Loading [MathJax]/extensions/AMSmath.js
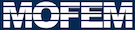 |
| v0.14.0
|
Go to the documentation of this file.
9 template <
class T,
int Tensor_Dim>
class Tensor1<T *, Tensor_Dim> {
17 mutable T *restrict data[Tensor_Dim];
30 Tensor1(T *d0, T *d1, T *d2,
const int i = 1) : inc(
i) {
33 Tensor1(T *d0, T *d1, T *d2, T *
d3,
const int i = 1) : inc(
i) {
36 Tensor1(T *d0, T *d1, T *d2, T *
d3, T *
d4, T *d5,
const int i = 1) : inc(
i) {
39 Tensor1(T *d0, T *d1, T *d2, T *
d3, T *
d4, T *d5, T *d6, T *d7,
45 Tensor1(T *d0, T *d1, T *d2, T *
d3, T *
d4, T *d5, T *d6, T *d7, T *d8,
52 template <
class...
U>
Tensor1(
U *...
d) : data(
d...), inc(1) {}
56 Tensor1(std::array<U *, Tensor_Dim> &
a,
const int i = 1) : inc(
i) {
57 std::copy(
a.begin(),
a.end(), data);
71 return (*data[Current_Dim - 1]) * (*data[Current_Dim - 1]) +
81 if(
N >= Tensor_Dim ||
N < 0)
84 s <<
"Bad index in Tensor1<T*," << Tensor_Dim <<
">.operator(" <<
N
86 throw std::out_of_range(s.str());
94 if(
N >= Tensor_Dim ||
N < 0)
97 s <<
"Bad index in Tensor1<T*," << Tensor_Dim <<
">.operator(" <<
N
98 <<
") const" << std::endl;
99 throw std::out_of_range(s.str());
107 if(
N >= Tensor_Dim ||
N < 0)
110 s <<
"Bad index in Tensor1<T*," << Tensor_Dim <<
">.ptr(" <<
N <<
")"
112 throw std::out_of_range(s.str());
120 if (
N >= Tensor_Dim ||
N < 0) {
122 s <<
"Bad index in Tensor1<T*," << Tensor_Dim <<
">.ptr(" <<
N <<
")"
124 throw std::out_of_range(s.str());
135 template <
char i,
int Dim>
142 template <
char i,
int Dim>
154 for(
int i = 0;
i < Tensor_Dim; ++
i)
171 template <
class T,
int Tensor_Dim,
int I>
189 for(
int i = 0;
i < Tensor_Dim; ++
i)
Tensor1(T *d0, T *d1, T *d2, T *d3, T *d4, T *d5, const int i=1)
static constexpr std::array< double, 3 > d3
const Tensor1_Expr< const dTensor0< T, Dim, i >, typename promote< T, double >::V, Dim, i > d(const Tensor0< T * > &a, const Index< i, Dim > index, const Tensor1< int, Dim > &d_ijk, const Tensor1< double, Dim > &d_xyz)
Tensor1(T *d0, const int i=1)
Tensor1(T *d0, T *d1, T *d2, T *d3, T *d4, T *d5, T *d6, T *d7, const int i=1)
T l2_squared(const Number< 1 > &) const
constexpr IntegrationType I
Tensor1(std::array< U *, Tensor_Dim > &a, const int i=1)
Tensor1(double d0, double d1, double d2)
Tensor1_Expr< const Tensor1< T *, Tensor_Dim >, T, Dim, i > operator()(const Index< i, Dim > &index) const
T l2_squared(const Number< Current_Dim > &) const
Tensor1(T *d0, T *d1, T *d2, T *d3, const int i=1)
Tensor1< T, Tensor_Dim > normalize()
const Tensor1 & operator++() const
Tensor1(T *d0, T *d1, T *d2, const int i=1)
FTensor::Index< 'i', SPACE_DIM > i
Tensor1(std::array< U *, Tensor_Dim > &a)
Tensor1(T *d0, T *d1, const int i=1)
T operator()(const int N) const
const Tensor1 & operator++() const
T & operator()(const int N)
static constexpr std::array< double, 3 > d4
Tensor1_Expr< Tensor1< T *, Tensor_Dim >, T, Dim, i > operator()(const Index< i, Dim > &index)
Tensor1(T *d0, T *d1, T *d2, T *d3, T *d4, T *d5, T *d6, T *d7, T *d8, const int i=1)
T * ptr(const int N) const