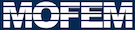 |
| v0.14.0
|
Go to the documentation of this file.
7 #ifndef __DOFSMULTIINDICES_HPP__
8 #define __DOFSMULTIINDICES_HPP__
26 const boost::shared_ptr<FieldEntity> &ent_ptr) {
28 (
static_cast<ShortId>(ent_ptr->getBitNumber()) << 9);
31 DofEntity(
const boost::shared_ptr<FieldEntity> &entity_ptr,
46 return this->
sPtr->getLocalUniqueId();
51 return this->
sPtr->getGlobalUniqueId();
60 const boost::shared_ptr<FieldEntity> &ent_ptr) {
94 const boost::shared_ptr<FieldEntity> &ent_ptr) {
154 template <
typename T>
164 return this->
sPtr->getGlobalUniqueId();
169 return this->
sPtr->getEntGlobalUniqueId();
177 return this->
sPtr->getEntLocalUniqueId();
182 return this->
sPtr->getNonNonuniqueShortId();
196 return this->
sPtr->getDofOrder();
201 return this->
sPtr->getDofCoeffIdx();
214 return this->
sPtr->getFieldEntityPtr();
258 const int dof_idx = -1,
const int petsc_gloabl_dof_idx = -1,
259 const int petsc_local_dof_idx = -1,
const int part = -1);
292 friend std::ostream &
operator<<(std::ostream &os,
303 typedef multi_index_container<
304 boost::shared_ptr<DofEntity>,
309 const_mem_fun<DofEntity, UId, &DofEntity::getLocalUniqueId>>,
314 const_mem_fun<DofEntity, EntityHandle, &DofEntity::getEnt>>
331 multi_index_container<boost::shared_ptr<DofEntity>,
334 ordered_unique<const_mem_fun<
343 boost::shared_ptr<DofEntity>,
347 const_mem_fun<DofEntity, UId, &DofEntity::getGlobalUniqueId>>
359 typedef multi_index_container<
360 boost::shared_ptr<DofEntity>,
364 const_mem_fun<DofEntity, UId, &DofEntity::getLocalUniqueId>>,
367 const_mem_fun<DofEntity, char, &DofEntity::getActive>>
378 typedef multi_index_container<
379 boost::shared_ptr<FEDofEntity>,
382 ordered_unique<tag<Unique_mi_tag>,
386 ordered_non_unique<tag<Ent_mi_tag>,
405 typedef multi_index_container<
406 boost::shared_ptr<FENumeredDofEntity>,
407 indexed_by<ordered_unique<
438 typedef multi_index_container<
439 boost::shared_ptr<NumeredDofEntity>,
443 ordered_unique<tag<Unique_mi_tag>,
450 member<NumeredDofEntity, unsigned int, &NumeredDofEntity::pArt>>,
455 ordered_non_unique<tag<PetscGlobalIdx_mi_tag>,
459 ordered_non_unique<tag<PetscLocalIdx_mi_tag>,
493 multi_index_container<boost::shared_ptr<NumeredDofEntity>,
496 ordered_unique<const_mem_fun<
506 boost::shared_ptr<NumeredDofEntity>,
511 multi_index_container<boost::shared_ptr<NumeredDofEntity>,
512 indexed_by<ordered_non_unique<const_mem_fun<
517 multi_index_container<
518 boost::shared_ptr<NumeredDofEntity>,
519 indexed_by<ordered_non_unique<const_mem_fun<
530 void operator()(boost::shared_ptr<DofEntity> &dof);
541 const DofIdx petsc_gloabl_dof_idx)
543 inline void operator()(boost::shared_ptr<NumeredDofEntity> &dof)
const {
554 const unsigned int part,
const DofIdx mofem_dof_idx,
555 const DofIdx petsc_gloabl_dof_idx)
