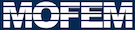 |
| v0.14.0
|
Go to the source code of this file.
|
struct | MyOp< OP > |
| Operator used to check consistency between local coordinates and global cooridnates for integrated points and evaluated points. More...
|
|
struct | CallingOp |
|
struct | MyOp2 |
|
|
int | main (int argc, char *argv[]) |
|
|
static char | help [] = "...\n\n" |
|
◆ TeeDevice
typedef tee_device<std::ostream, std::ofstream> TeeDevice |
◆ TeeStream
◆ main()
int main |
( |
int |
argc, |
|
|
char * |
argv[] |
|
) |
| |
Definition at line 174 of file forces_and_sources_testing_contact_prism_element.cpp.
183 PetscBool flg = PETSC_TRUE;
187 if (flg != PETSC_TRUE)
190 enum spaces { MYH1, MYHDIV, MYLASTSPACEOP };
191 const char *list_spaces[] = {
"h1",
"hdiv"};
192 PetscInt choice_space_value =
H1;
194 MYLASTSPACEOP, &choice_space_value, &flg);
195 bool is_hdiv = (choice_space_value == MYHDIV) ?
true :
false;
202 auto add_atom_logging = [is_hdiv]() {
204 auto get_log_file_name = [is_hdiv]() {
206 return "forces_and_sources_testing_contact_prism_element_HDIV.txt";
208 return "forces_and_sources_testing_contact_prism_element.txt";
211 auto core_log = logging::core::get();
213 LogManager::createSink(LogManager::getStrmSelf(),
"ATOM_TEST"));
214 LogManager::setLog(
"ATOM_TEST");
218 logging::add_file_log(keywords::file_name = get_log_file_name(),
220 MoFEM::LogKeywords::channel ==
"ATOM_TEST");
224 CHKERR add_atom_logging();
235 std::vector<BitRefLevel> bit_levels;
241 CHKERR PetscPrintf(PETSC_COMM_WORLD,
"Insert Interface %d\n",
242 cit->getMeshsetId());
247 CHKERR moab.create_meshset(MESHSET_SET, ref_level_meshset);
249 ->getEntitiesByTypeAndRefLevel(bit_levels.back(),
253 ->getEntitiesByTypeAndRefLevel(bit_levels.back(),
256 Range ref_level_tets;
257 CHKERR moab.get_entities_by_handle(ref_level_meshset, ref_level_tets,
260 CHKERR interface->
getSides(cubit_meshset, bit_levels.back(),
true, 0);
265 cubit_meshset,
true,
true, 0);
267 CHKERR moab.delete_entities(&ref_level_meshset, 1);
273 ->updateMeshsetByEntitiesChildren(cubit_meshset, bit_levels.back(),
274 cubit_meshset, MBMAXTYPE,
true);
288 auto set_no_field_vertex = [&]() {
291 const double coords[] = {0, 0, 0};
294 Range range_no_field_vertex;
295 range_no_field_vertex.insert(no_field_vertex);
299 CHKERR m_field.
get_moab().add_entities(meshset, range_no_field_vertex);
303 CHKERR set_no_field_vertex();
314 "MESH_NODE_POSITIONS");
338 "MESH_NODE_POSITIONS");
346 constexpr
int order = 3;
373 m_field,
"MESH_NODE_POSITIONS");
374 CHKERR m_field.
loop_dofs(
"MESH_NODE_POSITIONS", ent_method_mesh_positions);
393 using ContactDataOp =
397 fe1.getOpPtrVector().push_back(
398 new MyOp(UMDataOp::OPROW, ContactDataOp::FACEMASTER));
399 fe1.getOpPtrVector().push_back(
400 new MyOp(UMDataOp::OPROW, ContactDataOp::FACESLAVE));
401 fe1.getOpPtrVector().push_back(
402 new MyOp(UMDataOp::OPROWCOL, ContactDataOp::FACEMASTERMASTER));
403 fe1.getOpPtrVector().push_back(
404 new MyOp(UMDataOp::OPROWCOL, ContactDataOp::FACEMASTERSLAVE));
405 fe1.getOpPtrVector().push_back(
406 new MyOp(UMDataOp::OPROWCOL, ContactDataOp::FACESLAVEMASTER));
407 fe1.getOpPtrVector().push_back(
408 new MyOp(UMDataOp::OPROWCOL, ContactDataOp::FACESLAVESLAVE));
409 fe1.getOpPtrVector().push_back(
new CallingOp(UMDataOp::OPCOL));
410 fe1.getOpPtrVector().push_back(
new CallingOp(UMDataOp::OPROW));
411 fe1.getOpPtrVector().push_back(
new CallingOp(UMDataOp::OPROWCOL));
415 fe2.getOpPtrVector().push_back(
416 new MyOp2(UMDataOp::OPCOL, ContactDataOp::FACEMASTER));
417 fe2.getOpPtrVector().push_back(
418 new MyOp2(UMDataOp::OPCOL, ContactDataOp::FACESLAVE));
419 fe2.getOpPtrVector().push_back(
420 new MyOp2(UMDataOp::OPROWCOL, ContactDataOp::FACEMASTERMASTER));
421 fe2.getOpPtrVector().push_back(
422 new MyOp2(UMDataOp::OPROWCOL, ContactDataOp::FACEMASTERSLAVE));
423 fe2.getOpPtrVector().push_back(
424 new MyOp2(UMDataOp::OPROWCOL, ContactDataOp::FACESLAVEMASTER));
425 fe2.getOpPtrVector().push_back(
426 new MyOp2(UMDataOp::OPROWCOL, ContactDataOp::FACESLAVESLAVE));
◆ help
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
virtual MoFEMErrorCode loop_finite_elements(const std::string problem_name, const std::string &fe_name, FEMethod &method, boost::shared_ptr< NumeredEntFiniteElement_multiIndex > fe_ptr=nullptr, MoFEMTypes bh=MF_EXIST, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr(), int verb=DEFAULT_VERBOSITY)=0
Make a loop over finite elements.
