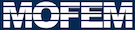 |
| v0.14.0
|
#include <src/interfaces/Lie.hpp>
|
template<typename T > |
static auto | getVee (T &&w1, T &&w2, T &&w3) |
|
template<typename T > |
static auto | getHat (T &&w1, T &&w2, T &&w3) |
|
template<typename A > |
static auto | getVee (A &&t_w_hat) |
|
template<typename A > |
static auto | getHat (A &&t_w_vee) |
|
template<typename A , typename B > |
static auto | exp (A &&t_w_vee, B &&theta) |
|
template<typename A , typename B > |
static auto | Jl (A &&t_w_vee, B &&theta) |
|
template<typename A , typename B > |
static auto | Jr (A &&t_w_vee, B &&theta) |
|
template<typename A , typename B , typename C > |
static auto | action (A &&t_w_vee, B &&theta, C &&t_A) |
|
template<typename A , typename B > |
static auto | dActionJl (A &&t_w_vee, B &&t_A) |
|
template<typename A , typename B > |
static auto | dActionJr (A &&t_w_vee, B &&t_A) |
|
template<typename A , typename B > |
static auto | diffExp (A &&t_w_vee, B &&theta) |
|
|
static | FTENSOR_INDEX (dim, i) |
|
static | FTENSOR_INDEX (dim, j) |
|
static | FTENSOR_INDEX (dim, k) |
|
static | FTENSOR_INDEX (dim, l) |
|
static | FTENSOR_INDEX (dim, m) |
|
static | FTENSOR_INDEX (dim, n) |
|
template<typename T > |
static auto | getVeeImpl (FTensor::Tensor2< T, dim, dim > &t_w_hat) |
|
template<typename T > |
static auto | getHatImpl (FTensor::Tensor1< T, dim > &t_w_vee) |
|
template<typename T1 , typename T2 , typename T3 > |
static auto | genericFormImpl (FTensor::Tensor1< T1, dim > &t_w_vee, const T2 alpha, const T3 beta) |
|
template<typename T1 , typename T2 > |
static auto | expImpl (FTensor::Tensor1< T1, dim > &t_w_vee, const T2 theta) |
|
template<typename T1 , typename T2 > |
static auto | diffExpImpl (FTensor::Tensor1< T1, dim > &t_w_vee, const T2 theta) |
|
template<typename T1 , typename T2 > |
static auto | JlImpl (FTensor::Tensor1< T1, dim > &t_w_vee, const T2 &theta) |
|
template<typename T1 , typename T2 > |
static auto | JrImpl (FTensor::Tensor1< T1, dim > &t_w_vee, const T2 theta) |
|
template<typename T1 , typename T2 , typename T3 > |
static auto | actionImpl (FTensor::Tensor1< T1, dim > &t_w_vee, const T2 theta, FTensor::Tensor2_symmetric< T3, dim > &t_A) |
|
|
static constexpr int | dim = 3 |
|
Definition at line 23 of file Lie.hpp.
◆ SO3()
◆ ~SO3()
◆ action()
template<typename A , typename B , typename C >
static auto LieGroups::SO3::action |
( |
A && |
t_w_vee, |
|
|
B && |
theta, |
|
|
C && |
t_A |
|
) |
| |
|
inlinestatic |
Definition at line 64 of file Lie.hpp.
65 return actionImpl(std::forward<A>(t_w_vee), std::forward<B>(theta),
66 std::forward<C>(t_A));
◆ actionImpl()
template<typename T1 , typename T2 , typename T3 >
◆ dActionJl()
template<typename A , typename B >
static auto LieGroups::SO3::dActionJl |
( |
A && |
t_w_vee, |
|
|
B && |
t_A |
|
) |
| |
|
inlinestatic |
Definition at line 70 of file Lie.hpp.
71 return dActionJlImpl(std::forward<A>(t_w_vee), std::forward<B>(t_A));
◆ dActionJr()
template<typename A , typename B >
static auto LieGroups::SO3::dActionJr |
( |
A && |
t_w_vee, |
|
|
B && |
t_A |
|
) |
| |
|
inlinestatic |
Definition at line 75 of file Lie.hpp.
