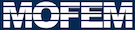 |
| v0.14.0
|
structure to get information form mofem into EntitiesFieldData
More...
#include <src/finite_elements/ForcesAndSourcesCore.hpp>
|
typedef boost::function< int(int order_row, int order_col, int order_data)> | RuleHookFun |
|
typedef boost::function< MoFEMErrorCode(ForcesAndSourcesCore *fe_raw_ptr, int order_row, int order_col, int order_data)> | GaussHookFun |
|
enum | KSPContext { CTX_SETFUNCTION,
CTX_OPERATORS,
CTX_KSPNONE
} |
| pass information about context of KSP/DM for with finite element is computed More...
|
|
enum | DataContext {
CTX_SET_NONE = 0,
CTX_SET_F = 1 << 0,
CTX_SET_A = 1 << 1,
CTX_SET_B = 1 << 2,
CTX_SET_X = 1 << 3,
CTX_SET_X_T = 1 << 4,
CTX_SET_X_TT = 1 << 6,
CTX_SET_TIME = 1 << 7
} |
|
using | Switches = std::bitset< 8 > |
|
enum | SNESContext { CTX_SNESSETFUNCTION,
CTX_SNESSETJACOBIAN,
CTX_SNESNONE
} |
|
enum | TSContext {
CTX_TSSETRHSFUNCTION,
CTX_TSSETRHSJACOBIAN,
CTX_TSSETIFUNCTION,
CTX_TSSETIJACOBIAN,
CTX_TSTSMONITORSET,
CTX_TSNONE
} |
|
|
MoFEMErrorCode | getEntitySense (const EntityType type, boost::ptr_vector< EntitiesFieldData::EntData > &data) const |
| get sense (orientation) of entity More...
|
|
MoFEMErrorCode | getEntityDataOrder (const EntityType type, const FieldSpace space, boost::ptr_vector< EntitiesFieldData::EntData > &data) const |
| Get the entity data order. More...
|
|
template<EntityType type> |
MoFEMErrorCode | getEntitySense (EntitiesFieldData &data) const |
| Get the entity sense (orientation) More...
|
|
template<EntityType type> |
MoFEMErrorCode | getEntityDataOrder (EntitiesFieldData &data, const FieldSpace space) const |
| Get the entity data order for given space. More...
|
|
MoFEMErrorCode | getFaceNodes (EntitiesFieldData &data) const |
| Get nodes on faces. More...
|
|
MoFEMErrorCode | getSpacesAndBaseOnEntities (EntitiesFieldData &data) const |
| Get field approximation space and base on entities. More...
|
|
virtual int | getRule (int order_row, int order_col, int order_data) |
| another variant of getRule More...
|
|
virtual MoFEMErrorCode | setGaussPts (int order_row, int order_col, int order_data) |
| set user specific integration rule More...
|
|
MoFEMErrorCode | calHierarchicalBaseFunctionsOnElement (const FieldApproximationBase b) |
| Calculate base functions. More...
|
|
MoFEMErrorCode | calHierarchicalBaseFunctionsOnElement () |
| Calculate base functions. More...
|
|
MoFEMErrorCode | calBernsteinBezierBaseFunctionsOnElement () |
| Calculate Bernstein-Bezier base. More...
|
|
MoFEMErrorCode | createDataOnElement (EntityType type) |
| Create a entity data on element object. More...
|
|
MoFEMErrorCode | loopOverOperators () |
| Iterate user data operators. More...
|
|
|
template<typename EXTRACTOR > |
MoFEMErrorCode | getNodesIndices (const int bit_number, FieldEntity_vector_view &ents_field, VectorInt &nodes_indices, VectorInt &local_nodes_indices, EXTRACTOR &&extractor) const |
| get node indices More...
|
|
MoFEMErrorCode | getRowNodesIndices (EntitiesFieldData &data, const int bit_number) const |
| get row node indices from FENumeredDofEntity_multiIndex More...
|
|
MoFEMErrorCode | getColNodesIndices (EntitiesFieldData &data, const int bit_number) const |
| get col node indices from FENumeredDofEntity_multiIndex More...
|
|
template<typename EXTRACTOR > |
MoFEMErrorCode | getEntityIndices (EntitiesFieldData &data, const int bit_number, FieldEntity_vector_view &ents_field, const EntityType type_lo, const EntityType type_hi, EXTRACTOR &&extractor) const |
|
MoFEMErrorCode | getEntityRowIndices (EntitiesFieldData &data, const int bit_number, const EntityType type_lo=MBVERTEX, const EntityType type_hi=MBPOLYHEDRON) const |
|
MoFEMErrorCode | getEntityColIndices (EntitiesFieldData &data, const int bit_number, const EntityType type_lo=MBVERTEX, const EntityType type_hi=MBPOLYHEDRON) const |
|
MoFEMErrorCode | getNoFieldIndices (const int bit_number, boost::shared_ptr< FENumeredDofEntity_multiIndex > dofs, VectorInt &nodes_indices) const |
| get NoField indices More...
|
|
MoFEMErrorCode | getNoFieldRowIndices (EntitiesFieldData &data, const int bit_number) const |
| get col NoField indices More...
|
|
MoFEMErrorCode | getNoFieldColIndices (EntitiesFieldData &data, const int bit_number) const |
| get col NoField indices More...
|
|
|
MoFEMErrorCode | getBitRefLevelOnData () |
|
MoFEMErrorCode | getNoFieldFieldData (const int bit_number, VectorDouble &ent_field_data, VectorDofs &ent_field_dofs, VectorFieldEntities &ent_field) const |
| Get field data on nodes. More...
|
|
MoFEMErrorCode | getNoFieldFieldData (EntitiesFieldData &data, const int bit_number) const |
|
MoFEMErrorCode | getNodesFieldData (EntitiesFieldData &data, const int bit_number) const |
| Get data on nodes. More...
|
|
MoFEMErrorCode | getEntityFieldData (EntitiesFieldData &data, const int bit_number, const EntityType type_lo=MBVERTEX, const EntityType type_hi=MBPOLYHEDRON) const |
|
|
MoFEMErrorCode | getProblemNodesIndices (const std::string &field_name, const NumeredDofEntity_multiIndex &dofs, VectorInt &nodes_indices) const |
| get indices of nodal indices which are declared for problem but not this particular element More...
|
|
MoFEMErrorCode | getProblemTypeIndices (const std::string &field_name, const NumeredDofEntity_multiIndex &dofs, EntityType type, int side_number, VectorInt &indices) const |
| get indices by type (generic function) which are declared for problem but not this particular element More...
|
|
MoFEMErrorCode | getProblemNodesRowIndices (const std::string &field_name, VectorInt &nodes_indices) const |
|
MoFEMErrorCode | getProblemTypeRowIndices (const std::string &field_name, EntityType type, int side_number, VectorInt &indices) const |
|
MoFEMErrorCode | getProblemNodesColIndices (const std::string &field_name, VectorInt &nodes_indices) const |
|
MoFEMErrorCode | getProblemTypeColIndices (const std::string &field_name, EntityType type, int side_number, VectorInt &indices) const |
|
|
virtual int | getRule (int order) |
|
virtual MoFEMErrorCode | setGaussPts (int order) |
|
◆ GaussHookFun
◆ RuleHookFun
◆ ForcesAndSourcesCore()
MoFEM::ForcesAndSourcesCore::ForcesAndSourcesCore |
( |
Interface & |
m_field | ) |
|
Definition at line 40 of file ForcesAndSourcesCore.cpp.
46 boost::make_shared<EntitiesFieldData>(MBENTITYSET),
47 boost::make_shared<EntitiesFieldData>(MBENTITYSET),
48 boost::make_shared<EntitiesFieldData>(MBENTITYSET),
49 boost::make_shared<EntitiesFieldData>(MBENTITYSET),
50 boost::make_shared<EntitiesFieldData>(MBENTITYSET),
51 boost::make_shared<EntitiesFieldData>(MBENTITYSET)
57 boost::make_shared<DerivedEntitiesFieldData>(
60 boost::make_shared<DerivedEntitiesFieldData>(
62 boost::make_shared<DerivedEntitiesFieldData>(
◆ calBernsteinBezierBaseFunctionsOnElement()
MoFEMErrorCode MoFEM::ForcesAndSourcesCore::calBernsteinBezierBaseFunctionsOnElement |
( |
| ) |
|
|
protected |
Calculate Bernstein-Bezier base.
- Returns
- MoFEMErrorCode
Definition at line 1132 of file ForcesAndSourcesCore.cpp.
