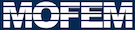 |
| v0.14.0
|
Go to the documentation of this file.
7 #ifndef __PRISMS_FORM_SURFACE_HPP__
8 #define __PRISMS_FORM_SURFACE_HPP__
53 Range &prisms,
int verb = -1);
80 Range &out_prisms,
int verb = -1);
90 const double director4[]);
124 #endif //__PRISMS_FORM_SURFACE_HPP__
merge node from two bit levels
SwapType
List of types of node swapping performed on a created prism Node swapping is required to satisfy the ...
PrismsFromSurfaceInterface(const MoFEM::Core &core)
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
implementation of Data Operators for Forces and Sources
MoFEMErrorCode updateMeshestByTriBlock(const Range &prisms)
Add prism to bockset.
MoFEMErrorCode seedPrismsEntities(Range &prisms, const BitRefLevel &bit, int verb=-1)
Seed prism entities by bit level.
MoFEMErrorCode createPrismsFromPrisms(const Range &prisms, bool from_down, Range &out_prisms, int verb=-1)
Make prisms by extruding top or bottom prisms.
base class for all interface classes
MoFEMErrorCode query_interface(boost::typeindex::type_index type_index, UnknownInterface **iface) const
std::map< EntityHandle, EntityHandle > createdVertices
MoFEMErrorCode updateMeshestByEdgeBlock(const Range &prisms)
Add quads to bockset.
std::bitset< BITREFLEVEL_SIZE > BitRefLevel
Bit structure attached to each entity identifying to what mesh entity is attached.
MoFEMErrorCode createPrisms(const Range &ents, const SwapType swap_type, Range &prisms, int verb=-1)
Make prisms from triangles.
MoFEMErrorCode setThickness(const Range &prisms, const double director3[], const double director4[])
MoFEMErrorCode setNormalThickness(const Range &prisms, double thickness3, double thickness4)