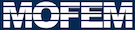 |
| v0.14.0
|
Go to the documentation of this file.
11 using namespace MoFEM;
13 static char help[] =
"...\n\n";
31 PETSC>::LinearForm<GAUSS>::OpSource<1, 1>;
58 CHKERR boundaryCondition();
71 CHKERR mField.getInterface(simpleInterface);
72 CHKERR simpleInterface->getOptions();
73 CHKERR simpleInterface->loadFile();
83 CHKERR simpleInterface->addDomainField(
"P_REAL",
H1,
85 CHKERR simpleInterface->addDomainField(
"P_IMAG",
H1,
87 CHKERR simpleInterface->addBoundaryField(
"P_REAL",
H1,
89 CHKERR simpleInterface->addBoundaryField(
"P_IMAG",
H1,
93 CHKERR simpleInterface->setFieldOrder(
"P_REAL",
order);
94 CHKERR simpleInterface->setFieldOrder(
"P_IMAG",
order);
95 CHKERR simpleInterface->setUp();
104 auto get_ents_on_mesh_skin = [&]() {
105 Range boundary_entities;
107 std::string entity_name = it->getName();
108 if (entity_name.compare(0, 2,
"BC") == 0) {
109 CHKERR it->getMeshsetIdEntitiesByDimension(mField.get_moab(), 1,
110 boundary_entities,
true);
114 Range boundary_vertices;
115 CHKERR mField.get_moab().get_connectivity(boundary_entities,
116 boundary_vertices,
true);
117 boundary_entities.merge(boundary_vertices);
119 return boundary_entities;
122 auto mark_boundary_dofs = [&](
Range &&skin_edges) {
124 auto marker_ptr = boost::make_shared<std::vector<unsigned char>>();
125 problem_manager->
markDofs(simpleInterface->getProblemName(),
ROW,
126 skin_edges, *marker_ptr);
130 auto remove_dofs_from_problem = [&](
Range &&skin_edges) {
133 CHKERR problem_manager->removeDofsOnEntities(
134 simpleInterface->getProblemName(),
"P_IMAG", skin_edges, 0, 1);
141 boundaryMarker = mark_boundary_dofs(get_ents_on_mesh_skin());
142 CHKERR remove_dofs_from_problem(get_ents_on_mesh_skin());
162 auto set_domain = [&]() {
168 new OpSetBc(
"P_REAL",
true, boundaryMarker));
186 auto set_boundary = [&]() {
192 new OpSetBc(
"P_REAL",
true, boundaryMarker));
200 new OpSetBc(
"P_REAL",
false, boundaryMarker));
206 new OpSetBc(
"P_REAL",
false, boundaryMarker));
228 CHKERR KSPSetFromOptions(solver);
231 auto dm = simpleInterface->getDM();
236 CHKERR VecGhostUpdateBegin(
D, INSERT_VALUES, SCATTER_FORWARD);
237 CHKERR VecGhostUpdateEnd(
D, INSERT_VALUES, SCATTER_FORWARD);
254 auto post_proc_fe = boost::make_shared<PostProcEle>(mField);
256 auto p_real_ptr = boost::make_shared<VectorDouble>();
257 auto p_imag_ptr = boost::make_shared<VectorDouble>();
259 post_proc_fe->getOpPtrVector().push_back(
261 post_proc_fe->getOpPtrVector().push_back(
266 post_proc_fe->getOpPtrVector().push_back(
270 post_proc_fe->getPostProcMesh(), post_proc_fe->getMapGaussPts(),
272 {{
"P_REAL", p_real_ptr}, {
"P_IMAG", p_imag_ptr}},
282 CHKERR post_proc_fe->writeFile(
"out_helmholtz.h5m");
296 CHKERR VecNorm(
D, NORM_2, &nrm2);
298 << std::setprecision(9) <<
"Solution norm " << nrm2;
300 PetscBool test_flg = PETSC_FALSE;
303 constexpr
double regression_test = 97.261672;
304 constexpr
double eps = 1e-6;
305 if (std::abs(nrm2 - regression_test) / regression_test >
eps)
307 "Not converged solution");
313 int main(
int argc,
char *argv[]) {
316 const char param_file[] =
"param_file.petsc";
322 DMType dm_name =
"DMMOFEM";
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
MoFEMErrorCode checkResults()
[Postprocess results]
int main(int argc, char *argv[])
[Check results]
boost::shared_ptr< FEMethod > & getDomainRhsFE()
MoFEMErrorCode assembleSystem()
[Push operators to pipeline]
boost::shared_ptr< std::vector< unsigned char > > boundaryMarker
Example(MoFEM::Interface &m_field)
Problem manager is used to build and partition problems.
MoFEMErrorCode loopFiniteElements(SmartPetscObj< DM > dm=nullptr)
Iterate finite elements.
