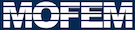 |
| v0.14.0
|
Go to the source code of this file.
|
using | EdgeEle = EdgeElementForcesAndSourcesCore |
|
typedef multi_index_container< CoordsAndHandle, indexed_by< hashed_unique< composite_key< CoordsAndHandle, member< CoordsAndHandle, int, &CoordsAndHandle::x >, member< CoordsAndHandle, int, &CoordsAndHandle::y >, member< CoordsAndHandle, int, &CoordsAndHandle::z > > > > > | MapCoords |
|
|
int | main (int argc, char *argv[]) |
|
◆ EdgeEle
◆ MapCoords
typedef multi_index_container< CoordsAndHandle, indexed_by< hashed_unique<composite_key< CoordsAndHandle, member<CoordsAndHandle, int, &CoordsAndHandle::x>, member<CoordsAndHandle, int, &CoordsAndHandle::y>, member<CoordsAndHandle, int, &CoordsAndHandle::z> > > > > MapCoords |
◆ main()
int main |
( |
int |
argc, |
|
|
char * |
argv[] |
|
) |
| |
- Examples
- prism_elements_from_surface.cpp.
Definition at line 132 of file prism_elements_from_surface.cpp.
141 MPI_Comm_rank(PETSC_COMM_WORLD, &rank);
144 PetscBool flg = PETSC_TRUE;
148 if (flg != PETSC_TRUE)
150 "error -my_file (MESH FILE NEEDED)");
158 CHKERR moab.get_entities_by_type(0, MBTRI, tris,
false);
160 CHKERR moab.get_connectivity(tris, tris_verts);
162 CHKERR moab.get_coords(tris_verts, &tri_coords(0, 0));
167 std::array<Range, 3> edge_block;
168 for (
auto b : {1, 2, 3})
177 tris, PrismsFromSurfaceInterface::NO_SWAP, prisms);
179 Range add_prims_layer;
180 Range extrude_prisms = prisms;
185 extrude_prisms,
false, add_prims_layer);
186 prisms_from_surface_interface->
setThickness(add_prims_layer,
d3.data(),
188 extrude_prisms = add_prims_layer;
189 prisms.merge(add_prims_layer);
190 add_prims_layer.clear();
194 CHKERR moab.create_meshset(MESHSET_SET | MESHSET_TRACK_OWNER, meshset);
195 CHKERR moab.add_entities(meshset, prisms);
199 CHKERR moab.get_connectivity(prisms, verts);
201 CHKERR moab.get_coords(verts, &coords(0, 0));
203 for (
size_t v = 0;
v != verts.size(); ++
v)
207 CHKERR moab.create_meshset(MESHSET_SET | MESHSET_TRACK_OWNER,
210 std::array<double, 18> one_prism_coords = {0, 0, 0, 1, 0, 0, 0, 1, 0,
211 0, 0, 1, 1, 0, 1, 0, 1, 1};
212 std::array<EntityHandle, 6> one_prism_nodes;
213 for (
int n = 0;
n != 6; ++
n)
214 CHKERR moab.create_vertex(&one_prism_coords[3 *
n], one_prism_nodes[
n]);
216 CHKERR m_field.
get_moab().create_element(MBPRISM, one_prism_nodes.data(), 6,
218 Range one_prism_range;
219 one_prism_range.insert(one_prism);
220 CHKERR moab.add_entities(one_prism_meshset, one_prism_range);
221 Range one_prism_verts;
222 CHKERR moab.get_connectivity(one_prism_range, one_prism_verts);
223 CHKERR moab.add_entities(one_prism_meshset, one_prism_verts);
224 Range one_prism_adj_ents;
225 for (
int d = 1;
d != 3; ++
d)
226 CHKERR moab.get_adjacencies(one_prism_range,
d,
true, one_prism_adj_ents,
227 moab::Interface::UNION);
228 CHKERR moab.add_entities(one_prism_meshset, one_prism_adj_ents);
233 one_prism_range, bit_level0);
310 PrismFE fe_prism(m_field, tri_coords);
311 fe_prism.getOpPtrVector().push_back(
new PrismOp(moab, map_coords));
314 EdgeFE fe_edge(m_field, edge_block, one_prism);
315 fe_edge.getOpPtrVector().push_back(
317 moab, map_coords, one_prism));
320 TriFE fe_tri(m_field, tri_coords, one_prism);
321 fe_tri.getOpPtrVector().push_back(
323 moab, map_coords, one_prism));
326 QuadFE fe_quad(m_field, edge_block, one_prism);
327 fe_quad.getOpPtrVector().push_back(
329 moab, map_coords, one_prism));
332 CHKERR moab.write_file(
"prism_mesh.vtk",
"VTK",
"", &meshset, 1);
333 CHKERR moab.write_file(
"one_prism_mesh.vtk",
"VTK",
"", &one_prism_meshset,
◆ d3
constexpr std::array<double, 3> d3 = {0, 0, 0} |
|
staticconstexpr |
◆ d4
◆ delta
◆ help
◆ number_of_prisms_layers
constexpr int number_of_prisms_layers = 18 |
|
staticconstexpr |
◆ precision_exponent
constexpr int precision_exponent = 5 |
|
staticconstexpr |
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
virtual MoFEMErrorCode loop_finite_elements(const std::string problem_name, const std::string &fe_name, FEMethod &method, boost::shared_ptr< NumeredEntFiniteElement_multiIndex > fe_ptr=nullptr, MoFEMTypes bh=MF_EXIST, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr(), int verb=DEFAULT_VERBOSITY)=0
Make a loop over finite elements.