1137 auto get_nodal_base_data = [&](EntitiesFieldData &data,
auto field_ptr) {
1139 auto &space = data.dataOnEntities[MBVERTEX][0].getSpace();
1140 auto &base = data.dataOnEntities[MBVERTEX][0].getBase();
1141 auto &bb_node_order = data.dataOnEntities[MBVERTEX][0].getBBNodeOrder();
1144 auto bit_number = field_ptr->getBitNumber();
1146 bit_number, get_id_for_min_type<MBVERTEX>());
1147 auto lo = std::lower_bound(field_ents.begin(), field_ents.end(), lo_uid,
1149 if (lo != field_ents.end()) {
1151 bit_number, get_id_for_max_type<MBVERTEX>());
1152 auto hi = std::upper_bound(lo, field_ents.end(), hi_uid,
cmp_uid_hi);
1155 for (
auto it = lo; it != hi; ++it)
1156 if (
auto first_e = it->lock()) {
1157 space = first_e->getSpace();
1158 base = first_e->getApproxBase();
1160 bb_node_order.resize(num_nodes,
false);
1161 bb_node_order.clear();
1163 std::vector<boost::weak_ptr<FieldEntity>> brother_ents_vec;
1165 for (; it != hi; ++it) {
1166 if (
auto e = it->lock()) {
1167 const auto &sn = e->getSideNumberPtr();
1168 const int side_number = sn->side_number;
1169 const int brother_side_number = sn->brother_side_number;
1170 if (brother_side_number != -1)
1171 brother_ents_vec.emplace_back(e);
1172 bb_node_order[side_number] = e->getMaxOrder();
1176 for (
auto &it : brother_ents_vec) {
1177 if (
const auto e = it.lock()) {
1178 const auto &sn = e->getSideNumberPtr();
1179 const int side_number = sn->side_number;
1180 const int brother_side_number = sn->brother_side_number;
1181 bb_node_order[brother_side_number] = bb_node_order[side_number];
1193 auto get_entity_base_data = [&](EntitiesFieldData &data,
auto field_ptr,
1194 const EntityType type_lo,
1195 const EntityType type_hi) {
1197 for (EntityType
t = MBEDGE;
t != MBPOLYHEDRON; ++
t) {
1198 for (
auto &dat : data.dataOnEntities[
t]) {
1202 dat.getFieldData().resize(0,
false);
1203 dat.getFieldDofs().resize(0,
false);
1208 auto bit_number = field_ptr->getBitNumber();
1211 auto lo = std::lower_bound(field_ents.begin(), field_ents.end(), lo_uid,
1213 if (lo != field_ents.end()) {
1216 auto hi = std::upper_bound(lo, field_ents.end(), hi_uid,
cmp_uid_hi);
1218 std::vector<boost::weak_ptr<FieldEntity>> brother_ents_vec;
1219 for (; lo != hi; ++lo) {
1220 if (
auto e = lo->lock()) {
1221 if (
auto cache = e->entityCacheDataDofs.lock()) {
1222 if (cache->loHi[0] != cache->loHi[1]) {
1223 if (
const auto side = e->getSideNumberPtr()->side_number;
1225 const EntityType
type = e->getEntType();
1226 auto &dat = data.dataOnEntities[
type][side];
1227 const int brother_side =
1228 e->getSideNumberPtr()->brother_side_number;
1229 if (brother_side != -1)
1230 brother_ents_vec.emplace_back(e);
1231 dat.getBase() = e->getApproxBase();
1232 dat.getSpace() = e->getSpace();
1233 const auto ent_order = e->getMaxOrder();
1235 dat.getOrder() > ent_order ? dat.getOrder() : ent_order;
1242 for (
auto &ent_ptr : brother_ents_vec) {
1243 if (
auto e = ent_ptr.lock()) {
1244 const EntityType
type = e->getEntType();
1245 const int side = e->getSideNumberPtr()->side_number;
1246 const int brother_side = e->getSideNumberPtr()->brother_side_number;
1247 auto &dat = data.dataOnEntities[
type][side];
1248 auto &dat_brother = data.dataOnEntities[
type][brother_side];
1249 dat_brother.getBase() = dat.getBase();
1250 dat_brother.getSpace() = dat.getSpace();
1251 dat_brother.getOrder() = dat.getOrder();
1259 if (
auto ent_data_ptr = e.lock()) {
1261 auto space = ent_data_ptr->getSpace();
1262 for (EntityType
t = MBVERTEX;
t != MBPOLYHEDRON; ++
t) {
1264 for (
auto &ptr : dat.getBBAlphaIndicesByOrderArray())
1266 for (
auto &ptr : dat.getBBNByOrderArray())
1268 for (
auto &ptr : dat.getBBDiffNByOrderArray())
1276 auto check_space = [&](
const auto space) {
1279 for (
auto t = MBVERTEX;
t <= ele_type; ++
t) {
1285 for (
auto t = MBEDGE;
t <= ele_type; ++
t) {
1291 for (
auto t = MBTRI;
t <= ele_type; ++
t) {
1304 std::set<string> fields_list;
1306 if (
auto ent_data_ptr = e.lock()) {
1309 if (fields_list.find(
field_name) == fields_list.end()) {
1310 auto field_ptr = ent_data_ptr->getFieldRawPtr();
1311 auto space = ent_data_ptr->getSpace();
1315 if (check_space(space)) {
1317 if (ent_data_ptr->getContinuity() !=
CONTINUOUS)
1319 "Broken space not implemented");
1323 boost::make_shared<EntPolynomialBaseCtx>(
◆ calHierarchicalBaseFunctionsOnElement() [1/2]
MoFEMErrorCode MoFEM::ForcesAndSourcesCore::calHierarchicalBaseFunctionsOnElement |
( |
| ) |
|
|
protected |
Calculate base functions.
- Returns
- Error code
Use the some node base. Node base is usually used for construction other bases.
Definition at line 1112 of file ForcesAndSourcesCore.cpp.
1119 dataOnElement[space]->dataOnEntities[MBVERTEX][0].getDiffNSharedPtr(
◆ calHierarchicalBaseFunctionsOnElement() [2/2]
Calculate base functions.
- Returns
- Error code
Definition at line 1049 of file ForcesAndSourcesCore.cpp.
1064 auto get_elem_base = [&](
auto base) {
1069 "Functions generating approximation base not defined");
1075 "Functions generating user approximation base not defined");
1080 auto get_ctx = [&](
auto space,
auto base,
auto continuity) {
1081 return boost::make_shared<EntPolynomialBaseCtx>(
1087 return elem_base->getValue(
gaussPts, ctx);
1090 for (
int space =
H1; space !=
LASTSPACE; ++space) {
1096 "Discontinuous and continuous bases on the same space");
◆ createDataOnElement()
MoFEMErrorCode MoFEM::ForcesAndSourcesCore::createDataOnElement |
( |
EntityType |
type | ) |
|
|
protected |
◆ getBitRefLevelOnData()
Get bit ref level in entities, and set it to data
Definition at line 595 of file ForcesAndSourcesCore.cpp.
600 for (
auto &dat : data->dataOnEntities) {
601 for (
auto &ent_dat : dat) {
602 ent_dat.getEntDataBitRefLevel().clear();
609 for (
auto it : field_ents) {
610 if (
auto e = it.lock()) {
613 const EntityType
type = e->getEntType();
614 const signed char side = e->getSideNumberPtr()->side_number;
617 if (
type == MBVERTEX) {
618 auto &dat = data->dataOnEntities[
type][0];
620 dat.getEntDataBitRefLevel()[side] = e->getBitRefLevel();
622 auto &dat = data->dataOnEntities[
type][side];
623 dat.getEntDataBitRefLevel().resize(1,
false);
624 dat.getEntDataBitRefLevel()[0] = e->getBitRefLevel();
630 auto &dat = data->dataOnEntities[MBENTITYSET][0];
631 dat.getEntDataBitRefLevel().resize(1,
false);
632 dat.getEntDataBitRefLevel()[0] = e->getBitRefLevel();
◆ getColNodesIndices()
get col node indices from FENumeredDofEntity_multiIndex
Definition at line 328 of file ForcesAndSourcesCore.cpp.
332 boost::weak_ptr<EntityCacheNumeredDofs>
333 operator()(boost::shared_ptr<FieldEntity> &e) {
334 return e->entityCacheColDofs;
339 data.dataOnEntities[MBVERTEX][0].getIndices(),
340 data.dataOnEntities[MBVERTEX][0].getLocalIndices(),
◆ getDataOnElementBySpaceArray()
auto& MoFEM::ForcesAndSourcesCore::getDataOnElementBySpaceArray |
( |
| ) |
|
|
inline |
Get data on entities and space.
Entities data are stored by space, by entity type, and entity side.
- Returns
- std::array<boost::shared_ptr<EntitiesFieldData>, LASTSPACE>
Definition at line 156 of file ForcesAndSourcesCore.hpp.
◆ getDerivedDataOnElementBySpaceArray()
auto& MoFEM::ForcesAndSourcesCore::getDerivedDataOnElementBySpaceArray |
( |
| ) |
|
|
inline |
Get derived data on entities and space.
Entities data are stored by space, by entity type, and entity side. Derived data is used to store data on columns, so it shares information about shape functions wih rows.
- Returns
- std::array<boost::shared_ptr<EntitiesFieldData>, LASTSPACE>
Definition at line 167 of file ForcesAndSourcesCore.hpp.
◆ getElementPolynomialBase()
auto& MoFEM::ForcesAndSourcesCore::getElementPolynomialBase |
( |
| ) |
|
|
inline |
Get the Entity Polynomial Base object.
