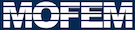 |
| v0.14.0
|
Test iterating over boundary and skeleton elements only when L2 field is presents on the domain.
static char help[] =
"...\n\n";
};
int main(
int argc,
char *argv[]) {
try {
DMType dm_name = "DMMOFEM";
{
int count_fe;
int count_side_fe;
op_side_fe->doWorkRhsHook = [&](
DataOperator *op_ptr,
int side,
<< "Side element name [ " << count_side_fe << " ] "
<< domain_op->getFEName();
++count_side_fe;
};
auto side_fe = boost::make_shared<DomainEle>(m_field);
side_fe->getOpPtrVector().push_back(op_side_fe);
MOFEM_LOG(
"SELF", Sev::verbose) <<
"Element name [ " << count_fe
<< " ] " << bdy_op->getFEName();
++count_fe;
};
op_bdy_fe->doWorkRhsHook = do_work_rhs;
op_skeleton_fe->doWorkRhsHook = do_work_rhs;
count_fe = 0;
count_side_fe = 0;
MOFEM_LOG(
"SELF", Sev::inform) <<
"Number of elements " << count_fe;
<< "Number of side elements " << count_side_fe;
if (count_fe != 16)
"Wrong numbers of FEs");
if (count_side_fe != 24)
"Wrong numbers of side FEs");
}
}
return 0;
}
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
Data on single entity (This is passed as argument to DataOperator::doWork)
ElementsAndOps< SPACE_DIM >::BoundaryEle BoundaryEle
base operator to do operations at Gauss Pt. level
boost::ptr_deque< UserDataOperator > & getOpSkeletonRhsPipeline()
Get the Op Skeleton Rhs Pipeline object.
BoundaryEle::UserDataOperator BoundaryEleOp
@ L2
field with C-1 continuity
MoFEMErrorCode loopFiniteElements(SmartPetscObj< DM > dm=nullptr)
Iterate finite elements.
MoFEMErrorCode loadFile(const std::string options, const std::string mesh_file_name, LoadFileFunc loadFunc=defaultLoadFileFunc)
Load mesh file.
PipelineManager interface.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
Simple interface for fast problem set-up.
Deprecated interface functions.
DomainEle::UserDataOperator DomainEleOp
DeprecatedCoreInterface Interface
MoFEMErrorCode getOptions()
get options
MoFEM::EdgeElementForcesAndSourcesCore EdgeEle
bool & getAddSkeletonFE()
Get the addSkeletonFE.
#define CHKERR
Inline error check.
implementation of Data Operators for Forces and Sources
MoFEM::FaceElementForcesAndSourcesCore FaceEle
MoFEMErrorCode addDomainField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on domain.
bool & getAddBoundaryFE()
Get the addSkeletonFE.
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
const std::string getDomainFEName() const
Get the Domain FE Name.
MoFEMErrorCode setFieldOrder(const std::string field_name, const int order, const Range *ents=NULL)
Set field order.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define MOFEM_LOG(channel, severity)
Log.
#define CATCH_ERRORS
Catch errors.
ForcesAndSourcesCore::UserDataOperator UserDataOperator
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
boost::ptr_deque< UserDataOperator > & getOpBoundaryRhsPipeline()
Get the Op Boundary Rhs Pipeline object.
EntitiesFieldData::EntData EntData
@ MOFEM_ATOM_TEST_INVALID
ElementsAndOps< SPACE_DIM >::DomainEle DomainEle
int main(int argc, char *argv[])
MoFEMErrorCode setUp(const PetscBool is_partitioned=PETSC_TRUE)
Setup problem.
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...