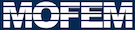 |
| v0.14.0
|
Testing edge elements nodesets.
namespace bio = boost::iostreams;
using bio::stream;
using bio::tee_device;
static char help[] =
"...\n\n";
int main(
int argc,
char *argv[]) {
try {
int rank;
MPI_Comm_rank(PETSC_COMM_WORLD, &rank);
PetscBool flg = PETSC_TRUE;
#if PETSC_VERSION_GE(3, 6, 4)
255, &flg);
#else
#endif
if (flg != PETSC_TRUE) {
SETERRQ(PETSC_COMM_SELF, 1, "*** ERROR -my_file (MESH FILE NEEDED)");
}
const char *option;
option = ""; ;
bit_level0.set(0);
0, 3, bit_level0);
enum bases { AINSWORTH, BERNSTEIN_BEZIER, LASBASETOP };
const char *list_bases[] = {"ainsworth", "bernstein_bezier"};
PetscInt choice_base_value = AINSWORTH;
LASBASETOP, &choice_base_value, &flg);
if (choice_base_value == AINSWORTH)
else if (choice_base_value == BERNSTEIN_BEZIER)
3);
auto add_field_to_fe = [&m_field](
const std::string
field_name) {
};
CHKERR add_field_to_fe(
"FIELD1");
"MESH_NODE_POSITIONS");
"MESH_NODE_POSITIONS");
1);
CHKERR moab.get_entities_by_type(0, MBTET, tets,
false);
CHKERR skin.find_skin(0, tets,
false, tets_skin);
CHKERR moab.get_adjacencies(tets_skin, 1,
false, tets_skin_edges,
moab::Interface::UNION);
"TEST_FE");
}
{
}
typedef tee_device<std::ostream, std::ofstream>
TeeDevice;
std::ofstream ofs(
(std ::string("forces_and_sources_testing_edge_element_") +
.c_str());
my_split(_my_split) {}
my_split << "NH1" << std::endl;
my_split <<
"side: " << side <<
" type: " <<
type << std::endl;
my_split << "data: " << data << std::endl;
my_split << "coords: " << std::setprecision(3) << getCoords()
<< std::endl;
my_split << "getCoordsAtGaussPts: " << std::setprecision(3)
<< getCoordsAtGaussPts() << std::endl;
my_split << "length: " << std::setprecision(3) << getLength()
<< std::endl;
my_split << "direction: " << std::setprecision(3) << getDirection()
<< std::endl;
int nb_gauss_pts = data.
getN().size1();
for (int gg = 0; gg < nb_gauss_pts; gg++) {
my_split << "tangent " << gg << " " << getTangentAtGaussPts()
<< std::endl;
}
}
MoFEMErrorCode doWork(
int row_side,
int col_side, EntityType row_type,
EntityType col_type,
my_split << "ROW NH1NH1" << std::endl;
my_split << "row side: " << row_side << " row_type: " << row_type
<< std::endl;
my_split << row_data << std::endl;
my_split << "COL NH1NH1" << std::endl;
my_split << "col side: " << col_side << " col_type: " << col_type
<< std::endl;
my_split << col_data << std::endl;
}
};
}
return 0;
}
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
Data on single entity (This is passed as argument to DataOperator::doWork)
virtual MoFEMErrorCode loop_finite_elements(const std::string problem_name, const std::string &fe_name, FEMethod &method, boost::shared_ptr< NumeredEntFiniteElement_multiIndex > fe_ptr=nullptr, MoFEMTypes bh=MF_EXIST, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr(), int verb=DEFAULT_VERBOSITY)=0
Make a loop over finite elements.
Calculate tangent vector on edge form HO geometry approximation.
virtual MoFEMErrorCode loop_dofs(const Problem *problem_ptr, const std::string &field_name, RowColData rc, DofMethod &method, int lower_rank, int upper_rank, int verb=DEFAULT_VERBOSITY)=0
Make a loop over dofs.
MoFEMErrorCode buildProblem(const std::string name, const bool square_matrix, int verb=VERBOSE)
build problem data structures
Problem manager is used to build and partition problems.
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
const static char *const ApproximationBaseNames[]
int main(int argc, char *argv[])
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
Projection of edge entities with one mid-node on hierarchical basis.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
@ OPROWCOL
operator doWork is executed on FE rows &columns
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
Deprecated interface functions.
DeprecatedCoreInterface Interface
virtual MoFEMErrorCode add_ents_to_finite_element_by_type(const EntityHandle entities, const EntityType type, const std::string &name, const bool recursive=true)=0
add entities to finite element
#define CHKERR
Inline error check.
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
virtual moab::Interface & get_moab()=0
implementation of Data Operators for Forces and Sources
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
friend class UserDataOperator
virtual MoFEMErrorCode modify_finite_element_add_field_data(const std::string &fe_name, const std::string name_field)=0
set finite element field data
MoFEMErrorCode partitionFiniteElements(const std::string name, bool part_from_moab=false, int low_proc=-1, int hi_proc=-1, int verb=VERBOSE)
partition finite elements
virtual MoFEMErrorCode modify_problem_ref_level_add_bit(const std::string &name_problem, const BitRefLevel &bit)=0
add ref level to problem
constexpr auto field_name
@ AINSWORTH_BERNSTEIN_BEZIER_BASE
default operator for EDGE element
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
MatrixDouble & getN(const FieldApproximationBase base)
get base functions this return matrix (nb. of rows is equal to nb. of Gauss pts, nb....
MoFEMErrorCode partitionSimpleProblem(const std::string name, int verb=VERBOSE)
partition problem dofs
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
Operator used to check consistency between local coordinates and global cooridnates for integrated po...
boost::ptr_deque< UserDataOperator > & getOpPtrVector()
Use to push back operator for row operator.
PetscErrorCode PetscOptionsGetString(PetscOptions *, const char pre[], const char name[], char str[], size_t size, PetscBool *set)
PetscErrorCode PetscOptionsGetEList(PetscOptions *, const char pre[], const char name[], const char *const *list, PetscInt next, PetscInt *value, PetscBool *set)
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
FieldApproximationBase
approximation base
virtual MoFEMErrorCode modify_problem_add_finite_element(const std::string name_problem, const std::string &fe_name)=0
add finite element to problem, this add entities assigned to finite element to a particular problem
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
MoFEMErrorCode partitionGhostDofs(const std::string name, int verb=VERBOSE)
determine ghost nodes
virtual MoFEMErrorCode add_problem(const std::string &name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add problem.
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
virtual MoFEMErrorCode add_field(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
@ OPROW
operator doWork function is executed on FE rows