- Returns
- boost::shared_ptr<BaseFunction>&&
Definition at line 90 of file ForcesAndSourcesCore.hpp.
◆ getEntData()
auto& MoFEM::ForcesAndSourcesCore::getEntData |
( |
const FieldSpace |
space, |
|
|
const EntityType |
type, |
|
|
const int |
side |
|
) |
| |
|
inline |
◆ getEntityColIndices()
MoFEMErrorCode MoFEM::ForcesAndSourcesCore::getEntityColIndices |
( |
EntitiesFieldData & |
data, |
|
|
const int |
bit_number, |
|
|
const EntityType |
type_lo = MBVERTEX , |
|
|
const EntityType |
type_hi = MBPOLYHEDRON |
|
) |
| const |
|
protected |
Definition at line 427 of file ForcesAndSourcesCore.cpp.
432 boost::weak_ptr<EntityCacheNumeredDofs>
433 operator()(boost::shared_ptr<FieldEntity> &e) {
434 return e->entityCacheColDofs;
◆ getEntityDataOrder() [1/2]
Get the entity data order.
- Parameters
-
- Returns
- MoFEMErrorCode
Definition at line 139 of file ForcesAndSourcesCore.cpp.
144 auto set_order = [&]() {
148 for (
unsigned int s = 0; s != data.size(); ++s)
149 data[s].getOrder() = 0;
153 for (
auto r =
fieldsPtr->get<BitFieldId_space_mi_tag>().equal_range(space);
154 r.first !=
r.second; ++
r.first) {
156 const auto field_bit_number = (*
r.first)->getBitNumber();
159 auto lo = std::lower_bound(data_field_ent.begin(), data_field_ent.end(),
161 if (lo != data_field_ent.end()) {
165 std::upper_bound(lo, data_field_ent.end(), hi_uid,
cmp_uid_hi);
166 for (; lo != hi; ++lo) {
168 if (
auto ptr = lo->lock()) {
171 auto sit = side_table.find(e.getEnt());
172 if (sit != side_table.end()) {
174 if (
const auto side_number = side->side_number;
177 auto &dat = data[side_number];
179 dat.getOrder() > ent_order ? dat.getOrder() : ent_order;
183 "Entity on side of the element not found");
192 auto set_order_on_brother = [&]() {
197 if (sit != side_table.end()) {
199 for (; sit != hi_sit; ++sit) {
200 const int brother_side_number = (*sit)->brother_side_number;
201 if (brother_side_number != -1) {
202 const int side_number = (*sit)->side_number;
203 data[brother_side_number].getOrder() = data[side_number].getOrder();
211 CHKERR set_order_on_brother();
◆ getEntityDataOrder() [2/2]
template<EntityType type>
Get the entity data order for given space.
- Template Parameters
-
- Parameters
-
- Returns
- MoFEMErrorCode
Definition at line 213 of file ForcesAndSourcesCore.hpp.
◆ getEntityFieldData()
MoFEMErrorCode MoFEM::ForcesAndSourcesCore::getEntityFieldData |
( |
EntitiesFieldData & |
data, |
|
|
const int |
bit_number, |
|
|
const EntityType |
type_lo = MBVERTEX , |
|
|
const EntityType |
type_hi = MBPOLYHEDRON |
|
) |
| const |
|
protected |
Definition at line 770 of file ForcesAndSourcesCore.cpp.
774 for (EntityType
t = type_lo;
t != type_hi; ++
t) {
775 for (
auto &dat : data.dataOnEntities[
t]) {
779 dat.getFieldData().resize(0,
false);
780 dat.getFieldDofs().resize(0,
false);
781 dat.getFieldEntities().resize(0,
false);
788 auto lo = std::lower_bound(field_ents.begin(), field_ents.end(), lo_uid,
790 if (lo != field_ents.end()) {
793 auto hi = std::upper_bound(lo, field_ents.end(), hi_uid,
cmp_uid_hi);
796 std::vector<boost::weak_ptr<FieldEntity>> brother_ents_vec;
798 for (
auto it = lo; it != hi; ++it)
799 if (
auto e = it->lock()) {
800 auto side_ptr = e->getSideNumberPtr();
801 if (
const auto side = side_ptr->side_number; side >= 0) {
802 const EntityType
type = e->getEntType();
803 auto &dat = data.dataOnEntities[
type][side];
804 auto &ent_field = dat.getFieldEntities();
805 auto &ent_field_dofs = dat.getFieldDofs();
806 auto &ent_field_data = dat.getFieldData();
808 const int brother_side = side_ptr->brother_side_number;
809 if (brother_side != -1)
810 brother_ents_vec.emplace_back(e);
812 dat.getBase() = e->getApproxBase();
813 dat.getSpace() = e->getSpace();
814 const int ent_order = e->getMaxOrder();
816 dat.getOrder() > ent_order ? dat.getOrder() : ent_order;
818 auto ent_data = e->getEntFieldData();
819 ent_field_data.resize(ent_data.size(),
false);
820 noalias(ent_field_data) = ent_data;
821 ent_field_dofs.resize(ent_data.size(),
false);
822 std::fill(ent_field_dofs.begin(), ent_field_dofs.end(),
nullptr);
823 ent_field.resize(1,
false);
824 ent_field[0] = e.get();
825 if (
auto cache = e->entityCacheDataDofs.lock()) {
826 for (
auto dit = cache->loHi[0]; dit != cache->loHi[1]; ++dit) {
827 ent_field_dofs[(*dit)->getEntDofIdx()] =
828 reinterpret_cast<FEDofEntity *
>((*dit).get());
834 for (
auto &it : brother_ents_vec) {
835 if (
const auto e = it.lock()) {
836 const EntityType
type = e->getEntType();
837 const int side = e->getSideNumberPtr()->side_number;
838 const int brother_side = e->getSideNumberPtr()->brother_side_number;
839 auto &dat = data.dataOnEntities[
type][side];
840 auto &dat_brother = data.dataOnEntities[
type][brother_side];
841 dat_brother.getBase() = dat.getBase();
842 dat_brother.getSpace() = dat.getSpace();
843 dat_brother.getOrder() = dat.getOrder();
844 dat_brother.getFieldData() = dat.getFieldData();
845 dat_brother.getFieldDofs() = dat.getFieldDofs();
846 dat_brother.getFieldEntities() = dat.getFieldEntities();
◆ getEntityIndices()
template<typename EXTRACTOR >
Definition at line 345 of file ForcesAndSourcesCore.cpp.
351 for (EntityType
t = type_lo;
t != type_hi; ++
t) {
352 for (
auto &dat : data.dataOnEntities[
t]) {
353 dat.getIndices().resize(0,
false);
354 dat.getLocalIndices().resize(0,
false);
360 auto lo = std::lower_bound(ents_field.begin(), ents_field.end(), lo_uid,
362 if (lo != ents_field.end()) {
365 auto hi = std::upper_bound(lo, ents_field.end(), hi_uid,
cmp_uid_hi);
368 std::vector<boost::weak_ptr<FieldEntity>> brother_ents_vec;
370 for (
auto it = lo; it != hi; ++it)
371 if (
auto e = it->lock()) {
373 const EntityType
type = e->getEntType();
374 auto side_ptr = e->getSideNumberPtr();
375 if (
const auto side = side_ptr->side_number; side >= 0) {
376 const auto nb_dofs_on_ent = e->getNbDofsOnEnt();
377 const auto brother_side = side_ptr->brother_side_number;
378 auto &dat = data.dataOnEntities[
type][side];
379 auto &ent_field_indices = dat.getIndices();
380 auto &ent_field_local_indices = dat.getLocalIndices();
382 ent_field_indices.resize(nb_dofs_on_ent,
false);
383 ent_field_local_indices.resize(nb_dofs_on_ent,
false);
384 std::fill(ent_field_indices.data().begin(),
385 ent_field_indices.data().end(), -1);
386 std::fill(ent_field_local_indices.data().begin(),
387 ent_field_local_indices.data().end(), -1);
389 if (
auto cache = extractor(e).lock()) {
390 for (
auto dit = cache->loHi[0]; dit != cache->loHi[1]; ++dit) {
391 const int idx = (*dit)->getEntDofIdx();
392 ent_field_indices[idx] = (*dit)->getPetscGlobalDofIdx();
393 ent_field_local_indices[idx] = (*dit)->getPetscLocalDofIdx();
397 if (brother_side != -1) {
398 auto &dat_brother = data.dataOnEntities[
type][brother_side];
399 dat_brother.getIndices().resize(nb_dofs_on_ent,
false);
400 dat_brother.getLocalIndices().resize(nb_dofs_on_ent,
false);
401 noalias(dat_brother.getIndices()) = dat.getIndices();
402 noalias(dat_brother.getLocalIndices()) = dat.getLocalIndices();
◆ getEntityRowIndices()
MoFEMErrorCode MoFEM::ForcesAndSourcesCore::getEntityRowIndices |
( |
EntitiesFieldData & |
data, |
|
|
const int |
bit_number, |
|
|
const EntityType |
type_lo = MBVERTEX , |
|
|
const EntityType |
type_hi = MBPOLYHEDRON |
|
) |
| const |
|
protected |
Definition at line 412 of file ForcesAndSourcesCore.cpp.
