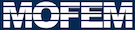 |
| v0.14.0
|
Go to the documentation of this file.
5 #ifndef __SNESCTX_HPP__
6 #define __SNESCTX_HPP__
50 auto core_log = logging::core::get();
117 friend PetscErrorCode
SnesRhs(SNES snes,
Vec x,
Vec f,
void *ctx);
118 friend PetscErrorCode
SnesMat(SNES snes,
Vec x, Mat
A, Mat B,
void *ctx);
121 MatAssemblyType
type);
190 PetscErrorCode
SnesMat(SNES snes,
Vec x, Mat
A, Mat B,
void *ctx);
224 #endif // __SNESCTX_HPP__
BasicMethodsSequence & getPostProcComputeRhs()
static bool checkIfChannelExist(const std::string channel)
Check if channel exist.
PetscLogEvent MOFEM_EVENT_SnesMat
Log events to assemble tangent matrix.
friend PetscErrorCode SnesRhs(SNES snes, Vec x, Vec f, void *ctx)
This is MoFEM implementation for the right hand side (residual vector) evaluation in SNES solver.
boost::movelib::unique_ptr< bool > vecAssembleSwitch
bool vErify
If true verify vector.
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
DEPRECATED BasicMethodsSequence & get_preProcess_to_do_Mat()
friend MoFEMErrorCode SNESMoFEMSetAssmblyType(SNES snes, MatAssemblyType type)
BasicMethodsSequence & getPreProcSetOperators()
std::deque< PairNameFEMethodPtr > FEMethodsSequence
moab::Interface & moab
moab Interface
MoFEM::FEMethodsSequence FEMethodsSequence
SnesCtx(Interface &m_field, const std::string &problem_name)
static boost::shared_ptr< SinkType > createSink(boost::shared_ptr< std::ostream > stream_ptr, std::string comm_filter)
Create a sink object.
BasicMethodsSequence postProcess_Mat
Deprecated interface functions.
BasicMethodsSequence & getPostProcSetOperators()
DeprecatedCoreInterface Interface
FEMethodsSequence loops_to_do_Rhs
MoFEMErrorCode copyLoops(const SnesCtx &snes_ctx)
Copy sequences from other SNES contex.
implementation of Data Operators for Forces and Sources
MoFEM::Interface & mField
database Interface
MoFEMErrorCode clearLoops()
Clear loops.
MatAssemblyType typeOfAssembly
type of assembly at the end
DEPRECATED FEMethodsSequence & get_loops_to_do_Rhs()
std::deque< BasicMethodPtr > BasicMethodsSequence
std::string problemName
problem name
friend MoFEMErrorCode SnesMoFEMSetBehavior(SNES snes, MoFEMTypes bh)
Set behavior if finite element in sequence does not exist.
BasicMethodsSequence postProcess_Rhs
Sequence of methods run after residual is assembled.
friend PetscErrorCode SnesMat(SNES snes, Vec x, Mat A, Mat B, void *ctx)
This is MoFEM implementation for the left hand side (tangent matrix) evaluation in SNES solver.
static boost::shared_ptr< std::ostream > getStrmWorld()
Get the strm world object.
FEMethodsSequence & getComputeRhs()
PetscLogEvent MOFEM_EVENT_SnesRhs
Log events to assemble residual.
MoFEMErrorCode SnesMoFEMSetBehavior(SNES snes, MoFEMTypes bh)
Set behavior if finite element in sequence does not exist.
BasicMethodsSequence preProcess_Rhs
Sequence of methods run before residual is assembled.
DEPRECATED BasicMethodsSequence & get_postProcess_to_do_Rhs()
#define MOFEM_LOG_TAG(channel, tag)
Tag channel.
MoFEM::BasicMethodsSequence BasicMethodsSequence
FEMethodsSequence & getSetOperators()
FEMethodsSequence loops_to_do_Mat
PetscErrorCode SnesRhs(SNES snes, Vec x, Vec f, void *ctx)
This is MoFEM implementation for the right hand side (residual vector) evaluation in SNES solver.
BasicMethodsSequence & getPreProcComputeRhs()
DEPRECATED BasicMethodsSequence & get_postProcess_to_do_Mat()
boost::movelib::unique_ptr< bool > matAssembleSwitch
DEPRECATED FEMethodsSequence & get_loops_to_do_Mat()
virtual ~SnesCtx()=default
BasicMethodsSequence preProcess_Mat
const FTensor::Tensor2< T, Dim, Dim > Vec
MoFEMTypes bH
If set to MF_EXIST check if element exist, default MF_EXIST.
MoFEMErrorCode MoFEMSNESMonitorFields(SNES snes, PetscInt its, PetscReal fgnorm, SnesCtx *snes_ctx)
Sens monitor printing residual field by field.
MoFEMTypes
Those types control how functions respond on arguments, f.e. error handling.
MoFEM::PairNameFEMethodPtr PairNameFEMethodPtr
Interface for nonlinear (SNES) solver.
static LoggerType & setLog(const std::string channel)
Set ans resset chanel logger.
PetscErrorCode SnesMat(SNES snes, Vec x, Mat A, Mat B, void *ctx)
This is MoFEM implementation for the left hand side (tangent matrix) evaluation in SNES solver.
DEPRECATED BasicMethodsSequence & get_preProcess_to_do_Rhs()
MoFEMErrorCode SnesMoFEMSetAssemblyType(SNES snes, MatAssemblyType type)
Set assembly type at the end of SnesMat.