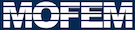 |
| v0.14.0
|
Go to the documentation of this file.
9 #ifndef __BITREFMANAGER_HPP__
10 #define __BITREFMANAGER_HPP__
80 const bool only_tets =
true,
int verb = 0,
81 Range *adj_ents_ptr =
nullptr)
const;
98 int verb =
QUIET)
const;
115 int verb =
QUIET)
const;
128 int verb =
QUIET)
const;
144 int verb =
QUIET)
const;
159 const EntityType
type,
161 int verb =
QUIET)
const;
192 int verb =
QUIET)
const;
212 int verb =
QUIET)
const;
245 int verb =
QUIET)
const;
258 int verb =
QUIET)
const;
271 int verb =
QUIET)
const;
281 int verb =
QUIET)
const;
318 int verb =
QUIET)
const;
333 const EntityType
type,
350 const EntityType
type,
351 Range &ents,
int verb = 0)
const;
383 const int dim,
Range &ents,
399 const int verb =
QUIET)
const;
412 const int verb =
QUIET)
const;
428 const int verb =
QUIET)
const;
460 const int to_dimension,
461 Range &adj_entities)
const;
471 const int to_dimension,
472 Range &adj_entities)
const;
483 const int num_entities,
const int to_dimension,
485 const int operation_type = moab::Interface::INTERSECT,
486 const int verb = 0)
const;
497 const int num_entities,
const int to_dimension,
499 const int operation_type = moab::Interface::INTERSECT,
500 const int verb = 0)
const;
535 EntityType child_type,
const bool recursive =
false,
int verb = 0);
542 EntityType child_type,
543 const bool recursive =
false,
560 const std::string name,
const BitRefLevel &child_bit,
int verb = 0);
566 const std::string name,
const BitRefLevel &child_bit,
567 const EntityType fe_ent_type,
int verb = 0);
619 const char *file_name,
const char *file_type,
621 const bool check_for_empty =
true)
const;
635 const char *file_name,
636 const char *file_type,
const char *options,
637 const bool check_for_empty =
true)
const;
651 const EntityType
type,
652 const char *file_name,
653 const char *file_type,
const char *options,
654 const bool check_for_empty =
true)
const;
668 const bool check_for_empty =
true)
const;
681 const EntityType
type,
682 const char *file_name,
683 const char *file_type,
684 const char *options);
697 bool *changed =
nullptr);
716 #endif //__BITREFMANAGER_HPP__
virtual ~BitRefManager()=default
Destructor.
MoFEMErrorCode addBitRefLevelByDim(const EntityHandle meshset, const int dim, const BitRefLevel bit, int verb=QUIET) const
add bit ref level by dimension
Tag get_th_RefParentHandle() const
MoFEMErrorCode getEntitiesByRefLevel(const BitRefLevel bit, const BitRefLevel mask, const EntityHandle meshset, const int verb=QUIET) const
add all ents from ref level given by bit to meshset
MoFEMErrorCode shiftLeftBitRef(const int shift, const BitRefLevel mask=BitRefLevel().set(), int verb=DEFAULT_VERBOSITY) const
left shift bit ref level
MoFEMErrorCode setElementsBitRefLevel(const Range &ents, const BitRefLevel bit=BitRefLevel(), int verb=QUIET) const
add entities to database and set bit ref level
MoFEMErrorCode setBitRefLevelByType(const EntityHandle meshset, const EntityType type, const BitRefLevel bit, int verb=QUIET) const
Set the Bit Ref Level By Type object.
MoFEMErrorCode getEntitiesByParentType(const BitRefLevel bit, const BitRefLevel mask, const EntityType type, Range &ents, const int verb=QUIET) const
get entities by bit ref level and type of parent
MoFEMErrorCode filterEntitiesNotInDatabase(Range &ents) const
Get entities not in database.
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
MoFEMErrorCode setFieldEntitiesBitRefLevel(const std::string field_name, const BitRefLevel bit=BitRefLevel(), int verb=QUIET) const
Set the bit ref level to entities in the field meshset.
MoFEMErrorCode lambdaBitRefLevel(boost::function< void(EntityHandle ent, BitRefLevel &bit)> fun) const
Process bit ref level by lambda function.
MoFEMErrorCode writeBitLevel(const BitRefLevel bit, const BitRefLevel mask, const char *file_name, const char *file_type, const char *options, const bool check_for_empty=true) const
Write bit ref level to file.
MoFEMErrorCode filterEntitiesByRefLevel(const BitRefLevel bit, const BitRefLevel mask, Range &ents, int verb=QUIET) const
filter entities by bit ref level
DeprecatedCoreInterface Interface
static MoFEMErrorCode fixTagSize(moab::Interface &moab, bool *changed=nullptr)
Fix tag size when BITREFLEVEL_SIZE of core library is different than file BITREFLEVEL_SIZE.
MoFEMErrorCode addToDatabaseBitRefLevelByType(const EntityType type, const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), int verb=QUIET) const
Add entities which exist in MoAB database, and have set appropiate BitRef level tag,...