417 boost::weak_ptr<EntityCacheNumeredDofs>
418 operator()(boost::shared_ptr<FieldEntity> &e) {
419 return e->entityCacheRowDofs;
◆ getEntitySense() [1/2]
get sense (orientation) of entity
- Parameters
-
type | type of entity |
data | entity data |
- Returns
- error code
Definition at line 84 of file ForcesAndSourcesCore.cpp.
91 if (sit != side_table.end()) {
93 for (; sit != hi_sit; ++sit) {
94 if (
const auto side_number = (*sit)->side_number; side_number >= 0) {
95 const int brother_side_number = (*sit)->brother_side_number;
96 const int sense = (*sit)->sense;
98 data[side_number].getSense() = sense;
99 if (brother_side_number != -1)
100 data[brother_side_number].getSense() = sense;
◆ getEntitySense() [2/2]
template<EntityType type>
Get the entity sense (orientation)
- Template Parameters
-
- Parameters
-
- Returns
- MoFEMErrorCode
Definition at line 200 of file ForcesAndSourcesCore.hpp.
◆ getFaceNodes()
Get nodes on faces.
Definition at line 921 of file ForcesAndSourcesCore.cpp.
923 auto &face_nodes = data.facesNodes;
924 auto &face_nodes_order = data.facesNodesOrder;
929 const auto nb_faces = CN::NumSubEntities(
type, 2);
933 auto side_ptr_it = side_table.get<1>().lower_bound(
934 boost::make_tuple(CN::TypeDimensionMap[2].first, 0));
935 auto hi_side_ptr_it = side_table.get<1>().upper_bound(
936 boost::make_tuple(CN::TypeDimensionMap[2].second, 100));
938 for (; side_ptr_it != hi_side_ptr_it; ++side_ptr_it) {
939 const auto side = (*side_ptr_it)->side_number;
940 const auto sense = (*side_ptr_it)->sense;
941 const auto offset = (*side_ptr_it)->offset;
943 EntityType face_type;
946 CN::SubEntityVertexIndices(
type, 2, side, face_type, nb_nodes_face);
947 face_nodes.resize(nb_faces, nb_nodes_face);
948 face_nodes_order.resize(nb_faces, nb_nodes_face);
951 for (
int n = 0;
n != nb_nodes_face; ++
n)
952 face_nodes_order(side,
n) = (
n + offset) % nb_nodes_face;
954 for (
int n = 0;
n != nb_nodes_face; ++
n)
955 face_nodes_order(side,
n) =
956 (nb_nodes_face - (
n - offset) % nb_nodes_face) % nb_nodes_face;
958 for (
int n = 0;
n != nb_nodes_face; ++
n)
959 face_nodes(side,
n) = face_indices[face_nodes_order(side,
n)];
962 const auto face_ent = (*side_ptr_it)->ent;
968 nb_nodes_face,
true);
969 face_nodes.resize(nb_faces, nb_nodes_face);
970 for (
int nn = 0; nn != nb_nodes_face; ++nn) {
971 if (face_nodes(side, nn) !=
974 std::find(conn_ele, &conn_ele[num_nodes_ele], conn_face[nn]))) {
976 "Wrong face numeration");
◆ getMaxColOrder()
int MoFEM::ForcesAndSourcesCore::getMaxColOrder |
( |
| ) |
const |
◆ getMaxDataOrder()
int MoFEM::ForcesAndSourcesCore::getMaxDataOrder |
( |
| ) |
const |
Get max order of approximation for data fields.
getMeasure getMaxDataOrder () return maximal order on entities, for all data on the element. So for example if finite element is triangle, and triangle base function have order 4 and on edges base function have order 2, this function return 4.
If finite element has for example 2 or more approximated fields, for example Pressure (order 3) and displacement field (order 5), this function returns 5.
Definition at line 120 of file ForcesAndSourcesCore.cpp.
123 if (
auto ptr = e.lock()) {
124 const int order = ptr->getMaxOrder();
125 max_order = (max_order <
order) ?
order : max_order;
◆ getMaxRowOrder()
int MoFEM::ForcesAndSourcesCore::getMaxRowOrder |
( |
| ) |
const |
◆ getNodesFieldData()
Get data on nodes.
- Parameters
-
data | Data structure |
field_name | Field name |
- Returns
- Error code
Definition at line 642 of file ForcesAndSourcesCore.cpp.
645 auto get_nodes_field_data = [&](
VectorDouble &nodes_data,
652 nodes_data.resize(0,
false);
653 nodes_dofs.resize(0,
false);
654 field_entities.resize(0,
false);
661 if ((*field_it)->getBitNumber() != bit_number)
664 const int nb_dofs_on_vert = (*field_it)->getNbOfCoeffs();
665 space = (*field_it)->getSpace();
666 base = (*field_it)->getApproxBase();
670 bit_number, get_id_for_min_type<MBVERTEX>());
671 auto lo = std::lower_bound(field_ents.begin(), field_ents.end(), lo_uid,
673 if (lo != field_ents.end()) {
675 bit_number, get_id_for_max_type<MBVERTEX>());
676 auto hi = std::upper_bound(lo, field_ents.end(), hi_uid,
cmp_uid_hi);
680 for (
auto it = lo; it != hi; ++it) {
681 if (
auto e = it->lock()) {
682 if (
auto cache = e->entityCacheDataDofs.lock()) {
683 if (cache->loHi[0] != cache->loHi[1]) {
684 nb_dofs += std::distance(cache->loHi[0], cache->loHi[1]);
694 bb_node_order.resize(num_nodes,
false);
695 bb_node_order.clear();
696 const int max_nb_dofs = nb_dofs_on_vert * num_nodes;
697 nodes_data.resize(max_nb_dofs,
false);
698 nodes_dofs.resize(max_nb_dofs,
false);
699 field_entities.resize(num_nodes,
false);
700 std::fill(nodes_data.begin(), nodes_data.end(), 0);
701 std::fill(nodes_dofs.begin(), nodes_dofs.end(),
nullptr);
702 std::fill(field_entities.begin(), field_entities.end(),
nullptr);
704 std::vector<boost::weak_ptr<FieldEntity>> brother_ents_vec;
706 for (
auto it = lo; it != hi; ++it) {
707 if (
auto e = it->lock()) {
708 const auto &sn = e->getSideNumberPtr();
711 if (
const auto side_number = sn->side_number;
713 const int brother_side_number = sn->brother_side_number;
715 field_entities[side_number] = e.get();
716 if (brother_side_number != -1) {
717 brother_ents_vec.emplace_back(e);
718 field_entities[side_number] = field_entities[side_number];
721 bb_node_order[side_number] = e->getMaxOrder();
722 int pos = side_number * nb_dofs_on_vert;
723 auto ent_filed_data_vec = e->getEntFieldData();
724 if (
auto cache = e->entityCacheDataDofs.lock()) {
725 for (
auto dit = cache->loHi[0]; dit != cache->loHi[1];
727 const auto dof_idx = (*dit)->getEntDofIdx();
728 nodes_data[pos + dof_idx] = ent_filed_data_vec[dof_idx];
729 nodes_dofs[pos + dof_idx] =
730 reinterpret_cast<FEDofEntity *
>((*dit).get());
737 for (
auto &it : brother_ents_vec) {
738 if (
const auto e = it.lock()) {
739 const auto &sn = e->getSideNumberPtr();
740 const int side_number = sn->side_number;
741 const int brother_side_number = sn->brother_side_number;
742 bb_node_order[brother_side_number] = bb_node_order[side_number];
743 int pos = side_number * nb_dofs_on_vert;
744 int brother_pos = brother_side_number * nb_dofs_on_vert;
745 for (
int ii = 0; ii != nb_dofs_on_vert; ++ii) {
746 nodes_data[brother_pos] = nodes_data[pos];
747 nodes_dofs[brother_pos] = nodes_dofs[pos];
761 return get_nodes_field_data(
762 data.dataOnEntities[MBVERTEX][0].getFieldData(),
763 data.dataOnEntities[MBVERTEX][0].getFieldEntities(),
764 data.dataOnEntities[MBVERTEX][0].getFieldDofs(),
765 data.dataOnEntities[MBVERTEX][0].getSpace(),
766 data.dataOnEntities[MBVERTEX][0].getBase(),
767 data.dataOnEntities[MBVERTEX][0].getBBNodeOrder());
◆ getNodesIndices()
template<typename EXTRACTOR >
get node indices
Definition at line 219 of file ForcesAndSourcesCore.cpp.
