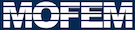 |
| v0.14.0
|
Go to the documentation of this file.
5 #ifndef __PETSCSPARTOBJ_HPP__
6 #define __PETSCSPARTOBJ_HPP__
10 return reinterpret_cast<PetscObject
>(obj);
49 ierr = PetscObjectDestroy(
reinterpret_cast<PetscObject *
>(&obj));
53 CHKERRABORT(comm,
ierr);
81 template <
typename OBJ>
83 :
public boost::intrusive_ptr<typename std::remove_pointer<OBJ>::type> {
87 using Derived::Derived;
100 : boost::intrusive_ptr<typename
std::remove_pointer<OBJ>::
type>(o,
104 operator OBJ() {
return this->get(); }
105 explicit operator PetscObject() {
106 return reinterpret_cast<PetscObject
>(this->get());
141 inline auto createDM(MPI_Comm comm,
const std::string dm_type_name) {
150 const std::string dm_type_name) {
151 return createDM(comm, dm_type_name);
163 "Failed to get comm from PETSc object");
180 PetscInt nghost,
const PetscInt ghosts[]) {
183 "Failed to create ghosted Vec");
189 PetscInt
N, PetscInt nghost,
190 const PetscInt ghosts[]) {
237 "Failed to duplicate Mat");
245 MatDuplicateOption op) {
288 PetscCopyMode mode) {
321 "Failed to create AO");
339 const PetscInt myapp[],
const PetscInt mypetsc[]) {
384 ISLocalToGlobalMapping map_raw;
386 "create local to global mapping");
auto createAOMappingIS(IS isapp, IS ispetsc)
Creates an application mapping using two index sets.
void intrusive_ptr_release< KSP >(KSP obj)
auto createISGeneral(MPI_Comm comm, PetscInt n, const PetscInt idx[], PetscCopyMode mode)
Creates a data structure for an index set containing a list of integers.
auto createTS(MPI_Comm comm)
#define CHK_THROW_MESSAGE(err, msg)
Check and throw MoFEM exception.
auto createSNES(MPI_Comm comm)
DEPRECATED auto createSmartDM(MPI_Comm comm, const std::string dm_type_name)
DEPRECATED auto createSmartVectorMPI(MPI_Comm comm, PetscInt n, PetscInt N)
void intrusive_ptr_release< TS >(TS obj)
PetscObject getPetscObject(T obj)
auto createKSP(MPI_Comm comm)
void intrusive_ptr_release< SNES >(SNES obj)
DEPRECATED SmartPetscObj< Mat > smartMatDuplicate(Mat mat, MatDuplicateOption op)
void intrusive_ptr_release< IS >(IS obj)
auto createISLocalToGlobalMapping(IS is)
auto createAOMapping(MPI_Comm comm, PetscInt napp, const PetscInt myapp[], const PetscInt mypetsc[])
Creates an application mapping using two integer arrays.
auto matCreateVecs(Mat mat)
auto isDifference(IS is1, IS is2)
Get ISDifference.
auto createGhostVector(MPI_Comm comm, PetscInt n, PetscInt N, PetscInt nghost, const PetscInt ghosts[])
Create smart ghost vector.
void intrusive_ptr_release< Vec >(Vec obj)
implementation of Data Operators for Forces and Sources
auto createDM(MPI_Comm comm, const std::string dm_type_name)
Creates smart DM object.
auto createPC(MPI_Comm comm)
DEPRECATED SmartPetscObj< Vec > smartVectorDuplicate(Vec vec)
void intrusive_ptr_release< DM >(DM obj)
SmartPetscObj< Mat > matDuplicate(Mat mat, MatDuplicateOption op)
DEPRECATED auto createSmartGhostVector(MPI_Comm comm, PetscInt n, PetscInt N, PetscInt nghost, const PetscInt ghosts[])
void intrusive_ptr_add_ref(OBJ obj)
It is used by intrusive_ptr to bump reference.
SmartPetscObj< Vec > vectorDuplicate(Vec vec)
Create duplicate vector of smart vector.
void intrusive_ptr_release< Mat >(Mat obj)
SmartPetscObj(OBJ o, bool add_ref=false)
Construct a new Smart Petsc Obj object.
void intrusive_ptr_release(OBJ obj)
It is used by intrusive_ptr to dereference and destroy petsc object.
auto createVecScatter(Vec x, IS ix, Vec y, IS iy)
Create a Vec Scatter object.
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
const FTensor::Tensor2< T, Dim, Dim > Vec
MPI_Comm getCommFromPetscObject(PetscObject obj)
Get the Comm From Petsc Object object.
SmartPetscObj(std::nullptr_t ptr)
auto createVectorMPI(MPI_Comm comm, PetscInt n, PetscInt N)
Create MPI Vector.
intrusive_ptr for managing petsc objects
void intrusive_ptr_release< AO >(AO obj)
boost::intrusive_ptr< typename std::remove_pointer< AO >::type > Derived
auto isAllGather(IS is)
IS All gather.