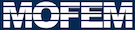 |
| v0.14.0
|
Go to the documentation of this file.
13 static PetscErrorCode
ierr;
16 double *diffL,
const int dim) {
29 for (
int d = 0;
d != dim; ++
d) {
30 diffL[
d * (p + 1) + 0] = 0;
43 for (
int d = 0;
d != dim; ++
d) {
44 diffL[
d * (p + 1) + 1] = diff_s[
d];
53 double B = ((
double)
l / ((
double)
l + 1));
54 L[
l + 1] =
A * s *
L[
l] - B *
L[
l - 1];
56 for (
int d = 0;
d != dim; ++
d) {
57 diffL[
d * (p + 1) +
l + 1] =
58 A * (diff_s[
d] *
L[
l] + s * diffL[
d * (p + 1) +
l]) -
59 B * diffL[
d * (p + 1) +
l - 1];
68 double *diff_x,
double *diff_t,
double *
L,
69 double *diffL,
const int dim) {
88 diffL[0 * (p + 1) + 0] = 0;
90 diffL[1 * (p + 1) + 0] = 0;
92 diffL[2 * (p + 1) + 0] = 0;
98 L[1] = 2 * x -
t + alpha * x;
101 for (;
d < dim; ++
d) {
102 double d_t = (diff_t) ? diff_t[
d] : 0;
103 diffL[
d * (p + 1) + 1] = (2 + alpha) * diff_x[
d] - d_t;
111 double a = 2 * lp1 * (lp1 + alpha) * (2 * lp1 + alpha - 2);
112 double b = 2 * lp1 + alpha - 1;
113 double c = (2 * lp1 + alpha) * (2 * lp1 + alpha - 2);
114 double d = 2 * (lp1 + alpha - 1) * (lp1 - 1) * (2 * lp1 + alpha);
115 double A = b * (
c * (2 * x -
t) + alpha * alpha *
t) /
a;
116 double B =
d *
t *
t /
a;
117 L[lp1] =
A *
L[
l] - B *
L[
l - 1];
120 for (; z < dim; ++z) {
121 double d_t = (diff_t) ? diff_t[z] : 0;
123 b * (
c * (2 * diff_x[z] - d_t) + alpha * alpha * d_t) /
a;
124 double diffB =
d * 2 *
t * d_t /
a;
125 diffL[z * (p + 1) + lp1] =
A * diffL[z * (p + 1) +
l] -
126 B * diffL[z * (p + 1) +
l - 1] +
127 diffA *
L[
l] - diffB *
L[
l - 1];
135 double t,
double *diff_x,
136 double *diff_t,
double *
L,
137 double *diffL,
const int dim) {
150 for (;
d != dim; ++
d) {
151 diffL[
d * p + 0] = diff_x[
d];
156 double jacobi[(p + 1)];
157 double diff_jacobi[(p + 1) * dim];
164 const double a = (
i + alpha) / ((2 *
i + alpha - 1) * (2 *
i + alpha));
165 const double b = alpha / ((2 *
i + alpha - 2) * (2 *
i + alpha));
166 const double c = (
i - 1) / ((2 *
i + alpha - 2) * (2 *
i + alpha - 1));
167 L[
l] =
a * jacobi[
i] + b *
t * jacobi[
i - 1] -
c *
t *
t * jacobi[
i - 2];
170 for (;
d != dim; ++
d) {
171 diffL[
d * p +
l] =
a * diff_jacobi[
d * (p + 1) +
i] +
172 b * (
t * diff_jacobi[
d * (p + 1) +
i - 1] +
173 diff_t[
d] * jacobi[
i - 1]) -
174 c * (
t *
t * diff_jacobi[
d * (p + 1) +
i - 2] +
175 2 *
t * diff_t[
d] * jacobi[
i - 2]);
183 double *diffL,
const int dim) {
200 for (
int d = 0;
d != dim; ++
d) {
201 diffL[
d * (p + 1) + 0] = 0;
207 if (diff_s == NULL) {
211 for (
int d = 0;
d != dim; ++
d) {
212 diffL[
d * (p + 1) + 1] = diff_s[
d];
217 for (
int k = 2;
k <= p;
k++) {
218 const double factor = 2 * (2 *
k - 1);
219 L[
k] = 1.0 / factor * (
l[
k] -
l[
k - 2]);
223 for (
int k = 2;
k <= p;
k++) {
224 double a =
l[
k - 1] / 2.;
225 for (
int d = 0;
d != dim; ++
d) {
226 diffL[
d * (p + 1) +
k] =
a * diff_s[
d];
329 double *
L,
double *diffL,
341 "Polynomial beyond order 9 is not implemented");
343 if (diff_s == NULL) {
351 for (;
l != p + 1;
l++) {
357 for (;
l != p + 1;
l++) {
360 for (;
d < dim; ++
d) {
361 diffL[
d * (p + 1) +
l] = diff_s[
d] *
a;
#define MoFEMFunctionReturnHot(a)
Last executable line of each PETSc function used for error handling. Replaces return()
static double f_phi5x(double x)
static double f_phi7(double x)
PetscErrorCode IntegratedJacobi_polynomials(int p, double alpha, double x, double t, double *diff_x, double *diff_t, double *L, double *diffL, const int dim)
Calculate integrated Jacobi approximation basis.
static double f_phi7x(double x)
static PetscErrorCode ierr
static double f_phi1x(double x)
static double f_phi4x(double x)
static double f_phi8x(double x)
PetscErrorCode Lobatto_polynomials(int p, double s, double *diff_s, double *L, double *diffL, const int dim)
Calculate Lobatto base functions .
const double c
speed of light (cm/ns)
static double f_phi3(double x)
PetscErrorCode Jacobi_polynomials(int p, double alpha, double x, double t, double *diff_x, double *diff_t, double *L, double *diffL, const int dim)
Calculate Jacobi approximation basis.
static double f_phi4(double x)
static double(* f_phi[])(double x)
static double f_phi8(double x)
static double f_phi2x(double x)
static double f_phi9(double x)
static double f_phi9x(double x)
static double f_phi0(double x)
PetscErrorCode Legendre_polynomials(int p, double s, double *diff_s, double *L, double *diffL, const int dim)
Calculate Legendre approximation basis.
useful compiler derivatives and definitions
constexpr double t
plate stiffness
static double f_phi6x(double x)
FTensor::Index< 'i', SPACE_DIM > i
static double f_phi3x(double x)
PetscErrorCode LobattoKernel_polynomials(int p, double s, double *diff_s, double *L, double *diffL, const int dim)
Calculate Kernel Lobatto base functions.
static double f_phi6(double x)
#define LOBATTO_PHI0(x)
Definitions taken from Hermes2d code.
static double f_phi1(double x)
static double f_phi0x(double x)
static double f_phi5(double x)
static double f_phi2(double x)
#define MoFEMFunctionBeginHot
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...
FTensor::Index< 'k', 3 > k
static double(* f_phix[])(double x)
FTensor::Index< 'l', 3 > l
#define LOBATTO_PHI0X(x)
Derivatives of kernel functions for Lobbatto base.