230 if ((*field_it)->getBitNumber() != bit_number)
234 bit_number, get_id_for_min_type<MBVERTEX>());
235 auto lo = std::lower_bound(ents_field.begin(), ents_field.end(), lo_uid,
237 if (lo != ents_field.end()) {
239 bit_number, get_id_for_max_type<MBVERTEX>());
240 auto hi = std::upper_bound(lo, ents_field.end(), hi_uid,
cmp_uid_hi);
243 const int nb_dofs_on_vert = (*field_it)->getNbOfCoeffs();
244 const int max_nb_dofs = nb_dofs_on_vert * num_nodes;
247 for (
auto it = lo; it != hi; ++it) {
248 if (
auto e = it->lock()) {
249 if (
auto cache = extractor(e).lock()) {
250 if (cache->loHi[0] != cache->loHi[1]) {
251 nb_dofs += std::distance(cache->loHi[0], cache->loHi[1]);
259 nodes_indices.resize(max_nb_dofs,
false);
260 local_nodes_indices.resize(max_nb_dofs,
false);
262 nodes_indices.resize(0,
false);
263 local_nodes_indices.resize(0,
false);
266 if (nb_dofs != max_nb_dofs) {
267 std::fill(nodes_indices.begin(), nodes_indices.end(), -1);
268 std::fill(local_nodes_indices.begin(), local_nodes_indices.end(), -1);
271 for (
auto it = lo; it != hi; ++it) {
272 if (
auto e = it->lock()) {
273 auto side_ptr = e->getSideNumberPtr();
274 if (
const auto side_number = side_ptr->side_number;
276 const auto brother_side_number = side_ptr->brother_side_number;
277 if (
auto cache = extractor(e).lock()) {
278 for (
auto dit = cache->loHi[0]; dit != cache->loHi[1]; ++dit) {
280 const int idx = dof.getPetscGlobalDofIdx();
281 const int local_idx = dof.getPetscLocalDofIdx();
283 side_number * nb_dofs_on_vert + dof.getDofCoeffIdx();
284 nodes_indices[pos] = idx;
285 local_nodes_indices[pos] = local_idx;
286 if (brother_side_number != -1) {
287 const int elem_idx = brother_side_number * nb_dofs_on_vert +
288 (*dit)->getDofCoeffIdx();
289 nodes_indices[elem_idx] = idx;
290 local_nodes_indices[elem_idx] = local_idx;
298 nodes_indices.resize(0,
false);
299 local_nodes_indices.resize(0,
false);
303 nodes_indices.resize(0,
false);
304 local_nodes_indices.resize(0,
false);
◆ getNoFieldColIndices()
get col NoField indices
Definition at line 474 of file ForcesAndSourcesCore.cpp.
478 if (data.dataOnEntities[MBENTITYSET].size() == 0) {
480 "data.dataOnEntities[MBENTITYSET] is empty");
484 data.dataOnEntities[MBENTITYSET][0].getIndices());
◆ getNoFieldFieldData() [1/2]
Get field data on nodes.
Definition at line 855 of file ForcesAndSourcesCore.cpp.
861 ent_field_data.resize(0,
false);
862 ent_field_dofs.resize(0,
false);
863 ent_field.resize(0,
false);
867 bit_number, get_id_for_min_type<MBVERTEX>());
868 auto lo = std::lower_bound(field_ents.begin(), field_ents.end(), lo_uid,
870 if (lo != field_ents.end()) {
872 ent_field.resize(field_ents.size(),
false);
873 std::fill(ent_field.begin(), ent_field.end(),
nullptr);
876 bit_number, get_id_for_max_type<MBENTITYSET>());
877 auto hi = std::upper_bound(lo, field_ents.end(), hi_uid,
cmp_uid_hi);
881 for (
auto it = lo; it != hi; ++it, ++side)
882 if (
auto e = it->lock()) {
884 const auto size = e->getNbDofsOnEnt();
885 ent_field_data.resize(size,
false);
886 ent_field_dofs.resize(size,
false);
887 ent_field[side] = e.get();
888 noalias(ent_field_data) = e->getEntFieldData();
890 if (
auto cache = e->entityCacheDataDofs.lock()) {
891 for (
auto dit = cache->loHi[0]; dit != cache->loHi[1]; ++dit) {
892 ent_field_dofs[(*dit)->getEntDofIdx()] =
893 reinterpret_cast<FEDofEntity *
>((*dit).get());
◆ getNoFieldFieldData() [2/2]
Definition at line 904 of file ForcesAndSourcesCore.cpp.
907 if (data.dataOnEntities[MBENTITYSET].size() == 0)
909 "No space to insert data");
912 bit_number, data.dataOnEntities[MBENTITYSET][0].getFieldData(),
913 data.dataOnEntities[MBENTITYSET][0].getFieldDofs(),
914 data.dataOnEntities[MBENTITYSET][0].getFieldEntities());
◆ getNoFieldIndices()
get NoField indices
Definition at line 442 of file ForcesAndSourcesCore.cpp.
446 auto dit = dofs->get<Unique_mi_tag>().lower_bound(
448 auto hi_dit = dofs->get<Unique_mi_tag>().upper_bound(
450 indices.resize(std::distance(dit, hi_dit));
451 for (; dit != hi_dit; dit++) {
452 int idx = (*dit)->getPetscGlobalDofIdx();
453 indices[(*dit)->getDofCoeffIdx()] = idx;
◆ getNoFieldRowIndices()
get col NoField indices
Definition at line 459 of file ForcesAndSourcesCore.cpp.
463 if (data.dataOnEntities[MBENTITYSET].size() == 0) {
465 "data.dataOnEntities[MBENTITYSET] is empty");
469 data.dataOnEntities[MBENTITYSET][0].getIndices());
◆ getOpPtrVector()
boost::ptr_deque<UserDataOperator>& MoFEM::ForcesAndSourcesCore::getOpPtrVector |
( |
| ) |
|
|
inline |
Use to push back operator for row operator.
It can be used to calculate nodal forces or other quantities on the mesh.
- Examples
- continuity_check_on_contact_prism_side_ele.cpp, continuity_check_on_skeleton_3d.cpp, continuity_check_on_skeleton_with_simple_2d_for_h1.cpp, continuity_check_on_skeleton_with_simple_2d_for_hcurl.cpp, continuity_check_on_skeleton_with_simple_2d_for_hdiv.cpp, elasticity_mixed_formulation.cpp, EshelbianOperators.cpp, forces_and_sources_testing_edge_element.cpp, forces_and_sources_testing_flat_prism_element.cpp, lorentz_force.cpp, MagneticElement.hpp, nonlinear_dynamics.cpp, and simple_contact.cpp.
Definition at line 83 of file ForcesAndSourcesCore.hpp.
◆ getProblemNodesColIndices()
MoFEMErrorCode MoFEM::ForcesAndSourcesCore::getProblemNodesColIndices |
( |
const std::string & |
field_name, |
|
|
VectorInt & |
nodes_indices |
|
) |
| const |
|
protected |
◆ getProblemNodesIndices()
get indices of nodal indices which are declared for problem but not this particular element
Definition at line 490 of file ForcesAndSourcesCore.cpp.
496 if (field_struture->getSpace() ==
H1) {
499 nodes_indices.resize(field_struture->getNbOfCoeffs() * num_nodes,
false);
500 std::fill(nodes_indices.begin(), nodes_indices.end(), -1);
504 auto siit = side_table.get<1>().lower_bound(boost::make_tuple(MBVERTEX, 0));
506 side_table.get<1>().lower_bound(boost::make_tuple(MBVERTEX, 10000));
509 for (; siit != hi_siit; siit++, nn++) {
510 if (siit->get()->side_number >= 0) {
513 auto dit = dofs.get<Unique_mi_tag>().lower_bound(
515 auto hi_dit = dofs.get<Unique_mi_tag>().upper_bound(
517 for (; dit != hi_dit; dit++) {
518 nodes_indices[siit->get()->side_number * (*dit)->getNbOfCoeffs() +
519 (*dit)->getDofCoeffIdx()] =
520 (*dit)->getPetscGlobalDofIdx();
525 nodes_indices.resize(0,
false);
◆ getProblemNodesRowIndices()
MoFEMErrorCode MoFEM::ForcesAndSourcesCore::getProblemNodesRowIndices |
( |
const std::string & |
field_name, |
|
|
VectorInt & |
nodes_indices |
|
) |
| const |
|
protected |
◆ getProblemTypeColIndices()
MoFEMErrorCode MoFEM::ForcesAndSourcesCore::getProblemTypeColIndices |
( |
const std::string & |
field_name, |
|
|
EntityType |
type, |
|
|
int |
side_number, |
|
|
VectorInt & |
indices |
|
) |
| const |
|
protected |
◆ getProblemTypeIndices()
get indices by type (generic function) which are declared for problem but not this particular element
Definition at line 531 of file ForcesAndSourcesCore.cpp.
541 side_table.get<1>().lower_bound(boost::make_tuple(
type, side_number));
543 side_table.get<1>().upper_bound(boost::make_tuple(
type, side_number));
545 for (; siit != hi_siit; siit++) {
546 if (siit->get()->side_number >= 0) {
550 auto dit = dofs.get<Unique_mi_tag>().lower_bound(
552 auto hi_dit = dofs.get<Unique_mi_tag>().upper_bound(
554 indices.resize(std::distance(dit, hi_dit));
555 for (; dit != hi_dit; dit++) {
557 indices[(*dit)->getEntDofIdx()] = (*dit)->getPetscGlobalDofIdx();
◆ getProblemTypeRowIndices()
MoFEMErrorCode MoFEM::ForcesAndSourcesCore::getProblemTypeRowIndices |
( |
const std::string & |
field_name, |
|
|
EntityType |
type, |
|
|
int |
side_number, |
|
|
VectorInt & |
indices |
|
) |
| const |
|
protected |
◆ getRowNodesIndices()
get row node indices from FENumeredDofEntity_multiIndex
Definition at line 311 of file ForcesAndSourcesCore.cpp.
