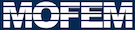 |
| v0.14.0
|
Go to the documentation of this file.
10 #define DM_NO_ELEMENT "DMNONEFE"
151 PetscLayout *layout);
194 ScatterMode scatter_mode);
211 ScatterMode scatter_mode);
250 DM dm,
const std::string fe_name, boost::shared_ptr<MoFEM::FEMethod> method,
251 int low_rank,
int up_rank,
276 boost::shared_ptr<MoFEM::FEMethod> method,
311 boost::shared_ptr<MoFEM::FEMethod> method,
312 boost::shared_ptr<MoFEM::BasicMethod> pre_only,
313 boost::shared_ptr<MoFEM::BasicMethod> post_only);
348 boost::shared_ptr<MoFEM::FEMethod> method,
349 boost::shared_ptr<MoFEM::BasicMethod> pre_only,
350 boost::shared_ptr<MoFEM::BasicMethod> post_only);
367 boost::shared_ptr<MoFEM::FEMethod> method,
368 boost::shared_ptr<MoFEM::BasicMethod> pre_only,
369 boost::shared_ptr<MoFEM::BasicMethod> post_only);
386 boost::shared_ptr<MoFEM::FEMethod> method,
387 boost::shared_ptr<MoFEM::BasicMethod> pre_only,
388 boost::shared_ptr<MoFEM::BasicMethod> post_only);
405 boost::shared_ptr<MoFEM::FEMethod> method,
406 boost::shared_ptr<MoFEM::BasicMethod> pre_only,
407 boost::shared_ptr<MoFEM::BasicMethod> post_only);
415 boost::shared_ptr<MoFEM::FEMethod> method,
416 boost::shared_ptr<MoFEM::BasicMethod> pre_only,
417 boost::shared_ptr<MoFEM::BasicMethod> post_only);
444 boost::shared_ptr<MoFEM::FEMethod> method,
445 boost::shared_ptr<MoFEM::BasicMethod> pre_only,
446 boost::shared_ptr<MoFEM::BasicMethod> post_only);
469 boost::shared_ptr<MoFEM::FEMethod> method,
470 boost::shared_ptr<MoFEM::BasicMethod> pre_only,
471 boost::shared_ptr<MoFEM::BasicMethod> post_only);
484 boost::shared_ptr<MoFEM::FEMethod> method,
485 boost::shared_ptr<MoFEM::BasicMethod> pre_only,
486 boost::shared_ptr<MoFEM::BasicMethod> post_only);
502 boost::shared_ptr<MoFEM::FEMethod> method,
503 boost::shared_ptr<MoFEM::BasicMethod> pre_only,
504 boost::shared_ptr<MoFEM::BasicMethod> post_only);
532 boost::shared_ptr<MoFEM::FEMethod> method,
533 boost::shared_ptr<MoFEM::BasicMethod> pre_only,
534 boost::shared_ptr<MoFEM::BasicMethod> post_only);
574 boost::shared_ptr<MoFEM::KspCtx> ksp_ctx);
595 boost::shared_ptr<MoFEM::SnesCtx> snes_ctx);
608 const boost::shared_ptr<MoFEM::TsCtx> &
ts_ctx);
697 #if PETSC_VERSION_GE(3, 7, 0)
700 #elif PETSC_VERSION_GE(3, 5, 3)
737 boost::shared_ptr<Range> r_ptr);
741 boost::shared_ptr<Range> r_ptr);
761 boost::shared_ptr<Range> r_ptr);
766 boost::shared_ptr<Range> r_ptr);
901 char ***fieldNames, IS **fields);
977 virtual ~DMCtx() =
default;
994 "Get cot get problem ptr from DM");
1033 boost::shared_ptr<MoFEM::KspCtx> ksp_ctx;
1047 boost::shared_ptr<MoFEM::SnesCtx> snes_ctx;
1061 boost::shared_ptr<MoFEM::TsCtx>
ts_ctx;
1078 return prb_ptr->getSubData();
1084 #endif //__DMMOFEM_H
PetscBool destroyProblem
If true destroy problem with DM.
PetscErrorCode DMSetFromOptions_MoFEM(DM dm)
PetscErrorCode DMMoFEMSetSnesCtx(DM dm, boost::shared_ptr< MoFEM::SnesCtx > snes_ctx)
Set MoFEM::SnesCtx data structure.
