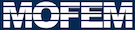 |
| v0.14.0
|
Go to the documentation of this file.
88 friend PetscErrorCode
KspRhs(KSP ksp,
Vec f,
void *ctx);
89 friend PetscErrorCode
KspMat(KSP ksp, Mat
A, Mat B,
void *ctx);
143 PetscErrorCode
KspRhs(KSP ksp,
Vec f,
void *ctx);
153 PetscErrorCode
KspMat(KSP ksp, Mat
A, Mat B,
void *ctx);
157 #endif // __KSPCTX_HPP__
PetscErrorCode KspRhs(KSP ksp, Vec f, void *ctx)
Run over elements in the lists.
FEMethodsSequence & getComputeRhs()
FEMethodsSequence loops_to_do_Rhs
BasicMethodsSequence & get_postProcess_to_do_Rhs()
std::string problemName
Problem name.
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
DEPRECATED FEMethodsSequence & get_loops_to_do_Rhs()
MoFEMTypes bH
If set to MF_EXIST check if element exist.
std::deque< PairNameFEMethodPtr > FEMethodsSequence
MoFEM::Interface & mField
DEPRECATED FEMethodsSequence & get_loops_to_do_Mat()
BasicMethodsSequence postProcess_Rhs
virtual ~KspCtx()=default
Deprecated interface functions.
DeprecatedCoreInterface Interface
BasicMethodsSequence & getPreProcSetOperators()
friend PetscErrorCode KspRhs(KSP ksp, Vec f, void *ctx)
Run over elements in the lists.
FEMethodsSequence loops_to_do_Mat
MoFEMErrorCode clearLoops()
Clear loops.
implementation of Data Operators for Forces and Sources
BasicMethodsSequence preProcess_Mat
FEMethodsSequence & getSetOperators()
friend PetscErrorCode KspMat(KSP ksp, Mat A, Mat B, void *ctx)
Run over elements in the list.
BasicMethodsSequence & getPostProcComputeRhs()
boost::movelib::unique_ptr< bool > matAssembleSwitch
std::deque< BasicMethodPtr > BasicMethodsSequence
BasicMethodsSequence postProcess_Mat
DEPRECATED BasicMethodsSequence & get_preProcess_to_do_Mat()
MoFEM::PairNameFEMethodPtr PairNameFEMethodPtr
DEPRECATED BasicMethodsSequence & get_preProcess_to_do_Rhs()
DEPRECATED BasicMethodsSequence & get_postProcess_to_do_Mat()
PetscLogEvent MOFEM_EVENT_KspRhs
PetscLogEvent MOFEM_EVENT_KspMat
BasicMethodsSequence & getPostProcSetOperators()
PetscErrorCode KspMat(KSP ksp, Mat A, Mat B, void *ctx)
Run over elements in the list.
KspCtx(MoFEM::Interface &m_field, const std::string &_problem_name)
const FTensor::Tensor2< T, Dim, Dim > Vec
BasicMethodsSequence & getPreProcComputeRhs()
Interface for linear (KSP) solver.
MoFEMTypes
Those types control how functions respond on arguments, f.e. error handling.
MoFEM::FEMethodsSequence FEMethodsSequence
boost::movelib::unique_ptr< bool > vecAssembleSwitch
MoFEM::BasicMethodsSequence BasicMethodsSequence
BasicMethodsSequence preProcess_Rhs