315 boost::weak_ptr<EntityCacheNumeredDofs>
316 operator()(boost::shared_ptr<FieldEntity> &e) {
317 return e->entityCacheRowDofs;
322 data.dataOnEntities[MBVERTEX][0].getIndices(),
323 data.dataOnEntities[MBVERTEX][0].getLocalIndices(),
◆ getRule() [1/2]
int MoFEM::ForcesAndSourcesCore::getRule |
( |
int |
order | ) |
|
|
protectedvirtual |
- Deprecated:
- Use getRule(int row_order, int col_order, int data order)
Reimplemented in PostProcEdgeOnRefinedMesh, PostProcFaceOnRefinedMesh, EdgeSlidingConstrains::MyEdgeFE, BoneRemodeling::DensityMapFe, GelModule::Gel::GelFE, SurfaceSlidingConstrains::MyTriangleFE, PostProcTemplateVolumeOnRefinedMesh< MoFEM::VolumeElementForcesAndSourcesCore >, NitscheMethod::MyVolumeFE, KelvinVoigtDamper::DamperFE, MoFEM::PostProcBrokenMeshInMoabBase< FaceElementForcesAndSourcesCore >, MoFEM::PostProcBrokenMeshInMoabBase< VolumeElementForcesAndSourcesCore >, MoFEM::PostProcBrokenMeshInMoabBase< EdgeElementForcesAndSourcesCore >, MoFEM::VolumeElementForcesAndSourcesCoreOnContactPrismSide, MoFEM::VolumeElementForcesAndSourcesCoreOnSide, NitscheMethod::MyFace, ThermalElement::MyTriFE, NeumannForcesSurface::MyTriangleFE, NeumannForcesSurfaceComplexForLazy::MyTriangleSpatialFE, MixTransport::MixTransportElement::MyTriFE, NonlinearElasticElement::MyVolumeFE, MagneticElement::TriFE, CellEngineering::FaceElement, ThermalElement::MyVolumeFE, BoneRemodeling::Remodeling::Fe, MixTransport::MixTransportElement::MyVolumeFE, MagneticElement::VolumeFE, AnalyticalDirichletBC::ApproxField::MyTriFE, FluidPressure::MyTriangleFE, CellEngineering::DispMapFe, MoFEM::EdgeElementForcesAndSourcesCoreOnChildParent, MoFEM::FaceElementForcesAndSourcesCoreOnChildParent, MoFEM::FaceElementForcesAndSourcesCoreOnSide, CohesiveElement::CohesiveInterfaceElement::MyPrism, EdgeForce::MyFE, ThermalStressElement::MyVolumeFE, and BodyForceConstantField::MyVolumeFE.
Definition at line 1964 of file ForcesAndSourcesCore.cpp.
1964 {
return 2 *
order; }
◆ getRule() [2/2]
int MoFEM::ForcesAndSourcesCore::getRule |
( |
int |
order_row, |
|
|
int |
order_col, |
|
|
int |
order_data |
|
) |
| |
|
protectedvirtual |
another variant of getRule
- Parameters
-
order_row | order of base function on row |
order_col | order of base function on columns |
order_data | order of base function approximating data |
- Returns
- integration rule
This function is overloaded by the user. The integration rule is set such that specific operator implemented by the user is integrated accurately. For example if user implement bilinear operator
\[ b(u,v) = \int_\mathcal{T} \frac{\partial u_i}{\partial x_j}\frac{\partial v_i}{\partial x_j} \textrm{d}\mathcal{T} \]
then if \(u\) and \(v\) are polynomial of given order, then exact integral would be
The integration points and weights are set appropriately for given entity type and integration rule from quad.c
Method ForcesAndSourcesCore::getRule takes at argument takes maximal polynomial order set on the element on all fields defined on the element. If a user likes to have more control, another variant of this function can be called which distinguishing between field orders on rows, columns and data, the i.e. first argument of a bilinear form, the second argument of bilinear form and field coefficients on the element.
- Note
- If user set rule to -1 or any other negative integer, then method ForcesAndSourcesCore::setGaussPts is called. In that method user can implement own (specific) integration method.
- Bug:
- this function should be const
Reimplemented in EdgeFE, QuadFE, and TriFE.
Definition at line 1952 of file ForcesAndSourcesCore.cpp.
◆ getSpacesAndBaseOnEntities()
Get field approximation space and base on entities.
Definition at line 990 of file ForcesAndSourcesCore.cpp.
996 for (EntityType
t = MBVERTEX;
t != MBMAXTYPE; ++
t) {
997 data.spacesOnEntities[
t].reset();
998 data.basesOnEntities[
t].reset();
1001 data.basesOnSpaces[s].reset();
1002 data.brokenBasesOnSpaces[s].reset();
1010 if (
auto ptr = e.lock()) {
1012 const EntityType
type = ptr->getEntType();
1019 switch (continuity) {
1021 data.basesOnSpaces[space].set(approx);
1024 data.brokenBasesOnSpaces[space].set(approx);
1028 "Continuity not defined");
1031 data.bAse.set(approx);
1032 data.spacesOnEntities[
type].set(space);
1033 data.basesOnEntities[
type].set(approx);
1039 "data fields ents not allocated on element");
1041 for (
auto space = 0; space !=
LASTSPACE; ++space)
1042 if ((data.brokenBasesOnSpaces[space] & data.basesOnSpaces[space]).any())
1044 "Discontinuous and continuous bases on the same space");
◆ getUserPolynomialBase()
auto& MoFEM::ForcesAndSourcesCore::getUserPolynomialBase |
( |
| ) |
|
|
inline |
Get the User Polynomial Base object.
- Returns
- boost::shared_ptr<BaseFunction>&
Definition at line 97 of file ForcesAndSourcesCore.hpp.
◆ loopOverOperators()
Iterate user data operators.
- Returns
- MoFEMErrorCode
Definition at line 1379 of file ForcesAndSourcesCore.cpp.
1385 std::array<const Field *, 3> field_struture;
1388 std::array<FieldSpace, 2> space;
1389 std::array<FieldApproximationBase, 2> base;
1391 constexpr std::array<UDO::OpType, 2> types = {UDO::OPROW, UDO::OPCOL};
1392 std::array<int, 2> last_eval_field_id = {0, 0};
1394 std::array<boost::shared_ptr<EntitiesFieldData>, 2> op_data;
1396 auto swap_bases = [&](
auto &op) {
1398 for (
size_t ss = 0; ss != 2; ++ss) {
1399 if (op.getOpType() & types[ss] || op.getOpType() & UDO::OPROWCOL) {
1416 auto evaluate_op_space = [&](
auto &op) {
1421 std::fill(last_eval_field_id.begin(), last_eval_field_id.end(), 0);
1439 for (EntityType
t = MBVERTEX;
t != MBMAXTYPE; ++
t) {
1441 e.getSpace() = op.sPace;
1451 "Not implemented for this space", op.sPace);
1458 auto set_op_entities_data = [&](
auto ss,
auto &op) {
1462 if ((op.getNumeredEntFiniteElementPtr()->getBitFieldIdData() &
1466 "no data field < %s > on finite element < %s >",
1474 for (
auto &data : op_data[ss]->dataOnEntities[MBENTITYSET]) {
1475 CHKERR data.resetFieldDependentData();
1478 auto get_data_for_nodes = [&]() {
1489 auto get_data_for_entities = [&]() {
1499 auto get_data_for_meshset = [&]() {
1510 switch (space[ss]) {
1512 CHKERR get_data_for_nodes();
1515 CHKERR get_data_for_entities();
1520 CHKERR get_data_for_nodes();
1523 CHKERR get_data_for_entities();
1530 CHKERR get_data_for_meshset();
1535 "not implemented for this space < %s >",
1542 auto evaluate_op_for_fields = [&](
auto &op) {
1545 if (op.getOpType() & UDO::OPROW) {
1547 CHKERR op.opRhs(*op_data[0],
false);
1552 if (op.getOpType() & UDO::OPCOL) {
1554 CHKERR op.opRhs(*op_data[1],
false);
1559 if (op.getOpType() & UDO::OPROWCOL) {
1561 CHKERR op.opLhs(*op_data[0], *op_data[1]);
1575 for (; oit != hi_oit; oit++) {
1579 CHKERR oit->setPtrFE(
this);
1581 if ((oit->opType & UDO::OPSPACE) == UDO::OPSPACE) {
1584 CHKERR evaluate_op_space(*oit);
1588 (oit->opType & (UDO::OPROW | UDO::OPCOL | UDO::OPROWCOL)) ==
1596 for (
size_t ss = 0; ss != 2; ss++) {
1601 "Not set Field name in operator %d (0-row, 1-column) in "
1604 (boost::typeindex::type_id_runtime(*oit).pretty_name())
1611 space[ss] = field_struture[ss]->getSpace();
1612 base[ss] = field_struture[ss]->getApproxBase();
1617 for (
size_t ss = 0; ss != 2; ss++) {
1619 if (oit->getOpType() & types[ss] ||
1620 oit->getOpType() & UDO::OPROWCOL) {
1621 if (last_eval_field_id[ss] != field_id[ss]) {
1622 CHKERR set_op_entities_data(ss, *oit);
1623 last_eval_field_id[ss] = field_id[ss];
1631 CHKERR evaluate_op_for_fields(*oit);
1637 for (
int i = 0;
i != 5; ++
i)
1638 if (oit->opType & (1 <<
i))
1639 MOFEM_LOG(
"SELF", Sev::error) << UDO::OpTypeNames[
i];
1641 "Impossible operator type");
◆ operator()()
◆ postProcess()
function is run at the end of loop
It is used to assembly matrices and vectors, calculating global variables, f.e. total internal energy, ect.
