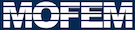 |
| v0.14.0
|
Go to the documentation of this file.
13 using namespace MoFEM;
15 static char help[] =
"...\n\n";
35 elemData(elem_data) {}
41 if (
type == MBEDGE && side == getEdgeSideNumber()) {
43 MatrixDouble diff = getCoordsAtGaussPts() - getEdgeCoordsAtGaussPts();
45 const double eps = 1e-12;
46 if (norm_inf(diff) >
eps) {
51 <<
"Quad coords: " << getCoordsAtGaussPts();
53 <<
"Edge coords: " << getEdgeCoordsAtGaussPts();
54 MOFEM_LOG(
"ATOM", Sev::error) <<
"Difference: " << diff;
56 "Coordinates at integration pts are different");
59 const size_t nb_dofs = data.
getN().size2();
60 const size_t nb_integration_pts = data.
getN().size1();
64 if (getFEMethod()->nInTheLoop == 0)
69 dot_elem_data.resize(nb_integration_pts, nb_dofs,
false);
71 for (
size_t gg = 0; gg != nb_integration_pts; ++gg) {
72 for (
size_t bb = 0; bb != nb_dofs; ++bb) {
73 dot_elem_data(gg, bb) = t_base;
87 faceSideFe(m_field), elemData(elem_data) {
97 const size_t nb_dofs = data.
getN().size2();
98 const size_t nb_integration_pts = data.
getN().size1();
101 <<
"Cords at integration points" << getCoordsAtGaussPts();
104 elemData.
dotEdge.resize(nb_integration_pts, nb_dofs,
false);
105 elemData.
dotEleLeft.resize(nb_integration_pts, 0,
false);
106 elemData.
dotEleRight.resize(nb_integration_pts, 0,
false);
108 for (
size_t gg = 0; gg != nb_integration_pts; ++gg) {
109 for (
size_t bb = 0; bb != nb_dofs; ++bb) {
110 elemData.
dotEdge(gg, bb) = t_base;
115 CHKERR loopSideFaces(
"dFE", faceSideFe);
117 auto check_continuity_of_base = [&](
auto &vol_dot_data) {
120 if (vol_dot_data.size1() != elemData.
dotEdge.size1())
122 "Inconsistent number of integration points");
124 if (vol_dot_data.size2() != elemData.
dotEdge.size2())
126 "Inconsistent number of base functions");
127 const double eps = 1e-12;
128 for (
size_t gg = 0; gg != vol_dot_data.size1(); ++gg)
129 for (
size_t bb = 0; bb != vol_dot_data.size2(); ++bb) {
131 std::abs(vol_dot_data(gg, bb) - elemData.
dotEdge(gg, bb));
134 "Inconsistency (%d, %d) %3.4e != %3.4e", gg, bb,
135 vol_dot_data(gg, bb), elemData.
dotEdge(gg, bb));
151 int main(
int argc,
char *argv[]) {
166 auto core_log = logging::core::get();
172 DMType dm_name =
"DMMOFEM";
188 enum bases { AINSWORTH, DEMKOWICZ, LASTBASEOP };
189 const char *list_bases[] = {
"ainsworth",
"demkowicz"};
191 PetscInt choice_base_value = AINSWORTH;
193 LASTBASEOP, &choice_base_value, &flg);
194 if (flg == PETSC_TRUE) {
196 if (choice_base_value == AINSWORTH)
198 else if (choice_base_value == DEMKOWICZ)
206 auto base = get_base();
217 auto dm = simple_interface->
getDM();
221 boost::shared_ptr<EdgeEle> skeleton_fe =
222 boost::shared_ptr<EdgeEle>(
new EdgeEle(m_field));
223 skeleton_fe->getOpPtrVector().push_back(
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode getInterface(IFACE *&iface) const
Get interface reference to pointer of interface.
Data on single entity (This is passed as argument to DataOperator::doWork)
PetscErrorCode MoFEMErrorCode
MoFEM/PETSc error code.
UBlasMatrix< double > MatrixDouble
const std::string getSkeletonFEName() const
Get the Skeleton FE Name.
#define MOFEM_LOG_ATTRIBUTES(channel, bit)
Add attributes to channel.
MoFEMErrorCode loadFile(const std::string options, const std::string mesh_file_name, LoadFileFunc loadFunc=defaultLoadFileFunc)
Load mesh file.
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
Operator for linear form, usually to calculate values on right hand side.
static boost::shared_ptr< SinkType > createSink(boost::shared_ptr< std::ostream > stream_ptr, std::string comm_filter)
Create a sink object.
Simple interface for fast problem set-up.
Deprecated interface functions.
MoFEMErrorCode doWork(int side, EntityType type, EntitiesFieldData::EntData &data)
Operator for linear form, usually to calculate values on right hand side.
DeprecatedCoreInterface Interface
FTensor::Tensor0< FTensor::PackPtr< double *, 1 > > getFTensor0N(const FieldApproximationBase base)
Get base function as Tensor0.
#define MOFEM_LOG_FUNCTION()
Set scope.
MoFEMErrorCode getOptions()
get options
bool & getAddSkeletonFE()
Get the addSkeletonFE.
MoFEMErrorCode getDM(DM *dm)
Get DM.
#define CHKERR
Inline error check.
friend class UserDataOperator
implementation of Data Operators for Forces and Sources
MoFEM::EdgeElementForcesAndSourcesCore EdgeEle
PetscErrorCode DMRegister_MoFEM(const char sname[])
Register MoFEM problem.
OpFaceSide(CommonData &elem_data)
static boost::shared_ptr< std::ostream > getStrmSelf()
Get the strm self object.
friend class UserDataOperator
MoFEMErrorCode setFieldOrder(const std::string field_name, const int order, const Range *ents=NULL)
Set field order.
default operator for EDGE element
SkeletonFE(MoFEM::Interface &m_field, CommonData &elem_data)
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
int main(int argc, char *argv[])
#define MOFEM_LOG(channel, severity)
Log.
#define CATCH_ERRORS
Catch errors.
MatrixDouble & getN(const FieldApproximationBase base)
get base functions this return matrix (nb. of rows is equal to nb. of Gauss pts, nb....
@ AINSWORTH_LEGENDRE_BASE
Ainsworth Cole (Legendre) approx. base .
boost::ptr_deque< UserDataOperator > & getOpPtrVector()
Use to push back operator for row operator.
PetscErrorCode PetscOptionsGetEList(PetscOptions *, const char pre[], const char name[], const char *const *list, PetscInt next, PetscInt *value, PetscBool *set)
default operator for Face element
FieldApproximationBase
approximation base
MoFEMErrorCode addSkeletonField(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on skeleton.
@ MOFEM_ATOM_TEST_INVALID
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
static LoggerType & setLog(const std::string channel)
Set ans resset chanel logger.
MoFEMErrorCode setUp(const PetscBool is_partitioned=PETSC_TRUE)
Setup problem.
PetscErrorCode DMoFEMLoopFiniteElements(DM dm, const char fe_name[], MoFEM::FEMethod *method, CacheTupleWeakPtr cache_ptr=CacheTupleSharedPtr())
Executes FEMethod for finite elements in DM.
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
MoFEMErrorCode addDomainField(const std::string name, const FieldSpace space, const FieldApproximationBase base, const FieldCoefficientsNumber nb_of_coefficients, const TagType tag_type=MB_TAG_SPARSE, const enum MoFEMTypes bh=MF_ZERO, int verb=-1)
Add field on domain.
Base face element used to integrate on skeleton.
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...