MoFEMErrorCode setEntitiesBitRefLevel(const Range &ents, const BitRefLevel bit=BitRefLevel(), int verb=QUIET) const
add entities to database and set bit ref level
MoFEMErrorCode writeEntitiesNotInDatabase(const char *file_name, const char *file_type, const char *options, const bool check_for_empty=true) const
Write ents not in database.
MoFEMErrorCode setBitRefLevel(const Range &ents, const BitRefLevel bit, const bool only_tets=true, int verb=0, Range *adj_ents_ptr=nullptr) const
add entities to database and set bit ref level
implementation of Data Operators for Forces and Sources
MoFEMErrorCode setBitRefLevelByDim(const EntityHandle meshset, const int dim, const BitRefLevel bit, int verb=QUIET) const
Set the Bit Ref Level By Dim object.
MoFEMErrorCode getEntitiesByDimAndRefLevel(const BitRefLevel bit, const BitRefLevel mask, const int dim, const EntityHandle meshset, int verb=0) const
add all ents from ref level given by bit to meshset
MoFEMErrorCode updateFiniteElementMeshsetByEntitiesChildren(const std::string name, const BitRefLevel &child_bit, const EntityType fe_ent_type, int verb=0)
update finite element meshset by child entities
Tag get_th_RefParentHandle() const
MoFEMErrorCode updateRangeByParent(const Range &child_ents, Range &parent_ents, MoFEMTypes bh=MF_ZERO)
Update range by parents.
Tag get_th_RefBitLevel() const
MoFEMErrorCode updateMeshsetByEntitiesChildren(const EntityHandle parent, const BitRefLevel &parent_bit, const BitRefLevel &parent_mask, const BitRefLevel &child_bit, const BitRefLevel &child_mask, const EntityHandle child, EntityType child_type, const bool recursive=false, int verb=0)
Get child entities form meshset containing parent entities.
MoFEMErrorCode addBitRefLevel(const Range &ents, const BitRefLevel &bit, int verb=QUIET) const
add bit ref level to ref entity
MoFEMErrorCode setBitLevelToMeshset(const EntityHandle meshset, const BitRefLevel bit, int verb=0) const
MoFEMErrorCode writeBitLevelByDim(const BitRefLevel bit, const BitRefLevel mask, const int dim, const char *file_name, const char *file_type, const char *options, const bool check_for_empty=true) const
Write bit ref level to file.
BitRefManager(const MoFEM::Core &core)
MoFEMErrorCode addToDatabaseBitRefLevelByDim(const int dim, const BitRefLevel bit, const BitRefLevel mask=BitRefLevel().set(), int verb=QUIET) const
Add entities which exist in MoAB database, and have set appropiate BitRef level tag,...
constexpr auto field_name
MoFEMErrorCode updateRangeByChildren(const Range &parent, Range &child, MoFEMTypes bh=MF_ZERO)
Update range by childrens.
base class for all interface classes
DEPRECATED MoFEMErrorCode updateRange(const Range &parent, Range &child, MoFEMTypes bh=MF_ZERO)
Tag get_th_RefBitLevel() const
virtual MoFEMErrorCode getAdjacencies(const Problem *problem_ptr, const EntityHandle *from_entities, const int num_entities, const int to_dimension, Range &adj_entities, const int operation_type=moab::Interface::INTERSECT, const int verb=0) const
Get the adjacencies associated with a entity to entities of a specified dimension.
virtual MoFEMErrorCode getAdjacenciesAny(const EntityHandle from_entity, const int to_dimension, Range &adj_entities) const
Get the adjacencies associated with a entity to entities of a specified dimension.
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const
MoFEMErrorCode updateFieldMeshsetByEntitiesChildren(const BitRefLevel &child_bit, int verb=0)
update fields meshesets by child entities
MoFEMErrorCode writeBitLevelByType(const BitRefLevel bit, const BitRefLevel mask, const EntityType type, const char *file_name, const char *file_type, const char *options, const bool check_for_empty=true) const
Write bit ref level to file.
MoFEMErrorCode setNthBitRefLevel(const Range &ents, const int n, const bool b, int verb=QUIET) const
Set nth bit ref level.
virtual MoFEMErrorCode getAdjacenciesEquality(const EntityHandle from_entity, const int to_dimension, Range &adj_entities) const
Get the adjacencies associated with a entity to entities of a specified dimension.
MoFEMTypes
Those types control how functions respond on arguments, f.e. error handling.
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
keeps basic data about problem
MoFEMErrorCode writeEntitiesAllBitLevelsByType(const BitRefLevel mask, const EntityType type, const char *file_name, const char *file_type, const char *options)
Write all entities by bit levels and type.
MoFEMErrorCode getEntitiesByTypeAndRefLevel(const BitRefLevel bit, const BitRefLevel mask, const EntityType type, const EntityHandle meshset, int verb=0) const
add all ents from ref level given by bit to meshset
auto fun
Function to approximate.
MoFEMErrorCode getAllEntitiesNotInDatabase(Range &ents) const
Get all entities not in database.
MoFEMErrorCode shiftRightBitRef(const int shift, const BitRefLevel mask=BitRefLevel().set(), int verb=DEFAULT_VERBOSITY, MoFEMTypes mf=MF_ZERO) const
right shift bit ref level