PetscErrorCode DMMoFEMSetKspCtx(DM dm, boost::shared_ptr< MoFEM::KspCtx > ksp_ctx)
set MoFEM::KspCtx data structure
DEPRECATED auto smartGetDMSnesCtx(DM dm)
PetscErrorCode DMMoFEMKSPSetComputeRHS(DM dm, const char fe_name[], MoFEM::FEMethod *method, MoFEM::BasicMethod *pre_only, MoFEM::BasicMethod *post_only)
set KSP right hand side evaluation function
PetscErrorCode DMMoFEMAddSubFieldCol(DM dm, const char field_name[])
DEPRECATED auto smartGetDMKspCtx(DM dm)
PetscErrorCode DMMoFEMGetDestroyProblem(DM dm, PetscBool *destroy_problem)
structure for User Loop Methods on finite elements
#define CHK_THROW_MESSAGE(err, msg)
Check and throw MoFEM exception.
PetscErrorCode DMMoFEMSetSquareProblem(DM dm, PetscBool square_problem)
set squared problem
auto getDMKspCtx(DM dm)
Get KSP context data structure used by DM.
const Problem * problemPtr
pinter to problem data structure
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
PetscErrorCode DMGlobalToLocalBegin_MoFEM(DM dm, Vec, InsertMode, Vec)
PetscErrorCode DMMoFEMGetSubColIS(DM dm, IS *is)
get sub problem is
PetscErrorCode DMMoFEMAddColCompositeProblem(DM dm, const char prb_name[])
Add problem to composite DM on col.
PetscErrorCode DMSetUp_MoFEM(DM dm)
PetscErrorCode DMoFEMMeshToLocalVector(DM dm, Vec l, InsertMode mode, ScatterMode scatter_mode)
set local (or ghosted) vector values on mesh for partition only
PetscErrorCode DMMoFEMTSSetRHSJacobian(DM dm, const std::string fe_name, boost::shared_ptr< MoFEM::FEMethod > method, boost::shared_ptr< MoFEM::BasicMethod > pre_only, boost::shared_ptr< MoFEM::BasicMethod > post_only)
set TS the right hand side jacobian
PetscErrorCode DMMoFEMGetIsSubDM(DM dm, PetscBool *is_sub_dm)
Data structure to exchange data between mofem and User Loop Methods on entities.
PetscErrorCode DMMoFEMKSPSetComputeOperators(DM dm, const char fe_name[], MoFEM::FEMethod *method, MoFEM::BasicMethod *pre_only, MoFEM::BasicMethod *post_only)
Set KSP operators and push mofem finite element methods.
PetscErrorCode DMLocalToGlobalBegin_MoFEM(DM, Vec, InsertMode, Vec)
auto getDMSubData(DM dm)
Get sub problem data structure.
PetscErrorCode DMMoFEMSwapDMCtx(DM dm, DM dm_swap)
Swap internal data struture.
auto createDMMatrix(DM dm)
Get smart matrix from DM.
PetscErrorCode DMMoFEMAddElement(DM dm, std::string fe_name)
add element to dm
Deprecated interface functions.
PetscErrorCode DMMoFEMGetIsCompDM(DM dm, PetscBool *is_comp_dm)
Get if this DM is composite DM.
PetscErrorCode DMCreateGlobalVector_MoFEM(DM dm, Vec *g)
DMShellSetCreateGlobalVector.
std::map< std::string, boost::shared_ptr< Range > > mapTypeRow
PetscErrorCode DMMoFEMSetDestroyProblem(DM dm, PetscBool destroy_problem)
std::map< std::string, boost::shared_ptr< Range > > mapTypeCol
FTensor::Index< 'M', 3 > M
DEPRECATED auto smartGetDMTsCtx(DM dm)
PetscErrorCode DMCreateMatrix_MoFEM(DM dm, Mat *M)
PetscErrorCode DMMoFEMAddRowCompositeProblem(DM dm, const char prb_name[])
Add problem to composite DM on row.
auto createDMVector(DM dm)
Get smart vector from DM.
