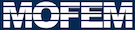 |
| v0.14.0
|
Go to the documentation of this file.
16 MOAB_THROW(moab.tag_get_handle(
"_ProblemId", th_ProblemId));
18 moab.tag_get_by_ptr(th_ProblemId, &
meshset, 1, (
const void **)&
tagId));
20 MOAB_THROW(moab.tag_get_handle(
"_ProblemName", th_ProblemName));
24 MOAB_THROW(moab.tag_get_handle(
"_ProblemFEId", th_ProblemFEId));
28 MOAB_THROW(moab.tag_get_handle(
"_RefBitLevel", th_RefBitLevel));
31 Tag th_RefBitLevel_Mask;
32 MOAB_THROW(moab.tag_get_handle(
"_RefBitLevelMask", th_RefBitLevel_Mask));
38 os <<
"Problem id " << e.
getId() <<
" name " << e.
getName() << endl;
39 os <<
"FiniteElement id " << e.
getBitFEId() << endl;
47 boost::weak_ptr<NumeredDofEntity>
50 boost::weak_ptr<NumeredDofEntity> dof_weak_ptr;
51 NumeredDofEntity_multiIndex::index<PetscGlobalIdx_mi_tag>::type::iterator dit;
58 boost::weak_ptr<NumeredDofEntity>
61 boost::weak_ptr<NumeredDofEntity> dof_weak_ptr;
62 NumeredDofEntity_multiIndex::index<PetscGlobalIdx_mi_tag>::type::iterator dit;
73 if (
auto shared_dof_ptr = weak_dof_ptr.lock()) {
74 *dof_ptr = shared_dof_ptr.get();
77 SETERRQ1(PETSC_COMM_SELF,
MOFEM_NOT_FOUND,
"row dof <%d> not found", idx);
87 if (
auto shared_dof_ptr = weak_dof_ptr.lock()) {
88 *dof_ptr = shared_dof_ptr.get();
91 SETERRQ1(PETSC_COMM_SELF,
MOFEM_NOT_FOUND,
"row dof <%d> not found", idx);
99 PetscLayout *layout)
const {
102 MPI_Comm_size(comm, &size);
103 MPI_Comm_rank(comm, &rank);
104 CHKERR PetscLayoutCreate(comm, layout);
105 CHKERR PetscLayoutSetBlockSize(*layout, 1);
106 const auto &fe_by_name_and_part =
109 nb_elems = fe_by_name_and_part.count(boost::make_tuple(name, rank));
110 CHKERR PetscLayoutSetLocalSize(*layout, nb_elems);
111 CHKERR PetscLayoutSetUp(*layout);
116 PetscLayout *layout)
const {
119 MPI_Comm_size(comm, &size);
120 MPI_Comm_rank(comm, &rank);
121 CHKERR PetscLayoutCreate(comm, layout);
122 CHKERR PetscLayoutSetBlockSize(*layout, 1);
126 nb_elems = fe_by_part.count(rank);
127 CHKERR PetscLayoutSetLocalSize(*layout, nb_elems);
128 CHKERR PetscLayoutSetUp(*layout);
133 const int field_bit_number,
const EntityHandle ent,
const int ent_dof_idx,
135 boost::shared_ptr<NumeredDofEntity> &dof_ptr)
const {
138 switch (row_or_col) {
142 "Row numbered index in problem not allocated");
149 "Col numbered index in problem not allocated");
155 "Only ROW and COL is possible for 3rd argument");
165 dof_ptr = boost::shared_ptr<NumeredDofEntity>();
172 for (
auto p = entities.pair_begin(); p != entities.pair_end(); ++p) {
void operator()(Problem &e)
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
std::ostream & operator<<(std::ostream &os, const EntitiesFieldData::EntData &e)
BitRefLevel * tagBitRefLevel
BitRef level of finite elements in problem.
DofIdx nbGhostDofsRow
Number of ghost DOFs in row.
MoFEMErrorCode getNumberOfElementsByNameAndPart(MPI_Comm comm, const std::string name, PetscLayout *layout) const
Get number of finite elements by name on processors.
MoFEMErrorCode eraseElements(Range entities) const
Erase elements by entities.
void operator()(Problem &e)
static UId getUniqueIdCalculate(const DofIdx dof, UId ent_uid)
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
void operator()(Problem &e)
BitFEId getBitFEId() const
BitProblemId getId() const
BitFEId * tagBitFEId
IDs of finite elements in problem.
#define MOAB_THROW(err)
Check error code of MoAB function and throw MoFEM exception.
int tagNameSize
Size of problem name.
std::bitset< BITFEID_SIZE > BitFEId
Finite element Id.
