interface
void operator()(Problem &e)
ProblemChangeRefLevelBitSet(const BitRefLevel _bit)
boost::shared_ptr< ComposedProblemsData > composedProblemsData
void operator()(Problem &p)
keeps information about indexed dofs for the problem
implementation of Data Operators for Forces and Sources
multi_index_container< boost::weak_ptr< std::vector< NumeredDofEntity > >, indexed_by< sequenced<> > > SequenceDofContainer
clear problem finite elements
ProblemChangeRefLevelBitDofMaskSet(const BitRefLevel _bit)
boost::shared_ptr< NumeredDofEntity_multiIndex > numeredColDofsPtr
store DOFs on columns for this problem
std::vector< SmartPetscObj< IS > > colIs
BlockFieldPair & addFieldToEmptyFieldBlocks(const BlockFieldPair add_fields) const
Add fields to the empty field blocks.
DofIdx getNbDofsRow() const
void operator()(Problem &p)
SmartPetscObj< IS > rowIs
indices of main problem of which sub problem is this
boost::shared_ptr< SubProblemData > & getSubData() const
Get main problem of sub-problem is.
ProblemFiniteElementChangeBitAdd(const BitFEId _f_id)
DofIdx nbDofsCol
Global number of DOFs in col.
std::vector< BlockFieldPair > EmptyFieldBlocks
auto getNumeredRowDofsByEntEnd(const EntityHandle ent) const
EmptyFieldBlocks & getEmptyFieldBlocks() const
Get the empty field blocks.
auto & getNumeredRowDofsPtr() const
get access to numeredRowDofsPtr storing DOFs on rows
MoFEMErrorCode getColDofsByPetscGlobalDofIdx(DofIdx idx, const NumeredDofEntity **dof_ptr, MoFEMTypes bh=MF_EXIST) const
Get the Col Dofs By Petsc Global Dof Idx object.
Clear composed problem data structure.
auto & getComposedProblemsData() const
Het composed problems data structure.
auto & getColDofsSequence() const
Get reference to sequence data numbered dof container.
Clear sub-problem data structure.
Problem::BlockFieldPair BlockFieldPair
virtual ~Problem()=default
DofIdx nbLocDofsRow
Local number of DOFs in row.
const char * tagName
Problem name.
BitProblemId * tagId
Unique problem ID.
DofIdx getNbLocalDofsRow() const
MoFEMErrorCode getColIs(IS *is)
boost::shared_ptr< NumeredEntFiniteElement_multiIndex > numeredFiniteElementsPtr
store finite elements
set prof dof bit ref mask
DofIdx nbGhostDofsCol
Number of ghost DOFs in col.
MoFEMErrorCode getRowDofsByPetscGlobalDofIdx(DofIdx idx, const NumeredDofEntity **dof_ptr, MoFEMTypes bh=MF_EXIST) const
Get the Row Dofs By Petsc Global Dof Idx object.
auto getNumeredColDofsByLocIdxBegin(const DofIdx locidx) const
multi_index_container< Problem, indexed_by< ordered_unique< tag< Meshset_mi_tag >, member< Problem, EntityHandle, &Problem::meshset > >, hashed_unique< tag< BitProblemId_mi_tag >, const_mem_fun< Problem, BitProblemId, &Problem::getId >, HashBit< BitProblemId >, EqBit< BitProblemId > >, hashed_unique< tag< Problem_mi_tag >, const_mem_fun< Problem, std::string, &Problem::getName > > > > Problem_multiIndex
MultiIndex for entities for Problem.
MoFEMErrorCode getColIs(IS *is, const unsigned int pp) const
Get the Col sub dm IS object.
void operator()(Problem &p)
boost::shared_ptr< NumeredDofEntity_multiIndex > numeredRowDofsPtr
store DOFs on rows for this problem
remove finite element from problem
std::vector< const Problem * > rowProblemsAdd
DofIdx nbLocDofsCol
Local number of DOFs in colIs.
boost::shared_ptr< SequenceDofContainer > sequenceRowDofContainer
NumeredDofEntity_multiIndex::iterator getNumeredColDofsBegin() const
auto getNumeredColDofsByLocIdxEnd(const DofIdx locidx) const
boost::shared_ptr< SequenceDofContainer > sequenceColDofContainer
MoFEMErrorCode getNumberOfElementsByPart(MPI_Comm comm, PetscLayout *layout) const
Get number of finite elements on processors.
void operator()(Problem &p)
void operator()(Problem &e)
DofIdx nbDofsRow
Global number of DOFs in row.
BitRefLevel getBitRefLevelMask() const
auto getNumeredRowDofsByLocIdxEnd(const DofIdx locidx) const
BitRefLevel getBitRefLevel() const
MoFEMTypes
Those types control how functions respond on arguments, f.e. error handling.
DofIdx getNbLocalDofsCol() const
MoFEMErrorCode getRowIs(IS *is, const unsigned int pp) const
Get the col sub dm IS.
auto & getNumeredColDofsPtr() const
get access to numeredColDofsPtr storing DOFs on cols
MoFEMErrorCode getRowMap(AO *ao)
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
MoFEMErrorCode getRowIs(IS *is)
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
keeps basic data about problem
NumeredDofEntity_multiIndex::iterator getNumeredColDofsEnd() const
EmptyFieldBlocks emptyFieldBlocks
MoFEMErrorCode getDofByNameEntAndEntDofIdx(const int field_bit_number, const EntityHandle ent, const int ent_dof_idx, const RowColData row_or_col, boost::shared_ptr< NumeredDofEntity > &dof_ptr) const
get DOFs from problem
Problem(Interface &moab, const EntityHandle meshset)
Problem::EmptyFieldBlocks EmptyFieldBlocks
virtual ~SubProblemData()=default
void operator()(Problem &p)
auto getNumeredColDofsByEntEnd(const EntityHandle ent) const
BitRefLevel * tagBitRefLevelMask
BItRefMask of elements in problem.
boost::shared_ptr< SubProblemData > subProblemData
virtual ~ComposedProblemsData()=default