558 inline void operator()(boost::shared_ptr<NumeredDofEntity> &dof)
const {
569 const DofIdx petsc_gloabl_dof_idx,
570 const DofIdx petsc_local_dof_idx)
573 inline void operator()(boost::shared_ptr<NumeredDofEntity> &dof)
const {
588 inline void operator()(boost::shared_ptr<NumeredDofEntity> &dof)
const {
601 inline void operator()(boost::shared_ptr<NumeredDofEntity> &dof)
const {
616 const unsigned int part,
const DofIdx mofem_idx,
617 const DofIdx petsc_gloabl_dof_idx,
const DofIdx petsc_local_dof_idx)
621 inline void operator()(boost::shared_ptr<NumeredDofEntity> &dof)
const {
630 #endif // __DOFSMULTIINDICES_HPP__
DofEntity(const boost::shared_ptr< FieldEntity > &entity_ptr, const ApproximationOrder dof_order, const FieldCoefficientsNumber dof_rank, const DofIdx dof)
NumeredDofEntity_part_and_indices_change(const unsigned int part, const DofIdx petsc_gloabl_dof_idx, const DofIdx petsc_local_dof_idx)
std::ostream & operator<<(std::ostream &os, const EntitiesFieldData::EntData &e)
friend std::ostream & operator<<(std::ostream &os, const DofEntity &e)
EntityHandle getEnt() const
static ShortId getNonNonuniqueShortId(const DofIdx dof, const boost::shared_ptr< FieldEntity > &ent_ptr)
const DofIdx petscGloablDofIdx
const UId & getEntGlobalUniqueId() const
void operator()(boost::shared_ptr< NumeredDofEntity > &dof) const
NumeredDofEntity_multiIndex::index< Ent_mi_tag >::type NumeredDofEntityByEnt
Numbered DoF multi-index by entity.
keeps information about DOF on the entity
static UId getLoFieldEntityUId(const UId &uid)
NumeredDofEntity_part_and_glob_idx_change(const unsigned int part, const DofIdx petsc_gloabl_dof_idx)
const DofIdx petscLocalDofIdx
DofIdx getPetscLocalDofIdx() const
static UId getUniqueIdCalculate(const DofIdx dof, UId ent_uid)
keeps information about indexed dofs for the finite element
friend std::ostream & operator<<(std::ostream &os, const FENumeredDofEntity &e)
std::vector< boost::weak_ptr< DofEntity > > DofEntity_vector_view
vector view on DofEntity by uid
FieldCoefficientsNumber getDofCoeffIdx() const
interface_DofEntity< DofEntity > interface_type_DofEntity
virtual ~DofEntity()=default
boost::shared_ptr< FieldEntity > sPtr
DofIdx getEntDofIdx() const
unsigned int getPart() const
Interface to FieldEntity.
NumeredDofEntity_multiIndex::index< PetscLocalIdx_mi_tag >::type NumeredDofEntityByLocalIdx
Numbered DoF multi-index by local index.
static UId getGlobalUniqueIdCalculate(const DofIdx dof, const boost::shared_ptr< FieldEntity > &ent_ptr)
Calculate UId for DOF.
DofEntity_active_change(bool active)
FENumeredDofEntity_multiIndex::index< Ent_mi_tag >::type FENumeredDofEntityByEnt
Numbered DoF multi-index by entity.
FEDofEntity_multiIndex::index< Unique_mi_tag >::type FEDofEntityByUId
Dof entity multi-index by UId and entity.