PetscErrorCode DMMoFEMTSSetIJacobian(DM dm, const std::string fe_name, boost::shared_ptr< MoFEM::FEMethod > method, boost::shared_ptr< MoFEM::BasicMethod > pre_only, boost::shared_ptr< MoFEM::BasicMethod > post_only)
set TS Jacobian evaluation function
PetscErrorCode DMoFEMLoopFiniteElementsUpAndLowRank(DM dm, const char fe_name[], MoFEM::FEMethod *method, int low_rank, int up_rank, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr())
Executes FEMethod for finite elements in DM.
implementation of Data Operators for Forces and Sources
PetscErrorCode DMMoFEMTSSetI2Jacobian(DM dm, const std::string fe_name, boost::shared_ptr< MoFEM::FEMethod > method, boost::shared_ptr< MoFEM::BasicMethod > pre_only, boost::shared_ptr< MoFEM::BasicMethod > post_only)
set TS Jacobian evaluation function
PetscErrorCode DMDestroy_MoFEM(DM dm)
Destroys dm with MoFEM data structure.
PetscErrorCode DMSubDMSetUp_MoFEM(DM subdm)
PetscErrorCode DMMoFEMTSSetI2Function(DM dm, const std::string fe_name, boost::shared_ptr< MoFEM::FEMethod > method, boost::shared_ptr< MoFEM::BasicMethod > pre_only, boost::shared_ptr< MoFEM::BasicMethod > post_only)
set TS implicit function evaluation function
PetscErrorCode DMMoFEMCreateSubDM(DM subdm, DM dm, const char problem_name[])
Must be called by user to set Sub DM MoFEM data structures.
PetscErrorCode DMMoFEMGetSubRowIS(DM dm, IS *is)
get sub problem is
boost::shared_ptr< KspCtx > kspCtx
data structure KSP
std::vector< std::string > colCompPrb
PetscErrorCode DMoFEMMeshToGlobalVector(DM dm, Vec g, InsertMode mode, ScatterMode scatter_mode)
set ghosted vector values on all existing mesh entities
PetscErrorCode DMMoFEMGetSnesCtx(DM dm, MoFEM::SnesCtx **snes_ctx)
get MoFEM::SnesCtx data structure
Interface for Time Stepping (TS) solver.
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
PetscBool isProblemBuild
True if problem is build.
boost::shared_ptr< TsCtx > tsCtx
data structure for TS solver
DEPRECATED auto smartCreateDMMatrix(DM dm)
PetscErrorCode DMMoFEMSNESSetJacobian(DM dm, const char fe_name[], MoFEM::FEMethod *method, MoFEM::BasicMethod *pre_only, MoFEM::BasicMethod *post_only)
set SNES Jacobian evaluation function
DEPRECATED auto smartCreateDMVector(DM dm)
PetscErrorCode DMoFEMPreProcessFiniteElements(DM dm, MoFEM::FEMethod *method)
execute finite element method for each element in dm (problem)
PETSc Discrete Manager data structure.
PetscBool isSquareMatrix
true if rows equals to cols
PetscErrorCode DMMoFEMGetSquareProblem(DM dm, PetscBool *square_problem)
get squared problem
PetscErrorCode DMGlobalToLocalEnd_MoFEM(DM dm, Vec, InsertMode, Vec)
Data structure to exchange data between mofem and User Loop Methods.
PetscErrorCode DMoFEMPostProcessFiniteElements(DM dm, MoFEM::FEMethod *method)
execute finite element method for each element in dm (problem)
boost::shared_ptr< CacheTuple > CacheTupleSharedPtr
PetscErrorCode DMMoFEMCreateMoFEM(DM dm, MoFEM::Interface *m_field_ptr, const char problem_name[], const MoFEM::BitRefLevel bit_level, const MoFEM::BitRefLevel bit_mask=MoFEM::BitRefLevel().set())
Must be called by user to set MoFEM data structures.
PetscBool isPartitioned
true if read mesh is on parts
PetscErrorCode DMSetOperators_MoFEM(DM dm)
Set operators for MoFEM dm.
PetscErrorCode DMCreateFieldIS_MoFEM(DM dm, PetscInt *numFields, char ***fieldNames, IS **fields)
constexpr auto field_name
boost::shared_ptr< SnesCtx > snesCtx
data structure SNES
PetscErrorCode DMMoFEMResolveSharedFiniteElements(DM dm, std::string fe_name)
Resolve shared entities.