76 return dActionJrImpl(std::forward<A>(t_w_vee), std::forward<B>(t_A));
◆ diffExp()
template<typename A , typename B >
static auto LieGroups::SO3::diffExp |
( |
A && |
t_w_vee, |
|
|
B && |
theta |
|
) |
| |
|
inlinestatic |
◆ diffExpImpl()
template<typename T1 , typename T2 >
static auto LieGroups::SO3::diffExpImpl |
( |
FTensor::Tensor1< T1, dim > & |
t_w_vee, |
|
|
const T2 |
theta |
|
) |
| |
|
inlinestaticprivate |
Definition at line 134 of file Lie.hpp.
137 auto get_tensor = [&t_w_vee](
auto a,
auto diff_a,
auto b,
auto diff_b) {
145 auto t_hat =
getHat(t_w_vee);
146 t_diff_exp(
i,
j,
k) =
148 a * FTensor::levi_civita<int>(
i,
j,
k)
152 diff_a * t_hat(
i,
j) * t_w_vee(
k)
156 b * (t_hat(
i,
l) * FTensor::levi_civita<int>(
l,
j,
k) +
157 FTensor::levi_civita<int>(
i,
l,
k) * t_hat(
l,
j))
161 diff_b * t_hat(
i,
l) * t_hat(
l,
j) * t_w_vee(
k);
166 if(std::fabs(theta) < std::numeric_limits<T2>::epsilon()){
167 return get_tensor(1., -1. / 3., 0., 1. / 1.2);
170 const auto ss = sin(theta);
171 const auto a = ss / theta;
173 const auto theta2 = theta * theta;
174 const auto cc = cos(theta);
175 const auto diff_a = (theta * cc - ss) / (theta2 * theta);
177 const auto ss_2 = sin(theta / 2.);
178 const auto cc_2 = cos(theta / 2.);
179 const auto b = 2. * ss_2 * ss_2 / theta2;
181 (2. * theta * ss_2 * cc_2 - 4. * ss_2 * ss_2) / (theta2 * theta2);
183 return get_tensor(
a, diff_a, b, diff_b);
◆ exp()
template<typename A , typename B >
static auto LieGroups::SO3::exp |
( |
A && |
t_w_vee, |
|
|
B && |
theta |
|
) |
| |
|
inlinestatic |
◆ expImpl()
template<typename T1 , typename T2 >
Definition at line 121 of file Lie.hpp.
123 if (std::fabs(theta) < std::numeric_limits<T2>::epsilon()) {
126 const auto s = sin(theta);
127 const auto s_half = sin(theta / 2);
128 const auto a = s / theta;
129 const auto b = 2 * (s_half / theta) * (s_half / theta);
◆ FTENSOR_INDEX() [1/6]
static LieGroups::SO3::FTENSOR_INDEX |
( |
dim |
, |
|
|
i |
|
|
) |
| |
|
inlinestaticprivate |
◆ FTENSOR_INDEX() [2/6]
static LieGroups::SO3::FTENSOR_INDEX |
( |
dim |
, |
|
|
j |
|
|
) |
| |
|
inlinestaticprivate |
◆ FTENSOR_INDEX() [3/6]
static LieGroups::SO3::FTENSOR_INDEX |
( |
dim |
, |
|
|
k |
|
|
) |
| |
|
inlinestaticprivate |
◆ FTENSOR_INDEX() [4/6]
static LieGroups::SO3::FTENSOR_INDEX |
( |
dim |
, |
|
|
l |
|
|
) |
| |
|
inlinestaticprivate |
◆ FTENSOR_INDEX() [5/6]
static LieGroups::SO3::FTENSOR_INDEX |
( |
dim |
, |
|
|
m |
|
|
) |
| |
|
inlinestaticprivate |
◆ FTENSOR_INDEX() [6/6]
static LieGroups::SO3::FTENSOR_INDEX |
( |
dim |
, |
|
|
n |
|
|
) |
| |
|
inlinestaticprivate |
◆ genericFormImpl()
template<typename T1 , typename T2 , typename T3 >
static auto LieGroups::SO3::genericFormImpl |
( |
FTensor::Tensor1< T1, dim > & |
t_w_vee, |
|
|
const T2 |
alpha, |
|
|
const T3 |
beta |
|
) |
| |
|
inlinestaticprivate |
Definition at line 110 of file Lie.hpp.