friend std::ostream & operator<<(std::ostream &os, const NumeredDofEntity &e)
DofEntity_multiIndex::index< Unique_mi_tag >::type DofEntityByUId
static UId getHiFieldEntityUId(const UId &uid)
const DofIdx petscGloablDofIdx
multi_index_container< boost::shared_ptr< DofEntity >, indexed_by< ordered_unique< const_mem_fun< DofEntity, UId, &DofEntity::getLocalUniqueId > >, ordered_non_unique< const_mem_fun< DofEntity, char, &DofEntity::getActive > > > > DofEntity_multiIndex_active_view
multi-index view on DofEntity activity
multi_index_container< boost::shared_ptr< DofEntity >, indexed_by< ordered_unique< const_mem_fun< DofEntity, UId, &DofEntity::getLocalUniqueId > > > > DofEntity_multiIndex_uid_view
multi-index view on DofEntity by uid
NumeredDofEntity_part_and_mofem_glob_idx_change(const unsigned int part, const DofIdx mofem_dof_idx, const DofIdx petsc_gloabl_dof_idx)
multi_index_container< boost::shared_ptr< NumeredDofEntity >, indexed_by< ordered_non_unique< const_mem_fun< NumeredDofEntity, DofIdx, &NumeredDofEntity::getPetscLocalDofIdx > >> > NumeredDofEntity_multiIndex_petsc_local_dof_view_ordered_non_unique
ShortId getNonNonuniqueShortId() const
multi_index_container< boost::shared_ptr< NumeredDofEntity >, indexed_by< ordered_unique< tag< Unique_mi_tag >, const_mem_fun< NumeredDofEntity::interface_type_DofEntity, UId, &NumeredDofEntity::getLocalUniqueId > >, ordered_non_unique< tag< Part_mi_tag >, member< NumeredDofEntity, unsigned int, &NumeredDofEntity::pArt > >, ordered_non_unique< tag< Idx_mi_tag >, member< NumeredDofEntity, DofIdx, &NumeredDofEntity::dofIdx > >, ordered_non_unique< tag< PetscGlobalIdx_mi_tag >, member< NumeredDofEntity, DofIdx, &NumeredDofEntity::petscGloablDofIdx > >, ordered_non_unique< tag< PetscLocalIdx_mi_tag >, member< NumeredDofEntity, DofIdx, &NumeredDofEntity::petscLocalDofIdx > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< NumeredDofEntity::interface_type_DofEntity, EntityHandle, &NumeredDofEntity::getEnt > > > > NumeredDofEntity_multiIndex
MultiIndex container keeps NumeredDofEntity.
NumeredDofEntity_multiIndex::index< Unique_mi_tag >::type NumeredDofEntityByUId
Numbered DoF multi-index by UId.
FieldCoefficientsNumber getDofCoeffIdx() const
static UId getLocalUniqueIdCalculate(const DofIdx dof, const boost::shared_ptr< FieldEntity > &ent_ptr)
boost::shared_ptr< FieldEntity > & getFieldEntityPtr() const
virtual ~interface_DofEntity()=default
void operator()(boost::shared_ptr< NumeredDofEntity > &dof) const
NumeredDofEntity_local_idx_change(const DofIdx petsc_local_dof_idx)
keeps information about indexed dofs for the problem
implementation of Data Operators for Forces and Sources
boost::shared_ptr< DofEntity > & getDofEntityPtr() const
int ShortId
Unique Id in the field.
ApproximationOrder getDofOrder() const
UId getLocalUniqueId() const
int FieldCoefficientsNumber
Number of field coefficients.
UId getLocalUniqueIdCalculate()
Get the Local Unique Id Calculate object.
FENumeredDofEntity_multiIndex::index< Unique_mi_tag >::type FENumeredDofEntityByUId
Dof entity multi-index by UId.
EntityHandle getEnt() const
multi_index_container< boost::shared_ptr< NumeredDofEntity >, indexed_by< ordered_unique< const_mem_fun< NumeredDofEntity::interface_type_DofEntity, UId, &NumeredDofEntity::getLocalUniqueId > > > > NumeredDofEntity_multiIndex_uid_view_ordered
NumeredDofEntity_mofem_index_change(const DofIdx mofem_idx)
const DofIdx petscGloablDofIdx
bool getHasLocalIndex() const
friend std::ostream & operator<<(std::ostream &os, const FEDofEntity &e)
DofIdx getPetscGlobalDofIdx() const
void operator()(boost::shared_ptr< DofEntity > &dof)
const DofIdx petscGloablDofIdx
virtual ~NumeredDofEntity()=default
FENumeredDofEntity()=delete
FieldCoefficientsNumber getNbOfCoeffs() const
UId getLocalUniqueId() const
ShortId getNonNonuniqueShortId() const
get short uid it is unique in combination with entity handle
multi_index_container< boost::shared_ptr< DofEntity >, indexed_by< ordered_unique< const_mem_fun< DofEntity, UId, &DofEntity::getGlobalUniqueId > > > > DofEntity_multiIndex_global_uid_view
multi-index view on DofEntity by uid
static UId getLoFieldEntityUId(const FieldBitNumber bit, const EntityHandle ent)
NumeredDofEntity(const boost::shared_ptr< DofEntity > &dof_entity_ptr, const int dof_idx=-1, const int petsc_gloabl_dof_idx=-1, const int petsc_local_dof_idx=-1, const int part=-1)
FieldData & getFieldData() const
const DofIdx petscLocalDofIdx
boost::shared_ptr< FieldEntity > & getFieldEntityPtr() const
multi_index_container< boost::shared_ptr< FEDofEntity >, indexed_by< ordered_unique< tag< Unique_mi_tag >, const_mem_fun< FEDofEntity::DofEntity, UId, &FEDofEntity::getLocalUniqueId > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< FEDofEntity::DofEntity, EntityHandle, &FEDofEntity::getEnt > > > > FEDofEntity_multiIndex
MultiIndex container keeps FEDofEntity.
