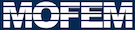 |
| v0.14.0
|
Go to the source code of this file.
|
int | main (int argc, char *argv[]) |
|
|
static char | help [] = "...\n\n" |
|
◆ EdgeEle
◆ EdgeEleOp
◆ FaceEleOnSide
◆ FaceEleOnSideOp
◆ main()
int main |
( |
int |
argc, |
|
|
char * |
argv[] |
|
) |
| |
- Examples
- continuity_check_on_skeleton_with_simple_2d_for_h1.cpp.
Definition at line 151 of file continuity_check_on_skeleton_with_simple_2d_for_h1.cpp.
166 auto core_log = logging::core::get();
168 LogManager::createSink(LogManager::getStrmSelf(),
"ATOM"));
169 LogManager::setLog(
"ATOM");
172 DMType dm_name =
"DMMOFEM";
188 enum bases { AINSWORTH, DEMKOWICZ, LASTBASEOP };
189 const char *list_bases[] = {
"ainsworth",
"demkowicz"};
191 PetscInt choice_base_value = AINSWORTH;
193 LASTBASEOP, &choice_base_value, &flg);
194 if (flg == PETSC_TRUE) {
196 if (choice_base_value == AINSWORTH)
198 else if (choice_base_value == DEMKOWICZ)
206 auto base = get_base();
217 auto dm = simple_interface->
getDM();
221 boost::shared_ptr<EdgeEle> skeleton_fe =
222 boost::shared_ptr<EdgeEle>(
new EdgeEle(m_field));
223 skeleton_fe->getOpPtrVector().push_back(
◆ help
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
const std::string getSkeletonFEName() const
Get the Skeleton FE Name.
MoFEMErrorCode loadFile(const std::string options, const std::string mesh_file_name, LoadFileFunc loadFunc=defaultLoadFileFunc)
Load mesh file.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
Simple interface for fast problem set-up.
Deprecated interface functions.
MoFEMErrorCode addSkeletonField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on skeleton.
DeprecatedCoreInterface Interface
MoFEMErrorCode getOptions()
get options
bool & getAddSkeletonFE()
Get the addSkeletonFE.
MoFEMErrorCode getDM(DM *dm)
Get DM.
#define CHKERR
Inline error check.
MoFEM::EdgeElementForcesAndSourcesCore EdgeEle
MoFEMErrorCode addDomainField(const std::string &name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on domain.
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
MoFEMErrorCode setFieldOrder(const std::string field_name, const int order, const Range *ents=NULL)
Set field order.
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
PetscErrorCode PetscOptionsGetEList(PetscOptions *, const char pre[], const char name[], const char *const *list, PetscInt next, PetscInt *value, PetscBool *set)
FieldApproximationBase
approximation base
MoFEMErrorCode setUp(const PetscBool is_partitioned=PETSC_TRUE)
Setup problem.
PetscErrorCode DMoFEMLoopFiniteElements(DM dm, const char fe_name[], MoFEM::FEMethod *method, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr())
Executes FEMethod for finite elements in DM.