merge node from two bit levels
MoFEMErrorCode buildProblem(const std::string name, const bool square_matrix, int verb=VERBOSE)
build problem data structures
Problem manager is used to build and partition problems.
static constexpr std::array< double, 3 > d3
virtual MoFEMErrorCode modify_finite_element_add_field_row(const std::string &fe_name, const std::string name_row)=0
set field row which finite element use
UBlasMatrix< double > MatrixDouble
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
virtual MoFEMErrorCode add_ents_to_field_by_type(const Range &ents, const EntityType type, const std::string &name, int verb=DEFAULT_VERBOSITY)=0
Add entities to field meshset.
Deprecated interface functions.
DeprecatedCoreInterface Interface
static constexpr int number_of_prisms_layers
#define CHKERR
Inline error check.
virtual MoFEMErrorCode add_finite_element(const std::string &fe_name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
add finite element
virtual moab::Interface & get_moab()=0
virtual MoFEMErrorCode add_ents_to_finite_element_by_type(const EntityHandle entities, const EntityType type, const std::string name, const bool recursive=true)=0
add entities to finite element
virtual MoFEMErrorCode modify_finite_element_add_field_col(const std::string &fe_name, const std::string name_row)=0
set field col which finite element use
virtual MoFEMErrorCode build_finite_elements(int verb=DEFAULT_VERBOSITY)=0
Build finite elements.
MoFEMErrorCode getEntitiesByDimension(const int ms_id, const unsigned int cubit_bc_type, const int dimension, Range &entities, const bool recursive=true) const
get entities from CUBIT/meshset of a particular entity dimension
MoFEMErrorCode seedPrismsEntities(Range &prisms, const BitRefLevel &bit, int verb=-1)
Seed prism entities by bit level.
MoFEMErrorCode createPrismsFromPrisms(const Range &prisms, bool from_down, Range &out_prisms, int verb=-1)
Make prisms by extruding top or bottom prisms.
virtual MoFEMErrorCode modify_finite_element_add_field_data(const std::string &fe_name, const std::string name_field)=0
set finite element field data
MoFEMErrorCode partitionFiniteElements(const std::string name, bool part_from_moab=false, int low_proc=-1, int hi_proc=-1, int verb=VERBOSE)
partition finite elements
virtual MoFEMErrorCode modify_problem_ref_level_add_bit(const std::string &name_problem, const BitRefLevel &bit)=0
add ref level to problem
const double v
phase velocity of light in medium (cm/ns)
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
MoFEMErrorCode partitionSimpleProblem(const std::string name, int verb=VERBOSE)
partition problem dofs
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
PetscErrorCode PetscOptionsGetString(PetscOptions *, const char pre[], const char name[], char str[], size_t size, PetscBool *set)
Interface for managing meshsets containing materials and boundary conditions.
virtual MoFEMErrorCode build_fields(int verb=DEFAULT_VERBOSITY)=0
std::map< EntityHandle, EntityHandle > createdVertices
static constexpr std::array< double, 3 > d4
virtual MoFEMErrorCode modify_problem_add_finite_element(const std::string name_problem, const std::string &fe_name)=0
add finite element to problem, this add entities assigned to finite element to a particular problem
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
virtual MoFEMErrorCode build_adjacencies(const Range &ents, int verb=DEFAULT_VERBOSITY)=0
build adjacencies
MoFEMErrorCode createPrisms(const Range &ents, const SwapType swap_type, Range &prisms, int verb=-1)
Make prisms from triangles.
virtual MoFEMErrorCode set_field_order(const EntityHandle meshset, const EntityType type, const std::string &name, const ApproximationOrder order, int verb=DEFAULT_VERBOSITY)=0
Set order approximation of the entities in the field.
MoFEMErrorCode partitionGhostDofs(const std::string name, int verb=VERBOSE)
determine ghost nodes
virtual MoFEMErrorCode add_problem(const std::string &name, enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add problem.
virtual MoFEMErrorCode add_field(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_EXCL, int verb=DEFAULT_VERBOSITY)=0
Add field.
MoFEMErrorCode setThickness(const Range &prisms, const double director3[], const double director4[])
multi_index_container< CoordsAndHandle, indexed_by< hashed_unique< composite_key< CoordsAndHandle, member< CoordsAndHandle, int, &CoordsAndHandle::x >, member< CoordsAndHandle, int, &CoordsAndHandle::y >, member< CoordsAndHandle, int, &CoordsAndHandle::z > > > > > MapCoords