114 auto t_hat =
getHat(t_w_vee);
116 beta * (t_hat(
i,
k) * t_hat(
k,
j));
◆ getHat() [1/2]
template<typename A >
static auto LieGroups::SO3::getHat |
( |
A && |
t_w_vee | ) |
|
|
inlinestatic |
◆ getHat() [2/2]
template<typename T >
static auto LieGroups::SO3::getHat |
( |
T && |
w1, |
|
|
T && |
w2, |
|
|
T && |
w3 |
|
) |
| |
|
inlinestatic |
◆ getHatImpl()
◆ getVee() [1/2]
template<typename A >
static auto LieGroups::SO3::getVee |
( |
A && |
t_w_hat | ) |
|
|
inlinestatic |
◆ getVee() [2/2]
template<typename T >
static auto LieGroups::SO3::getVee |
( |
T && |
w1, |
|
|
T && |
w2, |
|
|
T && |
w3 |
|
) |
| |
|
inlinestatic |
Definition at line 28 of file Lie.hpp.
31 std::forward<T>(w1), std::forward<T>(w2), std::forward<T>(w3)
◆ getVeeImpl()
◆ Jl()
template<typename A , typename B >
static auto LieGroups::SO3::Jl |
( |
A && |
t_w_vee, |
|
|
B && |
theta |
|
) |
| |
|
inlinestatic |
Definition at line 54 of file Lie.hpp.
55 return JlImpl(std::forward<A>(t_w_vee), std::forward<B>(theta));
◆ JlImpl()
template<typename T1 , typename T2 >
Definition at line 187 of file Lie.hpp.
189 if (std::fabs(theta) < std::numeric_limits<T2>::epsilon()) {
192 const auto s = sin(theta);
193 const auto s_half = sin(theta / 2);
194 const auto a = 2 * (s_half / theta) * (s_half / theta);
195 const auto b = ((theta - s) / theta) / theta / theta;
◆ Jr()
template<typename A , typename B >
static auto LieGroups::SO3::Jr |
( |
A && |
t_w_vee, |
|
|
B && |
theta |
|
) |
| |
|
inlinestatic |
Definition at line 59 of file Lie.hpp.
60 return JrImpl(std::forward<A>(t_w_vee), std::forward<B>(theta));
◆ JrImpl()
template<typename T1 , typename T2 >
Definition at line 200 of file Lie.hpp.
202 if (std::fabs(theta) < std::numeric_limits<T2>::epsilon()) {
205 const auto s = sin(theta);
206 const auto s_half = sin(theta / 2);
207 const auto a = 2 * (s_half / theta) * (s_half / theta);
208 const auto b = ((theta - s) / theta) / theta / theta;
◆ dim
constexpr int LieGroups::SO3::dim = 3 |
|
inlinestaticconstexprprivate |
The documentation for this struct was generated from the following file:
static auto actionImpl(FTensor::Tensor1< T1, dim > &t_w_vee, const T2 theta, FTensor::Tensor2_symmetric< T3, dim > &t_A)
static auto getVeeImpl(FTensor::Tensor2< T, dim, dim > &t_w_hat)
static auto getHat(T &&w1, T &&w2, T &&w3)
constexpr std::enable_if<(Dim0<=2 &&Dim1<=2), Tensor2_Expr< Levi_Civita< T >, T, Dim0, Dim1, i, j > >::type levi_civita(const Index< i, Dim0 > &, const Index< j, Dim1 > &)
levi_civita functions to make for easy adhoc use
static auto exp(A &&t_w_vee, B &&theta)
static auto diffExpImpl(FTensor::Tensor1< T1, dim > &t_w_vee, const T2 theta)
static auto expImpl(FTensor::Tensor1< T1, dim > &t_w_vee, const T2 theta)
static auto getVee(T &&w1, T &&w2, T &&w3)
FTensor::Index< 'i', SPACE_DIM > i
static auto genericFormImpl(FTensor::Tensor1< T1, dim > &t_w_vee, const T2 alpha, const T3 beta)
static auto JrImpl(FTensor::Tensor1< T1, dim > &t_w_vee, const T2 theta)
FTensor::Index< 'j', 3 > j
static auto getHatImpl(FTensor::Tensor1< T, dim > &t_w_vee)
static FTENSOR_INDEX(dim, i)
const double D
diffusivity
static auto JlImpl(FTensor::Tensor1< T1, dim > &t_w_vee, const T2 &theta)
FTensor::Index< 'k', 3 > k
FTensor::Index< 'l', 3 > l