DeprecatedCoreInterface Interface
multi_index_container< boost::shared_ptr< NumeredDofEntity >, indexed_by< ordered_unique< tag< Unique_mi_tag >, const_mem_fun< NumeredDofEntity::interface_type_DofEntity, UId, &NumeredDofEntity::getLocalUniqueId > >, ordered_non_unique< tag< Part_mi_tag >, member< NumeredDofEntity, unsigned int, &NumeredDofEntity::pArt > >, ordered_non_unique< tag< Idx_mi_tag >, member< NumeredDofEntity, DofIdx, &NumeredDofEntity::dofIdx > >, ordered_non_unique< tag< PetscGlobalIdx_mi_tag >, member< NumeredDofEntity, DofIdx, &NumeredDofEntity::petscGloablDofIdx > >, ordered_non_unique< tag< PetscLocalIdx_mi_tag >, member< NumeredDofEntity, DofIdx, &NumeredDofEntity::petscLocalDofIdx > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< NumeredDofEntity::interface_type_DofEntity, EntityHandle, &NumeredDofEntity::getEnt > > > > NumeredDofEntity_multiIndex
MultiIndex container keeps NumeredDofEntity.
#define CHKERR
Inline error check.
void operator()(Problem &p)
keeps information about indexed dofs for the problem
implementation of Data Operators for Forces and Sources
multi_index_container< boost::weak_ptr< std::vector< NumeredDofEntity > >, indexed_by< sequenced<> > > SequenceDofContainer
boost::shared_ptr< NumeredDofEntity_multiIndex > numeredColDofsPtr
store DOFs on columns for this problem
UId getLocalUniqueIdCalculate()
Get the Local Unique Id Calculate object.
DofIdx nbDofsCol
Global number of DOFs in col.
MoFEMErrorCode getColDofsByPetscGlobalDofIdx(DofIdx idx, const NumeredDofEntity **dof_ptr, MoFEMTypes bh=MF_EXIST) const
Get the Col Dofs By Petsc Global Dof Idx object.
DofIdx nbLocDofsRow
Local number of DOFs in row.
const char * tagName
Problem name.
BitProblemId * tagId
Unique problem ID.
boost::shared_ptr< NumeredEntFiniteElement_multiIndex > numeredFiniteElementsPtr
store finite elements
multi_index_container< boost::shared_ptr< NumeredEntFiniteElement >, indexed_by< ordered_unique< tag< Unique_mi_tag >, const_mem_fun< NumeredEntFiniteElement::interface_type_EntFiniteElement, UId, &NumeredEntFiniteElement::getLocalUniqueId > >, ordered_non_unique< tag< Part_mi_tag >, member< NumeredEntFiniteElement, unsigned int, &NumeredEntFiniteElement::part > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< NumeredEntFiniteElement::interface_type_RefEntity, EntityHandle, &NumeredEntFiniteElement::getEnt > >, ordered_non_unique< tag< Composite_Name_And_Part_mi_tag >, composite_key< NumeredEntFiniteElement, const_mem_fun< NumeredEntFiniteElement::interface_type_FiniteElement, boost::string_ref, &NumeredEntFiniteElement::getNameRef >, member< NumeredEntFiniteElement, unsigned int, &NumeredEntFiniteElement::part > >> >> NumeredEntFiniteElement_multiIndex
MultiIndex for entities for NumeredEntFiniteElement.
DofIdx nbGhostDofsCol
Number of ghost DOFs in col.
MoFEMErrorCode getRowDofsByPetscGlobalDofIdx(DofIdx idx, const NumeredDofEntity **dof_ptr, MoFEMTypes bh=MF_EXIST) const
Get the Row Dofs By Petsc Global Dof Idx object.
boost::shared_ptr< NumeredDofEntity_multiIndex > numeredRowDofsPtr
store DOFs on rows for this problem
DofIdx nbLocDofsCol
Local number of DOFs in colIs.
MoFEMErrorCode getNumberOfElementsByPart(MPI_Comm comm, PetscLayout *layout) const
Get number of finite elements on processors.
void operator()(Problem &p)
DofIdx nbDofsRow
Global number of DOFs in row.
BitRefLevel getBitRefLevelMask() const
BitRefLevel getBitRefLevel() const
MoFEMTypes
Those types control how functions respond on arguments, f.e. error handling.
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
keeps basic data about problem
MoFEMErrorCode getDofByNameEntAndEntDofIdx(const int field_bit_number, const EntityHandle ent, const int ent_dof_idx, const RowColData row_or_col, boost::shared_ptr< NumeredDofEntity > &dof_ptr) const
get DOFs from problem
Problem(Interface &moab, const EntityHandle meshset)
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
BitRefLevel * tagBitRefLevelMask
BItRefMask of elements in problem.
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...