PetscErrorCode DMMoFEMGetKspCtx(DM dm, MoFEM::KspCtx **ksp_ctx)
get MoFEM::KspCtx data structure
base class for all interface classes
PetscErrorCode DMMoFEMSetTsCtx(DM dm, boost::shared_ptr< MoFEM::TsCtx > ts_ctx)
Set MoFEM::TsCtx data structure.
const double v
phase velocity of light in medium (cm/ns)
PetscErrorCode DMMoFEMTSSetRHSFunction(DM dm, const std::string fe_name, boost::shared_ptr< MoFEM::FEMethod > method, boost::shared_ptr< MoFEM::BasicMethod > pre_only, boost::shared_ptr< MoFEM::BasicMethod > post_only)
set TS the right hand side function
PetscErrorCode DMCreate_MoFEM(DM dm)
Create dm data structure with MoFEM data structure.
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const
PetscErrorCode DMMoFEMGetFieldIS(DM dm, RowColData rc, const char field_name[], IS *is)
get field is in the problem
Interface * mField_ptr
MoFEM interface.
PetscErrorCode DMoFEMGetInterfacePtr(DM dm, MoFEM::Interface **m_field_ptr)
Get pointer to MoFEM::Interface.
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
PetscErrorCode DMMoFEMTSSetMonitor(DM dm, TS ts, const std::string fe_name, boost::shared_ptr< MoFEM::FEMethod > method, boost::shared_ptr< MoFEM::BasicMethod > pre_only, boost::shared_ptr< MoFEM::BasicMethod > post_only)
Set Monitor To TS solver.
PetscErrorCode DMMoFEMTSSetIFunction(DM dm, const char fe_name[], MoFEM::FEMethod *method, MoFEM::BasicMethod *pre_only, MoFEM::BasicMethod *post_only)
set TS implicit function evaluation function
auto getProblemPtr(DM dm)
get problem pointer from DM
const FTensor::Tensor2< T, Dim, Dim > Vec
MPI_Comm getCommFromPetscObject(PetscObject obj)
Get the Comm From Petsc Object object.
PetscErrorCode DMMoFEMGetIsPartitioned(DM dm, PetscBool *is_partitioned)
PetscErrorCode DMMoFEMGetProblemPtr(DM dm, const MoFEM::Problem **problem_ptr)
Get pointer to problem data structure.
PetscErrorCode DMMoFEMUnSetElement(DM dm, std::string fe_name)
unset element from dm
boost::weak_ptr< CacheTuple > CacheTupleWeakPtr
std::vector< std::string > colFields
PetscErrorCode DMMoFEMGetTsCtx(DM dm, MoFEM::TsCtx **ts_ctx)
get MoFEM::TsCtx data structure
Interface for linear (KSP) solver.
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
std::vector< std::string > rowFields
auto getDMSnesCtx(DM dm)
Get SNES context data structure used by DM.
Interface for nonlinear (SNES) solver.
keeps basic data about problem
PetscErrorCode DMMoFEMAddSubFieldRow(DM dm, const char field_name[])
PetscErrorCode DMMoFEMGetProblemFiniteElementLayout(DM dm, std::string fe_name, PetscLayout *layout)
Get finite elements layout in the problem.
PetscErrorCode DMMoFEMDuplicateDMCtx(DM dm, DM dm_duplicate)
Duplicate internal data struture.
PetscErrorCode DMLocalToGlobalEnd_MoFEM(DM, Vec, InsertMode, Vec)
PetscErrorCode DMoFEMLoopFiniteElements(DM dm, const char fe_name[], MoFEM::FEMethod *method, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr())
Executes FEMethod for finite elements in DM.
PetscErrorCode DMoFEMLoopDofs(DM dm, const char field_name[], MoFEM::DofMethod *method)
execute method for dofs on field in problem
PetscErrorCode DMCreateLocalVector_MoFEM(DM dm, Vec *l)
DMShellSetCreateLocalVector.
auto getDMTsCtx(DM dm)
Get TS context data structure used by DM.
std::string problemName
Problem name.
FTensor::Index< 'l', 3 > l
std::vector< std::string > rowCompPrb
const Problem * problemMainOfSubPtr
pinter to main problem to sub-problem
PetscErrorCode DMMoFEMSetVerbosity(DM dm, const int verb)
Set verbosity level.
PetscErrorCode DMMoFEMSetIsPartitioned(DM dm, PetscBool is_partitioned)
PetscErrorCode DMMoFEMSNESSetFunction(DM dm, const char fe_name[], MoFEM::FEMethod *method, MoFEM::BasicMethod *pre_only, MoFEM::BasicMethod *post_only)
set SNES residual evaluation function