Iterating over dofs: Example1 iterating over dofs in row by name of the field for(IT_GET_FEROW_BY_NAME_DOFS_FOR_LOOP(this,"DISPLACEMENT",it)) { ... }
Reimplemented from MoFEM::BasicMethod.
Reimplemented in PostProcEdgeOnRefinedMesh, PostProcFaceOnRefinedMesh, PostProcFatPrismOnRefinedMesh, PostProcTemplateVolumeOnRefinedMesh< MoFEM::VolumeElementForcesAndSourcesCore >, GelModule::Gel::GelFE, KelvinVoigtDamper::DamperFE, MoFEM::PostProcBrokenMeshInMoabBase< FaceElementForcesAndSourcesCore >, MoFEM::PostProcBrokenMeshInMoabBase< VolumeElementForcesAndSourcesCore >, MoFEM::PostProcBrokenMeshInMoabBase< EdgeElementForcesAndSourcesCore >, Smoother::MyVolumeFE, NonlinearElasticElement::MyVolumeFE, and EshelbianPlasticity::ContactTree.
Definition at line 2013 of file ForcesAndSourcesCore.cpp.
◆ preProcess()
function is run at the beginning of loop
It is used to zeroing matrices and vectors, calculation of shape functions on reference element, preprocessing boundary conditions, etc.
Reimplemented from MoFEM::BasicMethod.
Reimplemented in PostProcEdgeOnRefinedMesh, PostProcFaceOnRefinedMesh, PostProcFatPrismOnRefinedMesh, EdgeSlidingConstrains::MyEdgeFE, PostProcTemplateVolumeOnRefinedMesh< MoFEM::VolumeElementForcesAndSourcesCore >, GelModule::Gel::GelFE, SurfaceSlidingConstrains::MyTriangleFE, KelvinVoigtDamper::DamperFE, MoFEM::PostProcBrokenMeshInMoabBase< FaceElementForcesAndSourcesCore >, MoFEM::PostProcBrokenMeshInMoabBase< VolumeElementForcesAndSourcesCore >, MoFEM::PostProcBrokenMeshInMoabBase< EdgeElementForcesAndSourcesCore >, NeumannForcesSurfaceComplexForLazy::MyTriangleSpatialFE, Smoother::MyVolumeFE, NonlinearElasticElement::MyVolumeFE, EshelbianPlasticity::ContactTree, and FluidPressure::MyTriangleFE.
Definition at line 1990 of file ForcesAndSourcesCore.cpp.
◆ setGaussPts() [1/2]
- Deprecated:
- setGaussPts(int row_order, int col_order, int data order);
- Deprecated:
- setGaussPts(int row_order, int col_order, int data order);
Reimplemented in PostProcEdgeOnRefinedMesh, PostProcFaceOnRefinedMesh, PostProcTemplateVolumeOnRefinedMesh< MoFEM::VolumeElementForcesAndSourcesCore >, NitscheMethod::MyVolumeFE, MoFEM::PostProcBrokenMeshInMoabBase< FaceElementForcesAndSourcesCore >, MoFEM::PostProcBrokenMeshInMoabBase< VolumeElementForcesAndSourcesCore >, MoFEM::PostProcBrokenMeshInMoabBase< EdgeElementForcesAndSourcesCore >, MoFEM::VolumeElementForcesAndSourcesCoreOnContactPrismSide, MoFEM::VolumeElementForcesAndSourcesCoreOnSide, MoFEM::FaceElementForcesAndSourcesCoreOnSide, MoFEM::EdgeElementForcesAndSourcesCoreOnChildParent, and MoFEM::FaceElementForcesAndSourcesCoreOnChildParent.
Definition at line 1968 of file ForcesAndSourcesCore.cpp.
◆ setGaussPts() [2/2]
MoFEMErrorCode MoFEM::ForcesAndSourcesCore::setGaussPts |
( |
int |
order_row, |
|
|
int |
order_col, |
|
|
int |
order_data |
|
) |
| |
|
protectedvirtual |
set user specific integration rule
This function allows for user defined integration rule. The key is to called matrix gaussPts, which is used by other MoFEM procedures. Matrix has number of rows equal to problem dimension plus one, where last index is used to store weight values. Number of columns is equal to number of integration points.
- Note
- This function is called if method ForcesAndSourcesCore::getRule is returning integer -1 or any other negative integer.
User sets
where
number rows represents local coordinates of integration points in reference element, where last index in row is for integration weight.
Reimplemented in EdgeFE, QuadFE, and TriFE.
Definition at line 1958 of file ForcesAndSourcesCore.cpp.
◆ setRefineFEPtr()
◆ setSideFEPtr()
Set the pointer to face element on the side.
- Note
- Function is is used by face element, while it iterates over elements on the side
- Parameters
-
- Returns
- MoFEMErrorCode
Definition at line 1687 of file ForcesAndSourcesCore.cpp.
◆ EdgeElementForcesAndSourcesCoreOnChildParent
◆ FaceElementForcesAndSourcesCoreOnChildParent
◆ FaceElementForcesAndSourcesCoreOnSide
◆ OpBrokenLoopSide
◆ OpCopyGeomDataToE
◆ UserDataOperator
- Examples
- continuity_check_on_contact_prism_side_ele.cpp, continuity_check_on_skeleton_with_simple_2d_for_h1.cpp, continuity_check_on_skeleton_with_simple_2d_for_hcurl.cpp, continuity_check_on_skeleton_with_simple_2d_for_hdiv.cpp, forces_and_sources_testing_edge_element.cpp, free_surface.cpp, hanging_node_approx.cpp, hcurl_divergence_operator_2d.cpp, hello_world.cpp, helmholtz.cpp, mesh_smoothing.cpp, and phase.cpp.
Definition at line 482 of file ForcesAndSourcesCore.hpp.
◆ VolumeElementForcesAndSourcesCoreOnContactPrismSide
◆ VolumeElementForcesAndSourcesCoreOnSide
◆ coordsAtGaussPts
◆ dataH1
◆ dataHcurl
◆ dataHdiv
◆ dataL2
◆ dataNoField
◆ dataOnElement
◆ derivedDataOnElement
◆ elementMeasure
double MoFEM::ForcesAndSourcesCore::elementMeasure |
|
protected |
◆ elementPolynomialBasePtr
boost::shared_ptr<BaseFunction> MoFEM::ForcesAndSourcesCore::elementPolynomialBasePtr |
|
private |
◆ gaussPts
Matrix of integration points.
Columns is equal to number of integration points, numver of rows depends on dimension of finite element entity, for example for tetrahedron rows are x,y,z,weight. Last row is integration weight.
FIXME: that should be moved to private class data and acessed only by member function
- Examples
- hanging_node_approx.cpp, and prism_elements_from_surface.cpp.
Definition at line 109 of file ForcesAndSourcesCore.hpp.
◆ getRuleHook
◆ lastEvaluatedElementEntityType
EntityType MoFEM::ForcesAndSourcesCore::lastEvaluatedElementEntityType |
|
protected |
◆ mField
Interface& MoFEM::ForcesAndSourcesCore::mField |
◆ opPtrVector
◆ refinePtrFE
◆ setRuleHook
◆ sidePtrFE
◆ userPolynomialBasePtr
boost::shared_ptr<BaseFunction> MoFEM::ForcesAndSourcesCore::userPolynomialBasePtr |
|
private |
The documentation for this struct was generated from the following files:
EntitiesFieldData & dataNoField
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
auto & getRowFieldEnts() const
MoFEMErrorCode getEntityRowIndices(EntitiesFieldData &data, const int bit_number, const EntityType type_lo=MBVERTEX, const EntityType type_hi=MBPOLYHEDRON) const
#define CATCH_OP_ERRORS(OP)
MoFEMErrorCode getNoFieldFieldData(const int bit_number, VectorDouble &ent_field_data, VectorDofs &ent_field_dofs, VectorFieldEntities &ent_field) const
Get field data on nodes.
int nInTheLoop
number currently of processed method
MoFEMErrorCode getProblemNodesIndices(const std::string &field_name, const NumeredDofEntity_multiIndex &dofs, VectorInt &nodes_indices) const
get indices of nodal indices which are declared for problem but not this particular element
auto getColDofsPtr() const
const std::array< boost::shared_ptr< EntitiesFieldData >, LASTSPACE > derivedDataOnElement
Entity data on element entity columns fields.
