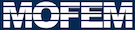 |
| v0.14.0
|
Go to the documentation of this file.
11 #ifndef __PRISMINTERFACE_HPP__
12 #define __PRISMINTERFACE_HPP__
53 const bool recursive,
int verb =
QUIET);
75 const bool recursive,
int verb =
QUIET);
102 const bool recursive,
int verb =
QUIET);
130 const bool recursive,
int verb =
QUIET);
151 const bool recursive,
Range &faces_with_three_nodes_on_front,
173 const bool add_interface_entities,
174 const bool recursive =
false,
int verb =
QUIET);
193 const bool add_interface_entities,
194 const bool recursive =
false,
int verb =
QUIET);
221 const bool add_interface_entities,
222 const bool recursive =
false,
int verb =
QUIET);
237 #endif // __PRISMINTERFACE_HPP__
Create interface from given surface and insert flat prisms in-between.
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
implementation of Data Operators for Forces and Sources
MoFEMErrorCode splitSides(const EntityHandle meshset, const BitRefLevel &bit, const int msId, const CubitBCType cubit_bc_type, const bool add_interface_entities, const bool recursive=false, int verb=QUIET)
Split nodes and other entities of tetrahedra on both sides of the interface and insert flat prisms in...
~PrismInterface()=default
destructor
base class for all interface classes
std::bitset< 32 > CubitBCType
MoFEMErrorCode getSides(const int msId, const CubitBCType cubit_bc_type, const BitRefLevel mesh_bit_level, const bool recursive, int verb=QUIET)
Store tetrahedra from each side of the interface separately in two child meshsets of the parent meshs...
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
MoFEMErrorCode findFacesWithThreeNodesOnInternalSurfaceSkin(const EntityHandle sideset, const BitRefLevel mesh_bit_level, const bool recursive, Range &faces_with_three_nodes_on_front, int verb=QUIET)
Find triangles which have three nodes on internal surface skin.
PrismInterface(const MoFEM::Core &core)