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
boost::shared_ptr< FEMethod > & getBoundaryLhsFE()
PipelineManager interface.
PetscErrorCode DMoFEMMeshToLocalVector(DM dm, Vec l, InsertMode mode, ScatterMode scatter_mode)
set local (or ghosted) vector values on mesh for partition only
boost::shared_ptr< FEMethod > & getBoundaryRhsFE()
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
Simple interface for fast problem set-up.
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::BiLinearForm< GAUSS >::OpMass< 1, FIELD_DIM > OpDomainMass
Deprecated interface functions.
FormsIntegrators< BoundaryEleOp >::Assembly< PETSC >::BiLinearForm< GAUSS >::OpMass< 1, SPACE_DIM > OpBoundaryMass
[Only used with Hencky/nonlinear material]
boost::ptr_deque< UserDataOperator > & getOpBoundaryLhsPipeline()
Get the Op Boundary Lhs Pipeline object.
DeprecatedCoreInterface Interface
MoFEMErrorCode readMesh()
[Run problem]
MoFEMErrorCode getDM(DM *dm)
Get DM.
#define CHKERR
Inline error check.
auto createDMVector(DM dm)
Get smart vector from DM.
implementation of Data Operators for Forces and Sources
FormsIntegrators< EdgeEleOp >::Assembly< PETSC >::LinearForm< GAUSS >::OpSource< 1, 1 > OpBoundarySource
OpPostProcMapInMoab< SPACE_DIM, SPACE_DIM > OpPPMap
MoFEMErrorCode solveSystem()
[Solve]
Get value at integration points for scalar field.
SmartPetscObj< KSP > createKSP(SmartPetscObj< DM > dm=nullptr)
Create KSP (linear) solver.
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
MoFEMErrorCode markDofs(const std::string problem_name, RowColData rc, const enum MarkOP op, const Range ents, std::vector< unsigned char > &marker) const
Create vector with marked indices.
MoFEMErrorCode setBoundaryLhsIntegrationRule(RuleHookFun rule)
boost::ptr_deque< UserDataOperator > & getOpDomainLhsPipeline()
Get the Op Domain Lhs Pipeline object.
friend class UserDataOperator
@ AINSWORTH_BERNSTEIN_BEZIER_BASE
Add operators pushing bases from local to physical configuration.
default operator for EDGE element
MoFEMErrorCode setDomainLhsIntegrationRule(RuleHookFun rule)
boost::shared_ptr< FEMethod > & getDomainLhsFE()
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define MOFEM_LOG(channel, severity)
Log.
SmartPetscObj< Vec > vectorDuplicate(Vec vec)
Create duplicate vector of smart vector.
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::BiLinearForm< GAUSS >::OpGradGrad< 1, 1, SPACE_DIM > OpDomainGradGrad
#define CATCH_ERRORS
Catch errors.
#define _IT_CUBITMESHSETS_BY_SET_TYPE_FOR_LOOP_(MESHSET_MANAGER, CUBITBCTYPE, IT)
Iterator that loops over a specific Cubit MeshSet having a particular BC meshset in a moFEM field.
MoFEMErrorCode setupProblem()
[Run problem]
ForcesAndSourcesCore::UserDataOperator UserDataOperator
MoFEMErrorCode boundaryCondition()
[Set up problem]
Set indices on entities on finite element.
@ MOFEM_DATA_INCONSISTENCY
boost::ptr_deque< UserDataOperator > & getOpBoundaryRhsPipeline()
Get the Op Boundary Rhs Pipeline object.
MoFEMErrorCode runProblem()
[Run problem]
const double D
diffusivity
MoFEMErrorCode setBoundaryRhsIntegrationRule(RuleHookFun rule)
MoFEM::Interface & mField
PetscErrorCode PetscOptionsGetScalar(PetscOptions *, const char pre[], const char name[], PetscScalar *dval, PetscBool *set)
ElementsAndOps< SPACE_DIM >::DomainEle DomainEle
FTensor::Index< 'k', 3 > k
MoFEMErrorCode outputResults()
[Solve]
PetscErrorCode PetscOptionsGetInt(PetscOptions *, const char pre[], const char name[], PetscInt *ivalue, PetscBool *set)
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
FormsIntegrators< DomainEleOp >::Assembly< PETSC >::BiLinearForm< GAUSS >::OpMass< 1, 1 > OpDomainMass
FormsIntegrators< EdgeEleOp >::Assembly< PETSC >::BiLinearForm< GAUSS >::OpMass< 1, 1 > OpBoundaryMass
PetscErrorCode PetscOptionsGetBool(PetscOptions *, const char pre[], const char name[], PetscBool *bval, PetscBool *set)
Post post-proc data at points from hash maps.