virtual MoFEMErrorCode loop_dofs(const Problem *problem_ptr, const std::string &field_name, RowColData rc, DofMethod &method, int lower_rank, int upper_rank, int verb=DEFAULT_VERBOSITY)=0
Make a loop over dofs.
MoFEMErrorCode buildProblem(const std::string name, const bool square_matrix, int verb=VERBOSE)
build problem data structures
Problem manager is used to build and partition problems.
Create interface from given surface and insert flat prisms in-between.
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
Projection of edge entities with one mid-node on hierarchical basis.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
virtual EntityHandle get_field_meshset(const std::string name) const =0
get field meshset
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
Deprecated interface functions.
DeprecatedCoreInterface Interface
#define CHKERR
Inline error check.
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
virtual moab::Interface & get_moab()=0
virtual MoFEMErrorCode add_ents_to_finite_element_by_type(const EntityHandle entities, const EntityType type, const std::string name, const bool recursive=true)=0
add entities to finite element
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
MoFEMErrorCode splitSides(const EntityHandle meshset, const BitRefLevel &bit, const int msId, const CubitBCType cubit_bc_type, const bool add_interface_entities, const bool recursive=false, int verb=QUIET)
Split nodes and other entities of tetrahedra on both sides of the interface and insert flat prisms in...
#define MOFEM_LOG_TAG(channel, tag)
Tag channel.
virtual MoFEMErrorCode modify_finite_element_add_field_data(const std::string &fe_name, const std::string name_field)=0
set finite element field data
MoFEMErrorCode partitionFiniteElements(const std::string name, bool part_from_moab=false, int low_proc=-1, int hi_proc=-1, int verb=VERBOSE)
partition finite elements
virtual MoFEMErrorCode modify_problem_ref_level_add_bit(const std::string &name_problem, const BitRefLevel &bit)=0
add ref level to problem
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
#define _IT_CUBITMESHSETS_BY_SET_TYPE_FOR_LOOP_(MESHSET_MANAGER, CUBITBCTYPE, IT)
Iterator that loops over a specific Cubit MeshSet having a particular BC meshset in a moFEM field.
MoFEMErrorCode partitionSimpleProblem(const std::string name, int verb=VERBOSE)
partition problem dofs
MoFEMErrorCode getSides(const int msId, const CubitBCType cubit_bc_type, const BitRefLevel mesh_bit_level, const bool recursive, int verb=QUIET)
Store tetrahedra from each side of the interface separately in two child meshsets of the parent meshs...
ForcesAndSourcesCore::UserDataOperator UserDataOperator
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
Operator used to check consistency between local coordinates and global cooridnates for integrated po...
PetscErrorCode PetscOptionsGetString(PetscOptions *, const char pre[], const char name[], char str[], size_t size, PetscBool *set)
PetscErrorCode PetscOptionsGetEList(PetscOptions *, const char pre[], const char name[], const char *const *list, PetscInt next, PetscInt *value, PetscBool *set)
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
#define _IT_CUBITMESHSETS_FOR_LOOP_(MESHSET_MANAGER, IT)
Iterator that loops over all the Cubit MeshSets in a moFEM field.
virtual MoFEMErrorCode modify_problem_add_finite_element(const std::string name_problem, const std::string &fe_name)=0
add finite element to problem, this add entities assigned to finite element to a particular problem
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
MoFEMErrorCode partitionGhostDofs(const std::string name, int verb=VERBOSE)
determine ghost nodes
virtual MoFEMErrorCode add_problem(const std::string &name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add problem.
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
@ HDIV
field with continuous normal traction
virtual MoFEMErrorCode add_field(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
@ NOFIELD
scalar or vector of scalars describe (no true field)