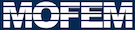 |
| v0.14.0
|
Go to the documentation of this file.
8 #include <petsc/private/tsimpl.h>
10 #define TSADAPTMOFEM "TSMoFEMAdapt"
49 virtual ~TsCtx() =
default;
192 PetscReal
a, Mat
A, Mat B,
void *ctx);
193 friend PetscErrorCode
TsMonitorSet(TS ts, PetscInt step, PetscReal
t,
Vec u,
204 Vec U_tt, PetscReal
v, PetscReal
a,
205 Mat
J, Mat
P,
void *ctx);
256 Mat
A, Mat B,
void *ctx);
330 PetscReal
a, PetscReal aa, Mat
A, Mat B,
373 PetscInt *next_sc, PetscReal *next_h,
374 PetscBool *accept, PetscReal *wlte,
375 PetscReal *wltea, PetscReal *wlter);
397 #endif // __TSCTX_HPP__
BasicMethodsSequence preProcessRHSJacobian
friend PetscErrorCode TsSetRHSFunction(TS ts, PetscReal t, Vec u, Vec F, void *ctx)
TS solver function.
FEMethodsSequence & getLoopsRHSFunction()
Get the loops to do RHSFunction object.
MoFEMErrorCode clearLoops()
Clear loops.
FEMethodsSequence & getLoopsRHSJacobian()
Get the loops to do RHSJacobian object.
PetscErrorCode TsSetIFunction(TS ts, PetscReal t, Vec u, Vec u_t, Vec F, void *ctx)
Set IFunction for TS solver.
PetscLogEvent MOFEM_EVENT_TsCtxIJacobian
PetscErrorCode TSAdaptDestroyMoFEM(TSAdapt adapt)
FEMethodsSequence & getLoopsMonitor()
Get the loops to do Monitor object.
BasicMethodsSequence & getPostProcessMonitor()
Get the postProcess to do Monitor object.
PetscLogEvent MOFEM_EVENT_TsCtxI2Function
FEMethodsSequence & getLoopsIFunction()
Get the loops to do IFunction object.
PetscLogEvent MOFEM_EVENT_TsCtxIFunction
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
std::deque< PairNameFEMethodPtr > FEMethodsSequence
PetscLogEvent MOFEM_EVENT_TsCtxMonitor
BasicMethodsSequence & getPostProcessRHSFunction()
Get the postProcess to do RHSFunction object.
BasicMethodsSequence & getPostProcessIJacobian()
Get the postProcess to do IJacobian object.
PetscErrorCode TsSetI2Jacobian(TS ts, PetscReal t, Vec u, Vec u_t, Vec u_tt, PetscReal a, PetscReal aa, Mat A, Mat B, void *ctx)
Calculation Jacobian for second order PDE in time.
boost::movelib::unique_ptr< bool > vecAssembleSwitch
FTensor::Index< 'J', DIM1 > J
MoFEM::FEMethodsSequence FEMethodsSequence
Custom TSAdaptivity in MoFEM.
FEMethodsSequence loopsIJacobian
Deprecated interface functions.
MoFEMTypes bH
If set to MF_EXIST check if element exist.
FEMethodsSequence loopsRHSJacobian
DeprecatedCoreInterface Interface
PetscErrorCode TsMonitorSet(TS ts, PetscInt step, PetscReal t, Vec u, void *ctx)
Set monitor for TS solver.
PetscErrorCode TSAdaptResetMoFEM(TSAdapt adapt)
friend PetscErrorCode TsSetRHSJacobian(TS ts, PetscReal t, Vec u, Mat A, Mat B, void *ctx)
TS solver function.
BasicMethodsSequence postProcessIJacobian
BasicMethodsSequence & getPostProcessRHSJacobian()
Get the postProcess to do RHSJacobian object.
implementation of Data Operators for Forces and Sources
friend PetscErrorCode TsSetIFunction(TS ts, PetscReal t, Vec u, Vec u_t, Vec F, void *ctx)
Set IFunction for TS solver.