MoFEMErrorCode getBitRefLevelOnData()
EntityType lastEvaluatedElementEntityType
Last evaluated type of element entity.
RuleHookFun getRuleHook
Hook to get rule.
static UId getLoLocalEntityBitNumber(const char bit_number, const EntityHandle ent)
static auto cmp_uid_hi(const UId &b, const boost::weak_ptr< FieldEntity > &a)
#define CHK_THROW_MESSAGE(err, msg)
Check and throw MoFEM exception.
@ L2
field with C-1 continuity
virtual const Field * get_field_structure(const std::string &name, enum MoFEMTypes bh=MF_EXIST) const =0
get field structure
std::vector< boost::weak_ptr< FieldEntity > > FieldEntity_vector_view
UBlasMatrix< double > MatrixDouble
virtual MoFEMErrorCode setGaussPts(int order_row, int order_col, int order_data)
set user specific integration rule
virtual FieldBitNumber get_field_bit_number(const std::string name) const =0
get field bit number
const FieldEntity_vector_view & getDataFieldEnts() const
std::bitset< BITFIELDID_SIZE > BitFieldId
Field Id.
EntitiesFieldData & dataHdiv
MoFEMErrorCode getEntityDataOrder(const EntityType type, const FieldSpace space, boost::ptr_vector< EntitiesFieldData::EntData > &data) const
Get the entity data order.
boost::function< MoFEMErrorCode()> preProcessHook
Hook function for pre-processing.
ForcesAndSourcesCore * sidePtrFE
Element to integrate on the sides.
auto & getElementPolynomialBase()
Get the Entity Polynomial Base object.
MoFEMErrorCode calHierarchicalBaseFunctionsOnElement()
Calculate base functions.
MoFEMErrorCode getNoFieldRowIndices(EntitiesFieldData &data, const int bit_number) const
get col NoField indices
MoFEMErrorCode getNoFieldIndices(const int bit_number, boost::shared_ptr< FENumeredDofEntity_multiIndex > dofs, VectorInt &nodes_indices) const
get NoField indices
std::bitset< BITFEID_SIZE > BitFEId
Finite element Id.
#define THROW_MESSAGE(msg)
Throw MoFEM exception.
@ USER_BASE
user implemented approximation base
MoFEMErrorCode getEntityFieldData(EntitiesFieldData &data, const int bit_number, const EntityType type_lo=MBVERTEX, const EntityType type_hi=MBPOLYHEDRON) const
const Field_multiIndex * fieldsPtr
raw pointer to fields container
FieldSpace
approximation spaces
EntitiesFieldData & dataL2
#define CHKERR
Inline error check.
MoFEMErrorCode getNoFieldColIndices(EntitiesFieldData &data, const int bit_number) const
get col NoField indices
auto getRowDofsPtr() const
virtual BitFieldId get_field_id(const std::string &name) const =0
Get field Id.
virtual moab::Interface & get_moab()=0
ForcesAndSourcesCore * refinePtrFE
Element to integrate parent or child.
static MoFEMErrorCode get_value(MatrixDouble &pts_x, MatrixDouble &pts_t, TYPE *ctx)
auto & getUserPolynomialBase()
Get the User Polynomial Base object.
MoFEMErrorCode getEntityIndices(EntitiesFieldData &data, const int bit_number, FieldEntity_vector_view &ents_field, const EntityType type_lo, const EntityType type_hi, EXTRACTOR &&extractor) const
MoFEMErrorCode getColNodesIndices(EntitiesFieldData &data, const int bit_number) const
get col node indices from FENumeredDofEntity_multiIndex
boost::shared_ptr< NumeredDofEntity_multiIndex > numeredColDofsPtr
store DOFs on columns for this problem
FieldContinuity
Field continuity.
auto getNumberOfNodes() const
UId getLocalUniqueIdCalculate()
Get the Local Unique Id Calculate object.
multi_index_container< boost::shared_ptr< SideNumber >, indexed_by< ordered_unique< member< SideNumber, EntityHandle, &SideNumber::ent > >, ordered_non_unique< composite_key< SideNumber, const_mem_fun< SideNumber, EntityType, &SideNumber::getEntType >, member< SideNumber, signed char, &SideNumber::side_number > > > > > SideNumber_multiIndex
SideNumber_multiIndex for SideNumber.
boost::ptr_deque< UserDataOperator > opPtrVector
Vector of finite element users data operators.
const std::array< boost::shared_ptr< EntitiesFieldData >, LASTSPACE > dataOnElement
Entity data on element entity rows fields.
static UId getLoBitNumberUId(const FieldBitNumber bit_number)
static auto cmp_uid_lo(const boost::weak_ptr< FieldEntity > &a, const UId &b)
ForcesAndSourcesCore(Interface &m_field)
ublas::vector< FEDofEntity *, DofsAllocator > VectorDofs
boost::function< MoFEMErrorCode()> postProcessHook
Hook function for post-processing.
@ LASTSPACE
FieldSpace in [ 0, LASTSPACE )
virtual int getRule(int order_row, int order_col, int order_data)
another variant of getRule
EntityHandle get_id_for_max_type()
boost::shared_ptr< BaseFunction > userPolynomialBasePtr
Pointer to user polynomial base.
MoFEMErrorCode getEntityColIndices(EntitiesFieldData &data, const int bit_number, const EntityType type_lo=MBVERTEX, const EntityType type_hi=MBPOLYHEDRON) const
virtual MoFEMErrorCode operator()()
function is run for every finite element
auto getFEName() const
get finite element name
constexpr double t
plate stiffness
friend class UserDataOperator
FTensor::Index< 'i', SPACE_DIM > i
MoFEMErrorCode getProblemTypeIndices(const std::string &field_name, const NumeredDofEntity_multiIndex &dofs, EntityType type, int side_number, VectorInt &indices) const
get indices by type (generic function) which are declared for problem but not this particular element
EntitiesFieldData::EntData EntData
constexpr auto field_name
@ AINSWORTH_BERNSTEIN_BEZIER_BASE
MoFEMErrorCode getRowNodesIndices(EntitiesFieldData &data, const int bit_number) const
get row node indices from FENumeredDofEntity_multiIndex
boost::shared_ptr< NumeredDofEntity_multiIndex > numeredRowDofsPtr
store DOFs on rows for this problem
EntitiesFieldData & dataH1
const static char *const FieldSpaceNames[]
#define MOFEM_LOG(channel, severity)
Log.
boost::function< MoFEMErrorCode()> operatorHook
Hook function for operator.
int ApproximationOrder
Approximation on the entity.
FieldBitNumber getBitNumber() const
Get number of set bit in Field ID. Each field has uid, get getBitNumber get number of bit set for giv...
UBlasVector< int > VectorInt
EntitiesFieldData & dataHcurl
@ DISCONTINUOUS
Broken continuity (No effect on L2 space)
static MoFEMErrorCodeGeneric< PetscErrorCode > ierr
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
static int getMaxOrder(const ENTMULTIINDEX &multi_index)
@ HCURL
field with continuous tangents
@ MOFEM_DATA_INCONSISTENCY
const Problem * problemPtr
raw pointer to problem
MatrixDouble gaussPts
Matrix of integration points.
auto & getColFieldEnts() const
boost::shared_ptr< BaseFunction > elementPolynomialBasePtr
Pointer to entity polynomial base.
FieldApproximationBase
approximation base
static UId getHiBitNumberUId(const FieldBitNumber bit_number)
UBlasVector< double > VectorDouble
static UId getHiLocalEntityBitNumber(const char bit_number, const EntityHandle ent)
ublas::vector< FieldEntity *, FieldEntAllocator > VectorFieldEntities
GaussHookFun setRuleHook
Set function to calculate integration rule.
boost::shared_ptr< const NumeredEntFiniteElement > numeredEntFiniteElementPtr
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
MoFEMErrorCode getNodesIndices(const int bit_number, FieldEntity_vector_view &ents_field, VectorInt &nodes_indices, VectorInt &local_nodes_indices, EXTRACTOR &&extractor) const
get node indices
Field_multiIndex::index< FieldName_mi_tag >::type::iterator field_it
MoFEMErrorCode getNodesFieldData(EntitiesFieldData &data, const int bit_number) const
Get data on nodes.
boost::shared_ptr< FieldEntity_vector_view > & getDataFieldEntsPtr() const
@ CONTINUOUS
Regular field.
EntityHandle get_id_for_min_type()
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
@ HDIV
field with continuous normal traction
#define CHKERRG(n)
Check error code of MoFEM/MOAB/PETSc function.
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
MoFEMErrorCode getEntitySense(const EntityType type, boost::ptr_vector< EntitiesFieldData::EntData > &data) const
get sense (orientation) of entity
@ NOFIELD
scalar or vector of scalars describe (no true field)
static bool getDefTypeMap(const EntityType fe_type, const EntityType ent_type)