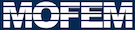 |
| v0.14.0
|
Go to the documentation of this file.
10 using namespace MoFEM;
12 static char help[] =
"...\n\n";
14 int main(
int argc,
char *argv[]) {
23 auto check = [&](
const int expected) {
27 "use count should be %d but is %d", expected,
35 CHKERR MatCreate(PETSC_COMM_SELF, &
m);
40 { std::ignore =
static_cast<PetscObject
>(m_ptr); }
50 CHKERR MatSetSizes(m_ptr, 2, 2, 2, 2);
static MoFEMErrorCode Finalize()
Checks for options to be called at the conclusion of the program.
int main(int argc, char *argv[])
#define CHKERR
Inline error check.
implementation of Data Operators for Forces and Sources
static MoFEMErrorCode Initialize(int *argc, char ***args, const char file[], const char help[])
Initializes the MoFEM database PETSc, MOAB and MPI.
#define CATCH_ERRORS
Catch errors.
@ MOFEM_DATA_INCONSISTENCY
FTensor::Index< 'm', 3 > m
#define MoFEMFunctionReturn(a)
Last executable line of each PETSc function used for error handling. Replaces return()
#define MoFEMFunctionBegin
First executable line of each MoFEM function, used for error handling. Final line of MoFEM functions ...