MoFEM::Interface & mField
BasicMethodsSequence & getPreProcessIJacobian()
Get the preProcess to do IJacobian object.
MoFEM::BasicMethodsSequence BasicMethodsSequence
BasicMethodsSequence postProcessMonitor
friend PetscErrorCode TsMonitorSet(TS ts, PetscInt step, PetscReal t, Vec u, void *ctx)
Set monitor for TS solver.
FEMethodsSequence loopsMonitor
std::deque< BasicMethodPtr > BasicMethodsSequence
BasicMethodsSequence & getPreProcessMonitor()
Get the preProcess to do Monitor object.
Interface for Time Stepping (TS) solver.
BasicMethodsSequence & getPostProcessIFunction()
Get the postProcess to do IFunction object.
boost::movelib::unique_ptr< bool > matAssembleSwitch
BasicMethodsSequence preProcessMonitor
BasicMethodsSequence postProcessIFunction
PetscLogEvent MOFEM_EVENT_TsCtxRHSFunction
BasicMethodsSequence & getPreProcessRHSJacobian()
Get the preProcess to do RHSJacobian object.
BasicMethodsSequence preProcessIFunction
FEMethodsSequence loopsIFunction
PetscErrorCode TsSetIJacobian(TS ts, PetscReal t, Vec u, Vec u_t, PetscReal a, Mat A, Mat B, void *ctx)
Set function evaluating jacobian in TS solver.
BasicMethodsSequence preProcessIJacobian
MoFEM::PairNameFEMethodPtr PairNameFEMethodPtr
constexpr double t
plate stiffness
FEMethodsSequence loopsRHSFunction
PetscLogEvent MOFEM_EVENT_TsCtxRHSJacobian
FEMethodsSequence & getLoopsIJacobian()
Get the loops to do IJacobian object.
PetscErrorCode TSAdaptChooseMoFEM(TSAdapt adapt, TS ts, PetscReal h, PetscInt *next_sc, PetscReal *next_h, PetscBool *accept, PetscReal *wlte, PetscReal *wltea, PetscReal *wlter)
friend PetscErrorCode TsSetIJacobian(TS ts, PetscReal t, Vec u, Vec U_t, PetscReal a, Mat A, Mat B, void *ctx)
Set function evaluating jacobian in TS solver.
BasicMethodsSequence & getPreProcessIFunction()
Get the preProcess to do IFunction object.
friend PetscErrorCode TsSetI2Jacobian(TS ts, PetscReal t, Vec U, Vec U_t, Vec U_tt, PetscReal v, PetscReal a, Mat J, Mat P, void *ctx)
Calculation Jacobian for second order PDE in time.
BasicMethodsSequence postProcessRHSFunction
const double v
phase velocity of light in medium (cm/ns)
PetscLogEvent MOFEM_EVENT_TsCtxI2Jacobian
const FTensor::Tensor2< T, Dim, Dim > Vec
MoFEMTypes
Those types control how functions respond on arguments, f.e. error handling.
BasicMethodsSequence postProcessRHSJacobian
PetscErrorCode TsSetRHSJacobian(TS ts, PetscReal t, Vec u, Mat A, Mat B, void *ctx)
TS solver function.
friend PetscErrorCode TsSetI2Function(TS ts, PetscReal t, Vec U, Vec U_t, Vec U_tt, Vec F, void *ctx)
Calculation the right hand side for second order PDE in time.
PetscErrorCode TsSetRHSFunction(TS ts, PetscReal t, Vec u, Vec F, void *ctx)
TS solver function.
PetscErrorCode TsSetI2Function(TS ts, PetscReal t, Vec u, Vec u_t, Vec u_tt, Vec F, void *ctx)
Calculation the right hand side for second order PDE in time.
TsCtx(MoFEM::Interface &m_field, const std::string &problem_name)
BasicMethodsSequence & getPreProcessRHSFunction()
Get the preProcess to do RHSFunction object.
BasicMethodsSequence preProcessRHSFunction
PetscErrorCode TSAdaptCreateMoFEM(TSAdapt adapt)
Craete MOFEM adapt.