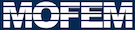 |
| v0.14.0
|
Go to the source code of this file.
|
int | main (int argc, char *argv[]) |
|
◆ BoundaryEle
◆ BoundaryEleOp
◆ DomainEle
◆ DomainEleOp
◆ EntData
◆ main()
int main |
( |
int |
argc, |
|
|
char * |
argv[] |
|
) |
| |
- Examples
- simple_l2_only.cpp.
Definition at line 33 of file simple_l2_only.cpp.
49 DMType dm_name =
"DMMOFEM";
86 int count_skeleton_fe;
89 int count_meshset_side_fe;
95 op_side_fe->doWorkRhsHook = [&](
DataOperator *op_ptr,
int side,
98 auto domain_op =
static_cast<DomainEleOp *
>(op_ptr);
102 <<
"Side element name [ " << count_side_fe <<
" ] "
103 << domain_op->getFEName();
111 auto side_fe = boost::make_shared<DomainEle>(m_field);
112 side_fe->getOpPtrVector().push_back(op_side_fe);
122 <<
"Element name [ " << count_skeleton_fe <<
" ] "
123 << bdy_op->getFEName();
134 op_bdy_fe->doWorkRhsHook = do_work_rhs;
137 op_skeleton_fe->doWorkRhsHook = do_work_rhs;
141 op_meshset_side_fe->doWorkRhsHook =
144 auto domain_op =
static_cast<DomainEleOp *
>(op_ptr);
148 <<
"Side element name [ " << count_side_fe <<
" ] "
149 << domain_op->getFEName();
151 ++count_meshset_side_fe;
156 auto meshset_side_fe = boost::make_shared<DomainEle>(m_field);
157 meshset_side_fe->getOpPtrVector().push_back(op_meshset_side_fe);
160 "GLOBAL", ForcesAndSourcesCore::UserDataOperator::OPROW);
161 op_meshset_fe->doWorkRhsHook = [&](
DataOperator *op_ptr,
int side,
166 <<
"Meshset element name " << data.getIndices();
170 boost::make_shared<Range>(vols));
177 count_skeleton_fe = 0;
179 count_meshset_fe = 0;
180 count_meshset_side_fe = 0;
188 <<
"Number of elements " << count_skeleton_fe;
190 <<
"Number of side elements " << count_side_fe;
192 <<
"Number of meshset elements " << count_meshset_fe;
194 <<
"Number of meshset side elements " << count_meshset_side_fe;
196 if (count_skeleton_fe != 16)
198 "Wrong numbers of FEs");
199 if (count_side_fe != 24)
201 "Wrong numbers of side FEs");
202 if (count_meshset_fe != 1)
204 "Wrong numbers of side FEs");
205 if (count_meshset_side_fe != 8)
207 "Wrong numbers of side FEs");
◆ help
◆ SPACE_DIM
constexpr int SPACE_DIM = 2 |
|
constexpr |
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
Data on single entity (This is passed as argument to DataOperator::doWork)
base operator to do operations at Gauss Pt. level
boost::ptr_deque< UserDataOperator > & getOpSkeletonRhsPipeline()
Get the Op Skeleton Rhs Pipeline object.
BoundaryEle::UserDataOperator BoundaryEleOp
@ L2
field with C-1 continuity
MoFEMErrorCode loopFiniteElements(SmartPetscObj< DM > dm=nullptr)
Iterate finite elements.
MoFEMErrorCode loadFile(const std::string options, const std::string mesh_file_name, LoadFileFunc loadFunc=defaultLoadFileFunc)
Load mesh file.
PipelineManager interface.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
Simple interface for fast problem set-up.
Deprecated interface functions.
DomainEle::UserDataOperator DomainEleOp
DeprecatedCoreInterface Interface
MoFEMErrorCode addMeshsetField(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add meshset field.
MoFEMErrorCode getOptions()
get options
bool & getAddSkeletonFE()
Get the addSkeletonFE.
#define CHKERR
Inline error check.
virtual moab::Interface & get_moab()=0
auto & getMeshsetFiniteElementEntities()
Get the Domain Fields.
bool & getAddBoundaryFE()
Get the addSkeletonFE.
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
const std::string getDomainFEName() const
Get the Domain FE Name.
MoFEMErrorCode setFieldOrder(const std::string field_name, const int order, const Range *ents=NULL)
Set field order.
boost::ptr_deque< UserDataOperator > & getOpMeshsetRhsPipeline()
Get the Op Meshset Rhs Pipeline object.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define MOFEM_LOG(channel, severity)
Log.
#define CATCH_ERRORS
Catch errors.
ForcesAndSourcesCore::UserDataOperator UserDataOperator
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
boost::ptr_deque< UserDataOperator > & getOpBoundaryRhsPipeline()
Get the Op Boundary Rhs Pipeline object.
@ MOFEM_ATOM_TEST_INVALID
MoFEMErrorCode setUp(const PetscBool is_partitioned=PETSC_TRUE)
Setup problem.
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode addDomainField(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on domain.
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
@ NOFIELD
scalar or vector of scalars describe (no true field)