int ApproximationOrder
Approximation on the entity.
ApproximationOrder getDofOrder() const
static UId getHiFieldEntityUId(const FieldBitNumber bit, const EntityHandle ent)
void operator()(boost::shared_ptr< NumeredDofEntity > &dof) const
multi_index_container< boost::shared_ptr< NumeredDofEntity >, indexed_by< ordered_non_unique< const_mem_fun< NumeredDofEntity::interface_type_DofEntity, FieldCoefficientsNumber, &NumeredDofEntity::getDofCoeffIdx > >> > NumeredDofEntity_multiIndex_coeff_idx_ordered_non_unique
interface_DofEntity(const boost::shared_ptr< T > &sptr)
const UId getEntGlobalUniqueId() const
char FieldBitNumber
Field bit number.
DofEntity_multiIndex::index< Ent_mi_tag >::type DofEntityByEnt
Dof multi-index by entity.
const UId & getEntLocalUniqueId() const
void operator()(boost::shared_ptr< NumeredDofEntity > &dof) const
UId getGlobalUniqueId() const
UId getGlobalUniqueId() const
NumeredDofEntity_part_and_all_indices_change(const unsigned int part, const DofIdx mofem_idx, const DofIdx petsc_gloabl_dof_idx, const DofIdx petsc_local_dof_idx)
void operator()(boost::shared_ptr< NumeredDofEntity > &dof) const
const DofIdx petscLocalDofIdx
multi_index_container< boost::shared_ptr< DofEntity >, indexed_by< ordered_unique< tag< Unique_mi_tag >, const_mem_fun< DofEntity, UId, &DofEntity::getLocalUniqueId > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< DofEntity, EntityHandle, &DofEntity::getEnt > > > > DofEntity_multiIndex
MultiIndex container keeps DofEntity.
multi_index_container< boost::shared_ptr< NumeredDofEntity >, indexed_by< hashed_unique< const_mem_fun< NumeredDofEntity, DofIdx, &NumeredDofEntity::getDofIdx > >> > NumeredDofEntity_multiIndex_idx_view_hashed
keeps information about indexed dofs for the finite element
const std::array< int, MAX_DOFS_ON_ENTITY > & getDofOrderMap() const
get hash-map relating dof index on entity with its order
multi_index_container< boost::shared_ptr< FENumeredDofEntity >, indexed_by< ordered_unique< tag< Unique_mi_tag >, const_mem_fun< FENumeredDofEntity::interface_type_DofEntity, UId, &FENumeredDofEntity::getLocalUniqueId > >, ordered_non_unique< tag< Ent_mi_tag >, const_mem_fun< FENumeredDofEntity::interface_type_DofEntity, EntityHandle, &FENumeredDofEntity::getEnt > > > > FENumeredDofEntity_multiIndex
MultiIndex container keeps FENumeredDofEntity.
void operator()(boost::shared_ptr< NumeredDofEntity > &dof) const
const UId & getEntLocalUniqueId() const
FieldData & getFieldData() const
DofIdx getEntDofIdx() const